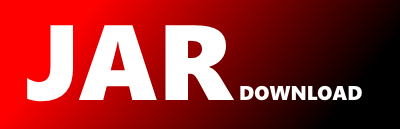
com.pulumi.gcp.applicationintegration.kotlin.inputs.ClientCloudKmsConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.applicationintegration.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.applicationintegration.inputs.ClientCloudKmsConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property key A Cloud KMS key is a named object containing one or more key versions, along
* with metadata for the key. A key exists on exactly one key ring tied to a
* specific location.
* @property keyVersion Each version of a key contains key material used for encryption or signing.
* A key's version is represented by an integer, starting at 1. To decrypt data
* or verify a signature, you must use the same key version that was used to
* encrypt or sign the data.
* @property kmsLocation Location name of the key ring, e.g. "us-west1".
* @property kmsProjectId The Google Cloud project id of the project where the kms key stored. If empty,
* the kms key is stored at the same project as customer's project and ecrypted
* with CMEK, otherwise, the kms key is stored in the tenant project and
* encrypted with GMEK.
* @property kmsRing A key ring organizes keys in a specific Google Cloud location and allows you to
* manage access control on groups of keys. A key ring's name does not need to be
* unique across a Google Cloud project, but must be unique within a given location.
*/
public data class ClientCloudKmsConfigArgs(
public val key: Output,
public val keyVersion: Output? = null,
public val kmsLocation: Output,
public val kmsProjectId: Output? = null,
public val kmsRing: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.applicationintegration.inputs.ClientCloudKmsConfigArgs =
com.pulumi.gcp.applicationintegration.inputs.ClientCloudKmsConfigArgs.builder()
.key(key.applyValue({ args0 -> args0 }))
.keyVersion(keyVersion?.applyValue({ args0 -> args0 }))
.kmsLocation(kmsLocation.applyValue({ args0 -> args0 }))
.kmsProjectId(kmsProjectId?.applyValue({ args0 -> args0 }))
.kmsRing(kmsRing.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClientCloudKmsConfigArgs].
*/
@PulumiTagMarker
public class ClientCloudKmsConfigArgsBuilder internal constructor() {
private var key: Output? = null
private var keyVersion: Output? = null
private var kmsLocation: Output? = null
private var kmsProjectId: Output? = null
private var kmsRing: Output? = null
/**
* @param value A Cloud KMS key is a named object containing one or more key versions, along
* with metadata for the key. A key exists on exactly one key ring tied to a
* specific location.
*/
@JvmName("qteqdidmuodygonf")
public suspend fun key(`value`: Output) {
this.key = value
}
/**
* @param value Each version of a key contains key material used for encryption or signing.
* A key's version is represented by an integer, starting at 1. To decrypt data
* or verify a signature, you must use the same key version that was used to
* encrypt or sign the data.
*/
@JvmName("dhpqwttkbqnaebwq")
public suspend fun keyVersion(`value`: Output) {
this.keyVersion = value
}
/**
* @param value Location name of the key ring, e.g. "us-west1".
*/
@JvmName("qfuxjpswkftxlqmp")
public suspend fun kmsLocation(`value`: Output) {
this.kmsLocation = value
}
/**
* @param value The Google Cloud project id of the project where the kms key stored. If empty,
* the kms key is stored at the same project as customer's project and ecrypted
* with CMEK, otherwise, the kms key is stored in the tenant project and
* encrypted with GMEK.
*/
@JvmName("lmbqftaygqctomfo")
public suspend fun kmsProjectId(`value`: Output) {
this.kmsProjectId = value
}
/**
* @param value A key ring organizes keys in a specific Google Cloud location and allows you to
* manage access control on groups of keys. A key ring's name does not need to be
* unique across a Google Cloud project, but must be unique within a given location.
*/
@JvmName("wdlgwcgqbfysdqjl")
public suspend fun kmsRing(`value`: Output) {
this.kmsRing = value
}
/**
* @param value A Cloud KMS key is a named object containing one or more key versions, along
* with metadata for the key. A key exists on exactly one key ring tied to a
* specific location.
*/
@JvmName("vunvcwivdyjfqvra")
public suspend fun key(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.key = mapped
}
/**
* @param value Each version of a key contains key material used for encryption or signing.
* A key's version is represented by an integer, starting at 1. To decrypt data
* or verify a signature, you must use the same key version that was used to
* encrypt or sign the data.
*/
@JvmName("kkjfubnfsqgoposp")
public suspend fun keyVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyVersion = mapped
}
/**
* @param value Location name of the key ring, e.g. "us-west1".
*/
@JvmName("bltinwdniecykjhk")
public suspend fun kmsLocation(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kmsLocation = mapped
}
/**
* @param value The Google Cloud project id of the project where the kms key stored. If empty,
* the kms key is stored at the same project as customer's project and ecrypted
* with CMEK, otherwise, the kms key is stored in the tenant project and
* encrypted with GMEK.
*/
@JvmName("rlbfltsoikrvkmuf")
public suspend fun kmsProjectId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsProjectId = mapped
}
/**
* @param value A key ring organizes keys in a specific Google Cloud location and allows you to
* manage access control on groups of keys. A key ring's name does not need to be
* unique across a Google Cloud project, but must be unique within a given location.
*/
@JvmName("mesdifnrxmcrodau")
public suspend fun kmsRing(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kmsRing = mapped
}
internal fun build(): ClientCloudKmsConfigArgs = ClientCloudKmsConfigArgs(
key = key ?: throw PulumiNullFieldException("key"),
keyVersion = keyVersion,
kmsLocation = kmsLocation ?: throw PulumiNullFieldException("kmsLocation"),
kmsProjectId = kmsProjectId,
kmsRing = kmsRing ?: throw PulumiNullFieldException("kmsRing"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy