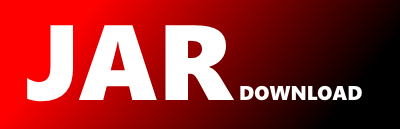
com.pulumi.gcp.apigee.kotlin.inputs.KeystoresAliasesKeyCertFileCertsInfoCertInfoArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.apigee.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.apigee.inputs.KeystoresAliasesKeyCertFileCertsInfoCertInfoArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property basicConstraints (Output)
* X.509 basic constraints extension.
* @property expiryDate (Output)
* X.509 notAfter validity period in milliseconds since epoch.
* @property isValid (Output)
* Flag that specifies whether the certificate is valid.
* Flag is set to Yes if the certificate is valid, No if expired, or Not yet if not yet valid.
* @property issuer (Output)
* X.509 issuer.
* @property publicKey (Output)
* Public key component of the X.509 subject public key info.
* @property serialNumber (Output)
* X.509 serial number.
* @property sigAlgName (Output)
* X.509 signatureAlgorithm.
* @property subject (Output)
* X.509 subject.
* @property subjectAlternativeNames (Output)
* X.509 subject alternative names (SANs) extension.
* @property validFrom (Output)
* X.509 notBefore validity period in milliseconds since epoch.
* @property version (Output)
* X.509 version.
*/
public data class KeystoresAliasesKeyCertFileCertsInfoCertInfoArgs(
public val basicConstraints: Output? = null,
public val expiryDate: Output? = null,
public val isValid: Output? = null,
public val issuer: Output? = null,
public val publicKey: Output? = null,
public val serialNumber: Output? = null,
public val sigAlgName: Output? = null,
public val subject: Output? = null,
public val subjectAlternativeNames: Output>? = null,
public val validFrom: Output? = null,
public val version: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.apigee.inputs.KeystoresAliasesKeyCertFileCertsInfoCertInfoArgs =
com.pulumi.gcp.apigee.inputs.KeystoresAliasesKeyCertFileCertsInfoCertInfoArgs.builder()
.basicConstraints(basicConstraints?.applyValue({ args0 -> args0 }))
.expiryDate(expiryDate?.applyValue({ args0 -> args0 }))
.isValid(isValid?.applyValue({ args0 -> args0 }))
.issuer(issuer?.applyValue({ args0 -> args0 }))
.publicKey(publicKey?.applyValue({ args0 -> args0 }))
.serialNumber(serialNumber?.applyValue({ args0 -> args0 }))
.sigAlgName(sigAlgName?.applyValue({ args0 -> args0 }))
.subject(subject?.applyValue({ args0 -> args0 }))
.subjectAlternativeNames(
subjectAlternativeNames?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.validFrom(validFrom?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KeystoresAliasesKeyCertFileCertsInfoCertInfoArgs].
*/
@PulumiTagMarker
public class KeystoresAliasesKeyCertFileCertsInfoCertInfoArgsBuilder internal constructor() {
private var basicConstraints: Output? = null
private var expiryDate: Output? = null
private var isValid: Output? = null
private var issuer: Output? = null
private var publicKey: Output? = null
private var serialNumber: Output? = null
private var sigAlgName: Output? = null
private var subject: Output? = null
private var subjectAlternativeNames: Output>? = null
private var validFrom: Output? = null
private var version: Output? = null
/**
* @param value (Output)
* X.509 basic constraints extension.
*/
@JvmName("saqmyomiahuwbjej")
public suspend fun basicConstraints(`value`: Output) {
this.basicConstraints = value
}
/**
* @param value (Output)
* X.509 notAfter validity period in milliseconds since epoch.
*/
@JvmName("qolicvhkfswtdhhs")
public suspend fun expiryDate(`value`: Output) {
this.expiryDate = value
}
/**
* @param value (Output)
* Flag that specifies whether the certificate is valid.
* Flag is set to Yes if the certificate is valid, No if expired, or Not yet if not yet valid.
*/
@JvmName("dvghwfdcjdavtslu")
public suspend fun isValid(`value`: Output) {
this.isValid = value
}
/**
* @param value (Output)
* X.509 issuer.
*/
@JvmName("pwnftpgkqxucygyq")
public suspend fun issuer(`value`: Output) {
this.issuer = value
}
/**
* @param value (Output)
* Public key component of the X.509 subject public key info.
*/
@JvmName("kstobdskolcxpica")
public suspend fun publicKey(`value`: Output) {
this.publicKey = value
}
/**
* @param value (Output)
* X.509 serial number.
*/
@JvmName("eeditttkujchabab")
public suspend fun serialNumber(`value`: Output) {
this.serialNumber = value
}
/**
* @param value (Output)
* X.509 signatureAlgorithm.
*/
@JvmName("rjmnkrsmcgafefil")
public suspend fun sigAlgName(`value`: Output) {
this.sigAlgName = value
}
/**
* @param value (Output)
* X.509 subject.
*/
@JvmName("nfyaqdwkoxnfelqw")
public suspend fun subject(`value`: Output) {
this.subject = value
}
/**
* @param value (Output)
* X.509 subject alternative names (SANs) extension.
*/
@JvmName("vwvqdmykpkcvahft")
public suspend fun subjectAlternativeNames(`value`: Output>) {
this.subjectAlternativeNames = value
}
@JvmName("osrngocejbajgrnk")
public suspend fun subjectAlternativeNames(vararg values: Output) {
this.subjectAlternativeNames = Output.all(values.asList())
}
/**
* @param values (Output)
* X.509 subject alternative names (SANs) extension.
*/
@JvmName("vkwmvxaqdtvttljl")
public suspend fun subjectAlternativeNames(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy