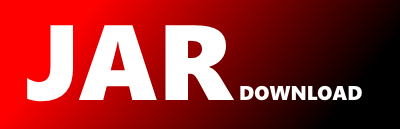
com.pulumi.gcp.alloydb.kotlin.InstanceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.alloydb.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.alloydb.InstanceArgs.builder
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceClientConnectionConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceClientConnectionConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceMachineConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceMachineConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceNetworkConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceNetworkConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceQueryInsightsConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceQueryInsightsConfigArgsBuilder
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceReadPoolConfigArgs
import com.pulumi.gcp.alloydb.kotlin.inputs.InstanceReadPoolConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ### Alloydb Instance Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultNetwork = new gcp.compute.Network("default", {name: "alloydb-network"});
* const defaultCluster = new gcp.alloydb.Cluster("default", {
* clusterId: "alloydb-cluster",
* location: "us-central1",
* network: defaultNetwork.id,
* initialUser: {
* password: "alloydb-cluster",
* },
* });
* const _default = new gcp.alloydb.Instance("default", {
* cluster: defaultCluster.name,
* instanceId: "alloydb-instance",
* instanceType: "PRIMARY",
* machineConfig: {
* cpuCount: 2,
* },
* });
* const project = gcp.organizations.getProject({});
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "alloydb-cluster",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: defaultNetwork.id,
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: defaultNetwork.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_network = gcp.compute.Network("default", name="alloydb-network")
* default_cluster = gcp.alloydb.Cluster("default",
* cluster_id="alloydb-cluster",
* location="us-central1",
* network=default_network.id,
* initial_user=gcp.alloydb.ClusterInitialUserArgs(
* password="alloydb-cluster",
* ))
* default = gcp.alloydb.Instance("default",
* cluster=default_cluster.name,
* instance_id="alloydb-instance",
* instance_type="PRIMARY",
* machine_config=gcp.alloydb.InstanceMachineConfigArgs(
* cpu_count=2,
* ))
* project = gcp.organizations.get_project()
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="alloydb-cluster",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default_network.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default_network.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultNetwork = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-network",
* });
* var defaultCluster = new Gcp.Alloydb.Cluster("default", new()
* {
* ClusterId = "alloydb-cluster",
* Location = "us-central1",
* Network = defaultNetwork.Id,
* InitialUser = new Gcp.Alloydb.Inputs.ClusterInitialUserArgs
* {
* Password = "alloydb-cluster",
* },
* });
* var @default = new Gcp.Alloydb.Instance("default", new()
* {
* Cluster = defaultCluster.Name,
* InstanceId = "alloydb-instance",
* InstanceType = "PRIMARY",
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "alloydb-cluster",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = defaultNetwork.Id,
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = defaultNetwork.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultNetwork, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-network"),
* })
* if err != nil {
* return err
* }
* defaultCluster, err := alloydb.NewCluster(ctx, "default", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-cluster"),
* Location: pulumi.String("us-central1"),
* Network: defaultNetwork.ID(),
* InitialUser: &alloydb.ClusterInitialUserArgs{
* Password: pulumi.String("alloydb-cluster"),
* },
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewInstance(ctx, "default", &alloydb.InstanceArgs{
* Cluster: defaultCluster.Name,
* InstanceId: pulumi.String("alloydb-instance"),
* InstanceType: pulumi.String("PRIMARY"),
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("alloydb-cluster"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: defaultNetwork.ID(),
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: defaultNetwork.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterInitialUserArgs;
* import com.pulumi.gcp.alloydb.Instance;
* import com.pulumi.gcp.alloydb.InstanceArgs;
* import com.pulumi.gcp.alloydb.inputs.InstanceMachineConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultNetwork = new Network("defaultNetwork", NetworkArgs.builder()
* .name("alloydb-network")
* .build());
* var defaultCluster = new Cluster("defaultCluster", ClusterArgs.builder()
* .clusterId("alloydb-cluster")
* .location("us-central1")
* .network(defaultNetwork.id())
* .initialUser(ClusterInitialUserArgs.builder()
* .password("alloydb-cluster")
* .build())
* .build());
* var default_ = new Instance("default", InstanceArgs.builder()
* .cluster(defaultCluster.name())
* .instanceId("alloydb-instance")
* .instanceType("PRIMARY")
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("alloydb-cluster")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(defaultNetwork.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(defaultNetwork.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:alloydb:Instance
* properties:
* cluster: ${defaultCluster.name}
* instanceId: alloydb-instance
* instanceType: PRIMARY
* machineConfig:
* cpuCount: 2
* defaultCluster:
* type: gcp:alloydb:Cluster
* name: default
* properties:
* clusterId: alloydb-cluster
* location: us-central1
* network: ${defaultNetwork.id}
* initialUser:
* password: alloydb-cluster
* defaultNetwork:
* type: gcp:compute:Network
* name: default
* properties:
* name: alloydb-network
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: alloydb-cluster
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${defaultNetwork.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${defaultNetwork.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Alloydb Secondary Instance Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {name: "alloydb-secondary-network"});
* const primary = new gcp.alloydb.Cluster("primary", {
* clusterId: "alloydb-primary-cluster",
* location: "us-central1",
* network: _default.id,
* });
* const primaryInstance = new gcp.alloydb.Instance("primary", {
* cluster: primary.name,
* instanceId: "alloydb-primary-instance",
* instanceType: "PRIMARY",
* machineConfig: {
* cpuCount: 2,
* },
* });
* const secondary = new gcp.alloydb.Cluster("secondary", {
* clusterId: "alloydb-secondary-cluster",
* location: "us-east1",
* network: _default.id,
* clusterType: "SECONDARY",
* continuousBackupConfig: {
* enabled: false,
* },
* secondaryConfig: {
* primaryClusterName: primary.name,
* },
* deletionPolicy: "FORCE",
* });
* const secondaryInstance = new gcp.alloydb.Instance("secondary", {
* cluster: secondary.name,
* instanceId: "alloydb-secondary-instance",
* instanceType: secondary.clusterType,
* machineConfig: {
* cpuCount: 2,
* },
* });
* const project = gcp.organizations.getProject({});
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "alloydb-secondary-instance",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: _default.id,
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: _default.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default", name="alloydb-secondary-network")
* primary = gcp.alloydb.Cluster("primary",
* cluster_id="alloydb-primary-cluster",
* location="us-central1",
* network=default.id)
* primary_instance = gcp.alloydb.Instance("primary",
* cluster=primary.name,
* instance_id="alloydb-primary-instance",
* instance_type="PRIMARY",
* machine_config=gcp.alloydb.InstanceMachineConfigArgs(
* cpu_count=2,
* ))
* secondary = gcp.alloydb.Cluster("secondary",
* cluster_id="alloydb-secondary-cluster",
* location="us-east1",
* network=default.id,
* cluster_type="SECONDARY",
* continuous_backup_config=gcp.alloydb.ClusterContinuousBackupConfigArgs(
* enabled=False,
* ),
* secondary_config=gcp.alloydb.ClusterSecondaryConfigArgs(
* primary_cluster_name=primary.name,
* ),
* deletion_policy="FORCE")
* secondary_instance = gcp.alloydb.Instance("secondary",
* cluster=secondary.name,
* instance_id="alloydb-secondary-instance",
* instance_type=secondary.cluster_type,
* machine_config=gcp.alloydb.InstanceMachineConfigArgs(
* cpu_count=2,
* ))
* project = gcp.organizations.get_project()
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="alloydb-secondary-instance",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-secondary-network",
* });
* var primary = new Gcp.Alloydb.Cluster("primary", new()
* {
* ClusterId = "alloydb-primary-cluster",
* Location = "us-central1",
* Network = @default.Id,
* });
* var primaryInstance = new Gcp.Alloydb.Instance("primary", new()
* {
* Cluster = primary.Name,
* InstanceId = "alloydb-primary-instance",
* InstanceType = "PRIMARY",
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* });
* var secondary = new Gcp.Alloydb.Cluster("secondary", new()
* {
* ClusterId = "alloydb-secondary-cluster",
* Location = "us-east1",
* Network = @default.Id,
* ClusterType = "SECONDARY",
* ContinuousBackupConfig = new Gcp.Alloydb.Inputs.ClusterContinuousBackupConfigArgs
* {
* Enabled = false,
* },
* SecondaryConfig = new Gcp.Alloydb.Inputs.ClusterSecondaryConfigArgs
* {
* PrimaryClusterName = primary.Name,
* },
* DeletionPolicy = "FORCE",
* });
* var secondaryInstance = new Gcp.Alloydb.Instance("secondary", new()
* {
* Cluster = secondary.Name,
* InstanceId = "alloydb-secondary-instance",
* InstanceType = secondary.ClusterType,
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "alloydb-secondary-instance",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = @default.Id,
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = @default.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-secondary-network"),
* })
* if err != nil {
* return err
* }
* primary, err := alloydb.NewCluster(ctx, "primary", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-primary-cluster"),
* Location: pulumi.String("us-central1"),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewInstance(ctx, "primary", &alloydb.InstanceArgs{
* Cluster: primary.Name,
* InstanceId: pulumi.String("alloydb-primary-instance"),
* InstanceType: pulumi.String("PRIMARY"),
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* })
* if err != nil {
* return err
* }
* secondary, err := alloydb.NewCluster(ctx, "secondary", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-secondary-cluster"),
* Location: pulumi.String("us-east1"),
* Network: _default.ID(),
* ClusterType: pulumi.String("SECONDARY"),
* ContinuousBackupConfig: &alloydb.ClusterContinuousBackupConfigArgs{
* Enabled: pulumi.Bool(false),
* },
* SecondaryConfig: &alloydb.ClusterSecondaryConfigArgs{
* PrimaryClusterName: primary.Name,
* },
* DeletionPolicy: pulumi.String("FORCE"),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewInstance(ctx, "secondary", &alloydb.InstanceArgs{
* Cluster: secondary.Name,
* InstanceId: pulumi.String("alloydb-secondary-instance"),
* InstanceType: secondary.ClusterType,
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("alloydb-secondary-instance"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: _default.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.Instance;
* import com.pulumi.gcp.alloydb.InstanceArgs;
* import com.pulumi.gcp.alloydb.inputs.InstanceMachineConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterSecondaryConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("alloydb-secondary-network")
* .build());
* var primary = new Cluster("primary", ClusterArgs.builder()
* .clusterId("alloydb-primary-cluster")
* .location("us-central1")
* .network(default_.id())
* .build());
* var primaryInstance = new Instance("primaryInstance", InstanceArgs.builder()
* .cluster(primary.name())
* .instanceId("alloydb-primary-instance")
* .instanceType("PRIMARY")
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build());
* var secondary = new Cluster("secondary", ClusterArgs.builder()
* .clusterId("alloydb-secondary-cluster")
* .location("us-east1")
* .network(default_.id())
* .clusterType("SECONDARY")
* .continuousBackupConfig(ClusterContinuousBackupConfigArgs.builder()
* .enabled(false)
* .build())
* .secondaryConfig(ClusterSecondaryConfigArgs.builder()
* .primaryClusterName(primary.name())
* .build())
* .deletionPolicy("FORCE")
* .build());
* var secondaryInstance = new Instance("secondaryInstance", InstanceArgs.builder()
* .cluster(secondary.name())
* .instanceId("alloydb-secondary-instance")
* .instanceType(secondary.clusterType())
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("alloydb-secondary-instance")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-primary-cluster
* location: us-central1
* network: ${default.id}
* primaryInstance:
* type: gcp:alloydb:Instance
* name: primary
* properties:
* cluster: ${primary.name}
* instanceId: alloydb-primary-instance
* instanceType: PRIMARY
* machineConfig:
* cpuCount: 2
* secondary:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-secondary-cluster
* location: us-east1
* network: ${default.id}
* clusterType: SECONDARY
* continuousBackupConfig:
* enabled: false
* secondaryConfig:
* primaryClusterName: ${primary.name}
* deletionPolicy: FORCE
* secondaryInstance:
* type: gcp:alloydb:Instance
* name: secondary
* properties:
* cluster: ${secondary.name}
* instanceId: alloydb-secondary-instance
* instanceType: ${secondary.clusterType}
* machineConfig:
* cpuCount: 2
* default:
* type: gcp:compute:Network
* properties:
* name: alloydb-secondary-network
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: alloydb-secondary-instance
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${default.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Instance can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/clusters/{{cluster}}/instances/{{instance_id}}`
* * `{{project}}/{{location}}/{{cluster}}/{{instance_id}}`
* * `{{location}}/{{cluster}}/{{instance_id}}`
* When using the `pulumi import` command, Instance can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:alloydb/instance:Instance default projects/{{project}}/locations/{{location}}/clusters/{{cluster}}/instances/{{instance_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/instance:Instance default {{project}}/{{location}}/{{cluster}}/{{instance_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/instance:Instance default {{location}}/{{cluster}}/{{instance_id}}
* ```
* @property annotations Annotations to allow client tools to store small amount of arbitrary data. This is distinct from labels.
* **Note**: This field is non-authoritative, and will only manage the annotations present in your configuration.
* Please refer to the field `effective_annotations` for all of the annotations present on the resource.
* @property availabilityType 'Availability type of an Instance. Defaults to REGIONAL for both primary and read instances.
* Note that primary and read instances can have different availability types.
* Only READ_POOL instance supports ZONAL type. Users can't specify the zone for READ_POOL instance.
* Zone is automatically chosen from the list of zones in the region specified.
* Read pool of size 1 can only have zonal availability. Read pools with node count of 2 or more
* can have regional availability (nodes are present in 2 or more zones in a region).'
* Possible values are: `AVAILABILITY_TYPE_UNSPECIFIED`, `ZONAL`, `REGIONAL`.
* @property clientConnectionConfig Client connection specific configurations.
* Structure is documented below.
* @property cluster Identifies the alloydb cluster. Must be in the format
* 'projects/{project}/locations/{location}/clusters/{cluster_id}'
* @property databaseFlags Database flags. Set at instance level. * They are copied from primary instance on read instance creation. * Read instances can set new or override existing flags that are relevant for reads, e.g. for enabling columnar cache on a read instance. Flags set on read instance may or may not be present on primary.
* @property displayName User-settable and human-readable display name for the Instance.
* @property gceZone The Compute Engine zone that the instance should serve from, per https://cloud.google.com/compute/docs/regions-zones This can ONLY be specified for ZONAL instances. If present for a REGIONAL instance, an error will be thrown. If this is absent for a ZONAL instance, instance is created in a random zone with available capacity.
* @property instanceId The ID of the alloydb instance.
* - - -
* @property instanceType
* @property labels User-defined labels for the alloydb instance.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property machineConfig Configurations for the machines that host the underlying database engine.
* Structure is documented below.
* @property networkConfig Instance level network configuration.
* Structure is documented below.
* @property queryInsightsConfig Configuration for query insights.
* Structure is documented below.
* @property readPoolConfig Read pool specific config. If the instance type is READ_POOL, this configuration must be provided.
* Structure is documented below.
*/
public data class InstanceArgs(
public val annotations: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy