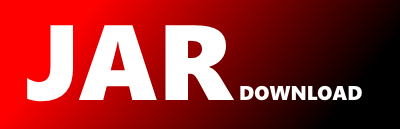
com.pulumi.gcp.applicationintegration.kotlin.inputs.AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.applicationintegration.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property clientId The client's ID.
* @property clientSecret The client's secret.
* @property requestType Represent how to pass parameters to fetch access token Possible values: ["REQUEST_TYPE_UNSPECIFIED", "REQUEST_BODY", "QUERY_PARAMETERS", "ENCODED_HEADER"]
* @property scope A space-delimited list of requested scope permissions.
* @property tokenEndpoint The token endpoint is used by the client to obtain an access token by presenting its authorization grant or refresh token.
* @property tokenParams Token parameters for the auth request.
*/
public data class AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs(
public val clientId: Output? = null,
public val clientSecret: Output? = null,
public val requestType: Output? = null,
public val scope: Output? = null,
public val tokenEndpoint: Output? = null,
public val tokenParams: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs =
com.pulumi.gcp.applicationintegration.inputs.AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs.builder()
.clientId(clientId?.applyValue({ args0 -> args0 }))
.clientSecret(clientSecret?.applyValue({ args0 -> args0 }))
.requestType(requestType?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0 }))
.tokenEndpoint(tokenEndpoint?.applyValue({ args0 -> args0 }))
.tokenParams(tokenParams?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs].
*/
@PulumiTagMarker
public class AuthConfigDecryptedCredentialOauth2ClientCredentialsArgsBuilder internal constructor() {
private var clientId: Output? = null
private var clientSecret: Output? = null
private var requestType: Output? = null
private var scope: Output? = null
private var tokenEndpoint: Output? = null
private var tokenParams:
Output? = null
/**
* @param value The client's ID.
*/
@JvmName("doxurgqxprhpcrqx")
public suspend fun clientId(`value`: Output) {
this.clientId = value
}
/**
* @param value The client's secret.
*/
@JvmName("hngfqfhvlacgdpwn")
public suspend fun clientSecret(`value`: Output) {
this.clientSecret = value
}
/**
* @param value Represent how to pass parameters to fetch access token Possible values: ["REQUEST_TYPE_UNSPECIFIED", "REQUEST_BODY", "QUERY_PARAMETERS", "ENCODED_HEADER"]
*/
@JvmName("wqokvmtlcblcfkdk")
public suspend fun requestType(`value`: Output) {
this.requestType = value
}
/**
* @param value A space-delimited list of requested scope permissions.
*/
@JvmName("tspkkjeaphrragbp")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value The token endpoint is used by the client to obtain an access token by presenting its authorization grant or refresh token.
*/
@JvmName("tvbongybgdyaxwdy")
public suspend fun tokenEndpoint(`value`: Output) {
this.tokenEndpoint = value
}
/**
* @param value Token parameters for the auth request.
*/
@JvmName("jbfmwbodfatlchmi")
public suspend fun tokenParams(`value`: Output) {
this.tokenParams = value
}
/**
* @param value The client's ID.
*/
@JvmName("ouhwbtvorvsmenjf")
public suspend fun clientId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientId = mapped
}
/**
* @param value The client's secret.
*/
@JvmName("wowcpbcaiaqfunvj")
public suspend fun clientSecret(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientSecret = mapped
}
/**
* @param value Represent how to pass parameters to fetch access token Possible values: ["REQUEST_TYPE_UNSPECIFIED", "REQUEST_BODY", "QUERY_PARAMETERS", "ENCODED_HEADER"]
*/
@JvmName("opnxlhwqvpojifnr")
public suspend fun requestType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestType = mapped
}
/**
* @param value A space-delimited list of requested scope permissions.
*/
@JvmName("cttmlhntjwjokoof")
public suspend fun scope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scope = mapped
}
/**
* @param value The token endpoint is used by the client to obtain an access token by presenting its authorization grant or refresh token.
*/
@JvmName("odtcijctldvvanpf")
public suspend fun tokenEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenEndpoint = mapped
}
/**
* @param value Token parameters for the auth request.
*/
@JvmName("ijsxnpuydeawkgli")
public suspend fun tokenParams(`value`: AuthConfigDecryptedCredentialOauth2ClientCredentialsTokenParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenParams = mapped
}
/**
* @param argument Token parameters for the auth request.
*/
@JvmName("bbpaalytbredpvqw")
public suspend fun tokenParams(argument: suspend AuthConfigDecryptedCredentialOauth2ClientCredentialsTokenParamsArgsBuilder.() -> Unit) {
val toBeMapped =
AuthConfigDecryptedCredentialOauth2ClientCredentialsTokenParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.tokenParams = mapped
}
internal fun build(): AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs =
AuthConfigDecryptedCredentialOauth2ClientCredentialsArgs(
clientId = clientId,
clientSecret = clientSecret,
requestType = requestType,
scope = scope,
tokenEndpoint = tokenEndpoint,
tokenParams = tokenParams,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy