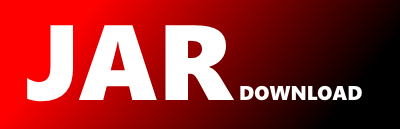
com.pulumi.gcp.appengine.kotlin.inputs.StandardAppVersionHandlerStaticFilesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.appengine.inputs.StandardAppVersionHandlerStaticFilesArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property applicationReadable Whether files should also be uploaded as code data. By default, files declared in static file handlers are uploaded as
* static data and are only served to end users; they cannot be read by the application. If enabled, uploads are charged
* against both your code and static data storage resource quotas.
* @property expiration Time a static file served by this handler should be cached by web proxies and browsers.
* A duration in seconds with up to nine fractional digits, terminated by 's'. Example "3.5s".
* @property httpHeaders HTTP headers to use for all responses from these URLs.
* An object containing a list of "key:value" value pairs.".
* @property mimeType MIME type used to serve all files served by this handler.
* Defaults to file-specific MIME types, which are derived from each file's filename extension.
* @property path Path to the static files matched by the URL pattern, from the application root directory. The path can refer to text matched in groupings in the URL pattern.
* @property requireMatchingFile Whether this handler should match the request if the file referenced by the handler does not exist.
* @property uploadPathRegex Regular expression that matches the file paths for all files that should be referenced by this handler.
*/
public data class StandardAppVersionHandlerStaticFilesArgs(
public val applicationReadable: Output? = null,
public val expiration: Output? = null,
public val httpHeaders: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy