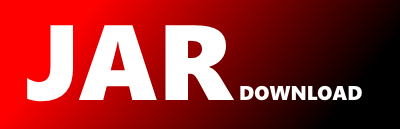
com.pulumi.gcp.appengine.kotlin.EngineSplitTrafficArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.appengine.EngineSplitTrafficArgs.builder
import com.pulumi.gcp.appengine.kotlin.inputs.EngineSplitTrafficSplitArgs
import com.pulumi.gcp.appengine.kotlin.inputs.EngineSplitTrafficSplitArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Traffic routing configuration for versions within a single service. Traffic splits define how traffic directed to the service is assigned to versions.
* To get more information about ServiceSplitTraffic, see:
* * [API documentation](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services)
* ## Example Usage
* ### App Engine Service Split Traffic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "appengine-static-content",
* location: "US",
* });
* const object = new gcp.storage.BucketObject("object", {
* name: "hello-world.zip",
* bucket: bucket.name,
* source: new pulumi.asset.FileAsset("./test-fixtures/hello-world.zip"),
* });
* const liveappV1 = new gcp.appengine.StandardAppVersion("liveapp_v1", {
* versionId: "v1",
* service: "liveapp",
* deleteServiceOnDestroy: true,
* runtime: "nodejs20",
* entrypoint: {
* shell: "node ./app.js",
* },
* deployment: {
* zip: {
* sourceUrl: pulumi.interpolate`https://storage.googleapis.com/${bucket.name}/${object.name}`,
* },
* },
* envVariables: {
* port: "8080",
* },
* });
* const liveappV2 = new gcp.appengine.StandardAppVersion("liveapp_v2", {
* versionId: "v2",
* service: "liveapp",
* noopOnDestroy: true,
* runtime: "nodejs20",
* entrypoint: {
* shell: "node ./app.js",
* },
* deployment: {
* zip: {
* sourceUrl: pulumi.interpolate`https://storage.googleapis.com/${bucket.name}/${object.name}`,
* },
* },
* envVariables: {
* port: "8080",
* },
* });
* const liveapp = new gcp.appengine.EngineSplitTraffic("liveapp", {
* service: liveappV2.service,
* migrateTraffic: false,
* split: {
* shardBy: "IP",
* allocations: pulumi.all([liveappV1.versionId, liveappV2.versionId]).apply(([liveappV1VersionId, liveappV2VersionId]) => {
* [liveappV1VersionId]: 0.75,
* [liveappV2VersionId]: 0.25,
* }),
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* bucket = gcp.storage.Bucket("bucket",
* name="appengine-static-content",
* location="US")
* object = gcp.storage.BucketObject("object",
* name="hello-world.zip",
* bucket=bucket.name,
* source=pulumi.FileAsset("./test-fixtures/hello-world.zip"))
* liveapp_v1 = gcp.appengine.StandardAppVersion("liveapp_v1",
* version_id="v1",
* service="liveapp",
* delete_service_on_destroy=True,
* runtime="nodejs20",
* entrypoint=gcp.appengine.StandardAppVersionEntrypointArgs(
* shell="node ./app.js",
* ),
* deployment=gcp.appengine.StandardAppVersionDeploymentArgs(
* zip=gcp.appengine.StandardAppVersionDeploymentZipArgs(
* source_url=pulumi.Output.all(bucket.name, object.name).apply(lambda bucketName, objectName: f"https://storage.googleapis.com/{bucket_name}/{object_name}"),
* ),
* ),
* env_variables={
* "port": "8080",
* })
* liveapp_v2 = gcp.appengine.StandardAppVersion("liveapp_v2",
* version_id="v2",
* service="liveapp",
* noop_on_destroy=True,
* runtime="nodejs20",
* entrypoint=gcp.appengine.StandardAppVersionEntrypointArgs(
* shell="node ./app.js",
* ),
* deployment=gcp.appengine.StandardAppVersionDeploymentArgs(
* zip=gcp.appengine.StandardAppVersionDeploymentZipArgs(
* source_url=pulumi.Output.all(bucket.name, object.name).apply(lambda bucketName, objectName: f"https://storage.googleapis.com/{bucket_name}/{object_name}"),
* ),
* ),
* env_variables={
* "port": "8080",
* })
* liveapp = gcp.appengine.EngineSplitTraffic("liveapp",
* service=liveapp_v2.service,
* migrate_traffic=False,
* split=gcp.appengine.EngineSplitTrafficSplitArgs(
* shard_by="IP",
* allocations=pulumi.Output.all(liveapp_v1.version_id, liveapp_v2.version_id).apply(lambda liveappV1Version_id, liveappV2Version_id: {
* liveapp_v1_version_id: 0.75,
* liveapp_v2_version_id: 0.25,
* }),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "appengine-static-content",
* Location = "US",
* });
* var @object = new Gcp.Storage.BucketObject("object", new()
* {
* Name = "hello-world.zip",
* Bucket = bucket.Name,
* Source = new FileAsset("./test-fixtures/hello-world.zip"),
* });
* var liveappV1 = new Gcp.AppEngine.StandardAppVersion("liveapp_v1", new()
* {
* VersionId = "v1",
* Service = "liveapp",
* DeleteServiceOnDestroy = true,
* Runtime = "nodejs20",
* Entrypoint = new Gcp.AppEngine.Inputs.StandardAppVersionEntrypointArgs
* {
* Shell = "node ./app.js",
* },
* Deployment = new Gcp.AppEngine.Inputs.StandardAppVersionDeploymentArgs
* {
* Zip = new Gcp.AppEngine.Inputs.StandardAppVersionDeploymentZipArgs
* {
* SourceUrl = Output.Tuple(bucket.Name, @object.Name).Apply(values =>
* {
* var bucketName = values.Item1;
* var objectName = values.Item2;
* return $"https://storage.googleapis.com/{bucketName}/{objectName}";
* }),
* },
* },
* EnvVariables =
* {
* { "port", "8080" },
* },
* });
* var liveappV2 = new Gcp.AppEngine.StandardAppVersion("liveapp_v2", new()
* {
* VersionId = "v2",
* Service = "liveapp",
* NoopOnDestroy = true,
* Runtime = "nodejs20",
* Entrypoint = new Gcp.AppEngine.Inputs.StandardAppVersionEntrypointArgs
* {
* Shell = "node ./app.js",
* },
* Deployment = new Gcp.AppEngine.Inputs.StandardAppVersionDeploymentArgs
* {
* Zip = new Gcp.AppEngine.Inputs.StandardAppVersionDeploymentZipArgs
* {
* SourceUrl = Output.Tuple(bucket.Name, @object.Name).Apply(values =>
* {
* var bucketName = values.Item1;
* var objectName = values.Item2;
* return $"https://storage.googleapis.com/{bucketName}/{objectName}";
* }),
* },
* },
* EnvVariables =
* {
* { "port", "8080" },
* },
* });
* var liveapp = new Gcp.AppEngine.EngineSplitTraffic("liveapp", new()
* {
* Service = liveappV2.Service,
* MigrateTraffic = false,
* Split = new Gcp.AppEngine.Inputs.EngineSplitTrafficSplitArgs
* {
* ShardBy = "IP",
* Allocations = Output.Tuple(liveappV1.VersionId, liveappV2.VersionId).Apply(values =>
* {
* var liveappV1VersionId = values.Item1;
* var liveappV2VersionId = values.Item2;
* return
* {
* { liveappV1VersionId, 0.75 },
* { liveappV2VersionId, 0.25 },
* };
* }),
* },
* });
* });
* ```
* ```yaml
* resources:
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: appengine-static-content
* location: US
* object:
* type: gcp:storage:BucketObject
* properties:
* name: hello-world.zip
* bucket: ${bucket.name}
* source:
* fn::FileAsset: ./test-fixtures/hello-world.zip
* liveappV1:
* type: gcp:appengine:StandardAppVersion
* name: liveapp_v1
* properties:
* versionId: v1
* service: liveapp
* deleteServiceOnDestroy: true
* runtime: nodejs20
* entrypoint:
* shell: node ./app.js
* deployment:
* zip:
* sourceUrl: https://storage.googleapis.com/${bucket.name}/${object.name}
* envVariables:
* port: '8080'
* liveappV2:
* type: gcp:appengine:StandardAppVersion
* name: liveapp_v2
* properties:
* versionId: v2
* service: liveapp
* noopOnDestroy: true
* runtime: nodejs20
* entrypoint:
* shell: node ./app.js
* deployment:
* zip:
* sourceUrl: https://storage.googleapis.com/${bucket.name}/${object.name}
* envVariables:
* port: '8080'
* liveapp:
* type: gcp:appengine:EngineSplitTraffic
* properties:
* service: ${liveappV2.service}
* migrateTraffic: false
* split:
* shardBy: IP
* allocations:
* ${liveappV1.versionId}: 0.75
* ${liveappV2.versionId}: 0.25
* ```
*
* ## Import
* ServiceSplitTraffic can be imported using any of these accepted formats:
* * `apps/{{project}}/services/{{service}}`
* * `{{project}}/{{service}}`
* * `{{service}}`
* When using the `pulumi import` command, ServiceSplitTraffic can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:appengine/engineSplitTraffic:EngineSplitTraffic default apps/{{project}}/services/{{service}}
* ```
* ```sh
* $ pulumi import gcp:appengine/engineSplitTraffic:EngineSplitTraffic default {{project}}/{{service}}
* ```
* ```sh
* $ pulumi import gcp:appengine/engineSplitTraffic:EngineSplitTraffic default {{service}}
* ```
* @property migrateTraffic If set to true traffic will be migrated to this version.
* @property project
* @property service The name of the service these settings apply to.
* @property split Mapping that defines fractional HTTP traffic diversion to different versions within the service.
* Structure is documented below.
*/
public data class EngineSplitTrafficArgs(
public val migrateTraffic: Output? = null,
public val project: Output? = null,
public val service: Output? = null,
public val split: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.appengine.EngineSplitTrafficArgs =
com.pulumi.gcp.appengine.EngineSplitTrafficArgs.builder()
.migrateTraffic(migrateTraffic?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.service(service?.applyValue({ args0 -> args0 }))
.split(split?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [EngineSplitTrafficArgs].
*/
@PulumiTagMarker
public class EngineSplitTrafficArgsBuilder internal constructor() {
private var migrateTraffic: Output? = null
private var project: Output? = null
private var service: Output? = null
private var split: Output? = null
/**
* @param value If set to true traffic will be migrated to this version.
*/
@JvmName("pynktcldncclukob")
public suspend fun migrateTraffic(`value`: Output) {
this.migrateTraffic = value
}
/**
* @param value
*/
@JvmName("bwktivsyiwgoxobx")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The name of the service these settings apply to.
*/
@JvmName("vcvhinbtkrqvgchx")
public suspend fun service(`value`: Output) {
this.service = value
}
/**
* @param value Mapping that defines fractional HTTP traffic diversion to different versions within the service.
* Structure is documented below.
*/
@JvmName("dgkgukbotdsqfbbv")
public suspend fun split(`value`: Output) {
this.split = value
}
/**
* @param value If set to true traffic will be migrated to this version.
*/
@JvmName("ejwmidupejqjsylj")
public suspend fun migrateTraffic(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.migrateTraffic = mapped
}
/**
* @param value
*/
@JvmName("pxoucishocysjydu")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The name of the service these settings apply to.
*/
@JvmName("wfurhpwynoduposs")
public suspend fun service(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.service = mapped
}
/**
* @param value Mapping that defines fractional HTTP traffic diversion to different versions within the service.
* Structure is documented below.
*/
@JvmName("lrqqrumqpqwldxmh")
public suspend fun split(`value`: EngineSplitTrafficSplitArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.split = mapped
}
/**
* @param argument Mapping that defines fractional HTTP traffic diversion to different versions within the service.
* Structure is documented below.
*/
@JvmName("afidnnhwxntbroli")
public suspend fun split(argument: suspend EngineSplitTrafficSplitArgsBuilder.() -> Unit) {
val toBeMapped = EngineSplitTrafficSplitArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.split = mapped
}
internal fun build(): EngineSplitTrafficArgs = EngineSplitTrafficArgs(
migrateTraffic = migrateTraffic,
project = project,
service = service,
split = split,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy