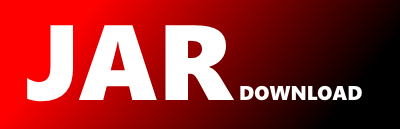
com.pulumi.gcp.bigquery.kotlin.CapacityCommitment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [CapacityCommitment].
*/
@PulumiTagMarker
public class CapacityCommitmentResourceBuilder internal constructor() {
public var name: String? = null
public var args: CapacityCommitmentArgs = CapacityCommitmentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend CapacityCommitmentArgsBuilder.() -> Unit) {
val builder = CapacityCommitmentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): CapacityCommitment {
val builtJavaResource = com.pulumi.gcp.bigquery.CapacityCommitment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return CapacityCommitment(builtJavaResource)
}
}
/**
* Capacity commitment is a way to purchase compute capacity for BigQuery jobs (in the form of slots) with some committed period of usage. Annual commitments renew by default. Commitments can be removed after their commitment end time passes.
* In order to remove annual commitment, its plan needs to be changed to monthly or flex first.
* To get more information about CapacityCommitment, see:
* * [API documentation](https://cloud.google.com/bigquery/docs/reference/reservations/rest/v1/projects.locations.capacityCommitments)
* * How-to Guides
* * [Introduction to Reservations](https://cloud.google.com/bigquery/docs/reservations-intro)
* ## Example Usage
* ### Bigquery Reservation Capacity Commitment Docs
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.bigquery.CapacityCommitment("example", {
* capacityCommitmentId: "example-commitment",
* location: "us-west2",
* slotCount: 100,
* plan: "FLEX_FLAT_RATE",
* edition: "ENTERPRISE",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.bigquery.CapacityCommitment("example",
* capacity_commitment_id="example-commitment",
* location="us-west2",
* slot_count=100,
* plan="FLEX_FLAT_RATE",
* edition="ENTERPRISE")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.BigQuery.CapacityCommitment("example", new()
* {
* CapacityCommitmentId = "example-commitment",
* Location = "us-west2",
* SlotCount = 100,
* Plan = "FLEX_FLAT_RATE",
* Edition = "ENTERPRISE",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/bigquery"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := bigquery.NewCapacityCommitment(ctx, "example", &bigquery.CapacityCommitmentArgs{
* CapacityCommitmentId: pulumi.String("example-commitment"),
* Location: pulumi.String("us-west2"),
* SlotCount: pulumi.Int(100),
* Plan: pulumi.String("FLEX_FLAT_RATE"),
* Edition: pulumi.String("ENTERPRISE"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.bigquery.CapacityCommitment;
* import com.pulumi.gcp.bigquery.CapacityCommitmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new CapacityCommitment("example", CapacityCommitmentArgs.builder()
* .capacityCommitmentId("example-commitment")
* .location("us-west2")
* .slotCount(100)
* .plan("FLEX_FLAT_RATE")
* .edition("ENTERPRISE")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:bigquery:CapacityCommitment
* properties:
* capacityCommitmentId: example-commitment
* location: us-west2
* slotCount: 100
* plan: FLEX_FLAT_RATE
* edition: ENTERPRISE
* ```
*
* ## Import
* CapacityCommitment can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/capacityCommitments/{{capacity_commitment_id}}`
* * `{{project}}/{{location}}/{{capacity_commitment_id}}`
* * `{{location}}/{{capacity_commitment_id}}`
* When using the `pulumi import` command, CapacityCommitment can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:bigquery/capacityCommitment:CapacityCommitment default projects/{{project}}/locations/{{location}}/capacityCommitments/{{capacity_commitment_id}}
* ```
* ```sh
* $ pulumi import gcp:bigquery/capacityCommitment:CapacityCommitment default {{project}}/{{location}}/{{capacity_commitment_id}}
* ```
* ```sh
* $ pulumi import gcp:bigquery/capacityCommitment:CapacityCommitment default {{location}}/{{capacity_commitment_id}}
* ```
*/
public class CapacityCommitment internal constructor(
override val javaResource: com.pulumi.gcp.bigquery.CapacityCommitment,
) : KotlinCustomResource(javaResource, CapacityCommitmentMapper) {
/**
* The optional capacity commitment ID. Capacity commitment name will be generated automatically if this field is
* empty. This field must only contain lower case alphanumeric characters or dashes. The first and last character
* cannot be a dash. Max length is 64 characters. NOTE: this ID won't be kept if the capacity commitment is split
* or merged.
*/
public val capacityCommitmentId: Output?
get() = javaResource.capacityCommitmentId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The start of the current commitment period. It is applicable only for ACTIVE capacity commitments.
*/
public val commitmentEndTime: Output
get() = javaResource.commitmentEndTime().applyValue({ args0 -> args0 })
/**
* The start of the current commitment period. It is applicable only for ACTIVE capacity commitments.
*/
public val commitmentStartTime: Output
get() = javaResource.commitmentStartTime().applyValue({ args0 -> args0 })
/**
* The edition type. Valid values are STANDARD, ENTERPRISE, ENTERPRISE_PLUS
*/
public val edition: Output?
get() = javaResource.edition().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* If true, fail the request if another project in the organization has a capacity commitment.
*/
public val enforceSingleAdminProjectPerOrg: Output?
get() = javaResource.enforceSingleAdminProjectPerOrg().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The geographic location where the transfer config should reside.
* Examples: US, EU, asia-northeast1. The default value is US.
*/
public val location: Output?
get() = javaResource.location().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The resource name of the capacity commitment, e.g., projects/myproject/locations/US/capacityCommitments/123
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Capacity commitment plan. Valid values are at https://cloud.google.com/bigquery/docs/reference/reservations/rpc/google.cloud.bigquery.reservation.v1#commitmentplan
* - - -
*/
public val plan: Output
get() = javaResource.plan().applyValue({ args0 -> args0 })
/**
* The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The plan this capacity commitment is converted to after commitmentEndTime passes. Once the plan is changed, committed period is extended according to commitment plan. Only applicable for some commitment plans.
*/
public val renewalPlan: Output?
get() = javaResource.renewalPlan().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Number of slots in this commitment.
*/
public val slotCount: Output
get() = javaResource.slotCount().applyValue({ args0 -> args0 })
/**
* State of the commitment
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 -> args0 })
}
public object CapacityCommitmentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.bigquery.CapacityCommitment::class == javaResource::class
override fun map(javaResource: Resource): CapacityCommitment = CapacityCommitment(
javaResource as
com.pulumi.gcp.bigquery.CapacityCommitment,
)
}
/**
* @see [CapacityCommitment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [CapacityCommitment].
*/
public suspend fun capacityCommitment(
name: String,
block: suspend CapacityCommitmentResourceBuilder.() -> Unit,
): CapacityCommitment {
val builder = CapacityCommitmentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [CapacityCommitment].
* @param name The _unique_ name of the resulting resource.
*/
public fun capacityCommitment(name: String): CapacityCommitment {
val builder = CapacityCommitmentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy