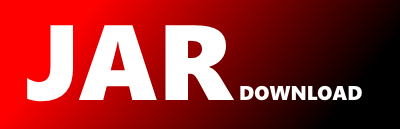
com.pulumi.gcp.apigee.kotlin.KeystoresAliasesSelfSignedCert.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.apigee.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.apigee.kotlin.outputs.KeystoresAliasesSelfSignedCertCertsInfo
import com.pulumi.gcp.apigee.kotlin.outputs.KeystoresAliasesSelfSignedCertSubject
import com.pulumi.gcp.apigee.kotlin.outputs.KeystoresAliasesSelfSignedCertSubjectAlternativeDnsNames
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.apigee.kotlin.outputs.KeystoresAliasesSelfSignedCertCertsInfo.Companion.toKotlin as keystoresAliasesSelfSignedCertCertsInfoToKotlin
import com.pulumi.gcp.apigee.kotlin.outputs.KeystoresAliasesSelfSignedCertSubject.Companion.toKotlin as keystoresAliasesSelfSignedCertSubjectToKotlin
import com.pulumi.gcp.apigee.kotlin.outputs.KeystoresAliasesSelfSignedCertSubjectAlternativeDnsNames.Companion.toKotlin as keystoresAliasesSelfSignedCertSubjectAlternativeDnsNamesToKotlin
/**
* Builder for [KeystoresAliasesSelfSignedCert].
*/
@PulumiTagMarker
public class KeystoresAliasesSelfSignedCertResourceBuilder internal constructor() {
public var name: String? = null
public var args: KeystoresAliasesSelfSignedCertArgs = KeystoresAliasesSelfSignedCertArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend KeystoresAliasesSelfSignedCertArgsBuilder.() -> Unit) {
val builder = KeystoresAliasesSelfSignedCertArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): KeystoresAliasesSelfSignedCert {
val builtJavaResource =
com.pulumi.gcp.apigee.KeystoresAliasesSelfSignedCert(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return KeystoresAliasesSelfSignedCert(builtJavaResource)
}
}
/**
* An Environment Keystore Alias for Self Signed Certificate Format in Apigee
* To get more information about KeystoresAliasesSelfSignedCert, see:
* * [API documentation](https://cloud.google.com/apigee/docs/reference/apis/apigee/rest/v1/organizations.environments.keystores.aliases/create)
* * How-to Guides
* * [Creating an environment](https://cloud.google.com/apigee/docs/api-platform/get-started/create-environment)
* ## Example Usage
* ### Apigee Env Keystore Alias Self Signed Cert
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = new gcp.organizations.Project("project", {
* projectId: "my-project",
* name: "my-project",
* orgId: "123456789",
* billingAccount: "000000-0000000-0000000-000000",
* });
* const apigee = new gcp.projects.Service("apigee", {
* project: project.projectId,
* service: "apigee.googleapis.com",
* });
* const servicenetworking = new gcp.projects.Service("servicenetworking", {
* project: project.projectId,
* service: "servicenetworking.googleapis.com",
* });
* const compute = new gcp.projects.Service("compute", {
* project: project.projectId,
* service: "compute.googleapis.com",
* });
* const apigeeNetwork = new gcp.compute.Network("apigee_network", {
* name: "apigee-network",
* project: project.projectId,
* });
* const apigeeRange = new gcp.compute.GlobalAddress("apigee_range", {
* name: "apigee-range",
* purpose: "VPC_PEERING",
* addressType: "INTERNAL",
* prefixLength: 16,
* network: apigeeNetwork.id,
* project: project.projectId,
* });
* const apigeeVpcConnection = new gcp.servicenetworking.Connection("apigee_vpc_connection", {
* network: apigeeNetwork.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [apigeeRange.name],
* });
* const apigeeOrg = new gcp.apigee.Organization("apigee_org", {
* analyticsRegion: "us-central1",
* projectId: project.projectId,
* authorizedNetwork: apigeeNetwork.id,
* });
* const apigeeEnvironmentKeystoreSsAlias = new gcp.apigee.Environment("apigee_environment_keystore_ss_alias", {
* orgId: apigeeOrg.id,
* name: "env-name",
* description: "Apigee Environment",
* displayName: "environment-1",
* });
* const apigeeEnvironmentKeystoreAlias = new gcp.apigee.EnvKeystore("apigee_environment_keystore_alias", {
* name: "env-keystore",
* envId: apigeeEnvironmentKeystoreSsAlias.id,
* });
* const apigeeEnvironmentKeystoreSsAliasKeystoresAliasesSelfSignedCert = new gcp.apigee.KeystoresAliasesSelfSignedCert("apigee_environment_keystore_ss_alias", {
* environment: apigeeEnvironmentKeystoreSsAlias.name,
* orgId: apigeeOrg.name,
* keystore: apigeeEnvironmentKeystoreAlias.name,
* alias: "alias",
* keySize: "1024",
* sigAlg: "SHA512withRSA",
* certValidityInDays: 4,
* subject: {
* commonName: "selfsigned_example",
* countryCode: "US",
* locality: "TX",
* org: "CCE",
* orgUnit: "PSO",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.Project("project",
* project_id="my-project",
* name="my-project",
* org_id="123456789",
* billing_account="000000-0000000-0000000-000000")
* apigee = gcp.projects.Service("apigee",
* project=project.project_id,
* service="apigee.googleapis.com")
* servicenetworking = gcp.projects.Service("servicenetworking",
* project=project.project_id,
* service="servicenetworking.googleapis.com")
* compute = gcp.projects.Service("compute",
* project=project.project_id,
* service="compute.googleapis.com")
* apigee_network = gcp.compute.Network("apigee_network",
* name="apigee-network",
* project=project.project_id)
* apigee_range = gcp.compute.GlobalAddress("apigee_range",
* name="apigee-range",
* purpose="VPC_PEERING",
* address_type="INTERNAL",
* prefix_length=16,
* network=apigee_network.id,
* project=project.project_id)
* apigee_vpc_connection = gcp.servicenetworking.Connection("apigee_vpc_connection",
* network=apigee_network.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[apigee_range.name])
* apigee_org = gcp.apigee.Organization("apigee_org",
* analytics_region="us-central1",
* project_id=project.project_id,
* authorized_network=apigee_network.id)
* apigee_environment_keystore_ss_alias = gcp.apigee.Environment("apigee_environment_keystore_ss_alias",
* org_id=apigee_org.id,
* name="env-name",
* description="Apigee Environment",
* display_name="environment-1")
* apigee_environment_keystore_alias = gcp.apigee.EnvKeystore("apigee_environment_keystore_alias",
* name="env-keystore",
* env_id=apigee_environment_keystore_ss_alias.id)
* apigee_environment_keystore_ss_alias_keystores_aliases_self_signed_cert = gcp.apigee.KeystoresAliasesSelfSignedCert("apigee_environment_keystore_ss_alias",
* environment=apigee_environment_keystore_ss_alias.name,
* org_id=apigee_org.name,
* keystore=apigee_environment_keystore_alias.name,
* alias="alias",
* key_size="1024",
* sig_alg="SHA512withRSA",
* cert_validity_in_days=4,
* subject=gcp.apigee.KeystoresAliasesSelfSignedCertSubjectArgs(
* common_name="selfsigned_example",
* country_code="US",
* locality="TX",
* org="CCE",
* org_unit="PSO",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = new Gcp.Organizations.Project("project", new()
* {
* ProjectId = "my-project",
* Name = "my-project",
* OrgId = "123456789",
* BillingAccount = "000000-0000000-0000000-000000",
* });
* var apigee = new Gcp.Projects.Service("apigee", new()
* {
* Project = project.ProjectId,
* ServiceName = "apigee.googleapis.com",
* });
* var servicenetworking = new Gcp.Projects.Service("servicenetworking", new()
* {
* Project = project.ProjectId,
* ServiceName = "servicenetworking.googleapis.com",
* });
* var compute = new Gcp.Projects.Service("compute", new()
* {
* Project = project.ProjectId,
* ServiceName = "compute.googleapis.com",
* });
* var apigeeNetwork = new Gcp.Compute.Network("apigee_network", new()
* {
* Name = "apigee-network",
* Project = project.ProjectId,
* });
* var apigeeRange = new Gcp.Compute.GlobalAddress("apigee_range", new()
* {
* Name = "apigee-range",
* Purpose = "VPC_PEERING",
* AddressType = "INTERNAL",
* PrefixLength = 16,
* Network = apigeeNetwork.Id,
* Project = project.ProjectId,
* });
* var apigeeVpcConnection = new Gcp.ServiceNetworking.Connection("apigee_vpc_connection", new()
* {
* Network = apigeeNetwork.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* apigeeRange.Name,
* },
* });
* var apigeeOrg = new Gcp.Apigee.Organization("apigee_org", new()
* {
* AnalyticsRegion = "us-central1",
* ProjectId = project.ProjectId,
* AuthorizedNetwork = apigeeNetwork.Id,
* });
* var apigeeEnvironmentKeystoreSsAlias = new Gcp.Apigee.Environment("apigee_environment_keystore_ss_alias", new()
* {
* OrgId = apigeeOrg.Id,
* Name = "env-name",
* Description = "Apigee Environment",
* DisplayName = "environment-1",
* });
* var apigeeEnvironmentKeystoreAlias = new Gcp.Apigee.EnvKeystore("apigee_environment_keystore_alias", new()
* {
* Name = "env-keystore",
* EnvId = apigeeEnvironmentKeystoreSsAlias.Id,
* });
* var apigeeEnvironmentKeystoreSsAliasKeystoresAliasesSelfSignedCert = new Gcp.Apigee.KeystoresAliasesSelfSignedCert("apigee_environment_keystore_ss_alias", new()
* {
* Environment = apigeeEnvironmentKeystoreSsAlias.Name,
* OrgId = apigeeOrg.Name,
* Keystore = apigeeEnvironmentKeystoreAlias.Name,
* Alias = "alias",
* KeySize = "1024",
* SigAlg = "SHA512withRSA",
* CertValidityInDays = 4,
* Subject = new Gcp.Apigee.Inputs.KeystoresAliasesSelfSignedCertSubjectArgs
* {
* CommonName = "selfsigned_example",
* CountryCode = "US",
* Locality = "TX",
* Org = "CCE",
* OrgUnit = "PSO",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/apigee"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/projects"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.NewProject(ctx, "project", &organizations.ProjectArgs{
* ProjectId: pulumi.String("my-project"),
* Name: pulumi.String("my-project"),
* OrgId: pulumi.String("123456789"),
* BillingAccount: pulumi.String("000000-0000000-0000000-000000"),
* })
* if err != nil {
* return err
* }
* _, err = projects.NewService(ctx, "apigee", &projects.ServiceArgs{
* Project: project.ProjectId,
* Service: pulumi.String("apigee.googleapis.com"),
* })
* if err != nil {
* return err
* }
* _, err = projects.NewService(ctx, "servicenetworking", &projects.ServiceArgs{
* Project: project.ProjectId,
* Service: pulumi.String("servicenetworking.googleapis.com"),
* })
* if err != nil {
* return err
* }
* _, err = projects.NewService(ctx, "compute", &projects.ServiceArgs{
* Project: project.ProjectId,
* Service: pulumi.String("compute.googleapis.com"),
* })
* if err != nil {
* return err
* }
* apigeeNetwork, err := compute.NewNetwork(ctx, "apigee_network", &compute.NetworkArgs{
* Name: pulumi.String("apigee-network"),
* Project: project.ProjectId,
* })
* if err != nil {
* return err
* }
* apigeeRange, err := compute.NewGlobalAddress(ctx, "apigee_range", &compute.GlobalAddressArgs{
* Name: pulumi.String("apigee-range"),
* Purpose: pulumi.String("VPC_PEERING"),
* AddressType: pulumi.String("INTERNAL"),
* PrefixLength: pulumi.Int(16),
* Network: apigeeNetwork.ID(),
* Project: project.ProjectId,
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "apigee_vpc_connection", &servicenetworking.ConnectionArgs{
* Network: apigeeNetwork.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* apigeeRange.Name,
* },
* })
* if err != nil {
* return err
* }
* apigeeOrg, err := apigee.NewOrganization(ctx, "apigee_org", &apigee.OrganizationArgs{
* AnalyticsRegion: pulumi.String("us-central1"),
* ProjectId: project.ProjectId,
* AuthorizedNetwork: apigeeNetwork.ID(),
* })
* if err != nil {
* return err
* }
* apigeeEnvironmentKeystoreSsAlias, err := apigee.NewEnvironment(ctx, "apigee_environment_keystore_ss_alias", &apigee.EnvironmentArgs{
* OrgId: apigeeOrg.ID(),
* Name: pulumi.String("env-name"),
* Description: pulumi.String("Apigee Environment"),
* DisplayName: pulumi.String("environment-1"),
* })
* if err != nil {
* return err
* }
* apigeeEnvironmentKeystoreAlias, err := apigee.NewEnvKeystore(ctx, "apigee_environment_keystore_alias", &apigee.EnvKeystoreArgs{
* Name: pulumi.String("env-keystore"),
* EnvId: apigeeEnvironmentKeystoreSsAlias.ID(),
* })
* if err != nil {
* return err
* }
* _, err = apigee.NewKeystoresAliasesSelfSignedCert(ctx, "apigee_environment_keystore_ss_alias", &apigee.KeystoresAliasesSelfSignedCertArgs{
* Environment: apigeeEnvironmentKeystoreSsAlias.Name,
* OrgId: apigeeOrg.Name,
* Keystore: apigeeEnvironmentKeystoreAlias.Name,
* Alias: pulumi.String("alias"),
* KeySize: pulumi.String("1024"),
* SigAlg: pulumi.String("SHA512withRSA"),
* CertValidityInDays: pulumi.Int(4),
* Subject: &apigee.KeystoresAliasesSelfSignedCertSubjectArgs{
* CommonName: pulumi.String("selfsigned_example"),
* CountryCode: pulumi.String("US"),
* Locality: pulumi.String("TX"),
* Org: pulumi.String("CCE"),
* OrgUnit: pulumi.String("PSO"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Project;
* import com.pulumi.gcp.organizations.ProjectArgs;
* import com.pulumi.gcp.projects.Service;
* import com.pulumi.gcp.projects.ServiceArgs;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.apigee.Organization;
* import com.pulumi.gcp.apigee.OrganizationArgs;
* import com.pulumi.gcp.apigee.Environment;
* import com.pulumi.gcp.apigee.EnvironmentArgs;
* import com.pulumi.gcp.apigee.EnvKeystore;
* import com.pulumi.gcp.apigee.EnvKeystoreArgs;
* import com.pulumi.gcp.apigee.KeystoresAliasesSelfSignedCert;
* import com.pulumi.gcp.apigee.KeystoresAliasesSelfSignedCertArgs;
* import com.pulumi.gcp.apigee.inputs.KeystoresAliasesSelfSignedCertSubjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var project = new Project("project", ProjectArgs.builder()
* .projectId("my-project")
* .name("my-project")
* .orgId("123456789")
* .billingAccount("000000-0000000-0000000-000000")
* .build());
* var apigee = new Service("apigee", ServiceArgs.builder()
* .project(project.projectId())
* .service("apigee.googleapis.com")
* .build());
* var servicenetworking = new Service("servicenetworking", ServiceArgs.builder()
* .project(project.projectId())
* .service("servicenetworking.googleapis.com")
* .build());
* var compute = new Service("compute", ServiceArgs.builder()
* .project(project.projectId())
* .service("compute.googleapis.com")
* .build());
* var apigeeNetwork = new Network("apigeeNetwork", NetworkArgs.builder()
* .name("apigee-network")
* .project(project.projectId())
* .build());
* var apigeeRange = new GlobalAddress("apigeeRange", GlobalAddressArgs.builder()
* .name("apigee-range")
* .purpose("VPC_PEERING")
* .addressType("INTERNAL")
* .prefixLength(16)
* .network(apigeeNetwork.id())
* .project(project.projectId())
* .build());
* var apigeeVpcConnection = new Connection("apigeeVpcConnection", ConnectionArgs.builder()
* .network(apigeeNetwork.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(apigeeRange.name())
* .build());
* var apigeeOrg = new Organization("apigeeOrg", OrganizationArgs.builder()
* .analyticsRegion("us-central1")
* .projectId(project.projectId())
* .authorizedNetwork(apigeeNetwork.id())
* .build());
* var apigeeEnvironmentKeystoreSsAlias = new Environment("apigeeEnvironmentKeystoreSsAlias", EnvironmentArgs.builder()
* .orgId(apigeeOrg.id())
* .name("env-name")
* .description("Apigee Environment")
* .displayName("environment-1")
* .build());
* var apigeeEnvironmentKeystoreAlias = new EnvKeystore("apigeeEnvironmentKeystoreAlias", EnvKeystoreArgs.builder()
* .name("env-keystore")
* .envId(apigeeEnvironmentKeystoreSsAlias.id())
* .build());
* var apigeeEnvironmentKeystoreSsAliasKeystoresAliasesSelfSignedCert = new KeystoresAliasesSelfSignedCert("apigeeEnvironmentKeystoreSsAliasKeystoresAliasesSelfSignedCert", KeystoresAliasesSelfSignedCertArgs.builder()
* .environment(apigeeEnvironmentKeystoreSsAlias.name())
* .orgId(apigeeOrg.name())
* .keystore(apigeeEnvironmentKeystoreAlias.name())
* .alias("alias")
* .keySize(1024)
* .sigAlg("SHA512withRSA")
* .certValidityInDays(4)
* .subject(KeystoresAliasesSelfSignedCertSubjectArgs.builder()
* .commonName("selfsigned_example")
* .countryCode("US")
* .locality("TX")
* .org("CCE")
* .orgUnit("PSO")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* project:
* type: gcp:organizations:Project
* properties:
* projectId: my-project
* name: my-project
* orgId: '123456789'
* billingAccount: 000000-0000000-0000000-000000
* apigee:
* type: gcp:projects:Service
* properties:
* project: ${project.projectId}
* service: apigee.googleapis.com
* servicenetworking:
* type: gcp:projects:Service
* properties:
* project: ${project.projectId}
* service: servicenetworking.googleapis.com
* compute:
* type: gcp:projects:Service
* properties:
* project: ${project.projectId}
* service: compute.googleapis.com
* apigeeNetwork:
* type: gcp:compute:Network
* name: apigee_network
* properties:
* name: apigee-network
* project: ${project.projectId}
* apigeeRange:
* type: gcp:compute:GlobalAddress
* name: apigee_range
* properties:
* name: apigee-range
* purpose: VPC_PEERING
* addressType: INTERNAL
* prefixLength: 16
* network: ${apigeeNetwork.id}
* project: ${project.projectId}
* apigeeVpcConnection:
* type: gcp:servicenetworking:Connection
* name: apigee_vpc_connection
* properties:
* network: ${apigeeNetwork.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${apigeeRange.name}
* apigeeOrg:
* type: gcp:apigee:Organization
* name: apigee_org
* properties:
* analyticsRegion: us-central1
* projectId: ${project.projectId}
* authorizedNetwork: ${apigeeNetwork.id}
* apigeeEnvironmentKeystoreSsAlias:
* type: gcp:apigee:Environment
* name: apigee_environment_keystore_ss_alias
* properties:
* orgId: ${apigeeOrg.id}
* name: env-name
* description: Apigee Environment
* displayName: environment-1
* apigeeEnvironmentKeystoreAlias:
* type: gcp:apigee:EnvKeystore
* name: apigee_environment_keystore_alias
* properties:
* name: env-keystore
* envId: ${apigeeEnvironmentKeystoreSsAlias.id}
* apigeeEnvironmentKeystoreSsAliasKeystoresAliasesSelfSignedCert:
* type: gcp:apigee:KeystoresAliasesSelfSignedCert
* name: apigee_environment_keystore_ss_alias
* properties:
* environment: ${apigeeEnvironmentKeystoreSsAlias.name}
* orgId: ${apigeeOrg.name}
* keystore: ${apigeeEnvironmentKeystoreAlias.name}
* alias: alias
* keySize: 1024
* sigAlg: SHA512withRSA
* certValidityInDays: 4
* subject:
* commonName: selfsigned_example
* countryCode: US
* locality: TX
* org: CCE
* orgUnit: PSO
* ```
*
* ## Import
* KeystoresAliasesSelfSignedCert can be imported using any of these accepted formats:
* * `organizations/{{org_id}}/environments/{{environment}}/keystores/{{keystore}}/aliases/{{alias}}`
* * `{{org_id}}/{{environment}}/{{keystore}}/{{alias}}`
* When using the `pulumi import` command, KeystoresAliasesSelfSignedCert can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:apigee/keystoresAliasesSelfSignedCert:KeystoresAliasesSelfSignedCert default organizations/{{org_id}}/environments/{{environment}}/keystores/{{keystore}}/aliases/{{alias}}
* ```
* ```sh
* $ pulumi import gcp:apigee/keystoresAliasesSelfSignedCert:KeystoresAliasesSelfSignedCert default {{org_id}}/{{environment}}/{{keystore}}/{{alias}}
* ```
*/
public class KeystoresAliasesSelfSignedCert internal constructor(
override val javaResource: com.pulumi.gcp.apigee.KeystoresAliasesSelfSignedCert,
) : KotlinCustomResource(javaResource, KeystoresAliasesSelfSignedCertMapper) {
/**
* Alias for the key/certificate pair. Values must match the regular expression [\w\s-.]{1,255}.
* This must be provided for all formats except selfsignedcert; self-signed certs may specify the alias in either
* this parameter or the JSON body.
*/
public val alias: Output
get() = javaResource.alias().applyValue({ args0 -> args0 })
/**
* Validity duration of certificate, in days. Accepts positive non-zero value. Defaults to 365.
*/
public val certValidityInDays: Output?
get() = javaResource.certValidityInDays().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Chain of certificates under this alias.
* Structure is documented below.
*/
public val certsInfos: Output>
get() = javaResource.certsInfos().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
keystoresAliasesSelfSignedCertCertsInfoToKotlin(args0)
})
})
})
/**
* The Apigee environment name
*/
public val environment: Output
get() = javaResource.environment().applyValue({ args0 -> args0 })
/**
* Key size. Default and maximum value is 2048 bits.
*/
public val keySize: Output?
get() = javaResource.keySize().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Apigee keystore name associated in an Apigee environment
*/
public val keystore: Output
get() = javaResource.keystore().applyValue({ args0 -> args0 })
/**
* The Apigee Organization name associated with the Apigee environment
*/
public val orgId: Output
get() = javaResource.orgId().applyValue({ args0 -> args0 })
/**
* Signature algorithm to generate private key. Valid values are SHA512withRSA, SHA384withRSA, and SHA256withRSA
*/
public val sigAlg: Output
get() = javaResource.sigAlg().applyValue({ args0 -> args0 })
/**
* Subject details.
* Structure is documented below.
*/
public val subject: Output
get() = javaResource.subject().applyValue({ args0 ->
args0.let({ args0 ->
keystoresAliasesSelfSignedCertSubjectToKotlin(args0)
})
})
/**
* List of alternative host names. Maximum length is 255 characters for each value.
*/
public val subjectAlternativeDnsNames:
Output?
get() = javaResource.subjectAlternativeDnsNames().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
keystoresAliasesSelfSignedCertSubjectAlternativeDnsNamesToKotlin(args0)
})
}).orElse(null)
})
/**
* Optional.Type of Alias
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object KeystoresAliasesSelfSignedCertMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.apigee.KeystoresAliasesSelfSignedCert::class == javaResource::class
override fun map(javaResource: Resource): KeystoresAliasesSelfSignedCert =
KeystoresAliasesSelfSignedCert(
javaResource as
com.pulumi.gcp.apigee.KeystoresAliasesSelfSignedCert,
)
}
/**
* @see [KeystoresAliasesSelfSignedCert].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [KeystoresAliasesSelfSignedCert].
*/
public suspend fun keystoresAliasesSelfSignedCert(
name: String,
block: suspend KeystoresAliasesSelfSignedCertResourceBuilder.() -> Unit,
): KeystoresAliasesSelfSignedCert {
val builder = KeystoresAliasesSelfSignedCertResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [KeystoresAliasesSelfSignedCert].
* @param name The _unique_ name of the resulting resource.
*/
public fun keystoresAliasesSelfSignedCert(name: String): KeystoresAliasesSelfSignedCert {
val builder = KeystoresAliasesSelfSignedCertResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy