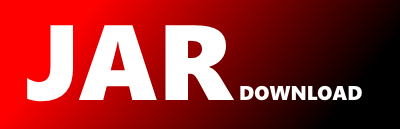
com.pulumi.gcp.appengine.kotlin.inputs.FlexibleAppVersionHandlerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.appengine.inputs.FlexibleAppVersionHandlerArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property authFailAction Actions to take when the user is not logged in.
* Possible values are: `AUTH_FAIL_ACTION_REDIRECT`, `AUTH_FAIL_ACTION_UNAUTHORIZED`.
* @property login Methods to restrict access to a URL based on login status.
* Possible values are: `LOGIN_OPTIONAL`, `LOGIN_ADMIN`, `LOGIN_REQUIRED`.
* @property redirectHttpResponseCode 30x code to use when performing redirects for the secure field.
* Possible values are: `REDIRECT_HTTP_RESPONSE_CODE_301`, `REDIRECT_HTTP_RESPONSE_CODE_302`, `REDIRECT_HTTP_RESPONSE_CODE_303`, `REDIRECT_HTTP_RESPONSE_CODE_307`.
* @property script Executes a script to handle the requests that match this URL pattern.
* Only the auto value is supported for Node.js in the App Engine standard environment, for example "script:" "auto".
* Structure is documented below.
* @property securityLevel Security (HTTPS) enforcement for this URL.
* Possible values are: `SECURE_DEFAULT`, `SECURE_NEVER`, `SECURE_OPTIONAL`, `SECURE_ALWAYS`.
* @property staticFiles Files served directly to the user for a given URL, such as images, CSS stylesheets, or JavaScript source files.
* Static file handlers describe which files in the application directory are static files, and which URLs serve them.
* Structure is documented below.
* @property urlRegex URL prefix. Uses regular expression syntax, which means regexp special characters must be escaped, but should not contain groupings.
* All URLs that begin with this prefix are handled by this handler, using the portion of the URL after the prefix as part of the file path.
*/
public data class FlexibleAppVersionHandlerArgs(
public val authFailAction: Output? = null,
public val login: Output? = null,
public val redirectHttpResponseCode: Output? = null,
public val script: Output? = null,
public val securityLevel: Output? = null,
public val staticFiles: Output? = null,
public val urlRegex: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.appengine.inputs.FlexibleAppVersionHandlerArgs =
com.pulumi.gcp.appengine.inputs.FlexibleAppVersionHandlerArgs.builder()
.authFailAction(authFailAction?.applyValue({ args0 -> args0 }))
.login(login?.applyValue({ args0 -> args0 }))
.redirectHttpResponseCode(redirectHttpResponseCode?.applyValue({ args0 -> args0 }))
.script(script?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.securityLevel(securityLevel?.applyValue({ args0 -> args0 }))
.staticFiles(staticFiles?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.urlRegex(urlRegex?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FlexibleAppVersionHandlerArgs].
*/
@PulumiTagMarker
public class FlexibleAppVersionHandlerArgsBuilder internal constructor() {
private var authFailAction: Output? = null
private var login: Output? = null
private var redirectHttpResponseCode: Output? = null
private var script: Output? = null
private var securityLevel: Output? = null
private var staticFiles: Output? = null
private var urlRegex: Output? = null
/**
* @param value Actions to take when the user is not logged in.
* Possible values are: `AUTH_FAIL_ACTION_REDIRECT`, `AUTH_FAIL_ACTION_UNAUTHORIZED`.
*/
@JvmName("kpqelywwkdnnnupw")
public suspend fun authFailAction(`value`: Output) {
this.authFailAction = value
}
/**
* @param value Methods to restrict access to a URL based on login status.
* Possible values are: `LOGIN_OPTIONAL`, `LOGIN_ADMIN`, `LOGIN_REQUIRED`.
*/
@JvmName("yvqcwbqxayxltprp")
public suspend fun login(`value`: Output) {
this.login = value
}
/**
* @param value 30x code to use when performing redirects for the secure field.
* Possible values are: `REDIRECT_HTTP_RESPONSE_CODE_301`, `REDIRECT_HTTP_RESPONSE_CODE_302`, `REDIRECT_HTTP_RESPONSE_CODE_303`, `REDIRECT_HTTP_RESPONSE_CODE_307`.
*/
@JvmName("npkvfsqrhabvdhdh")
public suspend fun redirectHttpResponseCode(`value`: Output) {
this.redirectHttpResponseCode = value
}
/**
* @param value Executes a script to handle the requests that match this URL pattern.
* Only the auto value is supported for Node.js in the App Engine standard environment, for example "script:" "auto".
* Structure is documented below.
*/
@JvmName("emhrtbvvhaaifdwq")
public suspend fun script(`value`: Output) {
this.script = value
}
/**
* @param value Security (HTTPS) enforcement for this URL.
* Possible values are: `SECURE_DEFAULT`, `SECURE_NEVER`, `SECURE_OPTIONAL`, `SECURE_ALWAYS`.
*/
@JvmName("ryxucvulasbxhyrk")
public suspend fun securityLevel(`value`: Output) {
this.securityLevel = value
}
/**
* @param value Files served directly to the user for a given URL, such as images, CSS stylesheets, or JavaScript source files.
* Static file handlers describe which files in the application directory are static files, and which URLs serve them.
* Structure is documented below.
*/
@JvmName("omgekwrvfwwvexjv")
public suspend fun staticFiles(`value`: Output) {
this.staticFiles = value
}
/**
* @param value URL prefix. Uses regular expression syntax, which means regexp special characters must be escaped, but should not contain groupings.
* All URLs that begin with this prefix are handled by this handler, using the portion of the URL after the prefix as part of the file path.
*/
@JvmName("gmtqdrmixrvfdbch")
public suspend fun urlRegex(`value`: Output) {
this.urlRegex = value
}
/**
* @param value Actions to take when the user is not logged in.
* Possible values are: `AUTH_FAIL_ACTION_REDIRECT`, `AUTH_FAIL_ACTION_UNAUTHORIZED`.
*/
@JvmName("kbhhbodsblhnwxtu")
public suspend fun authFailAction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authFailAction = mapped
}
/**
* @param value Methods to restrict access to a URL based on login status.
* Possible values are: `LOGIN_OPTIONAL`, `LOGIN_ADMIN`, `LOGIN_REQUIRED`.
*/
@JvmName("bymwvkblbvsxoojy")
public suspend fun login(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.login = mapped
}
/**
* @param value 30x code to use when performing redirects for the secure field.
* Possible values are: `REDIRECT_HTTP_RESPONSE_CODE_301`, `REDIRECT_HTTP_RESPONSE_CODE_302`, `REDIRECT_HTTP_RESPONSE_CODE_303`, `REDIRECT_HTTP_RESPONSE_CODE_307`.
*/
@JvmName("mfmhbbbmxuipsihh")
public suspend fun redirectHttpResponseCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redirectHttpResponseCode = mapped
}
/**
* @param value Executes a script to handle the requests that match this URL pattern.
* Only the auto value is supported for Node.js in the App Engine standard environment, for example "script:" "auto".
* Structure is documented below.
*/
@JvmName("vkicusebieoewkuj")
public suspend fun script(`value`: FlexibleAppVersionHandlerScriptArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.script = mapped
}
/**
* @param argument Executes a script to handle the requests that match this URL pattern.
* Only the auto value is supported for Node.js in the App Engine standard environment, for example "script:" "auto".
* Structure is documented below.
*/
@JvmName("gxfkmeuoincgfenv")
public suspend fun script(argument: suspend FlexibleAppVersionHandlerScriptArgsBuilder.() -> Unit) {
val toBeMapped = FlexibleAppVersionHandlerScriptArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.script = mapped
}
/**
* @param value Security (HTTPS) enforcement for this URL.
* Possible values are: `SECURE_DEFAULT`, `SECURE_NEVER`, `SECURE_OPTIONAL`, `SECURE_ALWAYS`.
*/
@JvmName("cgjylvkryfbckbeo")
public suspend fun securityLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securityLevel = mapped
}
/**
* @param value Files served directly to the user for a given URL, such as images, CSS stylesheets, or JavaScript source files.
* Static file handlers describe which files in the application directory are static files, and which URLs serve them.
* Structure is documented below.
*/
@JvmName("gykstlqyhkvqfrka")
public suspend fun staticFiles(`value`: FlexibleAppVersionHandlerStaticFilesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.staticFiles = mapped
}
/**
* @param argument Files served directly to the user for a given URL, such as images, CSS stylesheets, or JavaScript source files.
* Static file handlers describe which files in the application directory are static files, and which URLs serve them.
* Structure is documented below.
*/
@JvmName("aojhvhbrpdkrhnxg")
public suspend fun staticFiles(argument: suspend FlexibleAppVersionHandlerStaticFilesArgsBuilder.() -> Unit) {
val toBeMapped = FlexibleAppVersionHandlerStaticFilesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.staticFiles = mapped
}
/**
* @param value URL prefix. Uses regular expression syntax, which means regexp special characters must be escaped, but should not contain groupings.
* All URLs that begin with this prefix are handled by this handler, using the portion of the URL after the prefix as part of the file path.
*/
@JvmName("tdlbyqxwkmaryjrl")
public suspend fun urlRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.urlRegex = mapped
}
internal fun build(): FlexibleAppVersionHandlerArgs = FlexibleAppVersionHandlerArgs(
authFailAction = authFailAction,
login = login,
redirectHttpResponseCode = redirectHttpResponseCode,
script = script,
securityLevel = securityLevel,
staticFiles = staticFiles,
urlRegex = urlRegex,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy