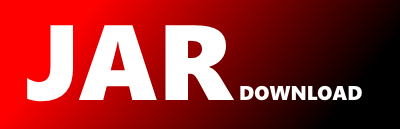
com.pulumi.gcp.appengine.kotlin.inputs.StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.appengine.inputs.StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property maxInstances Maximum number of instances to run for this version. Set to zero to disable maxInstances configuration.
* @property minInstances Minimum number of instances to run for this version. Set to zero to disable minInstances configuration.
* @property targetCpuUtilization Target CPU utilization ratio to maintain when scaling. Should be a value in the range [0.50, 0.95], zero, or a negative value.
* @property targetThroughputUtilization Target throughput utilization ratio to maintain when scaling. Should be a value in the range [0.50, 0.95], zero, or a negative value.
*/
public data class StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs(
public val maxInstances: Output? = null,
public val minInstances: Output? = null,
public val targetCpuUtilization: Output? = null,
public val targetThroughputUtilization: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.appengine.inputs.StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs =
com.pulumi.gcp.appengine.inputs.StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs.builder()
.maxInstances(maxInstances?.applyValue({ args0 -> args0 }))
.minInstances(minInstances?.applyValue({ args0 -> args0 }))
.targetCpuUtilization(targetCpuUtilization?.applyValue({ args0 -> args0 }))
.targetThroughputUtilization(targetThroughputUtilization?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs].
*/
@PulumiTagMarker
public class StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgsBuilder internal constructor() {
private var maxInstances: Output? = null
private var minInstances: Output? = null
private var targetCpuUtilization: Output? = null
private var targetThroughputUtilization: Output? = null
/**
* @param value Maximum number of instances to run for this version. Set to zero to disable maxInstances configuration.
*/
@JvmName("svdlyrlyyrwtolyg")
public suspend fun maxInstances(`value`: Output) {
this.maxInstances = value
}
/**
* @param value Minimum number of instances to run for this version. Set to zero to disable minInstances configuration.
*/
@JvmName("lepykskcheffdllt")
public suspend fun minInstances(`value`: Output) {
this.minInstances = value
}
/**
* @param value Target CPU utilization ratio to maintain when scaling. Should be a value in the range [0.50, 0.95], zero, or a negative value.
*/
@JvmName("mfogstmqukvxhnii")
public suspend fun targetCpuUtilization(`value`: Output) {
this.targetCpuUtilization = value
}
/**
* @param value Target throughput utilization ratio to maintain when scaling. Should be a value in the range [0.50, 0.95], zero, or a negative value.
*/
@JvmName("mrajqnnpcvrrqsix")
public suspend fun targetThroughputUtilization(`value`: Output) {
this.targetThroughputUtilization = value
}
/**
* @param value Maximum number of instances to run for this version. Set to zero to disable maxInstances configuration.
*/
@JvmName("aofekknsjlxvtatt")
public suspend fun maxInstances(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxInstances = mapped
}
/**
* @param value Minimum number of instances to run for this version. Set to zero to disable minInstances configuration.
*/
@JvmName("etgdmcktakcwfxhk")
public suspend fun minInstances(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minInstances = mapped
}
/**
* @param value Target CPU utilization ratio to maintain when scaling. Should be a value in the range [0.50, 0.95], zero, or a negative value.
*/
@JvmName("wlriunpqnjeaqrtm")
public suspend fun targetCpuUtilization(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetCpuUtilization = mapped
}
/**
* @param value Target throughput utilization ratio to maintain when scaling. Should be a value in the range [0.50, 0.95], zero, or a negative value.
*/
@JvmName("kfwslhhhgxwvfhwv")
public suspend fun targetThroughputUtilization(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetThroughputUtilization = mapped
}
internal fun build(): StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs =
StandardAppVersionAutomaticScalingStandardSchedulerSettingsArgs(
maxInstances = maxInstances,
minInstances = minInstances,
targetCpuUtilization = targetCpuUtilization,
targetThroughputUtilization = targetThroughputUtilization,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy