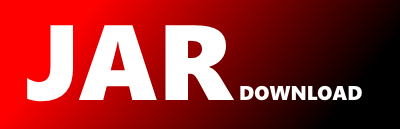
com.pulumi.gcp.bigquery.kotlin.inputs.DatasetAccessArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.DatasetAccessArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property dataset Grants all resources of particular types in a particular dataset read access to the current dataset.
* Structure is documented below.
* @property domain A domain to grant access to. Any users signed in with the
* domain specified will be granted the specified access
* @property groupByEmail An email address of a Google Group to grant access to.
* @property iamMember Some other type of member that appears in the IAM Policy but isn't a user,
* group, domain, or special group. For example: `allUsers`
* @property role Describes the rights granted to the user specified by the other
* member of the access object. Basic, predefined, and custom roles
* are supported. Predefined roles that have equivalent basic roles
* are swapped by the API to their basic counterparts. See
* [official docs](https://cloud.google.com/bigquery/docs/access-control).
* @property routine A routine from a different dataset to grant access to. Queries
* executed against that routine will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that routine is updated by any user, access to the routine
* needs to be granted again via an update operation.
* Structure is documented below.
* @property specialGroup A special group to grant access to. Possible values include:
* * `projectOwners`: Owners of the enclosing project.
* * `projectReaders`: Readers of the enclosing project.
* * `projectWriters`: Writers of the enclosing project.
* * `allAuthenticatedUsers`: All authenticated BigQuery users.
* @property userByEmail An email address of a user to grant access to. For example:
* [email protected]
* @property view A view from a different dataset to grant access to. Queries
* executed against that view will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that view is updated by any user, access to the view
* needs to be granted again via an update operation.
* Structure is documented below.
*/
public data class DatasetAccessArgs(
public val dataset: Output? = null,
public val domain: Output? = null,
public val groupByEmail: Output? = null,
public val iamMember: Output? = null,
public val role: Output? = null,
public val routine: Output? = null,
public val specialGroup: Output? = null,
public val userByEmail: Output? = null,
public val view: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.DatasetAccessArgs =
com.pulumi.gcp.bigquery.inputs.DatasetAccessArgs.builder()
.dataset(dataset?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.domain(domain?.applyValue({ args0 -> args0 }))
.groupByEmail(groupByEmail?.applyValue({ args0 -> args0 }))
.iamMember(iamMember?.applyValue({ args0 -> args0 }))
.role(role?.applyValue({ args0 -> args0 }))
.routine(routine?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.specialGroup(specialGroup?.applyValue({ args0 -> args0 }))
.userByEmail(userByEmail?.applyValue({ args0 -> args0 }))
.view(view?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DatasetAccessArgs].
*/
@PulumiTagMarker
public class DatasetAccessArgsBuilder internal constructor() {
private var dataset: Output? = null
private var domain: Output? = null
private var groupByEmail: Output? = null
private var iamMember: Output? = null
private var role: Output? = null
private var routine: Output? = null
private var specialGroup: Output? = null
private var userByEmail: Output? = null
private var view: Output? = null
/**
* @param value Grants all resources of particular types in a particular dataset read access to the current dataset.
* Structure is documented below.
*/
@JvmName("cyacuvfsbfvsjnfs")
public suspend fun dataset(`value`: Output) {
this.dataset = value
}
/**
* @param value A domain to grant access to. Any users signed in with the
* domain specified will be granted the specified access
*/
@JvmName("vforwvbdcqxhvmjx")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value An email address of a Google Group to grant access to.
*/
@JvmName("hihaajsmkcxynisw")
public suspend fun groupByEmail(`value`: Output) {
this.groupByEmail = value
}
/**
* @param value Some other type of member that appears in the IAM Policy but isn't a user,
* group, domain, or special group. For example: `allUsers`
*/
@JvmName("ycusilxhalgjgoke")
public suspend fun iamMember(`value`: Output) {
this.iamMember = value
}
/**
* @param value Describes the rights granted to the user specified by the other
* member of the access object. Basic, predefined, and custom roles
* are supported. Predefined roles that have equivalent basic roles
* are swapped by the API to their basic counterparts. See
* [official docs](https://cloud.google.com/bigquery/docs/access-control).
*/
@JvmName("blsxhqgeejvlmxoc")
public suspend fun role(`value`: Output) {
this.role = value
}
/**
* @param value A routine from a different dataset to grant access to. Queries
* executed against that routine will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that routine is updated by any user, access to the routine
* needs to be granted again via an update operation.
* Structure is documented below.
*/
@JvmName("krsxfuwdocrmyrpt")
public suspend fun routine(`value`: Output) {
this.routine = value
}
/**
* @param value A special group to grant access to. Possible values include:
* * `projectOwners`: Owners of the enclosing project.
* * `projectReaders`: Readers of the enclosing project.
* * `projectWriters`: Writers of the enclosing project.
* * `allAuthenticatedUsers`: All authenticated BigQuery users.
*/
@JvmName("hkhdfyuhalvxvjro")
public suspend fun specialGroup(`value`: Output) {
this.specialGroup = value
}
/**
* @param value An email address of a user to grant access to. For example:
* [email protected]
*/
@JvmName("gataxukdaequvlmh")
public suspend fun userByEmail(`value`: Output) {
this.userByEmail = value
}
/**
* @param value A view from a different dataset to grant access to. Queries
* executed against that view will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that view is updated by any user, access to the view
* needs to be granted again via an update operation.
* Structure is documented below.
*/
@JvmName("obgxpexaymcpexgl")
public suspend fun view(`value`: Output) {
this.view = value
}
/**
* @param value Grants all resources of particular types in a particular dataset read access to the current dataset.
* Structure is documented below.
*/
@JvmName("wlgrhvibylpysbog")
public suspend fun dataset(`value`: DatasetAccessDatasetArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataset = mapped
}
/**
* @param argument Grants all resources of particular types in a particular dataset read access to the current dataset.
* Structure is documented below.
*/
@JvmName("nyuwydrdlmtviuto")
public suspend fun dataset(argument: suspend DatasetAccessDatasetArgsBuilder.() -> Unit) {
val toBeMapped = DatasetAccessDatasetArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.dataset = mapped
}
/**
* @param value A domain to grant access to. Any users signed in with the
* domain specified will be granted the specified access
*/
@JvmName("puouwylntopbtfbq")
public suspend fun domain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domain = mapped
}
/**
* @param value An email address of a Google Group to grant access to.
*/
@JvmName("iuiybeydfnhftxpb")
public suspend fun groupByEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupByEmail = mapped
}
/**
* @param value Some other type of member that appears in the IAM Policy but isn't a user,
* group, domain, or special group. For example: `allUsers`
*/
@JvmName("ytdufekdsmnipopm")
public suspend fun iamMember(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iamMember = mapped
}
/**
* @param value Describes the rights granted to the user specified by the other
* member of the access object. Basic, predefined, and custom roles
* are supported. Predefined roles that have equivalent basic roles
* are swapped by the API to their basic counterparts. See
* [official docs](https://cloud.google.com/bigquery/docs/access-control).
*/
@JvmName("ifjoahkcfhgrxvly")
public suspend fun role(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value A routine from a different dataset to grant access to. Queries
* executed against that routine will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that routine is updated by any user, access to the routine
* needs to be granted again via an update operation.
* Structure is documented below.
*/
@JvmName("idbpjhrckpenmbwk")
public suspend fun routine(`value`: DatasetAccessRoutineArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.routine = mapped
}
/**
* @param argument A routine from a different dataset to grant access to. Queries
* executed against that routine will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that routine is updated by any user, access to the routine
* needs to be granted again via an update operation.
* Structure is documented below.
*/
@JvmName("juaphpxankkvmgjt")
public suspend fun routine(argument: suspend DatasetAccessRoutineArgsBuilder.() -> Unit) {
val toBeMapped = DatasetAccessRoutineArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.routine = mapped
}
/**
* @param value A special group to grant access to. Possible values include:
* * `projectOwners`: Owners of the enclosing project.
* * `projectReaders`: Readers of the enclosing project.
* * `projectWriters`: Writers of the enclosing project.
* * `allAuthenticatedUsers`: All authenticated BigQuery users.
*/
@JvmName("ugiwljhxubrbxaps")
public suspend fun specialGroup(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.specialGroup = mapped
}
/**
* @param value An email address of a user to grant access to. For example:
* [email protected]
*/
@JvmName("eiggluatuspjbnay")
public suspend fun userByEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userByEmail = mapped
}
/**
* @param value A view from a different dataset to grant access to. Queries
* executed against that view will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that view is updated by any user, access to the view
* needs to be granted again via an update operation.
* Structure is documented below.
*/
@JvmName("upcpbpppqylpjuvg")
public suspend fun view(`value`: DatasetAccessViewArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.view = mapped
}
/**
* @param argument A view from a different dataset to grant access to. Queries
* executed against that view will have read access to tables in
* this dataset. The role field is not required when this field is
* set. If that view is updated by any user, access to the view
* needs to be granted again via an update operation.
* Structure is documented below.
*/
@JvmName("oiwmejceovouistw")
public suspend fun view(argument: suspend DatasetAccessViewArgsBuilder.() -> Unit) {
val toBeMapped = DatasetAccessViewArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.view = mapped
}
internal fun build(): DatasetAccessArgs = DatasetAccessArgs(
dataset = dataset,
domain = domain,
groupByEmail = groupByEmail,
iamMember = iamMember,
role = role,
routine = routine,
specialGroup = specialGroup,
userByEmail = userByEmail,
view = view,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy