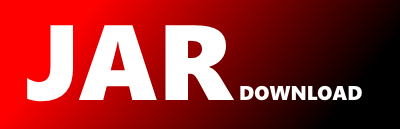
com.pulumi.gcp.alloydb.kotlin.ClusterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.alloydb.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.alloydb.ClusterArgs.builder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterAutomatedBackupPolicyArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterAutomatedBackupPolicyArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterContinuousBackupConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterContinuousBackupConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterEncryptionConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterEncryptionConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterInitialUserArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterInitialUserArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterMaintenanceUpdatePolicyArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterMaintenanceUpdatePolicyArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterNetworkConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterNetworkConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterRestoreBackupSourceArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterRestoreBackupSourceArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterRestoreContinuousBackupSourceArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterRestoreContinuousBackupSourceArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterSecondaryConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.ClusterSecondaryConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A managed alloydb cluster.
* To get more information about Cluster, see:
* * [API documentation](https://cloud.google.com/alloydb/docs/reference/rest/v1/projects.locations.clusters/create)
* * How-to Guides
* * [AlloyDB](https://cloud.google.com/alloydb/docs/)
* > **Note:** Users can promote a secondary cluster to a primary cluster with the help of `cluster_type`.
* To promote, users have to set the `cluster_type` property as `PRIMARY` and remove the `secondary_config` field from cluster configuration.
* See Example.
* ## Example Usage
* ### Alloydb Cluster Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultNetwork = new gcp.compute.Network("default", {name: "alloydb-cluster"});
* const _default = new gcp.alloydb.Cluster("default", {
* clusterId: "alloydb-cluster",
* location: "us-central1",
* network: defaultNetwork.id,
* });
* const project = gcp.organizations.getProject({});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_network = gcp.compute.Network("default", name="alloydb-cluster")
* default = gcp.alloydb.Cluster("default",
* cluster_id="alloydb-cluster",
* location="us-central1",
* network=default_network.id)
* project = gcp.organizations.get_project()
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultNetwork = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-cluster",
* });
* var @default = new Gcp.Alloydb.Cluster("default", new()
* {
* ClusterId = "alloydb-cluster",
* Location = "us-central1",
* Network = defaultNetwork.Id,
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultNetwork, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-cluster"),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "default", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-cluster"),
* Location: pulumi.String("us-central1"),
* Network: defaultNetwork.ID(),
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultNetwork = new Network("defaultNetwork", NetworkArgs.builder()
* .name("alloydb-cluster")
* .build());
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterId("alloydb-cluster")
* .location("us-central1")
* .network(defaultNetwork.id())
* .build());
* final var project = OrganizationsFunctions.getProject();
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-cluster
* location: us-central1
* network: ${defaultNetwork.id}
* defaultNetwork:
* type: gcp:compute:Network
* name: default
* properties:
* name: alloydb-cluster
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Alloydb Cluster Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {name: "alloydb-cluster-full"});
* const full = new gcp.alloydb.Cluster("full", {
* clusterId: "alloydb-cluster-full",
* location: "us-central1",
* network: _default.id,
* databaseVersion: "POSTGRES_15",
* initialUser: {
* user: "alloydb-cluster-full",
* password: "alloydb-cluster-full",
* },
* continuousBackupConfig: {
* enabled: true,
* recoveryWindowDays: 14,
* },
* automatedBackupPolicy: {
* location: "us-central1",
* backupWindow: "1800s",
* enabled: true,
* weeklySchedule: {
* daysOfWeeks: ["MONDAY"],
* startTimes: [{
* hours: 23,
* minutes: 0,
* seconds: 0,
* nanos: 0,
* }],
* },
* quantityBasedRetention: {
* count: 1,
* },
* labels: {
* test: "alloydb-cluster-full",
* },
* },
* labels: {
* test: "alloydb-cluster-full",
* },
* });
* const project = gcp.organizations.getProject({});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default", name="alloydb-cluster-full")
* full = gcp.alloydb.Cluster("full",
* cluster_id="alloydb-cluster-full",
* location="us-central1",
* network=default.id,
* database_version="POSTGRES_15",
* initial_user=gcp.alloydb.ClusterInitialUserArgs(
* user="alloydb-cluster-full",
* password="alloydb-cluster-full",
* ),
* continuous_backup_config=gcp.alloydb.ClusterContinuousBackupConfigArgs(
* enabled=True,
* recovery_window_days=14,
* ),
* automated_backup_policy=gcp.alloydb.ClusterAutomatedBackupPolicyArgs(
* location="us-central1",
* backup_window="1800s",
* enabled=True,
* weekly_schedule=gcp.alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleArgs(
* days_of_weeks=["MONDAY"],
* start_times=[gcp.alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs(
* hours=23,
* minutes=0,
* seconds=0,
* nanos=0,
* )],
* ),
* quantity_based_retention=gcp.alloydb.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs(
* count=1,
* ),
* labels={
* "test": "alloydb-cluster-full",
* },
* ),
* labels={
* "test": "alloydb-cluster-full",
* })
* project = gcp.organizations.get_project()
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-cluster-full",
* });
* var full = new Gcp.Alloydb.Cluster("full", new()
* {
* ClusterId = "alloydb-cluster-full",
* Location = "us-central1",
* Network = @default.Id,
* DatabaseVersion = "POSTGRES_15",
* InitialUser = new Gcp.Alloydb.Inputs.ClusterInitialUserArgs
* {
* User = "alloydb-cluster-full",
* Password = "alloydb-cluster-full",
* },
* ContinuousBackupConfig = new Gcp.Alloydb.Inputs.ClusterContinuousBackupConfigArgs
* {
* Enabled = true,
* RecoveryWindowDays = 14,
* },
* AutomatedBackupPolicy = new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyArgs
* {
* Location = "us-central1",
* BackupWindow = "1800s",
* Enabled = true,
* WeeklySchedule = new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyWeeklyScheduleArgs
* {
* DaysOfWeeks = new[]
* {
* "MONDAY",
* },
* StartTimes = new[]
* {
* new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs
* {
* Hours = 23,
* Minutes = 0,
* Seconds = 0,
* Nanos = 0,
* },
* },
* },
* QuantityBasedRetention = new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs
* {
* Count = 1,
* },
* Labels =
* {
* { "test", "alloydb-cluster-full" },
* },
* },
* Labels =
* {
* { "test", "alloydb-cluster-full" },
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-cluster-full"),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "full", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-cluster-full"),
* Location: pulumi.String("us-central1"),
* Network: _default.ID(),
* DatabaseVersion: pulumi.String("POSTGRES_15"),
* InitialUser: &alloydb.ClusterInitialUserArgs{
* User: pulumi.String("alloydb-cluster-full"),
* Password: pulumi.String("alloydb-cluster-full"),
* },
* ContinuousBackupConfig: &alloydb.ClusterContinuousBackupConfigArgs{
* Enabled: pulumi.Bool(true),
* RecoveryWindowDays: pulumi.Int(14),
* },
* AutomatedBackupPolicy: &alloydb.ClusterAutomatedBackupPolicyArgs{
* Location: pulumi.String("us-central1"),
* BackupWindow: pulumi.String("1800s"),
* Enabled: pulumi.Bool(true),
* WeeklySchedule: &alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleArgs{
* DaysOfWeeks: pulumi.StringArray{
* pulumi.String("MONDAY"),
* },
* StartTimes: alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArray{
* &alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs{
* Hours: pulumi.Int(23),
* Minutes: pulumi.Int(0),
* Seconds: pulumi.Int(0),
* Nanos: pulumi.Int(0),
* },
* },
* },
* QuantityBasedRetention: &alloydb.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs{
* Count: pulumi.Int(1),
* },
* Labels: pulumi.StringMap{
* "test": pulumi.String("alloydb-cluster-full"),
* },
* },
* Labels: pulumi.StringMap{
* "test": pulumi.String("alloydb-cluster-full"),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterInitialUserArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterAutomatedBackupPolicyArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterAutomatedBackupPolicyWeeklyScheduleArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("alloydb-cluster-full")
* .build());
* var full = new Cluster("full", ClusterArgs.builder()
* .clusterId("alloydb-cluster-full")
* .location("us-central1")
* .network(default_.id())
* .databaseVersion("POSTGRES_15")
* .initialUser(ClusterInitialUserArgs.builder()
* .user("alloydb-cluster-full")
* .password("alloydb-cluster-full")
* .build())
* .continuousBackupConfig(ClusterContinuousBackupConfigArgs.builder()
* .enabled(true)
* .recoveryWindowDays(14)
* .build())
* .automatedBackupPolicy(ClusterAutomatedBackupPolicyArgs.builder()
* .location("us-central1")
* .backupWindow("1800s")
* .enabled(true)
* .weeklySchedule(ClusterAutomatedBackupPolicyWeeklyScheduleArgs.builder()
* .daysOfWeeks("MONDAY")
* .startTimes(ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs.builder()
* .hours(23)
* .minutes(0)
* .seconds(0)
* .nanos(0)
* .build())
* .build())
* .quantityBasedRetention(ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs.builder()
* .count(1)
* .build())
* .labels(Map.of("test", "alloydb-cluster-full"))
* .build())
* .labels(Map.of("test", "alloydb-cluster-full"))
* .build());
* final var project = OrganizationsFunctions.getProject();
* }
* }
* ```
* ```yaml
* resources:
* full:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-cluster-full
* location: us-central1
* network: ${default.id}
* databaseVersion: POSTGRES_15
* initialUser:
* user: alloydb-cluster-full
* password: alloydb-cluster-full
* continuousBackupConfig:
* enabled: true
* recoveryWindowDays: 14
* automatedBackupPolicy:
* location: us-central1
* backupWindow: 1800s
* enabled: true
* weeklySchedule:
* daysOfWeeks:
* - MONDAY
* startTimes:
* - hours: 23
* minutes: 0
* seconds: 0
* nanos: 0
* quantityBasedRetention:
* count: 1
* labels:
* test: alloydb-cluster-full
* labels:
* test: alloydb-cluster-full
* default:
* type: gcp:compute:Network
* properties:
* name: alloydb-cluster-full
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Alloydb Cluster Restore
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const default = gcp.compute.getNetwork({
* name: "alloydb-network",
* });
* const source = new gcp.alloydb.Cluster("source", {
* clusterId: "alloydb-source-cluster",
* location: "us-central1",
* network: _default.then(_default => _default.id),
* initialUser: {
* password: "alloydb-source-cluster",
* },
* });
* const sourceInstance = new gcp.alloydb.Instance("source", {
* cluster: source.name,
* instanceId: "alloydb-instance",
* instanceType: "PRIMARY",
* machineConfig: {
* cpuCount: 2,
* },
* });
* const sourceBackup = new gcp.alloydb.Backup("source", {
* backupId: "alloydb-backup",
* location: "us-central1",
* clusterName: source.name,
* });
* const restoredFromBackup = new gcp.alloydb.Cluster("restored_from_backup", {
* clusterId: "alloydb-backup-restored",
* location: "us-central1",
* network: _default.then(_default => _default.id),
* restoreBackupSource: {
* backupName: sourceBackup.name,
* },
* });
* const restoredViaPitr = new gcp.alloydb.Cluster("restored_via_pitr", {
* clusterId: "alloydb-pitr-restored",
* location: "us-central1",
* network: _default.then(_default => _default.id),
* restoreContinuousBackupSource: {
* cluster: source.name,
* pointInTime: "2023-08-03T19:19:00.094Z",
* },
* });
* const project = gcp.organizations.getProject({});
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "alloydb-source-cluster",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: _default.then(_default => _default.id),
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: _default.then(_default => _default.id),
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.get_network(name="alloydb-network")
* source = gcp.alloydb.Cluster("source",
* cluster_id="alloydb-source-cluster",
* location="us-central1",
* network=default.id,
* initial_user=gcp.alloydb.ClusterInitialUserArgs(
* password="alloydb-source-cluster",
* ))
* source_instance = gcp.alloydb.Instance("source",
* cluster=source.name,
* instance_id="alloydb-instance",
* instance_type="PRIMARY",
* machine_config=gcp.alloydb.InstanceMachineConfigArgs(
* cpu_count=2,
* ))
* source_backup = gcp.alloydb.Backup("source",
* backup_id="alloydb-backup",
* location="us-central1",
* cluster_name=source.name)
* restored_from_backup = gcp.alloydb.Cluster("restored_from_backup",
* cluster_id="alloydb-backup-restored",
* location="us-central1",
* network=default.id,
* restore_backup_source=gcp.alloydb.ClusterRestoreBackupSourceArgs(
* backup_name=source_backup.name,
* ))
* restored_via_pitr = gcp.alloydb.Cluster("restored_via_pitr",
* cluster_id="alloydb-pitr-restored",
* location="us-central1",
* network=default.id,
* restore_continuous_backup_source=gcp.alloydb.ClusterRestoreContinuousBackupSourceArgs(
* cluster=source.name,
* point_in_time="2023-08-03T19:19:00.094Z",
* ))
* project = gcp.organizations.get_project()
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="alloydb-source-cluster",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = Gcp.Compute.GetNetwork.Invoke(new()
* {
* Name = "alloydb-network",
* });
* var source = new Gcp.Alloydb.Cluster("source", new()
* {
* ClusterId = "alloydb-source-cluster",
* Location = "us-central1",
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* InitialUser = new Gcp.Alloydb.Inputs.ClusterInitialUserArgs
* {
* Password = "alloydb-source-cluster",
* },
* });
* var sourceInstance = new Gcp.Alloydb.Instance("source", new()
* {
* Cluster = source.Name,
* InstanceId = "alloydb-instance",
* InstanceType = "PRIMARY",
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* });
* var sourceBackup = new Gcp.Alloydb.Backup("source", new()
* {
* BackupId = "alloydb-backup",
* Location = "us-central1",
* ClusterName = source.Name,
* });
* var restoredFromBackup = new Gcp.Alloydb.Cluster("restored_from_backup", new()
* {
* ClusterId = "alloydb-backup-restored",
* Location = "us-central1",
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* RestoreBackupSource = new Gcp.Alloydb.Inputs.ClusterRestoreBackupSourceArgs
* {
* BackupName = sourceBackup.Name,
* },
* });
* var restoredViaPitr = new Gcp.Alloydb.Cluster("restored_via_pitr", new()
* {
* ClusterId = "alloydb-pitr-restored",
* Location = "us-central1",
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* RestoreContinuousBackupSource = new Gcp.Alloydb.Inputs.ClusterRestoreContinuousBackupSourceArgs
* {
* Cluster = source.Name,
* PointInTime = "2023-08-03T19:19:00.094Z",
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "alloydb-source-cluster",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _default, err := compute.LookupNetwork(ctx, &compute.LookupNetworkArgs{
* Name: "alloydb-network",
* }, nil)
* if err != nil {
* return err
* }
* source, err := alloydb.NewCluster(ctx, "source", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-source-cluster"),
* Location: pulumi.String("us-central1"),
* Network: pulumi.String(_default.Id),
* InitialUser: &alloydb.ClusterInitialUserArgs{
* Password: pulumi.String("alloydb-source-cluster"),
* },
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewInstance(ctx, "source", &alloydb.InstanceArgs{
* Cluster: source.Name,
* InstanceId: pulumi.String("alloydb-instance"),
* InstanceType: pulumi.String("PRIMARY"),
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* })
* if err != nil {
* return err
* }
* sourceBackup, err := alloydb.NewBackup(ctx, "source", &alloydb.BackupArgs{
* BackupId: pulumi.String("alloydb-backup"),
* Location: pulumi.String("us-central1"),
* ClusterName: source.Name,
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "restored_from_backup", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-backup-restored"),
* Location: pulumi.String("us-central1"),
* Network: pulumi.String(_default.Id),
* RestoreBackupSource: &alloydb.ClusterRestoreBackupSourceArgs{
* BackupName: sourceBackup.Name,
* },
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "restored_via_pitr", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-pitr-restored"),
* Location: pulumi.String("us-central1"),
* Network: pulumi.String(_default.Id),
* RestoreContinuousBackupSource: &alloydb.ClusterRestoreContinuousBackupSourceArgs{
* Cluster: source.Name,
* PointInTime: pulumi.String("2023-08-03T19:19:00.094Z"),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("alloydb-source-cluster"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: pulumi.String(_default.Id),
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: pulumi.String(_default.Id),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetNetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterInitialUserArgs;
* import com.pulumi.gcp.alloydb.Instance;
* import com.pulumi.gcp.alloydb.InstanceArgs;
* import com.pulumi.gcp.alloydb.inputs.InstanceMachineConfigArgs;
* import com.pulumi.gcp.alloydb.Backup;
* import com.pulumi.gcp.alloydb.BackupArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterRestoreBackupSourceArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterRestoreContinuousBackupSourceArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var default = ComputeFunctions.getNetwork(GetNetworkArgs.builder()
* .name("alloydb-network")
* .build());
* var source = new Cluster("source", ClusterArgs.builder()
* .clusterId("alloydb-source-cluster")
* .location("us-central1")
* .network(default_.id())
* .initialUser(ClusterInitialUserArgs.builder()
* .password("alloydb-source-cluster")
* .build())
* .build());
* var sourceInstance = new Instance("sourceInstance", InstanceArgs.builder()
* .cluster(source.name())
* .instanceId("alloydb-instance")
* .instanceType("PRIMARY")
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build());
* var sourceBackup = new Backup("sourceBackup", BackupArgs.builder()
* .backupId("alloydb-backup")
* .location("us-central1")
* .clusterName(source.name())
* .build());
* var restoredFromBackup = new Cluster("restoredFromBackup", ClusterArgs.builder()
* .clusterId("alloydb-backup-restored")
* .location("us-central1")
* .network(default_.id())
* .restoreBackupSource(ClusterRestoreBackupSourceArgs.builder()
* .backupName(sourceBackup.name())
* .build())
* .build());
* var restoredViaPitr = new Cluster("restoredViaPitr", ClusterArgs.builder()
* .clusterId("alloydb-pitr-restored")
* .location("us-central1")
* .network(default_.id())
* .restoreContinuousBackupSource(ClusterRestoreContinuousBackupSourceArgs.builder()
* .cluster(source.name())
* .pointInTime("2023-08-03T19:19:00.094Z")
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("alloydb-source-cluster")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* source:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-source-cluster
* location: us-central1
* network: ${default.id}
* initialUser:
* password: alloydb-source-cluster
* sourceInstance:
* type: gcp:alloydb:Instance
* name: source
* properties:
* cluster: ${source.name}
* instanceId: alloydb-instance
* instanceType: PRIMARY
* machineConfig:
* cpuCount: 2
* sourceBackup:
* type: gcp:alloydb:Backup
* name: source
* properties:
* backupId: alloydb-backup
* location: us-central1
* clusterName: ${source.name}
* restoredFromBackup:
* type: gcp:alloydb:Cluster
* name: restored_from_backup
* properties:
* clusterId: alloydb-backup-restored
* location: us-central1
* network: ${default.id}
* restoreBackupSource:
* backupName: ${sourceBackup.name}
* restoredViaPitr:
* type: gcp:alloydb:Cluster
* name: restored_via_pitr
* properties:
* clusterId: alloydb-pitr-restored
* location: us-central1
* network: ${default.id}
* restoreContinuousBackupSource:
* cluster: ${source.name}
* pointInTime: 2023-08-03T19:19:00.094Z
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: alloydb-source-cluster
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${default.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* default:
* fn::invoke:
* Function: gcp:compute:getNetwork
* Arguments:
* name: alloydb-network
* ```
*
* ### Alloydb Secondary Cluster Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {name: "alloydb-secondary-cluster"});
* const primary = new gcp.alloydb.Cluster("primary", {
* clusterId: "alloydb-primary-cluster",
* location: "us-central1",
* network: _default.id,
* });
* const primaryInstance = new gcp.alloydb.Instance("primary", {
* cluster: primary.name,
* instanceId: "alloydb-primary-instance",
* instanceType: "PRIMARY",
* machineConfig: {
* cpuCount: 2,
* },
* });
* const secondary = new gcp.alloydb.Cluster("secondary", {
* clusterId: "alloydb-secondary-cluster",
* location: "us-east1",
* network: _default.id,
* clusterType: "SECONDARY",
* continuousBackupConfig: {
* enabled: false,
* },
* secondaryConfig: {
* primaryClusterName: primary.name,
* },
* });
* const project = gcp.organizations.getProject({});
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "alloydb-secondary-cluster",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: _default.id,
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: _default.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default", name="alloydb-secondary-cluster")
* primary = gcp.alloydb.Cluster("primary",
* cluster_id="alloydb-primary-cluster",
* location="us-central1",
* network=default.id)
* primary_instance = gcp.alloydb.Instance("primary",
* cluster=primary.name,
* instance_id="alloydb-primary-instance",
* instance_type="PRIMARY",
* machine_config=gcp.alloydb.InstanceMachineConfigArgs(
* cpu_count=2,
* ))
* secondary = gcp.alloydb.Cluster("secondary",
* cluster_id="alloydb-secondary-cluster",
* location="us-east1",
* network=default.id,
* cluster_type="SECONDARY",
* continuous_backup_config=gcp.alloydb.ClusterContinuousBackupConfigArgs(
* enabled=False,
* ),
* secondary_config=gcp.alloydb.ClusterSecondaryConfigArgs(
* primary_cluster_name=primary.name,
* ))
* project = gcp.organizations.get_project()
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="alloydb-secondary-cluster",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-secondary-cluster",
* });
* var primary = new Gcp.Alloydb.Cluster("primary", new()
* {
* ClusterId = "alloydb-primary-cluster",
* Location = "us-central1",
* Network = @default.Id,
* });
* var primaryInstance = new Gcp.Alloydb.Instance("primary", new()
* {
* Cluster = primary.Name,
* InstanceId = "alloydb-primary-instance",
* InstanceType = "PRIMARY",
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* });
* var secondary = new Gcp.Alloydb.Cluster("secondary", new()
* {
* ClusterId = "alloydb-secondary-cluster",
* Location = "us-east1",
* Network = @default.Id,
* ClusterType = "SECONDARY",
* ContinuousBackupConfig = new Gcp.Alloydb.Inputs.ClusterContinuousBackupConfigArgs
* {
* Enabled = false,
* },
* SecondaryConfig = new Gcp.Alloydb.Inputs.ClusterSecondaryConfigArgs
* {
* PrimaryClusterName = primary.Name,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "alloydb-secondary-cluster",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = @default.Id,
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = @default.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-secondary-cluster"),
* })
* if err != nil {
* return err
* }
* primary, err := alloydb.NewCluster(ctx, "primary", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-primary-cluster"),
* Location: pulumi.String("us-central1"),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewInstance(ctx, "primary", &alloydb.InstanceArgs{
* Cluster: primary.Name,
* InstanceId: pulumi.String("alloydb-primary-instance"),
* InstanceType: pulumi.String("PRIMARY"),
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "secondary", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-secondary-cluster"),
* Location: pulumi.String("us-east1"),
* Network: _default.ID(),
* ClusterType: pulumi.String("SECONDARY"),
* ContinuousBackupConfig: &alloydb.ClusterContinuousBackupConfigArgs{
* Enabled: pulumi.Bool(false),
* },
* SecondaryConfig: &alloydb.ClusterSecondaryConfigArgs{
* PrimaryClusterName: primary.Name,
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("alloydb-secondary-cluster"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: _default.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.Instance;
* import com.pulumi.gcp.alloydb.InstanceArgs;
* import com.pulumi.gcp.alloydb.inputs.InstanceMachineConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterSecondaryConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("alloydb-secondary-cluster")
* .build());
* var primary = new Cluster("primary", ClusterArgs.builder()
* .clusterId("alloydb-primary-cluster")
* .location("us-central1")
* .network(default_.id())
* .build());
* var primaryInstance = new Instance("primaryInstance", InstanceArgs.builder()
* .cluster(primary.name())
* .instanceId("alloydb-primary-instance")
* .instanceType("PRIMARY")
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build());
* var secondary = new Cluster("secondary", ClusterArgs.builder()
* .clusterId("alloydb-secondary-cluster")
* .location("us-east1")
* .network(default_.id())
* .clusterType("SECONDARY")
* .continuousBackupConfig(ClusterContinuousBackupConfigArgs.builder()
* .enabled(false)
* .build())
* .secondaryConfig(ClusterSecondaryConfigArgs.builder()
* .primaryClusterName(primary.name())
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("alloydb-secondary-cluster")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-primary-cluster
* location: us-central1
* network: ${default.id}
* primaryInstance:
* type: gcp:alloydb:Instance
* name: primary
* properties:
* cluster: ${primary.name}
* instanceId: alloydb-primary-instance
* instanceType: PRIMARY
* machineConfig:
* cpuCount: 2
* secondary:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-secondary-cluster
* location: us-east1
* network: ${default.id}
* clusterType: SECONDARY
* continuousBackupConfig:
* enabled: false
* secondaryConfig:
* primaryClusterName: ${primary.name}
* default:
* type: gcp:compute:Network
* properties:
* name: alloydb-secondary-cluster
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: alloydb-secondary-cluster
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${default.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Cluster can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/clusters/{{cluster_id}}`
* * `{{project}}/{{location}}/{{cluster_id}}`
* * `{{location}}/{{cluster_id}}`
* * `{{cluster_id}}`
* When using the `pulumi import` command, Cluster can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default projects/{{project}}/locations/{{location}}/clusters/{{cluster_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default {{project}}/{{location}}/{{cluster_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default {{location}}/{{cluster_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default {{cluster_id}}
* ```
* @property annotations Annotations to allow client tools to store small amount of arbitrary data. This is distinct from labels. https://google.aip.dev/128
* An object containing a list of "key": value pairs. Example: { "name": "wrench", "mass": "1.3kg", "count": "3" }.
* **Note**: This field is non-authoritative, and will only manage the annotations present in your configuration.
* Please refer to the field `effective_annotations` for all of the annotations present on the resource.
* @property automatedBackupPolicy The automated backup policy for this cluster. AutomatedBackupPolicy is disabled by default.
* Structure is documented below.
* @property clusterId The ID of the alloydb cluster.
* @property clusterType The type of cluster. If not set, defaults to PRIMARY.
* Default value is `PRIMARY`.
* Possible values are: `PRIMARY`, `SECONDARY`.
* @property continuousBackupConfig The continuous backup config for this cluster.
* If no policy is provided then the default policy will be used. The default policy takes one backup a day and retains backups for 14 days.
* Structure is documented below.
* @property databaseVersion The database engine major version. This is an optional field and it's populated at the Cluster creation time. This field cannot be changed after cluster creation.
* @property deletionPolicy Policy to determine if the cluster should be deleted forcefully.
* Deleting a cluster forcefully, deletes the cluster and all its associated instances within the cluster.
* Deleting a Secondary cluster with a secondary instance REQUIRES setting deletion_policy = "FORCE" otherwise an error is returned. This is needed as there is no support to delete just the secondary instance, and the only way to delete secondary instance is to delete the associated secondary cluster forcefully which also deletes the secondary instance.
* @property displayName User-settable and human-readable display name for the Cluster.
* @property encryptionConfig EncryptionConfig describes the encryption config of a cluster or a backup that is encrypted with a CMEK (customer-managed encryption key).
* Structure is documented below.
* @property etag For Resource freshness validation (https://google.aip.dev/154)
* @property initialUser Initial user to setup during cluster creation.
* Structure is documented below.
* @property labels User-defined labels for the alloydb cluster.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property location The location where the alloydb cluster should reside.
* - - -
* @property maintenanceUpdatePolicy MaintenanceUpdatePolicy defines the policy for system updates.
* Structure is documented below.
* @property network (Optional, Deprecated)
* The relative resource name of the VPC network on which the instance can be accessed. It is specified in the following form:
* "projects/{projectNumber}/global/networks/{network_id}".
* > **Warning:** `network` is deprecated and will be removed in a future major release. Instead, use `network_config` to define the network configuration.
* @property networkConfig Metadata related to network configuration.
* Structure is documented below.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property restoreBackupSource The source when restoring from a backup. Conflicts with 'restore_continuous_backup_source', both can't be set together.
* Structure is documented below.
* @property restoreContinuousBackupSource The source when restoring via point in time recovery (PITR). Conflicts with 'restore_backup_source', both can't be set together.
* Structure is documented below.
* @property secondaryConfig Configuration of the secondary cluster for Cross Region Replication. This should be set if and only if the cluster is of type SECONDARY.
* Structure is documented below.
*/
public data class ClusterArgs(
public val annotations: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy