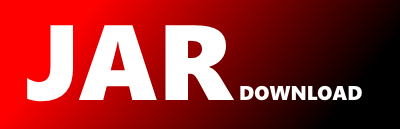
com.pulumi.gcp.apigee.kotlin.inputs.AddonsConfigAddonsConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.apigee.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.apigee.inputs.AddonsConfigAddonsConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property advancedApiOpsConfig Configuration for the Monetization add-on.
* Structure is documented below.
* @property apiSecurityConfig Configuration for the Monetization add-on.
* Structure is documented below.
* @property connectorsPlatformConfig Configuration for the Monetization add-on.
* Structure is documented below.
* @property integrationConfig Configuration for the Monetization add-on.
* Structure is documented below.
* @property monetizationConfig Configuration for the Monetization add-on.
* Structure is documented below.
*/
public data class AddonsConfigAddonsConfigArgs(
public val advancedApiOpsConfig: Output? = null,
public val apiSecurityConfig: Output? = null,
public val connectorsPlatformConfig: Output? =
null,
public val integrationConfig: Output? = null,
public val monetizationConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.apigee.inputs.AddonsConfigAddonsConfigArgs =
com.pulumi.gcp.apigee.inputs.AddonsConfigAddonsConfigArgs.builder()
.advancedApiOpsConfig(
advancedApiOpsConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.apiSecurityConfig(apiSecurityConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.connectorsPlatformConfig(
connectorsPlatformConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.integrationConfig(integrationConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monetizationConfig(
monetizationConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [AddonsConfigAddonsConfigArgs].
*/
@PulumiTagMarker
public class AddonsConfigAddonsConfigArgsBuilder internal constructor() {
private var advancedApiOpsConfig: Output? = null
private var apiSecurityConfig: Output? = null
private var connectorsPlatformConfig:
Output? = null
private var integrationConfig: Output? = null
private var monetizationConfig: Output? = null
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("juybuvqgcuxxwibs")
public suspend fun advancedApiOpsConfig(`value`: Output) {
this.advancedApiOpsConfig = value
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("osvvrgttmwwfjepg")
public suspend fun apiSecurityConfig(`value`: Output) {
this.apiSecurityConfig = value
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("koaytsfrfnbicijv")
public suspend fun connectorsPlatformConfig(`value`: Output) {
this.connectorsPlatformConfig = value
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("uwsyklfmrxqgehlo")
public suspend fun integrationConfig(`value`: Output) {
this.integrationConfig = value
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("sbmtyjwchpedpgym")
public suspend fun monetizationConfig(`value`: Output) {
this.monetizationConfig = value
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("jlofnlhpbxljwfkg")
public suspend fun advancedApiOpsConfig(`value`: AddonsConfigAddonsConfigAdvancedApiOpsConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.advancedApiOpsConfig = mapped
}
/**
* @param argument Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("ntfwlklyopgqvavb")
public suspend fun advancedApiOpsConfig(argument: suspend AddonsConfigAddonsConfigAdvancedApiOpsConfigArgsBuilder.() -> Unit) {
val toBeMapped = AddonsConfigAddonsConfigAdvancedApiOpsConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.advancedApiOpsConfig = mapped
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("ybsqhmfacrdiwoco")
public suspend fun apiSecurityConfig(`value`: AddonsConfigAddonsConfigApiSecurityConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiSecurityConfig = mapped
}
/**
* @param argument Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("spsgalqvcsmnidof")
public suspend fun apiSecurityConfig(argument: suspend AddonsConfigAddonsConfigApiSecurityConfigArgsBuilder.() -> Unit) {
val toBeMapped = AddonsConfigAddonsConfigApiSecurityConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.apiSecurityConfig = mapped
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("pfxqboajxophkpeq")
public suspend fun connectorsPlatformConfig(`value`: AddonsConfigAddonsConfigConnectorsPlatformConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorsPlatformConfig = mapped
}
/**
* @param argument Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("wlthuicxdwhfluxb")
public suspend fun connectorsPlatformConfig(argument: suspend AddonsConfigAddonsConfigConnectorsPlatformConfigArgsBuilder.() -> Unit) {
val toBeMapped = AddonsConfigAddonsConfigConnectorsPlatformConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.connectorsPlatformConfig = mapped
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("pjddmocmdxwfjwdp")
public suspend fun integrationConfig(`value`: AddonsConfigAddonsConfigIntegrationConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integrationConfig = mapped
}
/**
* @param argument Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("pabojcfqgsthaxcg")
public suspend fun integrationConfig(argument: suspend AddonsConfigAddonsConfigIntegrationConfigArgsBuilder.() -> Unit) {
val toBeMapped = AddonsConfigAddonsConfigIntegrationConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.integrationConfig = mapped
}
/**
* @param value Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("mrigbudawibkagkg")
public suspend fun monetizationConfig(`value`: AddonsConfigAddonsConfigMonetizationConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monetizationConfig = mapped
}
/**
* @param argument Configuration for the Monetization add-on.
* Structure is documented below.
*/
@JvmName("talhcgtdeqdbmcbb")
public suspend fun monetizationConfig(argument: suspend AddonsConfigAddonsConfigMonetizationConfigArgsBuilder.() -> Unit) {
val toBeMapped = AddonsConfigAddonsConfigMonetizationConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.monetizationConfig = mapped
}
internal fun build(): AddonsConfigAddonsConfigArgs = AddonsConfigAddonsConfigArgs(
advancedApiOpsConfig = advancedApiOpsConfig,
apiSecurityConfig = apiSecurityConfig,
connectorsPlatformConfig = connectorsPlatformConfig,
integrationConfig = integrationConfig,
monetizationConfig = monetizationConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy