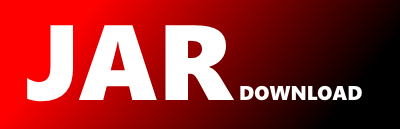
com.pulumi.gcp.bigquery.kotlin.Table.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.bigquery.kotlin.outputs.TableEncryptionConfiguration
import com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfiguration
import com.pulumi.gcp.bigquery.kotlin.outputs.TableMaterializedView
import com.pulumi.gcp.bigquery.kotlin.outputs.TableRangePartitioning
import com.pulumi.gcp.bigquery.kotlin.outputs.TableTableConstraints
import com.pulumi.gcp.bigquery.kotlin.outputs.TableTableReplicationInfo
import com.pulumi.gcp.bigquery.kotlin.outputs.TableTimePartitioning
import com.pulumi.gcp.bigquery.kotlin.outputs.TableView
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.bigquery.kotlin.outputs.TableEncryptionConfiguration.Companion.toKotlin as tableEncryptionConfigurationToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfiguration.Companion.toKotlin as tableExternalDataConfigurationToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableMaterializedView.Companion.toKotlin as tableMaterializedViewToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableRangePartitioning.Companion.toKotlin as tableRangePartitioningToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableTableConstraints.Companion.toKotlin as tableTableConstraintsToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableTableReplicationInfo.Companion.toKotlin as tableTableReplicationInfoToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableTimePartitioning.Companion.toKotlin as tableTimePartitioningToKotlin
import com.pulumi.gcp.bigquery.kotlin.outputs.TableView.Companion.toKotlin as tableViewToKotlin
/**
* Builder for [Table].
*/
@PulumiTagMarker
public class TableResourceBuilder internal constructor() {
public var name: String? = null
public var args: TableArgs = TableArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TableArgsBuilder.() -> Unit) {
val builder = TableArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Table {
val builtJavaResource = com.pulumi.gcp.bigquery.Table(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Table(builtJavaResource)
}
}
/**
* Creates a table resource in a dataset for Google BigQuery. For more information see
* [the official documentation](https://cloud.google.com/bigquery/docs/) and
* [API](https://cloud.google.com/bigquery/docs/reference/rest/v2/tables).
* > **Note**: On newer versions of the provider, you must explicitly set `deletion_protection=false`
* (and run `pulumi update` to write the field to state) in order to destroy an instance.
* It is recommended to not set this field (or set it to true) until you're ready to destroy.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.bigquery.Dataset("default", {
* datasetId: "foo",
* friendlyName: "test",
* description: "This is a test description",
* location: "EU",
* defaultTableExpirationMs: 3600000,
* labels: {
* env: "default",
* },
* });
* const defaultTable = new gcp.bigquery.Table("default", {
* datasetId: _default.datasetId,
* tableId: "bar",
* timePartitioning: {
* type: "DAY",
* },
* labels: {
* env: "default",
* },
* schema: `[
* {
* "name": "permalink",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The Permalink"
* },
* {
* "name": "state",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "State where the head office is located"
* }
* ]
* `,
* });
* const sheet = new gcp.bigquery.Table("sheet", {
* datasetId: _default.datasetId,
* tableId: "sheet",
* externalDataConfiguration: {
* autodetect: true,
* sourceFormat: "GOOGLE_SHEETS",
* googleSheetsOptions: {
* skipLeadingRows: 1,
* },
* sourceUris: ["https://docs.google.com/spreadsheets/d/123456789012345"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.bigquery.Dataset("default",
* dataset_id="foo",
* friendly_name="test",
* description="This is a test description",
* location="EU",
* default_table_expiration_ms=3600000,
* labels={
* "env": "default",
* })
* default_table = gcp.bigquery.Table("default",
* dataset_id=default.dataset_id,
* table_id="bar",
* time_partitioning=gcp.bigquery.TableTimePartitioningArgs(
* type="DAY",
* ),
* labels={
* "env": "default",
* },
* schema="""[
* {
* "name": "permalink",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The Permalink"
* },
* {
* "name": "state",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "State where the head office is located"
* }
* ]
* """)
* sheet = gcp.bigquery.Table("sheet",
* dataset_id=default.dataset_id,
* table_id="sheet",
* external_data_configuration=gcp.bigquery.TableExternalDataConfigurationArgs(
* autodetect=True,
* source_format="GOOGLE_SHEETS",
* google_sheets_options=gcp.bigquery.TableExternalDataConfigurationGoogleSheetsOptionsArgs(
* skip_leading_rows=1,
* ),
* source_uris=["https://docs.google.com/spreadsheets/d/123456789012345"],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.BigQuery.Dataset("default", new()
* {
* DatasetId = "foo",
* FriendlyName = "test",
* Description = "This is a test description",
* Location = "EU",
* DefaultTableExpirationMs = 3600000,
* Labels =
* {
* { "env", "default" },
* },
* });
* var defaultTable = new Gcp.BigQuery.Table("default", new()
* {
* DatasetId = @default.DatasetId,
* TableId = "bar",
* TimePartitioning = new Gcp.BigQuery.Inputs.TableTimePartitioningArgs
* {
* Type = "DAY",
* },
* Labels =
* {
* { "env", "default" },
* },
* Schema = @"[
* {
* ""name"": ""permalink"",
* ""type"": ""STRING"",
* ""mode"": ""NULLABLE"",
* ""description"": ""The Permalink""
* },
* {
* ""name"": ""state"",
* ""type"": ""STRING"",
* ""mode"": ""NULLABLE"",
* ""description"": ""State where the head office is located""
* }
* ]
* ",
* });
* var sheet = new Gcp.BigQuery.Table("sheet", new()
* {
* DatasetId = @default.DatasetId,
* TableId = "sheet",
* ExternalDataConfiguration = new Gcp.BigQuery.Inputs.TableExternalDataConfigurationArgs
* {
* Autodetect = true,
* SourceFormat = "GOOGLE_SHEETS",
* GoogleSheetsOptions = new Gcp.BigQuery.Inputs.TableExternalDataConfigurationGoogleSheetsOptionsArgs
* {
* SkipLeadingRows = 1,
* },
* SourceUris = new[]
* {
* "https://docs.google.com/spreadsheets/d/123456789012345",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/bigquery"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := bigquery.NewDataset(ctx, "default", &bigquery.DatasetArgs{
* DatasetId: pulumi.String("foo"),
* FriendlyName: pulumi.String("test"),
* Description: pulumi.String("This is a test description"),
* Location: pulumi.String("EU"),
* DefaultTableExpirationMs: pulumi.Int(3600000),
* Labels: pulumi.StringMap{
* "env": pulumi.String("default"),
* },
* })
* if err != nil {
* return err
* }
* _, err = bigquery.NewTable(ctx, "default", &bigquery.TableArgs{
* DatasetId: _default.DatasetId,
* TableId: pulumi.String("bar"),
* TimePartitioning: &bigquery.TableTimePartitioningArgs{
* Type: pulumi.String("DAY"),
* },
* Labels: pulumi.StringMap{
* "env": pulumi.String("default"),
* },
* Schema: pulumi.String(`[
* {
* "name": "permalink",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The Permalink"
* },
* {
* "name": "state",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "State where the head office is located"
* }
* ]
* `),
* })
* if err != nil {
* return err
* }
* _, err = bigquery.NewTable(ctx, "sheet", &bigquery.TableArgs{
* DatasetId: _default.DatasetId,
* TableId: pulumi.String("sheet"),
* ExternalDataConfiguration: &bigquery.TableExternalDataConfigurationArgs{
* Autodetect: pulumi.Bool(true),
* SourceFormat: pulumi.String("GOOGLE_SHEETS"),
* GoogleSheetsOptions: &bigquery.TableExternalDataConfigurationGoogleSheetsOptionsArgs{
* SkipLeadingRows: pulumi.Int(1),
* },
* SourceUris: pulumi.StringArray{
* pulumi.String("https://docs.google.com/spreadsheets/d/123456789012345"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.bigquery.Dataset;
* import com.pulumi.gcp.bigquery.DatasetArgs;
* import com.pulumi.gcp.bigquery.Table;
* import com.pulumi.gcp.bigquery.TableArgs;
* import com.pulumi.gcp.bigquery.inputs.TableTimePartitioningArgs;
* import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationArgs;
* import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationGoogleSheetsOptionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Dataset("default", DatasetArgs.builder()
* .datasetId("foo")
* .friendlyName("test")
* .description("This is a test description")
* .location("EU")
* .defaultTableExpirationMs(3600000)
* .labels(Map.of("env", "default"))
* .build());
* var defaultTable = new Table("defaultTable", TableArgs.builder()
* .datasetId(default_.datasetId())
* .tableId("bar")
* .timePartitioning(TableTimePartitioningArgs.builder()
* .type("DAY")
* .build())
* .labels(Map.of("env", "default"))
* .schema("""
* [
* {
* "name": "permalink",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The Permalink"
* },
* {
* "name": "state",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "State where the head office is located"
* }
* ]
* """)
* .build());
* var sheet = new Table("sheet", TableArgs.builder()
* .datasetId(default_.datasetId())
* .tableId("sheet")
* .externalDataConfiguration(TableExternalDataConfigurationArgs.builder()
* .autodetect(true)
* .sourceFormat("GOOGLE_SHEETS")
* .googleSheetsOptions(TableExternalDataConfigurationGoogleSheetsOptionsArgs.builder()
* .skipLeadingRows(1)
* .build())
* .sourceUris("https://docs.google.com/spreadsheets/d/123456789012345")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:bigquery:Dataset
* properties:
* datasetId: foo
* friendlyName: test
* description: This is a test description
* location: EU
* defaultTableExpirationMs: 3.6e+06
* labels:
* env: default
* defaultTable:
* type: gcp:bigquery:Table
* name: default
* properties:
* datasetId: ${default.datasetId}
* tableId: bar
* timePartitioning:
* type: DAY
* labels:
* env: default
* schema: |
* [
* {
* "name": "permalink",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The Permalink"
* },
* {
* "name": "state",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "State where the head office is located"
* }
* ]
* sheet:
* type: gcp:bigquery:Table
* properties:
* datasetId: ${default.datasetId}
* tableId: sheet
* externalDataConfiguration:
* autodetect: true
* sourceFormat: GOOGLE_SHEETS
* googleSheetsOptions:
* skipLeadingRows: 1
* sourceUris:
* - https://docs.google.com/spreadsheets/d/123456789012345
* ```
*
* ## Import
* BigQuery tables can be imported using any of these accepted formats:
* * `projects/{{project}}/datasets/{{dataset_id}}/tables/{{table_id}}`
* * `{{project}}/{{dataset_id}}/{{table_id}}`
* * `{{dataset_id}}/{{table_id}}`
* When using the `pulumi import` command, BigQuery tables can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:bigquery/table:Table default projects/{{project}}/datasets/{{dataset_id}}/tables/{{table_id}}
* ```
* ```sh
* $ pulumi import gcp:bigquery/table:Table default {{project}}/{{dataset_id}}/{{table_id}}
* ```
* ```sh
* $ pulumi import gcp:bigquery/table:Table default {{dataset_id}}/{{table_id}}
* ```
*/
public class Table internal constructor(
override val javaResource: com.pulumi.gcp.bigquery.Table,
) : KotlinCustomResource(javaResource, TableMapper) {
/**
* Whether or not to allow table deletion when there are still resource tags attached.
*/
public val allowResourceTagsOnDeletion: Output?
get() = javaResource.allowResourceTagsOnDeletion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies column names to use for data clustering.
* Up to four top-level columns are allowed, and should be specified in
* descending priority order.
*/
public val clusterings: Output>?
get() = javaResource.clusterings().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The time when this table was created, in milliseconds since the epoch.
*/
public val creationTime: Output
get() = javaResource.creationTime().applyValue({ args0 -> args0 })
/**
* The dataset ID to create the table in.
* Changing this forces a new resource to be created.
*/
public val datasetId: Output
get() = javaResource.datasetId().applyValue({ args0 -> args0 })
/**
* Whether or not to allow the provider to destroy the instance. Unless this field is set to false
* in state, a `=destroy` or `=update` that would delete the instance will fail.
*/
public val deletionProtection: Output?
get() = javaResource.deletionProtection().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The field description.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
* * `schema` - (Optional) A JSON schema for the table.
* ~>**NOTE:** Because this field expects a JSON string, any changes to the
* string will create a diff, even if the JSON itself hasn't changed.
* If the API returns a different value for the same schema, e.g. it
* switched the order of values or replaced `STRUCT` field type with `RECORD`
* field type, we currently cannot suppress the recurring diff this causes.
* As a workaround, we recommend using the schema as returned by the API.
* ~>**NOTE:** If you use `external_data_configuration`
* documented below and do **not** set
* `external_data_configuration.connection_id`, schemas must be specified
* with `external_data_configuration.schema`. Otherwise, schemas must be
* specified with this top-level field.
*/
public val effectiveLabels: Output