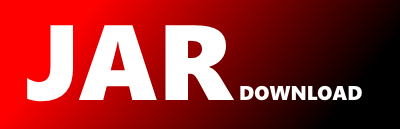
com.pulumi.gcp.artifactregistry.kotlin.RepositoryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.artifactregistry.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.artifactregistry.RepositoryArgs.builder
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryCleanupPolicyArgs
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryCleanupPolicyArgsBuilder
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryDockerConfigArgs
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryDockerConfigArgsBuilder
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryMavenConfigArgs
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryMavenConfigArgsBuilder
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryRemoteRepositoryConfigArgs
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryRemoteRepositoryConfigArgsBuilder
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryVirtualRepositoryConfigArgs
import com.pulumi.gcp.artifactregistry.kotlin.inputs.RepositoryVirtualRepositoryConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A repository for storing artifacts
* To get more information about Repository, see:
* * [API documentation](https://cloud.google.com/artifact-registry/docs/reference/rest/v1/projects.locations.repositories)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/artifact-registry/docs/overview)
* ## Example Usage
* ### Artifact Registry Repository Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "my-repository",
* description: "example docker repository",
* format: "DOCKER",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="my-repository",
* description="example docker repository",
* format="DOCKER")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository",
* Description = "example docker repository",
* Format = "DOCKER",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository"),
* Description: pulumi.String("example docker repository"),
* Format: pulumi.String("DOCKER"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository")
* .description("example docker repository")
* .format("DOCKER")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository
* description: example docker repository
* format: DOCKER
* ```
*
* ### Artifact Registry Repository Docker
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "my-repository",
* description: "example docker repository",
* format: "DOCKER",
* dockerConfig: {
* immutableTags: true,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="my-repository",
* description="example docker repository",
* format="DOCKER",
* docker_config=gcp.artifactregistry.RepositoryDockerConfigArgs(
* immutable_tags=True,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository",
* Description = "example docker repository",
* Format = "DOCKER",
* DockerConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryDockerConfigArgs
* {
* ImmutableTags = true,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository"),
* Description: pulumi.String("example docker repository"),
* Format: pulumi.String("DOCKER"),
* DockerConfig: &artifactregistry.RepositoryDockerConfigArgs{
* ImmutableTags: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryDockerConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository")
* .description("example docker repository")
* .format("DOCKER")
* .dockerConfig(RepositoryDockerConfigArgs.builder()
* .immutableTags(true)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository
* description: example docker repository
* format: DOCKER
* dockerConfig:
* immutableTags: true
* ```
*
* ### Artifact Registry Repository Cmek
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "my-repository",
* description: "example docker repository with cmek",
* format: "DOCKER",
* kmsKeyName: "kms-key",
* });
* const project = gcp.organizations.getProject({});
* const cryptoKey = new gcp.kms.CryptoKeyIAMMember("crypto_key", {
* cryptoKeyId: "kms-key",
* role: "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="my-repository",
* description="example docker repository with cmek",
* format="DOCKER",
* kms_key_name="kms-key")
* project = gcp.organizations.get_project()
* crypto_key = gcp.kms.CryptoKeyIAMMember("crypto_key",
* crypto_key_id="kms-key",
* role="roles/cloudkms.cryptoKeyEncrypterDecrypter",
* member=f"serviceAccount:service-{project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository",
* Description = "example docker repository with cmek",
* Format = "DOCKER",
* KmsKeyName = "kms-key",
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var cryptoKey = new Gcp.Kms.CryptoKeyIAMMember("crypto_key", new()
* {
* CryptoKeyId = "kms-key",
* Role = "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-artifactregistry.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository"),
* Description: pulumi.String("example docker repository with cmek"),
* Format: pulumi.String("DOCKER"),
* KmsKeyName: pulumi.String("kms-key"),
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = kms.NewCryptoKeyIAMMember(ctx, "crypto_key", &kms.CryptoKeyIAMMemberArgs{
* CryptoKeyId: pulumi.String("kms-key"),
* Role: pulumi.String("roles/cloudkms.cryptoKeyEncrypterDecrypter"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.kms.CryptoKeyIAMMember;
* import com.pulumi.gcp.kms.CryptoKeyIAMMemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository")
* .description("example docker repository with cmek")
* .format("DOCKER")
* .kmsKeyName("kms-key")
* .build());
* final var project = OrganizationsFunctions.getProject();
* var cryptoKey = new CryptoKeyIAMMember("cryptoKey", CryptoKeyIAMMemberArgs.builder()
* .cryptoKeyId("kms-key")
* .role("roles/cloudkms.cryptoKeyEncrypterDecrypter")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository
* description: example docker repository with cmek
* format: DOCKER
* kmsKeyName: kms-key
* cryptoKey:
* type: gcp:kms:CryptoKeyIAMMember
* name: crypto_key
* properties:
* cryptoKeyId: kms-key
* role: roles/cloudkms.cryptoKeyEncrypterDecrypter
* member: serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Artifact Registry Repository Virtual
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo_upstream_1 = new gcp.artifactregistry.Repository("my-repo-upstream-1", {
* location: "us-central1",
* repositoryId: "my-repository-upstream-1",
* description: "example docker repository (upstream source) 1",
* format: "DOCKER",
* });
* const my_repo_upstream_2 = new gcp.artifactregistry.Repository("my-repo-upstream-2", {
* location: "us-central1",
* repositoryId: "my-repository-upstream-2",
* description: "example docker repository (upstream source) 2",
* format: "DOCKER",
* });
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "my-repository",
* description: "example virtual docker repository",
* format: "DOCKER",
* mode: "VIRTUAL_REPOSITORY",
* virtualRepositoryConfig: {
* upstreamPolicies: [
* {
* id: "my-repository-upstream-1",
* repository: my_repo_upstream_1.id,
* priority: 20,
* },
* {
* id: "my-repository-upstream-2",
* repository: my_repo_upstream_2.id,
* priority: 10,
* },
* ],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo_upstream_1 = gcp.artifactregistry.Repository("my-repo-upstream-1",
* location="us-central1",
* repository_id="my-repository-upstream-1",
* description="example docker repository (upstream source) 1",
* format="DOCKER")
* my_repo_upstream_2 = gcp.artifactregistry.Repository("my-repo-upstream-2",
* location="us-central1",
* repository_id="my-repository-upstream-2",
* description="example docker repository (upstream source) 2",
* format="DOCKER")
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="my-repository",
* description="example virtual docker repository",
* format="DOCKER",
* mode="VIRTUAL_REPOSITORY",
* virtual_repository_config=gcp.artifactregistry.RepositoryVirtualRepositoryConfigArgs(
* upstream_policies=[
* gcp.artifactregistry.RepositoryVirtualRepositoryConfigUpstreamPolicyArgs(
* id="my-repository-upstream-1",
* repository=my_repo_upstream_1.id,
* priority=20,
* ),
* gcp.artifactregistry.RepositoryVirtualRepositoryConfigUpstreamPolicyArgs(
* id="my-repository-upstream-2",
* repository=my_repo_upstream_2.id,
* priority=10,
* ),
* ],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo_upstream_1 = new Gcp.ArtifactRegistry.Repository("my-repo-upstream-1", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository-upstream-1",
* Description = "example docker repository (upstream source) 1",
* Format = "DOCKER",
* });
* var my_repo_upstream_2 = new Gcp.ArtifactRegistry.Repository("my-repo-upstream-2", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository-upstream-2",
* Description = "example docker repository (upstream source) 2",
* Format = "DOCKER",
* });
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository",
* Description = "example virtual docker repository",
* Format = "DOCKER",
* Mode = "VIRTUAL_REPOSITORY",
* VirtualRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryVirtualRepositoryConfigArgs
* {
* UpstreamPolicies = new[]
* {
* new Gcp.ArtifactRegistry.Inputs.RepositoryVirtualRepositoryConfigUpstreamPolicyArgs
* {
* Id = "my-repository-upstream-1",
* Repository = my_repo_upstream_1.Id,
* Priority = 20,
* },
* new Gcp.ArtifactRegistry.Inputs.RepositoryVirtualRepositoryConfigUpstreamPolicyArgs
* {
* Id = "my-repository-upstream-2",
* Repository = my_repo_upstream_2.Id,
* Priority = 10,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo-upstream-1", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository-upstream-1"),
* Description: pulumi.String("example docker repository (upstream source) 1"),
* Format: pulumi.String("DOCKER"),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo-upstream-2", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository-upstream-2"),
* Description: pulumi.String("example docker repository (upstream source) 2"),
* Format: pulumi.String("DOCKER"),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository"),
* Description: pulumi.String("example virtual docker repository"),
* Format: pulumi.String("DOCKER"),
* Mode: pulumi.String("VIRTUAL_REPOSITORY"),
* VirtualRepositoryConfig: &artifactregistry.RepositoryVirtualRepositoryConfigArgs{
* UpstreamPolicies: artifactregistry.RepositoryVirtualRepositoryConfigUpstreamPolicyArray{
* &artifactregistry.RepositoryVirtualRepositoryConfigUpstreamPolicyArgs{
* Id: pulumi.String("my-repository-upstream-1"),
* Repository: my_repo_upstream_1.ID(),
* Priority: pulumi.Int(20),
* },
* &artifactregistry.RepositoryVirtualRepositoryConfigUpstreamPolicyArgs{
* Id: pulumi.String("my-repository-upstream-2"),
* Repository: my_repo_upstream_2.ID(),
* Priority: pulumi.Int(10),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryVirtualRepositoryConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo_upstream_1 = new Repository("my-repo-upstream-1", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository-upstream-1")
* .description("example docker repository (upstream source) 1")
* .format("DOCKER")
* .build());
* var my_repo_upstream_2 = new Repository("my-repo-upstream-2", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository-upstream-2")
* .description("example docker repository (upstream source) 2")
* .format("DOCKER")
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository")
* .description("example virtual docker repository")
* .format("DOCKER")
* .mode("VIRTUAL_REPOSITORY")
* .virtualRepositoryConfig(RepositoryVirtualRepositoryConfigArgs.builder()
* .upstreamPolicies(
* RepositoryVirtualRepositoryConfigUpstreamPolicyArgs.builder()
* .id("my-repository-upstream-1")
* .repository(my_repo_upstream_1.id())
* .priority(20)
* .build(),
* RepositoryVirtualRepositoryConfigUpstreamPolicyArgs.builder()
* .id("my-repository-upstream-2")
* .repository(my_repo_upstream_2.id())
* .priority(10)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo-upstream-1:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository-upstream-1
* description: example docker repository (upstream source) 1
* format: DOCKER
* my-repo-upstream-2:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository-upstream-2
* description: example docker repository (upstream source) 2
* format: DOCKER
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository
* description: example virtual docker repository
* format: DOCKER
* mode: VIRTUAL_REPOSITORY
* virtualRepositoryConfig:
* upstreamPolicies:
* - id: my-repository-upstream-1
* repository: ${["my-repo-upstream-1"].id}
* priority: 20
* - id: my-repository-upstream-2
* repository: ${["my-repo-upstream-2"].id}
* priority: 10
* ```
*
* ### Artifact Registry Repository Remote
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "my-repository",
* description: "example remote docker repository",
* format: "DOCKER",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "docker hub",
* dockerRepository: {
* publicRepository: "DOCKER_HUB",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="my-repository",
* description="example remote docker repository",
* format="DOCKER",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="docker hub",
* docker_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryArgs(
* public_repository="DOCKER_HUB",
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository",
* Description = "example remote docker repository",
* Format = "DOCKER",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "docker hub",
* DockerRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigDockerRepositoryArgs
* {
* PublicRepository = "DOCKER_HUB",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository"),
* Description: pulumi.String("example remote docker repository"),
* Format: pulumi.String("DOCKER"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("docker hub"),
* DockerRepository: &artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryArgs{
* PublicRepository: pulumi.String("DOCKER_HUB"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigDockerRepositoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository")
* .description("example remote docker repository")
* .format("DOCKER")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("docker hub")
* .dockerRepository(RepositoryRemoteRepositoryConfigDockerRepositoryArgs.builder()
* .publicRepository("DOCKER_HUB")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository
* description: example remote docker repository
* format: DOCKER
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: docker hub
* dockerRepository:
* publicRepository: DOCKER_HUB
* ```
*
* ### Artifact Registry Repository Remote Apt
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "debian-buster",
* description: "example remote apt repository",
* format: "APT",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "Debian buster remote repository",
* aptRepository: {
* publicRepository: {
* repositoryBase: "DEBIAN",
* repositoryPath: "debian/dists/buster",
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="debian-buster",
* description="example remote apt repository",
* format="APT",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="Debian buster remote repository",
* apt_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigAptRepositoryArgs(
* public_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigAptRepositoryPublicRepositoryArgs(
* repository_base="DEBIAN",
* repository_path="debian/dists/buster",
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "debian-buster",
* Description = "example remote apt repository",
* Format = "APT",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "Debian buster remote repository",
* AptRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigAptRepositoryArgs
* {
* PublicRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigAptRepositoryPublicRepositoryArgs
* {
* RepositoryBase = "DEBIAN",
* RepositoryPath = "debian/dists/buster",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("debian-buster"),
* Description: pulumi.String("example remote apt repository"),
* Format: pulumi.String("APT"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("Debian buster remote repository"),
* AptRepository: &artifactregistry.RepositoryRemoteRepositoryConfigAptRepositoryArgs{
* PublicRepository: &artifactregistry.RepositoryRemoteRepositoryConfigAptRepositoryPublicRepositoryArgs{
* RepositoryBase: pulumi.String("DEBIAN"),
* RepositoryPath: pulumi.String("debian/dists/buster"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigAptRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigAptRepositoryPublicRepositoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("debian-buster")
* .description("example remote apt repository")
* .format("APT")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("Debian buster remote repository")
* .aptRepository(RepositoryRemoteRepositoryConfigAptRepositoryArgs.builder()
* .publicRepository(RepositoryRemoteRepositoryConfigAptRepositoryPublicRepositoryArgs.builder()
* .repositoryBase("DEBIAN")
* .repositoryPath("debian/dists/buster")
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: debian-buster
* description: example remote apt repository
* format: APT
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: Debian buster remote repository
* aptRepository:
* publicRepository:
* repositoryBase: DEBIAN
* repositoryPath: debian/dists/buster
* ```
*
* ### Artifact Registry Repository Remote Yum
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "centos-8",
* description: "example remote yum repository",
* format: "YUM",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "Centos 8 remote repository",
* yumRepository: {
* publicRepository: {
* repositoryBase: "CENTOS",
* repositoryPath: "centos/8-stream/BaseOS/x86_64/os",
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="centos-8",
* description="example remote yum repository",
* format="YUM",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="Centos 8 remote repository",
* yum_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigYumRepositoryArgs(
* public_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigYumRepositoryPublicRepositoryArgs(
* repository_base="CENTOS",
* repository_path="centos/8-stream/BaseOS/x86_64/os",
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "centos-8",
* Description = "example remote yum repository",
* Format = "YUM",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "Centos 8 remote repository",
* YumRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigYumRepositoryArgs
* {
* PublicRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigYumRepositoryPublicRepositoryArgs
* {
* RepositoryBase = "CENTOS",
* RepositoryPath = "centos/8-stream/BaseOS/x86_64/os",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("centos-8"),
* Description: pulumi.String("example remote yum repository"),
* Format: pulumi.String("YUM"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("Centos 8 remote repository"),
* YumRepository: &artifactregistry.RepositoryRemoteRepositoryConfigYumRepositoryArgs{
* PublicRepository: &artifactregistry.RepositoryRemoteRepositoryConfigYumRepositoryPublicRepositoryArgs{
* RepositoryBase: pulumi.String("CENTOS"),
* RepositoryPath: pulumi.String("centos/8-stream/BaseOS/x86_64/os"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigYumRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigYumRepositoryPublicRepositoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("centos-8")
* .description("example remote yum repository")
* .format("YUM")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("Centos 8 remote repository")
* .yumRepository(RepositoryRemoteRepositoryConfigYumRepositoryArgs.builder()
* .publicRepository(RepositoryRemoteRepositoryConfigYumRepositoryPublicRepositoryArgs.builder()
* .repositoryBase("CENTOS")
* .repositoryPath("centos/8-stream/BaseOS/x86_64/os")
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: centos-8
* description: example remote yum repository
* format: YUM
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: Centos 8 remote repository
* yumRepository:
* publicRepository:
* repositoryBase: CENTOS
* repositoryPath: centos/8-stream/BaseOS/x86_64/os
* ```
*
* ### Artifact Registry Repository Cleanup
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "my-repository",
* description: "example docker repository with cleanup policies",
* format: "DOCKER",
* cleanupPolicyDryRun: false,
* cleanupPolicies: [
* {
* id: "delete-prerelease",
* action: "DELETE",
* condition: {
* tagState: "TAGGED",
* tagPrefixes: [
* "alpha",
* "v0",
* ],
* olderThan: "2592000s",
* },
* },
* {
* id: "keep-tagged-release",
* action: "KEEP",
* condition: {
* tagState: "TAGGED",
* tagPrefixes: ["release"],
* packageNamePrefixes: [
* "webapp",
* "mobile",
* ],
* },
* },
* {
* id: "keep-minimum-versions",
* action: "KEEP",
* mostRecentVersions: {
* packageNamePrefixes: [
* "webapp",
* "mobile",
* "sandbox",
* ],
* keepCount: 5,
* },
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="my-repository",
* description="example docker repository with cleanup policies",
* format="DOCKER",
* cleanup_policy_dry_run=False,
* cleanup_policies=[
* gcp.artifactregistry.RepositoryCleanupPolicyArgs(
* id="delete-prerelease",
* action="DELETE",
* condition=gcp.artifactregistry.RepositoryCleanupPolicyConditionArgs(
* tag_state="TAGGED",
* tag_prefixes=[
* "alpha",
* "v0",
* ],
* older_than="2592000s",
* ),
* ),
* gcp.artifactregistry.RepositoryCleanupPolicyArgs(
* id="keep-tagged-release",
* action="KEEP",
* condition=gcp.artifactregistry.RepositoryCleanupPolicyConditionArgs(
* tag_state="TAGGED",
* tag_prefixes=["release"],
* package_name_prefixes=[
* "webapp",
* "mobile",
* ],
* ),
* ),
* gcp.artifactregistry.RepositoryCleanupPolicyArgs(
* id="keep-minimum-versions",
* action="KEEP",
* most_recent_versions=gcp.artifactregistry.RepositoryCleanupPolicyMostRecentVersionsArgs(
* package_name_prefixes=[
* "webapp",
* "mobile",
* "sandbox",
* ],
* keep_count=5,
* ),
* ),
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "my-repository",
* Description = "example docker repository with cleanup policies",
* Format = "DOCKER",
* CleanupPolicyDryRun = false,
* CleanupPolicies = new[]
* {
* new Gcp.ArtifactRegistry.Inputs.RepositoryCleanupPolicyArgs
* {
* Id = "delete-prerelease",
* Action = "DELETE",
* Condition = new Gcp.ArtifactRegistry.Inputs.RepositoryCleanupPolicyConditionArgs
* {
* TagState = "TAGGED",
* TagPrefixes = new[]
* {
* "alpha",
* "v0",
* },
* OlderThan = "2592000s",
* },
* },
* new Gcp.ArtifactRegistry.Inputs.RepositoryCleanupPolicyArgs
* {
* Id = "keep-tagged-release",
* Action = "KEEP",
* Condition = new Gcp.ArtifactRegistry.Inputs.RepositoryCleanupPolicyConditionArgs
* {
* TagState = "TAGGED",
* TagPrefixes = new[]
* {
* "release",
* },
* PackageNamePrefixes = new[]
* {
* "webapp",
* "mobile",
* },
* },
* },
* new Gcp.ArtifactRegistry.Inputs.RepositoryCleanupPolicyArgs
* {
* Id = "keep-minimum-versions",
* Action = "KEEP",
* MostRecentVersions = new Gcp.ArtifactRegistry.Inputs.RepositoryCleanupPolicyMostRecentVersionsArgs
* {
* PackageNamePrefixes = new[]
* {
* "webapp",
* "mobile",
* "sandbox",
* },
* KeepCount = 5,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("my-repository"),
* Description: pulumi.String("example docker repository with cleanup policies"),
* Format: pulumi.String("DOCKER"),
* CleanupPolicyDryRun: pulumi.Bool(false),
* CleanupPolicies: artifactregistry.RepositoryCleanupPolicyArray{
* &artifactregistry.RepositoryCleanupPolicyArgs{
* Id: pulumi.String("delete-prerelease"),
* Action: pulumi.String("DELETE"),
* Condition: &artifactregistry.RepositoryCleanupPolicyConditionArgs{
* TagState: pulumi.String("TAGGED"),
* TagPrefixes: pulumi.StringArray{
* pulumi.String("alpha"),
* pulumi.String("v0"),
* },
* OlderThan: pulumi.String("2592000s"),
* },
* },
* &artifactregistry.RepositoryCleanupPolicyArgs{
* Id: pulumi.String("keep-tagged-release"),
* Action: pulumi.String("KEEP"),
* Condition: &artifactregistry.RepositoryCleanupPolicyConditionArgs{
* TagState: pulumi.String("TAGGED"),
* TagPrefixes: pulumi.StringArray{
* pulumi.String("release"),
* },
* PackageNamePrefixes: pulumi.StringArray{
* pulumi.String("webapp"),
* pulumi.String("mobile"),
* },
* },
* },
* &artifactregistry.RepositoryCleanupPolicyArgs{
* Id: pulumi.String("keep-minimum-versions"),
* Action: pulumi.String("KEEP"),
* MostRecentVersions: &artifactregistry.RepositoryCleanupPolicyMostRecentVersionsArgs{
* PackageNamePrefixes: pulumi.StringArray{
* pulumi.String("webapp"),
* pulumi.String("mobile"),
* pulumi.String("sandbox"),
* },
* KeepCount: pulumi.Int(5),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryCleanupPolicyArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryCleanupPolicyConditionArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryCleanupPolicyMostRecentVersionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("my-repository")
* .description("example docker repository with cleanup policies")
* .format("DOCKER")
* .cleanupPolicyDryRun(false)
* .cleanupPolicies(
* RepositoryCleanupPolicyArgs.builder()
* .id("delete-prerelease")
* .action("DELETE")
* .condition(RepositoryCleanupPolicyConditionArgs.builder()
* .tagState("TAGGED")
* .tagPrefixes(
* "alpha",
* "v0")
* .olderThan("2592000s")
* .build())
* .build(),
* RepositoryCleanupPolicyArgs.builder()
* .id("keep-tagged-release")
* .action("KEEP")
* .condition(RepositoryCleanupPolicyConditionArgs.builder()
* .tagState("TAGGED")
* .tagPrefixes("release")
* .packageNamePrefixes(
* "webapp",
* "mobile")
* .build())
* .build(),
* RepositoryCleanupPolicyArgs.builder()
* .id("keep-minimum-versions")
* .action("KEEP")
* .mostRecentVersions(RepositoryCleanupPolicyMostRecentVersionsArgs.builder()
* .packageNamePrefixes(
* "webapp",
* "mobile",
* "sandbox")
* .keepCount(5)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: my-repository
* description: example docker repository with cleanup policies
* format: DOCKER
* cleanupPolicyDryRun: false
* cleanupPolicies:
* - id: delete-prerelease
* action: DELETE
* condition:
* tagState: TAGGED
* tagPrefixes:
* - alpha
* - v0
* olderThan: 2592000s
* - id: keep-tagged-release
* action: KEEP
* condition:
* tagState: TAGGED
* tagPrefixes:
* - release
* packageNamePrefixes:
* - webapp
* - mobile
* - id: keep-minimum-versions
* action: KEEP
* mostRecentVersions:
* packageNamePrefixes:
* - webapp
* - mobile
* - sandbox
* keepCount: 5
* ```
*
* ### Artifact Registry Repository Remote Dockerhub Auth
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const example_remote_secret = new gcp.secretmanager.Secret("example-remote-secret", {
* secretId: "example-secret",
* replication: {
* auto: {},
* },
* });
* const example_remote_secretVersion = new gcp.secretmanager.SecretVersion("example-remote-secret_version", {
* secret: example_remote_secret.id,
* secretData: "remote-password",
* });
* const secret_access = new gcp.secretmanager.SecretIamMember("secret-access", {
* secretId: example_remote_secret.id,
* role: "roles/secretmanager.secretAccessor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com`),
* });
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "example-dockerhub-remote",
* description: "example remote dockerhub repository with credentials",
* format: "DOCKER",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "docker hub with custom credentials",
* disableUpstreamValidation: true,
* dockerRepository: {
* publicRepository: "DOCKER_HUB",
* },
* upstreamCredentials: {
* usernamePasswordCredentials: {
* username: "remote-username",
* passwordSecretVersion: example_remote_secretVersion.name,
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* example_remote_secret = gcp.secretmanager.Secret("example-remote-secret",
* secret_id="example-secret",
* replication=gcp.secretmanager.SecretReplicationArgs(
* auto=gcp.secretmanager.SecretReplicationAutoArgs(),
* ))
* example_remote_secret_version = gcp.secretmanager.SecretVersion("example-remote-secret_version",
* secret=example_remote_secret.id,
* secret_data="remote-password")
* secret_access = gcp.secretmanager.SecretIamMember("secret-access",
* secret_id=example_remote_secret.id,
* role="roles/secretmanager.secretAccessor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com")
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="example-dockerhub-remote",
* description="example remote dockerhub repository with credentials",
* format="DOCKER",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="docker hub with custom credentials",
* disable_upstream_validation=True,
* docker_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryArgs(
* public_repository="DOCKER_HUB",
* ),
* upstream_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs(
* username_password_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs(
* username="remote-username",
* password_secret_version=example_remote_secret_version.name,
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var example_remote_secret = new Gcp.SecretManager.Secret("example-remote-secret", new()
* {
* SecretId = "example-secret",
* Replication = new Gcp.SecretManager.Inputs.SecretReplicationArgs
* {
* Auto = null,
* },
* });
* var example_remote_secretVersion = new Gcp.SecretManager.SecretVersion("example-remote-secret_version", new()
* {
* Secret = example_remote_secret.Id,
* SecretData = "remote-password",
* });
* var secret_access = new Gcp.SecretManager.SecretIamMember("secret-access", new()
* {
* SecretId = example_remote_secret.Id,
* Role = "roles/secretmanager.secretAccessor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-artifactregistry.iam.gserviceaccount.com",
* });
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "example-dockerhub-remote",
* Description = "example remote dockerhub repository with credentials",
* Format = "DOCKER",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "docker hub with custom credentials",
* DisableUpstreamValidation = true,
* DockerRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigDockerRepositoryArgs
* {
* PublicRepository = "DOCKER_HUB",
* },
* UpstreamCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs
* {
* UsernamePasswordCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs
* {
* Username = "remote-username",
* PasswordSecretVersion = example_remote_secretVersion.Name,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/secretmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecret(ctx, "example-remote-secret", &secretmanager.SecretArgs{
* SecretId: pulumi.String("example-secret"),
* Replication: &secretmanager.SecretReplicationArgs{
* Auto: nil,
* },
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretVersion(ctx, "example-remote-secret_version", &secretmanager.SecretVersionArgs{
* Secret: example_remote_secret.ID(),
* SecretData: pulumi.String("remote-password"),
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretIamMember(ctx, "secret-access", &secretmanager.SecretIamMemberArgs{
* SecretId: example_remote_secret.ID(),
* Role: pulumi.String("roles/secretmanager.secretAccessor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("example-dockerhub-remote"),
* Description: pulumi.String("example remote dockerhub repository with credentials"),
* Format: pulumi.String("DOCKER"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("docker hub with custom credentials"),
* DisableUpstreamValidation: pulumi.Bool(true),
* DockerRepository: &artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryArgs{
* PublicRepository: pulumi.String("DOCKER_HUB"),
* },
* UpstreamCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs{
* UsernamePasswordCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs{
* Username: pulumi.String("remote-username"),
* PasswordSecretVersion: example_remote_secretVersion.Name,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.secretmanager.Secret;
* import com.pulumi.gcp.secretmanager.SecretArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationAutoArgs;
* import com.pulumi.gcp.secretmanager.SecretVersion;
* import com.pulumi.gcp.secretmanager.SecretVersionArgs;
* import com.pulumi.gcp.secretmanager.SecretIamMember;
* import com.pulumi.gcp.secretmanager.SecretIamMemberArgs;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigDockerRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var example_remote_secret = new Secret("example-remote-secret", SecretArgs.builder()
* .secretId("example-secret")
* .replication(SecretReplicationArgs.builder()
* .auto()
* .build())
* .build());
* var example_remote_secretVersion = new SecretVersion("example-remote-secretVersion", SecretVersionArgs.builder()
* .secret(example_remote_secret.id())
* .secretData("remote-password")
* .build());
* var secret_access = new SecretIamMember("secret-access", SecretIamMemberArgs.builder()
* .secretId(example_remote_secret.id())
* .role("roles/secretmanager.secretAccessor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("example-dockerhub-remote")
* .description("example remote dockerhub repository with credentials")
* .format("DOCKER")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("docker hub with custom credentials")
* .disableUpstreamValidation(true)
* .dockerRepository(RepositoryRemoteRepositoryConfigDockerRepositoryArgs.builder()
* .publicRepository("DOCKER_HUB")
* .build())
* .upstreamCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs.builder()
* .usernamePasswordCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs.builder()
* .username("remote-username")
* .passwordSecretVersion(example_remote_secretVersion.name())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example-remote-secret:
* type: gcp:secretmanager:Secret
* properties:
* secretId: example-secret
* replication:
* auto: {}
* example-remote-secretVersion:
* type: gcp:secretmanager:SecretVersion
* name: example-remote-secret_version
* properties:
* secret: ${["example-remote-secret"].id}
* secretData: remote-password
* secret-access:
* type: gcp:secretmanager:SecretIamMember
* properties:
* secretId: ${["example-remote-secret"].id}
* role: roles/secretmanager.secretAccessor
* member: serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: example-dockerhub-remote
* description: example remote dockerhub repository with credentials
* format: DOCKER
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: docker hub with custom credentials
* disableUpstreamValidation: true
* dockerRepository:
* publicRepository: DOCKER_HUB
* upstreamCredentials:
* usernamePasswordCredentials:
* username: remote-username
* passwordSecretVersion: ${["example-remote-secretVersion"].name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Artifact Registry Repository Remote Docker Custom With Auth
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const example_remote_secret = new gcp.secretmanager.Secret("example-remote-secret", {
* secretId: "example-secret",
* replication: {
* auto: {},
* },
* });
* const example_remote_secretVersion = new gcp.secretmanager.SecretVersion("example-remote-secret_version", {
* secret: example_remote_secret.id,
* secretData: "remote-password",
* });
* const secret_access = new gcp.secretmanager.SecretIamMember("secret-access", {
* secretId: example_remote_secret.id,
* role: "roles/secretmanager.secretAccessor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com`),
* });
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "example-docker-custom-remote",
* description: "example remote custom docker repository with credentials",
* format: "DOCKER",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "custom docker remote with credentials",
* disableUpstreamValidation: true,
* dockerRepository: {
* customRepository: {
* uri: "https://registry-1.docker.io",
* },
* },
* upstreamCredentials: {
* usernamePasswordCredentials: {
* username: "remote-username",
* passwordSecretVersion: example_remote_secretVersion.name,
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* example_remote_secret = gcp.secretmanager.Secret("example-remote-secret",
* secret_id="example-secret",
* replication=gcp.secretmanager.SecretReplicationArgs(
* auto=gcp.secretmanager.SecretReplicationAutoArgs(),
* ))
* example_remote_secret_version = gcp.secretmanager.SecretVersion("example-remote-secret_version",
* secret=example_remote_secret.id,
* secret_data="remote-password")
* secret_access = gcp.secretmanager.SecretIamMember("secret-access",
* secret_id=example_remote_secret.id,
* role="roles/secretmanager.secretAccessor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com")
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="example-docker-custom-remote",
* description="example remote custom docker repository with credentials",
* format="DOCKER",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="custom docker remote with credentials",
* disable_upstream_validation=True,
* docker_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryArgs(
* custom_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryCustomRepositoryArgs(
* uri="https://registry-1.docker.io",
* ),
* ),
* upstream_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs(
* username_password_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs(
* username="remote-username",
* password_secret_version=example_remote_secret_version.name,
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var example_remote_secret = new Gcp.SecretManager.Secret("example-remote-secret", new()
* {
* SecretId = "example-secret",
* Replication = new Gcp.SecretManager.Inputs.SecretReplicationArgs
* {
* Auto = null,
* },
* });
* var example_remote_secretVersion = new Gcp.SecretManager.SecretVersion("example-remote-secret_version", new()
* {
* Secret = example_remote_secret.Id,
* SecretData = "remote-password",
* });
* var secret_access = new Gcp.SecretManager.SecretIamMember("secret-access", new()
* {
* SecretId = example_remote_secret.Id,
* Role = "roles/secretmanager.secretAccessor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-artifactregistry.iam.gserviceaccount.com",
* });
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "example-docker-custom-remote",
* Description = "example remote custom docker repository with credentials",
* Format = "DOCKER",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "custom docker remote with credentials",
* DisableUpstreamValidation = true,
* DockerRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigDockerRepositoryArgs
* {
* CustomRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigDockerRepositoryCustomRepositoryArgs
* {
* Uri = "https://registry-1.docker.io",
* },
* },
* UpstreamCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs
* {
* UsernamePasswordCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs
* {
* Username = "remote-username",
* PasswordSecretVersion = example_remote_secretVersion.Name,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/secretmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecret(ctx, "example-remote-secret", &secretmanager.SecretArgs{
* SecretId: pulumi.String("example-secret"),
* Replication: &secretmanager.SecretReplicationArgs{
* Auto: nil,
* },
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretVersion(ctx, "example-remote-secret_version", &secretmanager.SecretVersionArgs{
* Secret: example_remote_secret.ID(),
* SecretData: pulumi.String("remote-password"),
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretIamMember(ctx, "secret-access", &secretmanager.SecretIamMemberArgs{
* SecretId: example_remote_secret.ID(),
* Role: pulumi.String("roles/secretmanager.secretAccessor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("example-docker-custom-remote"),
* Description: pulumi.String("example remote custom docker repository with credentials"),
* Format: pulumi.String("DOCKER"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("custom docker remote with credentials"),
* DisableUpstreamValidation: pulumi.Bool(true),
* DockerRepository: &artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryArgs{
* CustomRepository: &artifactregistry.RepositoryRemoteRepositoryConfigDockerRepositoryCustomRepositoryArgs{
* Uri: pulumi.String("https://registry-1.docker.io"),
* },
* },
* UpstreamCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs{
* UsernamePasswordCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs{
* Username: pulumi.String("remote-username"),
* PasswordSecretVersion: example_remote_secretVersion.Name,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.secretmanager.Secret;
* import com.pulumi.gcp.secretmanager.SecretArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationAutoArgs;
* import com.pulumi.gcp.secretmanager.SecretVersion;
* import com.pulumi.gcp.secretmanager.SecretVersionArgs;
* import com.pulumi.gcp.secretmanager.SecretIamMember;
* import com.pulumi.gcp.secretmanager.SecretIamMemberArgs;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigDockerRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigDockerRepositoryCustomRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var example_remote_secret = new Secret("example-remote-secret", SecretArgs.builder()
* .secretId("example-secret")
* .replication(SecretReplicationArgs.builder()
* .auto()
* .build())
* .build());
* var example_remote_secretVersion = new SecretVersion("example-remote-secretVersion", SecretVersionArgs.builder()
* .secret(example_remote_secret.id())
* .secretData("remote-password")
* .build());
* var secret_access = new SecretIamMember("secret-access", SecretIamMemberArgs.builder()
* .secretId(example_remote_secret.id())
* .role("roles/secretmanager.secretAccessor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("example-docker-custom-remote")
* .description("example remote custom docker repository with credentials")
* .format("DOCKER")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("custom docker remote with credentials")
* .disableUpstreamValidation(true)
* .dockerRepository(RepositoryRemoteRepositoryConfigDockerRepositoryArgs.builder()
* .customRepository(RepositoryRemoteRepositoryConfigDockerRepositoryCustomRepositoryArgs.builder()
* .uri("https://registry-1.docker.io")
* .build())
* .build())
* .upstreamCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs.builder()
* .usernamePasswordCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs.builder()
* .username("remote-username")
* .passwordSecretVersion(example_remote_secretVersion.name())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example-remote-secret:
* type: gcp:secretmanager:Secret
* properties:
* secretId: example-secret
* replication:
* auto: {}
* example-remote-secretVersion:
* type: gcp:secretmanager:SecretVersion
* name: example-remote-secret_version
* properties:
* secret: ${["example-remote-secret"].id}
* secretData: remote-password
* secret-access:
* type: gcp:secretmanager:SecretIamMember
* properties:
* secretId: ${["example-remote-secret"].id}
* role: roles/secretmanager.secretAccessor
* member: serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: example-docker-custom-remote
* description: example remote custom docker repository with credentials
* format: DOCKER
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: custom docker remote with credentials
* disableUpstreamValidation: true
* dockerRepository:
* customRepository:
* uri: https://registry-1.docker.io
* upstreamCredentials:
* usernamePasswordCredentials:
* username: remote-username
* passwordSecretVersion: ${["example-remote-secretVersion"].name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Artifact Registry Repository Remote Maven Custom With Auth
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const example_remote_secret = new gcp.secretmanager.Secret("example-remote-secret", {
* secretId: "example-secret",
* replication: {
* auto: {},
* },
* });
* const example_remote_secretVersion = new gcp.secretmanager.SecretVersion("example-remote-secret_version", {
* secret: example_remote_secret.id,
* secretData: "remote-password",
* });
* const secret_access = new gcp.secretmanager.SecretIamMember("secret-access", {
* secretId: example_remote_secret.id,
* role: "roles/secretmanager.secretAccessor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com`),
* });
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "example-maven-custom-remote",
* description: "example remote custom maven repository with credentials",
* format: "MAVEN",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "custom maven remote with credentials",
* disableUpstreamValidation: true,
* mavenRepository: {
* customRepository: {
* uri: "https://my.maven.registry",
* },
* },
* upstreamCredentials: {
* usernamePasswordCredentials: {
* username: "remote-username",
* passwordSecretVersion: example_remote_secretVersion.name,
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* example_remote_secret = gcp.secretmanager.Secret("example-remote-secret",
* secret_id="example-secret",
* replication=gcp.secretmanager.SecretReplicationArgs(
* auto=gcp.secretmanager.SecretReplicationAutoArgs(),
* ))
* example_remote_secret_version = gcp.secretmanager.SecretVersion("example-remote-secret_version",
* secret=example_remote_secret.id,
* secret_data="remote-password")
* secret_access = gcp.secretmanager.SecretIamMember("secret-access",
* secret_id=example_remote_secret.id,
* role="roles/secretmanager.secretAccessor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com")
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="example-maven-custom-remote",
* description="example remote custom maven repository with credentials",
* format="MAVEN",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="custom maven remote with credentials",
* disable_upstream_validation=True,
* maven_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigMavenRepositoryArgs(
* custom_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigMavenRepositoryCustomRepositoryArgs(
* uri="https://my.maven.registry",
* ),
* ),
* upstream_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs(
* username_password_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs(
* username="remote-username",
* password_secret_version=example_remote_secret_version.name,
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var example_remote_secret = new Gcp.SecretManager.Secret("example-remote-secret", new()
* {
* SecretId = "example-secret",
* Replication = new Gcp.SecretManager.Inputs.SecretReplicationArgs
* {
* Auto = null,
* },
* });
* var example_remote_secretVersion = new Gcp.SecretManager.SecretVersion("example-remote-secret_version", new()
* {
* Secret = example_remote_secret.Id,
* SecretData = "remote-password",
* });
* var secret_access = new Gcp.SecretManager.SecretIamMember("secret-access", new()
* {
* SecretId = example_remote_secret.Id,
* Role = "roles/secretmanager.secretAccessor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-artifactregistry.iam.gserviceaccount.com",
* });
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "example-maven-custom-remote",
* Description = "example remote custom maven repository with credentials",
* Format = "MAVEN",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "custom maven remote with credentials",
* DisableUpstreamValidation = true,
* MavenRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigMavenRepositoryArgs
* {
* CustomRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigMavenRepositoryCustomRepositoryArgs
* {
* Uri = "https://my.maven.registry",
* },
* },
* UpstreamCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs
* {
* UsernamePasswordCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs
* {
* Username = "remote-username",
* PasswordSecretVersion = example_remote_secretVersion.Name,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/secretmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecret(ctx, "example-remote-secret", &secretmanager.SecretArgs{
* SecretId: pulumi.String("example-secret"),
* Replication: &secretmanager.SecretReplicationArgs{
* Auto: nil,
* },
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretVersion(ctx, "example-remote-secret_version", &secretmanager.SecretVersionArgs{
* Secret: example_remote_secret.ID(),
* SecretData: pulumi.String("remote-password"),
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretIamMember(ctx, "secret-access", &secretmanager.SecretIamMemberArgs{
* SecretId: example_remote_secret.ID(),
* Role: pulumi.String("roles/secretmanager.secretAccessor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("example-maven-custom-remote"),
* Description: pulumi.String("example remote custom maven repository with credentials"),
* Format: pulumi.String("MAVEN"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("custom maven remote with credentials"),
* DisableUpstreamValidation: pulumi.Bool(true),
* MavenRepository: &artifactregistry.RepositoryRemoteRepositoryConfigMavenRepositoryArgs{
* CustomRepository: &artifactregistry.RepositoryRemoteRepositoryConfigMavenRepositoryCustomRepositoryArgs{
* Uri: pulumi.String("https://my.maven.registry"),
* },
* },
* UpstreamCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs{
* UsernamePasswordCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs{
* Username: pulumi.String("remote-username"),
* PasswordSecretVersion: example_remote_secretVersion.Name,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.secretmanager.Secret;
* import com.pulumi.gcp.secretmanager.SecretArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationAutoArgs;
* import com.pulumi.gcp.secretmanager.SecretVersion;
* import com.pulumi.gcp.secretmanager.SecretVersionArgs;
* import com.pulumi.gcp.secretmanager.SecretIamMember;
* import com.pulumi.gcp.secretmanager.SecretIamMemberArgs;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigMavenRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigMavenRepositoryCustomRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var example_remote_secret = new Secret("example-remote-secret", SecretArgs.builder()
* .secretId("example-secret")
* .replication(SecretReplicationArgs.builder()
* .auto()
* .build())
* .build());
* var example_remote_secretVersion = new SecretVersion("example-remote-secretVersion", SecretVersionArgs.builder()
* .secret(example_remote_secret.id())
* .secretData("remote-password")
* .build());
* var secret_access = new SecretIamMember("secret-access", SecretIamMemberArgs.builder()
* .secretId(example_remote_secret.id())
* .role("roles/secretmanager.secretAccessor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("example-maven-custom-remote")
* .description("example remote custom maven repository with credentials")
* .format("MAVEN")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("custom maven remote with credentials")
* .disableUpstreamValidation(true)
* .mavenRepository(RepositoryRemoteRepositoryConfigMavenRepositoryArgs.builder()
* .customRepository(RepositoryRemoteRepositoryConfigMavenRepositoryCustomRepositoryArgs.builder()
* .uri("https://my.maven.registry")
* .build())
* .build())
* .upstreamCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs.builder()
* .usernamePasswordCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs.builder()
* .username("remote-username")
* .passwordSecretVersion(example_remote_secretVersion.name())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example-remote-secret:
* type: gcp:secretmanager:Secret
* properties:
* secretId: example-secret
* replication:
* auto: {}
* example-remote-secretVersion:
* type: gcp:secretmanager:SecretVersion
* name: example-remote-secret_version
* properties:
* secret: ${["example-remote-secret"].id}
* secretData: remote-password
* secret-access:
* type: gcp:secretmanager:SecretIamMember
* properties:
* secretId: ${["example-remote-secret"].id}
* role: roles/secretmanager.secretAccessor
* member: serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: example-maven-custom-remote
* description: example remote custom maven repository with credentials
* format: MAVEN
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: custom maven remote with credentials
* disableUpstreamValidation: true
* mavenRepository:
* customRepository:
* uri: https://my.maven.registry
* upstreamCredentials:
* usernamePasswordCredentials:
* username: remote-username
* passwordSecretVersion: ${["example-remote-secretVersion"].name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Artifact Registry Repository Remote Npm Custom With Auth
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const example_remote_secret = new gcp.secretmanager.Secret("example-remote-secret", {
* secretId: "example-secret",
* replication: {
* auto: {},
* },
* });
* const example_remote_secretVersion = new gcp.secretmanager.SecretVersion("example-remote-secret_version", {
* secret: example_remote_secret.id,
* secretData: "remote-password",
* });
* const secret_access = new gcp.secretmanager.SecretIamMember("secret-access", {
* secretId: example_remote_secret.id,
* role: "roles/secretmanager.secretAccessor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com`),
* });
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "example-npm-custom-remote",
* description: "example remote custom npm repository with credentials",
* format: "NPM",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "custom npm with credentials",
* disableUpstreamValidation: true,
* npmRepository: {
* customRepository: {
* uri: "https://my.npm.registry",
* },
* },
* upstreamCredentials: {
* usernamePasswordCredentials: {
* username: "remote-username",
* passwordSecretVersion: example_remote_secretVersion.name,
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* example_remote_secret = gcp.secretmanager.Secret("example-remote-secret",
* secret_id="example-secret",
* replication=gcp.secretmanager.SecretReplicationArgs(
* auto=gcp.secretmanager.SecretReplicationAutoArgs(),
* ))
* example_remote_secret_version = gcp.secretmanager.SecretVersion("example-remote-secret_version",
* secret=example_remote_secret.id,
* secret_data="remote-password")
* secret_access = gcp.secretmanager.SecretIamMember("secret-access",
* secret_id=example_remote_secret.id,
* role="roles/secretmanager.secretAccessor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com")
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="example-npm-custom-remote",
* description="example remote custom npm repository with credentials",
* format="NPM",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="custom npm with credentials",
* disable_upstream_validation=True,
* npm_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigNpmRepositoryArgs(
* custom_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigNpmRepositoryCustomRepositoryArgs(
* uri="https://my.npm.registry",
* ),
* ),
* upstream_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs(
* username_password_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs(
* username="remote-username",
* password_secret_version=example_remote_secret_version.name,
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var example_remote_secret = new Gcp.SecretManager.Secret("example-remote-secret", new()
* {
* SecretId = "example-secret",
* Replication = new Gcp.SecretManager.Inputs.SecretReplicationArgs
* {
* Auto = null,
* },
* });
* var example_remote_secretVersion = new Gcp.SecretManager.SecretVersion("example-remote-secret_version", new()
* {
* Secret = example_remote_secret.Id,
* SecretData = "remote-password",
* });
* var secret_access = new Gcp.SecretManager.SecretIamMember("secret-access", new()
* {
* SecretId = example_remote_secret.Id,
* Role = "roles/secretmanager.secretAccessor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-artifactregistry.iam.gserviceaccount.com",
* });
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "example-npm-custom-remote",
* Description = "example remote custom npm repository with credentials",
* Format = "NPM",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "custom npm with credentials",
* DisableUpstreamValidation = true,
* NpmRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigNpmRepositoryArgs
* {
* CustomRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigNpmRepositoryCustomRepositoryArgs
* {
* Uri = "https://my.npm.registry",
* },
* },
* UpstreamCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs
* {
* UsernamePasswordCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs
* {
* Username = "remote-username",
* PasswordSecretVersion = example_remote_secretVersion.Name,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/secretmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecret(ctx, "example-remote-secret", &secretmanager.SecretArgs{
* SecretId: pulumi.String("example-secret"),
* Replication: &secretmanager.SecretReplicationArgs{
* Auto: nil,
* },
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretVersion(ctx, "example-remote-secret_version", &secretmanager.SecretVersionArgs{
* Secret: example_remote_secret.ID(),
* SecretData: pulumi.String("remote-password"),
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretIamMember(ctx, "secret-access", &secretmanager.SecretIamMemberArgs{
* SecretId: example_remote_secret.ID(),
* Role: pulumi.String("roles/secretmanager.secretAccessor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("example-npm-custom-remote"),
* Description: pulumi.String("example remote custom npm repository with credentials"),
* Format: pulumi.String("NPM"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("custom npm with credentials"),
* DisableUpstreamValidation: pulumi.Bool(true),
* NpmRepository: &artifactregistry.RepositoryRemoteRepositoryConfigNpmRepositoryArgs{
* CustomRepository: &artifactregistry.RepositoryRemoteRepositoryConfigNpmRepositoryCustomRepositoryArgs{
* Uri: pulumi.String("https://my.npm.registry"),
* },
* },
* UpstreamCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs{
* UsernamePasswordCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs{
* Username: pulumi.String("remote-username"),
* PasswordSecretVersion: example_remote_secretVersion.Name,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.secretmanager.Secret;
* import com.pulumi.gcp.secretmanager.SecretArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationAutoArgs;
* import com.pulumi.gcp.secretmanager.SecretVersion;
* import com.pulumi.gcp.secretmanager.SecretVersionArgs;
* import com.pulumi.gcp.secretmanager.SecretIamMember;
* import com.pulumi.gcp.secretmanager.SecretIamMemberArgs;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigNpmRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigNpmRepositoryCustomRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var example_remote_secret = new Secret("example-remote-secret", SecretArgs.builder()
* .secretId("example-secret")
* .replication(SecretReplicationArgs.builder()
* .auto()
* .build())
* .build());
* var example_remote_secretVersion = new SecretVersion("example-remote-secretVersion", SecretVersionArgs.builder()
* .secret(example_remote_secret.id())
* .secretData("remote-password")
* .build());
* var secret_access = new SecretIamMember("secret-access", SecretIamMemberArgs.builder()
* .secretId(example_remote_secret.id())
* .role("roles/secretmanager.secretAccessor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("example-npm-custom-remote")
* .description("example remote custom npm repository with credentials")
* .format("NPM")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("custom npm with credentials")
* .disableUpstreamValidation(true)
* .npmRepository(RepositoryRemoteRepositoryConfigNpmRepositoryArgs.builder()
* .customRepository(RepositoryRemoteRepositoryConfigNpmRepositoryCustomRepositoryArgs.builder()
* .uri("https://my.npm.registry")
* .build())
* .build())
* .upstreamCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs.builder()
* .usernamePasswordCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs.builder()
* .username("remote-username")
* .passwordSecretVersion(example_remote_secretVersion.name())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example-remote-secret:
* type: gcp:secretmanager:Secret
* properties:
* secretId: example-secret
* replication:
* auto: {}
* example-remote-secretVersion:
* type: gcp:secretmanager:SecretVersion
* name: example-remote-secret_version
* properties:
* secret: ${["example-remote-secret"].id}
* secretData: remote-password
* secret-access:
* type: gcp:secretmanager:SecretIamMember
* properties:
* secretId: ${["example-remote-secret"].id}
* role: roles/secretmanager.secretAccessor
* member: serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: example-npm-custom-remote
* description: example remote custom npm repository with credentials
* format: NPM
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: custom npm with credentials
* disableUpstreamValidation: true
* npmRepository:
* customRepository:
* uri: https://my.npm.registry
* upstreamCredentials:
* usernamePasswordCredentials:
* username: remote-username
* passwordSecretVersion: ${["example-remote-secretVersion"].name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Artifact Registry Repository Remote Python Custom With Auth
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const example_remote_secret = new gcp.secretmanager.Secret("example-remote-secret", {
* secretId: "example-secret",
* replication: {
* auto: {},
* },
* });
* const example_remote_secretVersion = new gcp.secretmanager.SecretVersion("example-remote-secret_version", {
* secret: example_remote_secret.id,
* secretData: "remote-password",
* });
* const secret_access = new gcp.secretmanager.SecretIamMember("secret-access", {
* secretId: example_remote_secret.id,
* role: "roles/secretmanager.secretAccessor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com`),
* });
* const my_repo = new gcp.artifactregistry.Repository("my-repo", {
* location: "us-central1",
* repositoryId: "example-python-custom-remote",
* description: "example remote custom python repository with credentials",
* format: "PYTHON",
* mode: "REMOTE_REPOSITORY",
* remoteRepositoryConfig: {
* description: "custom npm with credentials",
* disableUpstreamValidation: true,
* pythonRepository: {
* customRepository: {
* uri: "https://my.python.registry",
* },
* },
* upstreamCredentials: {
* usernamePasswordCredentials: {
* username: "remote-username",
* passwordSecretVersion: example_remote_secretVersion.name,
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* example_remote_secret = gcp.secretmanager.Secret("example-remote-secret",
* secret_id="example-secret",
* replication=gcp.secretmanager.SecretReplicationArgs(
* auto=gcp.secretmanager.SecretReplicationAutoArgs(),
* ))
* example_remote_secret_version = gcp.secretmanager.SecretVersion("example-remote-secret_version",
* secret=example_remote_secret.id,
* secret_data="remote-password")
* secret_access = gcp.secretmanager.SecretIamMember("secret-access",
* secret_id=example_remote_secret.id,
* role="roles/secretmanager.secretAccessor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com")
* my_repo = gcp.artifactregistry.Repository("my-repo",
* location="us-central1",
* repository_id="example-python-custom-remote",
* description="example remote custom python repository with credentials",
* format="PYTHON",
* mode="REMOTE_REPOSITORY",
* remote_repository_config=gcp.artifactregistry.RepositoryRemoteRepositoryConfigArgs(
* description="custom npm with credentials",
* disable_upstream_validation=True,
* python_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigPythonRepositoryArgs(
* custom_repository=gcp.artifactregistry.RepositoryRemoteRepositoryConfigPythonRepositoryCustomRepositoryArgs(
* uri="https://my.python.registry",
* ),
* ),
* upstream_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs(
* username_password_credentials=gcp.artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs(
* username="remote-username",
* password_secret_version=example_remote_secret_version.name,
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var example_remote_secret = new Gcp.SecretManager.Secret("example-remote-secret", new()
* {
* SecretId = "example-secret",
* Replication = new Gcp.SecretManager.Inputs.SecretReplicationArgs
* {
* Auto = null,
* },
* });
* var example_remote_secretVersion = new Gcp.SecretManager.SecretVersion("example-remote-secret_version", new()
* {
* Secret = example_remote_secret.Id,
* SecretData = "remote-password",
* });
* var secret_access = new Gcp.SecretManager.SecretIamMember("secret-access", new()
* {
* SecretId = example_remote_secret.Id,
* Role = "roles/secretmanager.secretAccessor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-artifactregistry.iam.gserviceaccount.com",
* });
* var my_repo = new Gcp.ArtifactRegistry.Repository("my-repo", new()
* {
* Location = "us-central1",
* RepositoryId = "example-python-custom-remote",
* Description = "example remote custom python repository with credentials",
* Format = "PYTHON",
* Mode = "REMOTE_REPOSITORY",
* RemoteRepositoryConfig = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigArgs
* {
* Description = "custom npm with credentials",
* DisableUpstreamValidation = true,
* PythonRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigPythonRepositoryArgs
* {
* CustomRepository = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigPythonRepositoryCustomRepositoryArgs
* {
* Uri = "https://my.python.registry",
* },
* },
* UpstreamCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs
* {
* UsernamePasswordCredentials = new Gcp.ArtifactRegistry.Inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs
* {
* Username = "remote-username",
* PasswordSecretVersion = example_remote_secretVersion.Name,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/artifactregistry"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/secretmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecret(ctx, "example-remote-secret", &secretmanager.SecretArgs{
* SecretId: pulumi.String("example-secret"),
* Replication: &secretmanager.SecretReplicationArgs{
* Auto: nil,
* },
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretVersion(ctx, "example-remote-secret_version", &secretmanager.SecretVersionArgs{
* Secret: example_remote_secret.ID(),
* SecretData: pulumi.String("remote-password"),
* })
* if err != nil {
* return err
* }
* _, err = secretmanager.NewSecretIamMember(ctx, "secret-access", &secretmanager.SecretIamMemberArgs{
* SecretId: example_remote_secret.ID(),
* Role: pulumi.String("roles/secretmanager.secretAccessor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = artifactregistry.NewRepository(ctx, "my-repo", &artifactregistry.RepositoryArgs{
* Location: pulumi.String("us-central1"),
* RepositoryId: pulumi.String("example-python-custom-remote"),
* Description: pulumi.String("example remote custom python repository with credentials"),
* Format: pulumi.String("PYTHON"),
* Mode: pulumi.String("REMOTE_REPOSITORY"),
* RemoteRepositoryConfig: &artifactregistry.RepositoryRemoteRepositoryConfigArgs{
* Description: pulumi.String("custom npm with credentials"),
* DisableUpstreamValidation: pulumi.Bool(true),
* PythonRepository: &artifactregistry.RepositoryRemoteRepositoryConfigPythonRepositoryArgs{
* CustomRepository: &artifactregistry.RepositoryRemoteRepositoryConfigPythonRepositoryCustomRepositoryArgs{
* Uri: pulumi.String("https://my.python.registry"),
* },
* },
* UpstreamCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs{
* UsernamePasswordCredentials: &artifactregistry.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs{
* Username: pulumi.String("remote-username"),
* PasswordSecretVersion: example_remote_secretVersion.Name,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.secretmanager.Secret;
* import com.pulumi.gcp.secretmanager.SecretArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationArgs;
* import com.pulumi.gcp.secretmanager.inputs.SecretReplicationAutoArgs;
* import com.pulumi.gcp.secretmanager.SecretVersion;
* import com.pulumi.gcp.secretmanager.SecretVersionArgs;
* import com.pulumi.gcp.secretmanager.SecretIamMember;
* import com.pulumi.gcp.secretmanager.SecretIamMemberArgs;
* import com.pulumi.gcp.artifactregistry.Repository;
* import com.pulumi.gcp.artifactregistry.RepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigPythonRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigPythonRepositoryCustomRepositoryArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs;
* import com.pulumi.gcp.artifactregistry.inputs.RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var example_remote_secret = new Secret("example-remote-secret", SecretArgs.builder()
* .secretId("example-secret")
* .replication(SecretReplicationArgs.builder()
* .auto()
* .build())
* .build());
* var example_remote_secretVersion = new SecretVersion("example-remote-secretVersion", SecretVersionArgs.builder()
* .secret(example_remote_secret.id())
* .secretData("remote-password")
* .build());
* var secret_access = new SecretIamMember("secret-access", SecretIamMemberArgs.builder()
* .secretId(example_remote_secret.id())
* .role("roles/secretmanager.secretAccessor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .location("us-central1")
* .repositoryId("example-python-custom-remote")
* .description("example remote custom python repository with credentials")
* .format("PYTHON")
* .mode("REMOTE_REPOSITORY")
* .remoteRepositoryConfig(RepositoryRemoteRepositoryConfigArgs.builder()
* .description("custom npm with credentials")
* .disableUpstreamValidation(true)
* .pythonRepository(RepositoryRemoteRepositoryConfigPythonRepositoryArgs.builder()
* .customRepository(RepositoryRemoteRepositoryConfigPythonRepositoryCustomRepositoryArgs.builder()
* .uri("https://my.python.registry")
* .build())
* .build())
* .upstreamCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsArgs.builder()
* .usernamePasswordCredentials(RepositoryRemoteRepositoryConfigUpstreamCredentialsUsernamePasswordCredentialsArgs.builder()
* .username("remote-username")
* .passwordSecretVersion(example_remote_secretVersion.name())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example-remote-secret:
* type: gcp:secretmanager:Secret
* properties:
* secretId: example-secret
* replication:
* auto: {}
* example-remote-secretVersion:
* type: gcp:secretmanager:SecretVersion
* name: example-remote-secret_version
* properties:
* secret: ${["example-remote-secret"].id}
* secretData: remote-password
* secret-access:
* type: gcp:secretmanager:SecretIamMember
* properties:
* secretId: ${["example-remote-secret"].id}
* role: roles/secretmanager.secretAccessor
* member: serviceAccount:service-${project.number}@gcp-sa-artifactregistry.iam.gserviceaccount.com
* my-repo:
* type: gcp:artifactregistry:Repository
* properties:
* location: us-central1
* repositoryId: example-python-custom-remote
* description: example remote custom python repository with credentials
* format: PYTHON
* mode: REMOTE_REPOSITORY
* remoteRepositoryConfig:
* description: custom npm with credentials
* disableUpstreamValidation: true
* pythonRepository:
* customRepository:
* uri: https://my.python.registry
* upstreamCredentials:
* usernamePasswordCredentials:
* username: remote-username
* passwordSecretVersion: ${["example-remote-secretVersion"].name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Repository can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/repositories/{{repository_id}}`
* * `{{project}}/{{location}}/{{repository_id}}`
* * `{{location}}/{{repository_id}}`
* * `{{repository_id}}`
* When using the `pulumi import` command, Repository can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:artifactregistry/repository:Repository default projects/{{project}}/locations/{{location}}/repositories/{{repository_id}}
* ```
* ```sh
* $ pulumi import gcp:artifactregistry/repository:Repository default {{project}}/{{location}}/{{repository_id}}
* ```
* ```sh
* $ pulumi import gcp:artifactregistry/repository:Repository default {{location}}/{{repository_id}}
* ```
* ```sh
* $ pulumi import gcp:artifactregistry/repository:Repository default {{repository_id}}
* ```
* @property cleanupPolicies Cleanup policies for this repository. Cleanup policies indicate when
* certain package versions can be automatically deleted.
* Map keys are policy IDs supplied by users during policy creation. They must
* unique within a repository and be under 128 characters in length.
* Structure is documented below.
* @property cleanupPolicyDryRun If true, the cleanup pipeline is prevented from deleting versions in this
* repository.
* @property description The user-provided description of the repository.
* @property dockerConfig Docker repository config contains repository level configuration for the repositories of docker type.
* Structure is documented below.
* @property format The format of packages that are stored in the repository. Supported formats
* can be found [here](https://cloud.google.com/artifact-registry/docs/supported-formats).
* You can only create alpha formats if you are a member of the
* [alpha user group](https://cloud.google.com/artifact-registry/docs/supported-formats#alpha-access).
* - - -
* @property kmsKeyName The Cloud KMS resource name of the customer managed encryption key that’s
* used to encrypt the contents of the Repository. Has the form:
* `projects/my-project/locations/my-region/keyRings/my-kr/cryptoKeys/my-key`.
* This value may not be changed after the Repository has been created.
* @property labels Labels with user-defined metadata.
* This field may contain up to 64 entries. Label keys and values may be no
* longer than 63 characters. Label keys must begin with a lowercase letter
* and may only contain lowercase letters, numeric characters, underscores,
* and dashes.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property location The name of the location this repository is located in.
* @property mavenConfig MavenRepositoryConfig is maven related repository details.
* Provides additional configuration details for repositories of the maven
* format type.
* Structure is documented below.
* @property mode The mode configures the repository to serve artifacts from different sources.
* Default value is `STANDARD_REPOSITORY`.
* Possible values are: `STANDARD_REPOSITORY`, `VIRTUAL_REPOSITORY`, `REMOTE_REPOSITORY`.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property remoteRepositoryConfig Configuration specific for a Remote Repository.
* Structure is documented below.
* @property repositoryId The last part of the repository name, for example:
* "repo1"
* @property virtualRepositoryConfig Configuration specific for a Virtual Repository.
* Structure is documented below.
*/
public data class RepositoryArgs(
public val cleanupPolicies: Output>? = null,
public val cleanupPolicyDryRun: Output? = null,
public val description: Output? = null,
public val dockerConfig: Output? = null,
public val format: Output? = null,
public val kmsKeyName: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy