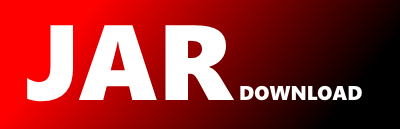
com.pulumi.gcp.appengine.kotlin.inputs.FlexibleAppVersionLivenessCheckArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.appengine.inputs.FlexibleAppVersionLivenessCheckArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property checkInterval Interval between health checks.
* @property failureThreshold Number of consecutive failed checks required before considering the VM unhealthy. Default: 4.
* @property host Host header to send when performing a HTTP Readiness check. Example: "myapp.appspot.com"
* @property initialDelay The initial delay before starting to execute the checks. Default: "300s"
* - - -
* @property path The request path.
* @property successThreshold Number of consecutive successful checks required before considering the VM healthy. Default: 2.
* @property timeout Time before the check is considered failed. Default: "4s"
*/
public data class FlexibleAppVersionLivenessCheckArgs(
public val checkInterval: Output? = null,
public val failureThreshold: Output? = null,
public val host: Output? = null,
public val initialDelay: Output? = null,
public val path: Output,
public val successThreshold: Output? = null,
public val timeout: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.appengine.inputs.FlexibleAppVersionLivenessCheckArgs =
com.pulumi.gcp.appengine.inputs.FlexibleAppVersionLivenessCheckArgs.builder()
.checkInterval(checkInterval?.applyValue({ args0 -> args0 }))
.failureThreshold(failureThreshold?.applyValue({ args0 -> args0 }))
.host(host?.applyValue({ args0 -> args0 }))
.initialDelay(initialDelay?.applyValue({ args0 -> args0 }))
.path(path.applyValue({ args0 -> args0 }))
.successThreshold(successThreshold?.applyValue({ args0 -> args0 }))
.timeout(timeout?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FlexibleAppVersionLivenessCheckArgs].
*/
@PulumiTagMarker
public class FlexibleAppVersionLivenessCheckArgsBuilder internal constructor() {
private var checkInterval: Output? = null
private var failureThreshold: Output? = null
private var host: Output? = null
private var initialDelay: Output? = null
private var path: Output? = null
private var successThreshold: Output? = null
private var timeout: Output? = null
/**
* @param value Interval between health checks.
*/
@JvmName("qxruqbmfjejqeoea")
public suspend fun checkInterval(`value`: Output) {
this.checkInterval = value
}
/**
* @param value Number of consecutive failed checks required before considering the VM unhealthy. Default: 4.
*/
@JvmName("mrewkbhgiibvbfxf")
public suspend fun failureThreshold(`value`: Output) {
this.failureThreshold = value
}
/**
* @param value Host header to send when performing a HTTP Readiness check. Example: "myapp.appspot.com"
*/
@JvmName("iysgyggemjaunftx")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value The initial delay before starting to execute the checks. Default: "300s"
* - - -
*/
@JvmName("jrvwwylybeurmkhg")
public suspend fun initialDelay(`value`: Output) {
this.initialDelay = value
}
/**
* @param value The request path.
*/
@JvmName("rwdfdbcbdudfvltq")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value Number of consecutive successful checks required before considering the VM healthy. Default: 2.
*/
@JvmName("kukxmxxlfmoapglm")
public suspend fun successThreshold(`value`: Output) {
this.successThreshold = value
}
/**
* @param value Time before the check is considered failed. Default: "4s"
*/
@JvmName("xembhoungswdxbay")
public suspend fun timeout(`value`: Output) {
this.timeout = value
}
/**
* @param value Interval between health checks.
*/
@JvmName("ocsqpijjhyiigias")
public suspend fun checkInterval(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.checkInterval = mapped
}
/**
* @param value Number of consecutive failed checks required before considering the VM unhealthy. Default: 4.
*/
@JvmName("ggmcgpvdfbgcuktc")
public suspend fun failureThreshold(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureThreshold = mapped
}
/**
* @param value Host header to send when performing a HTTP Readiness check. Example: "myapp.appspot.com"
*/
@JvmName("wceuoulrnfyninqp")
public suspend fun host(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value The initial delay before starting to execute the checks. Default: "300s"
* - - -
*/
@JvmName("roybplpcpejvarcd")
public suspend fun initialDelay(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.initialDelay = mapped
}
/**
* @param value The request path.
*/
@JvmName("tlfbpvxamplwctqi")
public suspend fun path(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value Number of consecutive successful checks required before considering the VM healthy. Default: 2.
*/
@JvmName("inklvvpkvqqnoxjc")
public suspend fun successThreshold(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successThreshold = mapped
}
/**
* @param value Time before the check is considered failed. Default: "4s"
*/
@JvmName("enhlvistgcgwnliq")
public suspend fun timeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeout = mapped
}
internal fun build(): FlexibleAppVersionLivenessCheckArgs = FlexibleAppVersionLivenessCheckArgs(
checkInterval = checkInterval,
failureThreshold = failureThreshold,
host = host,
initialDelay = initialDelay,
path = path ?: throw PulumiNullFieldException("path"),
successThreshold = successThreshold,
timeout = timeout,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy