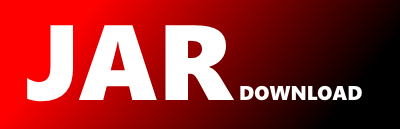
com.pulumi.gcp.appengine.kotlin.outputs.FlexibleAppVersionAutomaticScaling.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.appengine.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property coolDownPeriod The time period that the Autoscaler should wait before it starts collecting information from a new instance.
* This prevents the autoscaler from collecting information when the instance is initializing,
* during which the collected usage would not be reliable. Default: 120s
* @property cpuUtilization Target scaling by CPU usage.
* Structure is documented below.
* @property diskUtilization Target scaling by disk usage.
* Structure is documented below.
* @property maxConcurrentRequests Number of concurrent requests an automatic scaling instance can accept before the scheduler spawns a new instance.
* Defaults to a runtime-specific value.
* @property maxIdleInstances Maximum number of idle instances that should be maintained for this version.
* @property maxPendingLatency Maximum amount of time that a request should wait in the pending queue before starting a new instance to handle it.
* @property maxTotalInstances Maximum number of instances that should be started to handle requests for this version. Default: 20
* @property minIdleInstances Minimum number of idle instances that should be maintained for this version. Only applicable for the default version of a service.
* @property minPendingLatency Minimum amount of time a request should wait in the pending queue before starting a new instance to handle it.
* @property minTotalInstances Minimum number of running instances that should be maintained for this version. Default: 2
* @property networkUtilization Target scaling by network usage.
* Structure is documented below.
* @property requestUtilization Target scaling by request utilization.
* Structure is documented below.
*/
public data class FlexibleAppVersionAutomaticScaling(
public val coolDownPeriod: String? = null,
public val cpuUtilization: FlexibleAppVersionAutomaticScalingCpuUtilization,
public val diskUtilization: FlexibleAppVersionAutomaticScalingDiskUtilization? = null,
public val maxConcurrentRequests: Int? = null,
public val maxIdleInstances: Int? = null,
public val maxPendingLatency: String? = null,
public val maxTotalInstances: Int? = null,
public val minIdleInstances: Int? = null,
public val minPendingLatency: String? = null,
public val minTotalInstances: Int? = null,
public val networkUtilization: FlexibleAppVersionAutomaticScalingNetworkUtilization? = null,
public val requestUtilization: FlexibleAppVersionAutomaticScalingRequestUtilization? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.appengine.outputs.FlexibleAppVersionAutomaticScaling): FlexibleAppVersionAutomaticScaling = FlexibleAppVersionAutomaticScaling(
coolDownPeriod = javaType.coolDownPeriod().map({ args0 -> args0 }).orElse(null),
cpuUtilization = javaType.cpuUtilization().let({ args0 ->
com.pulumi.gcp.appengine.kotlin.outputs.FlexibleAppVersionAutomaticScalingCpuUtilization.Companion.toKotlin(args0)
}),
diskUtilization = javaType.diskUtilization().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.appengine.kotlin.outputs.FlexibleAppVersionAutomaticScalingDiskUtilization.Companion.toKotlin(args0)
})
}).orElse(null),
maxConcurrentRequests = javaType.maxConcurrentRequests().map({ args0 -> args0 }).orElse(null),
maxIdleInstances = javaType.maxIdleInstances().map({ args0 -> args0 }).orElse(null),
maxPendingLatency = javaType.maxPendingLatency().map({ args0 -> args0 }).orElse(null),
maxTotalInstances = javaType.maxTotalInstances().map({ args0 -> args0 }).orElse(null),
minIdleInstances = javaType.minIdleInstances().map({ args0 -> args0 }).orElse(null),
minPendingLatency = javaType.minPendingLatency().map({ args0 -> args0 }).orElse(null),
minTotalInstances = javaType.minTotalInstances().map({ args0 -> args0 }).orElse(null),
networkUtilization = javaType.networkUtilization().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.appengine.kotlin.outputs.FlexibleAppVersionAutomaticScalingNetworkUtilization.Companion.toKotlin(args0)
})
}).orElse(null),
requestUtilization = javaType.requestUtilization().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.appengine.kotlin.outputs.FlexibleAppVersionAutomaticScalingRequestUtilization.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy