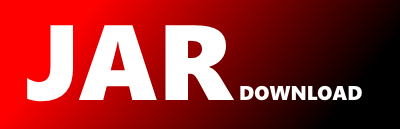
com.pulumi.gcp.alloydb.kotlin.inputs.ClusterContinuousBackupConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.alloydb.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enabled Whether continuous backup recovery is enabled. If not set, defaults to true.
* @property encryptionConfig EncryptionConfig describes the encryption config of a cluster or a backup that is encrypted with a CMEK (customer-managed encryption key).
* Structure is documented below.
* @property recoveryWindowDays The numbers of days that are eligible to restore from using PITR. To support the entire recovery window, backups and logs are retained for one day more than the recovery window.
* If not set, defaults to 14 days.
*/
public data class ClusterContinuousBackupConfigArgs(
public val enabled: Output? = null,
public val encryptionConfig: Output? = null,
public val recoveryWindowDays: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs =
com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.encryptionConfig(encryptionConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.recoveryWindowDays(recoveryWindowDays?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterContinuousBackupConfigArgs].
*/
@PulumiTagMarker
public class ClusterContinuousBackupConfigArgsBuilder internal constructor() {
private var enabled: Output? = null
private var encryptionConfig: Output? = null
private var recoveryWindowDays: Output? = null
/**
* @param value Whether continuous backup recovery is enabled. If not set, defaults to true.
*/
@JvmName("kxckolpvndepucqv")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value EncryptionConfig describes the encryption config of a cluster or a backup that is encrypted with a CMEK (customer-managed encryption key).
* Structure is documented below.
*/
@JvmName("tstecjnuiboybxaw")
public suspend fun encryptionConfig(`value`: Output) {
this.encryptionConfig = value
}
/**
* @param value The numbers of days that are eligible to restore from using PITR. To support the entire recovery window, backups and logs are retained for one day more than the recovery window.
* If not set, defaults to 14 days.
*/
@JvmName("npofehlmcowkgori")
public suspend fun recoveryWindowDays(`value`: Output) {
this.recoveryWindowDays = value
}
/**
* @param value Whether continuous backup recovery is enabled. If not set, defaults to true.
*/
@JvmName("drlcrykcnjdstcqs")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value EncryptionConfig describes the encryption config of a cluster or a backup that is encrypted with a CMEK (customer-managed encryption key).
* Structure is documented below.
*/
@JvmName("myvnurhoxgfwfufi")
public suspend fun encryptionConfig(`value`: ClusterContinuousBackupConfigEncryptionConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfig = mapped
}
/**
* @param argument EncryptionConfig describes the encryption config of a cluster or a backup that is encrypted with a CMEK (customer-managed encryption key).
* Structure is documented below.
*/
@JvmName("akrlymbmjljjiixy")
public suspend fun encryptionConfig(argument: suspend ClusterContinuousBackupConfigEncryptionConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterContinuousBackupConfigEncryptionConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.encryptionConfig = mapped
}
/**
* @param value The numbers of days that are eligible to restore from using PITR. To support the entire recovery window, backups and logs are retained for one day more than the recovery window.
* If not set, defaults to 14 days.
*/
@JvmName("drmxkjswsoqtheuu")
public suspend fun recoveryWindowDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoveryWindowDays = mapped
}
internal fun build(): ClusterContinuousBackupConfigArgs = ClusterContinuousBackupConfigArgs(
enabled = enabled,
encryptionConfig = encryptionConfig,
recoveryWindowDays = recoveryWindowDays,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy