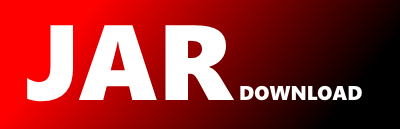
com.pulumi.gcp.applicationintegration.kotlin.AuthConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.applicationintegration.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.applicationintegration.AuthConfigArgs.builder
import com.pulumi.gcp.applicationintegration.kotlin.inputs.AuthConfigClientCertificateArgs
import com.pulumi.gcp.applicationintegration.kotlin.inputs.AuthConfigClientCertificateArgsBuilder
import com.pulumi.gcp.applicationintegration.kotlin.inputs.AuthConfigDecryptedCredentialArgs
import com.pulumi.gcp.applicationintegration.kotlin.inputs.AuthConfigDecryptedCredentialArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The AuthConfig resource use to hold channels and connection config data.
* To get more information about AuthConfig, see:
* * [API documentation](https://cloud.google.com/application-integration/docs/reference/rest/v1/projects.locations.authConfigs)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/application-integration/docs/overview)
* * [Manage authentication profiles](https://cloud.google.com/application-integration/docs/configure-authentication-profiles)
* ## Example Usage
* ### Integrations Auth Config Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const client = new gcp.applicationintegration.Client("client", {location: "us-west1"});
* const basicExample = new gcp.applicationintegration.AuthConfig("basic_example", {
* location: "us-west1",
* displayName: "test-authconfig",
* description: "Test auth config created via terraform",
* decryptedCredential: {
* credentialType: "USERNAME_AND_PASSWORD",
* usernameAndPassword: {
* username: "test-username",
* password: "test-password",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* client = gcp.applicationintegration.Client("client", location="us-west1")
* basic_example = gcp.applicationintegration.AuthConfig("basic_example",
* location="us-west1",
* display_name="test-authconfig",
* description="Test auth config created via terraform",
* decrypted_credential=gcp.applicationintegration.AuthConfigDecryptedCredentialArgs(
* credential_type="USERNAME_AND_PASSWORD",
* username_and_password=gcp.applicationintegration.AuthConfigDecryptedCredentialUsernameAndPasswordArgs(
* username="test-username",
* password="test-password",
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var client = new Gcp.ApplicationIntegration.Client("client", new()
* {
* Location = "us-west1",
* });
* var basicExample = new Gcp.ApplicationIntegration.AuthConfig("basic_example", new()
* {
* Location = "us-west1",
* DisplayName = "test-authconfig",
* Description = "Test auth config created via terraform",
* DecryptedCredential = new Gcp.ApplicationIntegration.Inputs.AuthConfigDecryptedCredentialArgs
* {
* CredentialType = "USERNAME_AND_PASSWORD",
* UsernameAndPassword = new Gcp.ApplicationIntegration.Inputs.AuthConfigDecryptedCredentialUsernameAndPasswordArgs
* {
* Username = "test-username",
* Password = "test-password",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/applicationintegration"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := applicationintegration.NewClient(ctx, "client", &applicationintegration.ClientArgs{
* Location: pulumi.String("us-west1"),
* })
* if err != nil {
* return err
* }
* _, err = applicationintegration.NewAuthConfig(ctx, "basic_example", &applicationintegration.AuthConfigArgs{
* Location: pulumi.String("us-west1"),
* DisplayName: pulumi.String("test-authconfig"),
* Description: pulumi.String("Test auth config created via terraform"),
* DecryptedCredential: &applicationintegration.AuthConfigDecryptedCredentialArgs{
* CredentialType: pulumi.String("USERNAME_AND_PASSWORD"),
* UsernameAndPassword: &applicationintegration.AuthConfigDecryptedCredentialUsernameAndPasswordArgs{
* Username: pulumi.String("test-username"),
* Password: pulumi.String("test-password"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.applicationintegration.Client;
* import com.pulumi.gcp.applicationintegration.ClientArgs;
* import com.pulumi.gcp.applicationintegration.AuthConfig;
* import com.pulumi.gcp.applicationintegration.AuthConfigArgs;
* import com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialArgs;
* import com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialUsernameAndPasswordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var client = new Client("client", ClientArgs.builder()
* .location("us-west1")
* .build());
* var basicExample = new AuthConfig("basicExample", AuthConfigArgs.builder()
* .location("us-west1")
* .displayName("test-authconfig")
* .description("Test auth config created via terraform")
* .decryptedCredential(AuthConfigDecryptedCredentialArgs.builder()
* .credentialType("USERNAME_AND_PASSWORD")
* .usernameAndPassword(AuthConfigDecryptedCredentialUsernameAndPasswordArgs.builder()
* .username("test-username")
* .password("test-password")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* client:
* type: gcp:applicationintegration:Client
* properties:
* location: us-west1
* basicExample:
* type: gcp:applicationintegration:AuthConfig
* name: basic_example
* properties:
* location: us-west1
* displayName: test-authconfig
* description: Test auth config created via terraform
* decryptedCredential:
* credentialType: USERNAME_AND_PASSWORD
* usernameAndPassword:
* username: test-username
* password: test-password
* ```
*
* ## Import
* AuthConfig can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, AuthConfig can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:applicationintegration/authConfig:AuthConfig default {{name}}
* ```
* @property clientCertificate Raw client certificate
* Structure is documented below.
* @property decryptedCredential Raw auth credentials.
* Structure is documented below.
* @property description A description of the auth config.
* @property displayName The name of the auth config.
* @property expiryNotificationDurations User can define the time to receive notification after which the auth config becomes invalid. Support up to 30 days. Support granularity in hours.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
* @property location Location in which client needs to be provisioned.
* - - -
* @property overrideValidTime User provided expiry time to override. For the example of Salesforce, username/password credentials can be valid for 6 months depending on the instance settings.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property visibility The visibility of the auth config.
* Possible values are: `PRIVATE`, `CLIENT_VISIBLE`.
*/
public data class AuthConfigArgs(
public val clientCertificate: Output? = null,
public val decryptedCredential: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val expiryNotificationDurations: Output>? = null,
public val location: Output? = null,
public val overrideValidTime: Output? = null,
public val project: Output? = null,
public val visibility: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.applicationintegration.AuthConfigArgs =
com.pulumi.gcp.applicationintegration.AuthConfigArgs.builder()
.clientCertificate(clientCertificate?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.decryptedCredential(
decryptedCredential?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.expiryNotificationDurations(
expiryNotificationDurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.location(location?.applyValue({ args0 -> args0 }))
.overrideValidTime(overrideValidTime?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.visibility(visibility?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AuthConfigArgs].
*/
@PulumiTagMarker
public class AuthConfigArgsBuilder internal constructor() {
private var clientCertificate: Output? = null
private var decryptedCredential: Output? = null
private var description: Output? = null
private var displayName: Output? = null
private var expiryNotificationDurations: Output>? = null
private var location: Output? = null
private var overrideValidTime: Output? = null
private var project: Output? = null
private var visibility: Output? = null
/**
* @param value Raw client certificate
* Structure is documented below.
*/
@JvmName("gganbvtcfjlbaiyq")
public suspend fun clientCertificate(`value`: Output) {
this.clientCertificate = value
}
/**
* @param value Raw auth credentials.
* Structure is documented below.
*/
@JvmName("scqdkeiyhljwycbg")
public suspend fun decryptedCredential(`value`: Output) {
this.decryptedCredential = value
}
/**
* @param value A description of the auth config.
*/
@JvmName("oayfqmqdcnufhjix")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name of the auth config.
*/
@JvmName("iyidjybgdbpdpycc")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value User can define the time to receive notification after which the auth config becomes invalid. Support up to 30 days. Support granularity in hours.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
@JvmName("necjmloqmwjuuuif")
public suspend fun expiryNotificationDurations(`value`: Output>) {
this.expiryNotificationDurations = value
}
@JvmName("bydgubsrsagcxveh")
public suspend fun expiryNotificationDurations(vararg values: Output) {
this.expiryNotificationDurations = Output.all(values.asList())
}
/**
* @param values User can define the time to receive notification after which the auth config becomes invalid. Support up to 30 days. Support granularity in hours.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
@JvmName("bbaddtyjdkpdvpax")
public suspend fun expiryNotificationDurations(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy