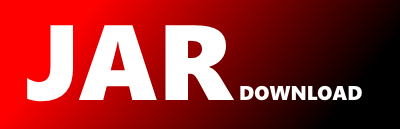
com.pulumi.gcp.appengine.kotlin.inputs.FlexibleAppVersionNetworkArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.appengine.inputs.FlexibleAppVersionNetworkArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property forwardedPorts List of ports, or port pairs, to forward from the virtual machine to the application container.
* @property instanceTag Tag to apply to the instance during creation.
* @property name Google Compute Engine network where the virtual machines are created. Specify the short name, not the resource path.
* @property sessionAffinity Enable session affinity.
* @property subnetwork Google Cloud Platform sub-network where the virtual machines are created. Specify the short name, not the resource path.
* If the network that the instance is being created in is a Legacy network, then the IP address is allocated from the IPv4Range.
* If the network that the instance is being created in is an auto Subnet Mode Network, then only network name should be specified (not the subnetworkName) and the IP address is created from the IPCidrRange of the subnetwork that exists in that zone for that network.
* If the network that the instance is being created in is a custom Subnet Mode Network, then the subnetworkName must be specified and the IP address is created from the IPCidrRange of the subnetwork.
* If specified, the subnetwork must exist in the same region as the App Engine flexible environment application.
*/
public data class FlexibleAppVersionNetworkArgs(
public val forwardedPorts: Output>? = null,
public val instanceTag: Output? = null,
public val name: Output,
public val sessionAffinity: Output? = null,
public val subnetwork: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.appengine.inputs.FlexibleAppVersionNetworkArgs =
com.pulumi.gcp.appengine.inputs.FlexibleAppVersionNetworkArgs.builder()
.forwardedPorts(forwardedPorts?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.instanceTag(instanceTag?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.sessionAffinity(sessionAffinity?.applyValue({ args0 -> args0 }))
.subnetwork(subnetwork?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FlexibleAppVersionNetworkArgs].
*/
@PulumiTagMarker
public class FlexibleAppVersionNetworkArgsBuilder internal constructor() {
private var forwardedPorts: Output>? = null
private var instanceTag: Output? = null
private var name: Output? = null
private var sessionAffinity: Output? = null
private var subnetwork: Output? = null
/**
* @param value List of ports, or port pairs, to forward from the virtual machine to the application container.
*/
@JvmName("vfqijktvgpmtewtl")
public suspend fun forwardedPorts(`value`: Output>) {
this.forwardedPorts = value
}
@JvmName("pekbsqkhgwauaslq")
public suspend fun forwardedPorts(vararg values: Output) {
this.forwardedPorts = Output.all(values.asList())
}
/**
* @param values List of ports, or port pairs, to forward from the virtual machine to the application container.
*/
@JvmName("vqbacouqiwsesmla")
public suspend fun forwardedPorts(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy