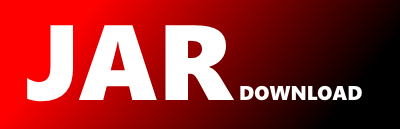
com.pulumi.gcp.applicationintegration.kotlin.inputs.AuthConfigDecryptedCredentialArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.applicationintegration.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property authToken Auth token credential.
* Structure is documented below.
* @property credentialType Credential type associated with auth configs.
* @property jwt JWT credential.
* Structure is documented below.
* @property oauth2AuthorizationCode OAuth2 authorization code credential.
* Structure is documented below.
* @property oauth2ClientCredentials OAuth2 client credentials.
* Structure is documented below.
* @property oidcToken Google OIDC ID Token.
* Structure is documented below.
* @property serviceAccountCredentials Service account credential.
* Structure is documented below.
* @property usernameAndPassword Username and password credential.
* Structure is documented below.
*/
public data class AuthConfigDecryptedCredentialArgs(
public val authToken: Output? = null,
public val credentialType: Output,
public val jwt: Output? = null,
public val oauth2AuthorizationCode: Output? = null,
public val oauth2ClientCredentials: Output? = null,
public val oidcToken: Output? = null,
public val serviceAccountCredentials: Output? = null,
public val usernameAndPassword: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialArgs =
com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialArgs.builder()
.authToken(authToken?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.credentialType(credentialType.applyValue({ args0 -> args0 }))
.jwt(jwt?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.oauth2AuthorizationCode(
oauth2AuthorizationCode?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.oauth2ClientCredentials(
oauth2ClientCredentials?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.oidcToken(oidcToken?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.serviceAccountCredentials(
serviceAccountCredentials?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.usernameAndPassword(
usernameAndPassword?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [AuthConfigDecryptedCredentialArgs].
*/
@PulumiTagMarker
public class AuthConfigDecryptedCredentialArgsBuilder internal constructor() {
private var authToken: Output? = null
private var credentialType: Output? = null
private var jwt: Output? = null
private var oauth2AuthorizationCode:
Output? = null
private var oauth2ClientCredentials:
Output? = null
private var oidcToken: Output? = null
private var serviceAccountCredentials:
Output? = null
private var usernameAndPassword: Output? =
null
/**
* @param value Auth token credential.
* Structure is documented below.
*/
@JvmName("qcwqjkrtqddkasix")
public suspend fun authToken(`value`: Output) {
this.authToken = value
}
/**
* @param value Credential type associated with auth configs.
*/
@JvmName("ybxxddiqswvhpgic")
public suspend fun credentialType(`value`: Output) {
this.credentialType = value
}
/**
* @param value JWT credential.
* Structure is documented below.
*/
@JvmName("tvwdjdgkrgmacagv")
public suspend fun jwt(`value`: Output) {
this.jwt = value
}
/**
* @param value OAuth2 authorization code credential.
* Structure is documented below.
*/
@JvmName("ynrcfrshcqyhcyik")
public suspend fun oauth2AuthorizationCode(`value`: Output) {
this.oauth2AuthorizationCode = value
}
/**
* @param value OAuth2 client credentials.
* Structure is documented below.
*/
@JvmName("xumjlvypoqorrhia")
public suspend fun oauth2ClientCredentials(`value`: Output) {
this.oauth2ClientCredentials = value
}
/**
* @param value Google OIDC ID Token.
* Structure is documented below.
*/
@JvmName("tqttiaxvnliywmih")
public suspend fun oidcToken(`value`: Output) {
this.oidcToken = value
}
/**
* @param value Service account credential.
* Structure is documented below.
*/
@JvmName("hpqpnqvfrejbdicv")
public suspend fun serviceAccountCredentials(`value`: Output) {
this.serviceAccountCredentials = value
}
/**
* @param value Username and password credential.
* Structure is documented below.
*/
@JvmName("cfuhjfiwbeojsgwq")
public suspend fun usernameAndPassword(`value`: Output) {
this.usernameAndPassword = value
}
/**
* @param value Auth token credential.
* Structure is documented below.
*/
@JvmName("lpxlxrpjuvlaiknj")
public suspend fun authToken(`value`: AuthConfigDecryptedCredentialAuthTokenArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authToken = mapped
}
/**
* @param argument Auth token credential.
* Structure is documented below.
*/
@JvmName("ljrwfskiqqmwcagb")
public suspend fun authToken(argument: suspend AuthConfigDecryptedCredentialAuthTokenArgsBuilder.() -> Unit) {
val toBeMapped = AuthConfigDecryptedCredentialAuthTokenArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.authToken = mapped
}
/**
* @param value Credential type associated with auth configs.
*/
@JvmName("xalduijgvgvdeidd")
public suspend fun credentialType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.credentialType = mapped
}
/**
* @param value JWT credential.
* Structure is documented below.
*/
@JvmName("jhfphrtsiykrdyxy")
public suspend fun jwt(`value`: AuthConfigDecryptedCredentialJwtArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jwt = mapped
}
/**
* @param argument JWT credential.
* Structure is documented below.
*/
@JvmName("tvbvrpgggrvbrggr")
public suspend fun jwt(argument: suspend AuthConfigDecryptedCredentialJwtArgsBuilder.() -> Unit) {
val toBeMapped = AuthConfigDecryptedCredentialJwtArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.jwt = mapped
}
/**
* @param value OAuth2 authorization code credential.
* Structure is documented below.
*/
@JvmName("nveffbmrygxtudrt")
public suspend fun oauth2AuthorizationCode(`value`: AuthConfigDecryptedCredentialOauth2AuthorizationCodeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.oauth2AuthorizationCode = mapped
}
/**
* @param argument OAuth2 authorization code credential.
* Structure is documented below.
*/
@JvmName("pijpkseluerdolkv")
public suspend fun oauth2AuthorizationCode(argument: suspend AuthConfigDecryptedCredentialOauth2AuthorizationCodeArgsBuilder.() -> Unit) {
val toBeMapped = AuthConfigDecryptedCredentialOauth2AuthorizationCodeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.oauth2AuthorizationCode = mapped
}
/**
* @param value OAuth2 client credentials.
* Structure is documented below.
*/
@JvmName("vrhvmfdkijiapgsb")
public suspend fun oauth2ClientCredentials(`value`: AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.oauth2ClientCredentials = mapped
}
/**
* @param argument OAuth2 client credentials.
* Structure is documented below.
*/
@JvmName("edmqhjrpejfyvgas")
public suspend fun oauth2ClientCredentials(argument: suspend AuthConfigDecryptedCredentialOauth2ClientCredentialsArgsBuilder.() -> Unit) {
val toBeMapped = AuthConfigDecryptedCredentialOauth2ClientCredentialsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.oauth2ClientCredentials = mapped
}
/**
* @param value Google OIDC ID Token.
* Structure is documented below.
*/
@JvmName("mrexcnhqdqlxsmdv")
public suspend fun oidcToken(`value`: AuthConfigDecryptedCredentialOidcTokenArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.oidcToken = mapped
}
/**
* @param argument Google OIDC ID Token.
* Structure is documented below.
*/
@JvmName("yjiwucmnmrtnlrkx")
public suspend fun oidcToken(argument: suspend AuthConfigDecryptedCredentialOidcTokenArgsBuilder.() -> Unit) {
val toBeMapped = AuthConfigDecryptedCredentialOidcTokenArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.oidcToken = mapped
}
/**
* @param value Service account credential.
* Structure is documented below.
*/
@JvmName("skahareajhwyregx")
public suspend fun serviceAccountCredentials(`value`: AuthConfigDecryptedCredentialServiceAccountCredentialsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceAccountCredentials = mapped
}
/**
* @param argument Service account credential.
* Structure is documented below.
*/
@JvmName("jodvgffnjilcqscs")
public suspend fun serviceAccountCredentials(argument: suspend AuthConfigDecryptedCredentialServiceAccountCredentialsArgsBuilder.() -> Unit) {
val toBeMapped =
AuthConfigDecryptedCredentialServiceAccountCredentialsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.serviceAccountCredentials = mapped
}
/**
* @param value Username and password credential.
* Structure is documented below.
*/
@JvmName("tmnwdfojxwwgtofq")
public suspend fun usernameAndPassword(`value`: AuthConfigDecryptedCredentialUsernameAndPasswordArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameAndPassword = mapped
}
/**
* @param argument Username and password credential.
* Structure is documented below.
*/
@JvmName("rkrynviubcxhqlau")
public suspend fun usernameAndPassword(argument: suspend AuthConfigDecryptedCredentialUsernameAndPasswordArgsBuilder.() -> Unit) {
val toBeMapped = AuthConfigDecryptedCredentialUsernameAndPasswordArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.usernameAndPassword = mapped
}
internal fun build(): AuthConfigDecryptedCredentialArgs = AuthConfigDecryptedCredentialArgs(
authToken = authToken,
credentialType = credentialType ?: throw PulumiNullFieldException("credentialType"),
jwt = jwt,
oauth2AuthorizationCode = oauth2AuthorizationCode,
oauth2ClientCredentials = oauth2ClientCredentials,
oidcToken = oidcToken,
serviceAccountCredentials = serviceAccountCredentials,
usernameAndPassword = usernameAndPassword,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy