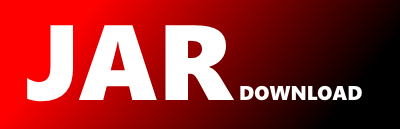
com.pulumi.gcp.apigee.kotlin.Flowhook.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.apigee.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Flowhook].
*/
@PulumiTagMarker
public class FlowhookResourceBuilder internal constructor() {
public var name: String? = null
public var args: FlowhookArgs = FlowhookArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FlowhookArgsBuilder.() -> Unit) {
val builder = FlowhookArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Flowhook {
val builtJavaResource = com.pulumi.gcp.apigee.Flowhook(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Flowhook(builtJavaResource)
}
}
/**
* Represents a sharedflow attachment to a flowhook point.
* To get more information about Flowhook, see:
* * [API documentation](https://cloud.google.com/apigee/docs/reference/apis/apigee/rest/v1/organizations.environments.flowhooks#FlowHook)
* * How-to Guides
* * [organizations.environments.flowhooks](https://cloud.google.com/apigee/docs/reference/apis/apigee/rest/v1/organizations.environments.flowhooks#FlowHook)
* ## Import
* Flowhook can be imported using any of these accepted formats:
* * `organizations/{{org_id}}/environments/{{environment}}/flowhooks/{{flow_hook_point}}`
* * `{{org_id}}/{{environment}}/{{flow_hook_point}}`
* When using the `pulumi import` command, Flowhook can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:apigee/flowhook:Flowhook default organizations/{{org_id}}/environments/{{environment}}/flowhooks/{{flow_hook_point}}
* ```
* ```sh
* $ pulumi import gcp:apigee/flowhook:Flowhook default {{org_id}}/{{environment}}/{{flow_hook_point}}
* ```
*/
public class Flowhook internal constructor(
override val javaResource: com.pulumi.gcp.apigee.Flowhook,
) : KotlinCustomResource(javaResource, FlowhookMapper) {
/**
* Flag that specifies whether execution should continue if the flow hook throws an exception. Set to true to continue execution. Set to false to stop execution if the flow hook throws an exception. Defaults to true.
*/
public val continueOnError: Output?
get() = javaResource.continueOnError().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Description of the flow hook.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The resource ID of the environment.
*/
public val environment: Output
get() = javaResource.environment().applyValue({ args0 -> args0 })
/**
* Where in the API call flow the flow hook is invoked. Must be one of PreProxyFlowHook, PostProxyFlowHook, PreTargetFlowHook, or PostTargetFlowHook.
*/
public val flowHookPoint: Output
get() = javaResource.flowHookPoint().applyValue({ args0 -> args0 })
/**
* The Apigee Organization associated with the environment
*/
public val orgId: Output
get() = javaResource.orgId().applyValue({ args0 -> args0 })
/**
* Id of the Sharedflow attaching to a flowhook point.
*/
public val sharedflow: Output
get() = javaResource.sharedflow().applyValue({ args0 -> args0 })
}
public object FlowhookMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.apigee.Flowhook::class == javaResource::class
override fun map(javaResource: Resource): Flowhook = Flowhook(
javaResource as
com.pulumi.gcp.apigee.Flowhook,
)
}
/**
* @see [Flowhook].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Flowhook].
*/
public suspend fun flowhook(name: String, block: suspend FlowhookResourceBuilder.() -> Unit): Flowhook {
val builder = FlowhookResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Flowhook].
* @param name The _unique_ name of the resulting resource.
*/
public fun flowhook(name: String): Flowhook {
val builder = FlowhookResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy