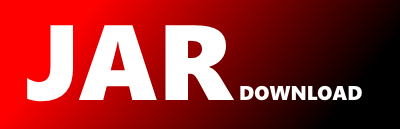
com.pulumi.gcp.apigee.kotlin.SharedflowDeploymentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.apigee.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.apigee.SharedflowDeploymentArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Deploys a revision of a sharedflow.
* To get more information about SharedflowDeployment, see:
* * [API documentation](https://cloud.google.com/apigee/docs/reference/apis/apigee/rest/v1/organizations.environments.sharedflows.revisions.deployments)
* * How-to Guides
* * [sharedflows.revisions.deployments](https://cloud.google.com/apigee/docs/reference/apis/apigee/rest/v1/organizations.environments.sharedflows.revisions.deployments)
* ## Import
* SharedflowDeployment can be imported using any of these accepted formats:
* * `organizations/{{org_id}}/environments/{{environment}}/sharedflows/{{sharedflow_id}}/revisions/{{revision}}/deployments/{{name}}`
* * `{{org_id}}/{{environment}}/{{sharedflow_id}}/{{revision}}/{{name}}`
* When using the `pulumi import` command, SharedflowDeployment can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:apigee/sharedflowDeployment:SharedflowDeployment default organizations/{{org_id}}/environments/{{environment}}/sharedflows/{{sharedflow_id}}/revisions/{{revision}}/deployments/{{name}}
* ```
* ```sh
* $ pulumi import gcp:apigee/sharedflowDeployment:SharedflowDeployment default {{org_id}}/{{environment}}/{{sharedflow_id}}/{{revision}}/{{name}}
* ```
* @property environment The resource ID of the environment.
* @property orgId The Apigee Organization associated with the Sharedflow
* @property revision Revision of the Sharedflow to be deployed.
* - - -
* @property serviceAccount The service account represents the identity of the deployed proxy, and determines what permissions it has. The format must be {ACCOUNT_ID}@{PROJECT}.iam.gserviceaccount.com.
* @property sharedflowId Id of the Sharedflow to be deployed.
*/
public data class SharedflowDeploymentArgs(
public val environment: Output? = null,
public val orgId: Output? = null,
public val revision: Output? = null,
public val serviceAccount: Output? = null,
public val sharedflowId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.apigee.SharedflowDeploymentArgs =
com.pulumi.gcp.apigee.SharedflowDeploymentArgs.builder()
.environment(environment?.applyValue({ args0 -> args0 }))
.orgId(orgId?.applyValue({ args0 -> args0 }))
.revision(revision?.applyValue({ args0 -> args0 }))
.serviceAccount(serviceAccount?.applyValue({ args0 -> args0 }))
.sharedflowId(sharedflowId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SharedflowDeploymentArgs].
*/
@PulumiTagMarker
public class SharedflowDeploymentArgsBuilder internal constructor() {
private var environment: Output? = null
private var orgId: Output? = null
private var revision: Output? = null
private var serviceAccount: Output? = null
private var sharedflowId: Output? = null
/**
* @param value The resource ID of the environment.
*/
@JvmName("gxpgjrhycrtasnwr")
public suspend fun environment(`value`: Output) {
this.environment = value
}
/**
* @param value The Apigee Organization associated with the Sharedflow
*/
@JvmName("frueobcffuqlxude")
public suspend fun orgId(`value`: Output) {
this.orgId = value
}
/**
* @param value Revision of the Sharedflow to be deployed.
* - - -
*/
@JvmName("psvbuoywiwmcpmhh")
public suspend fun revision(`value`: Output) {
this.revision = value
}
/**
* @param value The service account represents the identity of the deployed proxy, and determines what permissions it has. The format must be {ACCOUNT_ID}@{PROJECT}.iam.gserviceaccount.com.
*/
@JvmName("ohpkyfhymclyhqsh")
public suspend fun serviceAccount(`value`: Output) {
this.serviceAccount = value
}
/**
* @param value Id of the Sharedflow to be deployed.
*/
@JvmName("tfpjucgtebxagicg")
public suspend fun sharedflowId(`value`: Output) {
this.sharedflowId = value
}
/**
* @param value The resource ID of the environment.
*/
@JvmName("onluhmuowddwfsjd")
public suspend fun environment(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.environment = mapped
}
/**
* @param value The Apigee Organization associated with the Sharedflow
*/
@JvmName("bjtvgpggscerykta")
public suspend fun orgId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.orgId = mapped
}
/**
* @param value Revision of the Sharedflow to be deployed.
* - - -
*/
@JvmName("ivecnyjsefnlkyyi")
public suspend fun revision(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.revision = mapped
}
/**
* @param value The service account represents the identity of the deployed proxy, and determines what permissions it has. The format must be {ACCOUNT_ID}@{PROJECT}.iam.gserviceaccount.com.
*/
@JvmName("ygthqgxbfbtuuehv")
public suspend fun serviceAccount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceAccount = mapped
}
/**
* @param value Id of the Sharedflow to be deployed.
*/
@JvmName("esmknkavgtfxjoln")
public suspend fun sharedflowId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharedflowId = mapped
}
internal fun build(): SharedflowDeploymentArgs = SharedflowDeploymentArgs(
environment = environment,
orgId = orgId,
revision = revision,
serviceAccount = serviceAccount,
sharedflowId = sharedflowId,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy