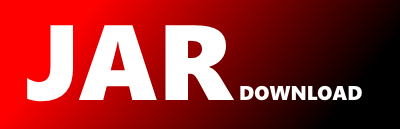
com.pulumi.gcp.bigquery.kotlin.inputs.JobQueryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.JobQueryArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property allowLargeResults If true and query uses legacy SQL dialect, allows the query to produce arbitrarily large result tables at a slight cost in performance.
* Requires destinationTable to be set. For standard SQL queries, this flag is ignored and large results are always allowed.
* However, you must still set destinationTable when result size exceeds the allowed maximum response size.
* @property createDisposition Specifies whether the job is allowed to create new tables. The following values are supported:
* CREATE_IF_NEEDED: If the table does not exist, BigQuery creates the table.
* CREATE_NEVER: The table must already exist. If it does not, a 'notFound' error is returned in the job result.
* Creation, truncation and append actions occur as one atomic update upon job completion
* Default value is `CREATE_IF_NEEDED`.
* Possible values are: `CREATE_IF_NEEDED`, `CREATE_NEVER`.
* @property defaultDataset Specifies the default dataset to use for unqualified table names in the query. Note that this does not alter behavior of unqualified dataset names.
* Structure is documented below.
* @property destinationEncryptionConfiguration Custom encryption configuration (e.g., Cloud KMS keys)
* Structure is documented below.
* @property destinationTable Describes the table where the query results should be stored.
* This property must be set for large results that exceed the maximum response size.
* For queries that produce anonymous (cached) results, this field will be populated by BigQuery.
* Structure is documented below.
* @property flattenResults If true and query uses legacy SQL dialect, flattens all nested and repeated fields in the query results.
* allowLargeResults must be true if this is set to false. For standard SQL queries, this flag is ignored and results are never flattened.
* @property maximumBillingTier Limits the billing tier for this job. Queries that have resource usage beyond this tier will fail (without incurring a charge).
* If unspecified, this will be set to your project default.
* @property maximumBytesBilled Limits the bytes billed for this job. Queries that will have bytes billed beyond this limit will fail (without incurring a charge).
* If unspecified, this will be set to your project default.
* @property parameterMode Standard SQL only. Set to POSITIONAL to use positional (?) query parameters or to NAMED to use named (@myparam) query parameters in this query.
* @property priority Specifies a priority for the query.
* Default value is `INTERACTIVE`.
* Possible values are: `INTERACTIVE`, `BATCH`.
* @property query SQL query text to execute. The useLegacySql field can be used to indicate whether the query uses legacy SQL or standard SQL.
* *NOTE*: queries containing [DML language](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language)
* (`DELETE`, `UPDATE`, `MERGE`, `INSERT`) must specify `create_disposition = ""` and `write_disposition = ""`.
* @property schemaUpdateOptions Allows the schema of the destination table to be updated as a side effect of the query job.
* Schema update options are supported in two cases: when writeDisposition is WRITE_APPEND;
* when writeDisposition is WRITE_TRUNCATE and the destination table is a partition of a table,
* specified by partition decorators. For normal tables, WRITE_TRUNCATE will always overwrite the schema.
* One or more of the following values are specified:
* ALLOW_FIELD_ADDITION: allow adding a nullable field to the schema.
* ALLOW_FIELD_RELAXATION: allow relaxing a required field in the original schema to nullable.
* @property scriptOptions Options controlling the execution of scripts.
* Structure is documented below.
* @property useLegacySql Specifies whether to use BigQuery's legacy SQL dialect for this query. The default value is true.
* If set to false, the query will use BigQuery's standard SQL.
* @property useQueryCache Whether to look for the result in the query cache. The query cache is a best-effort cache that will be flushed whenever
* tables in the query are modified. Moreover, the query cache is only available when a query does not have a destination table specified.
* The default value is true.
* @property userDefinedFunctionResources Describes user-defined function resources used in the query.
* Structure is documented below.
* @property writeDisposition Specifies the action that occurs if the destination table already exists. The following values are supported:
* WRITE_TRUNCATE: If the table already exists, BigQuery overwrites the table data and uses the schema from the query result.
* WRITE_APPEND: If the table already exists, BigQuery appends the data to the table.
* WRITE_EMPTY: If the table already exists and contains data, a 'duplicate' error is returned in the job result.
* Each action is atomic and only occurs if BigQuery is able to complete the job successfully.
* Creation, truncation and append actions occur as one atomic update upon job completion.
* Default value is `WRITE_EMPTY`.
* Possible values are: `WRITE_TRUNCATE`, `WRITE_APPEND`, `WRITE_EMPTY`.
*/
public data class JobQueryArgs(
public val allowLargeResults: Output? = null,
public val createDisposition: Output? = null,
public val defaultDataset: Output? = null,
public val destinationEncryptionConfiguration: Output? = null,
public val destinationTable: Output? = null,
public val flattenResults: Output? = null,
public val maximumBillingTier: Output? = null,
public val maximumBytesBilled: Output? = null,
public val parameterMode: Output? = null,
public val priority: Output? = null,
public val query: Output,
public val schemaUpdateOptions: Output>? = null,
public val scriptOptions: Output? = null,
public val useLegacySql: Output? = null,
public val useQueryCache: Output? = null,
public val userDefinedFunctionResources: Output>? =
null,
public val writeDisposition: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.JobQueryArgs =
com.pulumi.gcp.bigquery.inputs.JobQueryArgs.builder()
.allowLargeResults(allowLargeResults?.applyValue({ args0 -> args0 }))
.createDisposition(createDisposition?.applyValue({ args0 -> args0 }))
.defaultDataset(defaultDataset?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.destinationEncryptionConfiguration(
destinationEncryptionConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.destinationTable(destinationTable?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.flattenResults(flattenResults?.applyValue({ args0 -> args0 }))
.maximumBillingTier(maximumBillingTier?.applyValue({ args0 -> args0 }))
.maximumBytesBilled(maximumBytesBilled?.applyValue({ args0 -> args0 }))
.parameterMode(parameterMode?.applyValue({ args0 -> args0 }))
.priority(priority?.applyValue({ args0 -> args0 }))
.query(query.applyValue({ args0 -> args0 }))
.schemaUpdateOptions(schemaUpdateOptions?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.scriptOptions(scriptOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.useLegacySql(useLegacySql?.applyValue({ args0 -> args0 }))
.useQueryCache(useQueryCache?.applyValue({ args0 -> args0 }))
.userDefinedFunctionResources(
userDefinedFunctionResources?.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.writeDisposition(writeDisposition?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JobQueryArgs].
*/
@PulumiTagMarker
public class JobQueryArgsBuilder internal constructor() {
private var allowLargeResults: Output? = null
private var createDisposition: Output? = null
private var defaultDataset: Output? = null
private var destinationEncryptionConfiguration:
Output? = null
private var destinationTable: Output? = null
private var flattenResults: Output? = null
private var maximumBillingTier: Output? = null
private var maximumBytesBilled: Output? = null
private var parameterMode: Output? = null
private var priority: Output? = null
private var query: Output? = null
private var schemaUpdateOptions: Output>? = null
private var scriptOptions: Output? = null
private var useLegacySql: Output? = null
private var useQueryCache: Output? = null
private var userDefinedFunctionResources: Output>? =
null
private var writeDisposition: Output? = null
/**
* @param value If true and query uses legacy SQL dialect, allows the query to produce arbitrarily large result tables at a slight cost in performance.
* Requires destinationTable to be set. For standard SQL queries, this flag is ignored and large results are always allowed.
* However, you must still set destinationTable when result size exceeds the allowed maximum response size.
*/
@JvmName("mrahfwfkqusbocci")
public suspend fun allowLargeResults(`value`: Output) {
this.allowLargeResults = value
}
/**
* @param value Specifies whether the job is allowed to create new tables. The following values are supported:
* CREATE_IF_NEEDED: If the table does not exist, BigQuery creates the table.
* CREATE_NEVER: The table must already exist. If it does not, a 'notFound' error is returned in the job result.
* Creation, truncation and append actions occur as one atomic update upon job completion
* Default value is `CREATE_IF_NEEDED`.
* Possible values are: `CREATE_IF_NEEDED`, `CREATE_NEVER`.
*/
@JvmName("qpuuorvnxmjywrve")
public suspend fun createDisposition(`value`: Output) {
this.createDisposition = value
}
/**
* @param value Specifies the default dataset to use for unqualified table names in the query. Note that this does not alter behavior of unqualified dataset names.
* Structure is documented below.
*/
@JvmName("eihklppkeawhpdxj")
public suspend fun defaultDataset(`value`: Output) {
this.defaultDataset = value
}
/**
* @param value Custom encryption configuration (e.g., Cloud KMS keys)
* Structure is documented below.
*/
@JvmName("cpbdruqfvwwipobs")
public suspend fun destinationEncryptionConfiguration(`value`: Output) {
this.destinationEncryptionConfiguration = value
}
/**
* @param value Describes the table where the query results should be stored.
* This property must be set for large results that exceed the maximum response size.
* For queries that produce anonymous (cached) results, this field will be populated by BigQuery.
* Structure is documented below.
*/
@JvmName("aderyknfsdliddyh")
public suspend fun destinationTable(`value`: Output) {
this.destinationTable = value
}
/**
* @param value If true and query uses legacy SQL dialect, flattens all nested and repeated fields in the query results.
* allowLargeResults must be true if this is set to false. For standard SQL queries, this flag is ignored and results are never flattened.
*/
@JvmName("topihsmgjguckugh")
public suspend fun flattenResults(`value`: Output) {
this.flattenResults = value
}
/**
* @param value Limits the billing tier for this job. Queries that have resource usage beyond this tier will fail (without incurring a charge).
* If unspecified, this will be set to your project default.
*/
@JvmName("chsaoivrfuhbqudt")
public suspend fun maximumBillingTier(`value`: Output) {
this.maximumBillingTier = value
}
/**
* @param value Limits the bytes billed for this job. Queries that will have bytes billed beyond this limit will fail (without incurring a charge).
* If unspecified, this will be set to your project default.
*/
@JvmName("jttahhendsmcnfjt")
public suspend fun maximumBytesBilled(`value`: Output) {
this.maximumBytesBilled = value
}
/**
* @param value Standard SQL only. Set to POSITIONAL to use positional (?) query parameters or to NAMED to use named (@myparam) query parameters in this query.
*/
@JvmName("tvpotrqsncbkpbkj")
public suspend fun parameterMode(`value`: Output) {
this.parameterMode = value
}
/**
* @param value Specifies a priority for the query.
* Default value is `INTERACTIVE`.
* Possible values are: `INTERACTIVE`, `BATCH`.
*/
@JvmName("ohedffgmwwjkgqjm")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value SQL query text to execute. The useLegacySql field can be used to indicate whether the query uses legacy SQL or standard SQL.
* *NOTE*: queries containing [DML language](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language)
* (`DELETE`, `UPDATE`, `MERGE`, `INSERT`) must specify `create_disposition = ""` and `write_disposition = ""`.
*/
@JvmName("nqbdvkxchkoyneyt")
public suspend fun query(`value`: Output) {
this.query = value
}
/**
* @param value Allows the schema of the destination table to be updated as a side effect of the query job.
* Schema update options are supported in two cases: when writeDisposition is WRITE_APPEND;
* when writeDisposition is WRITE_TRUNCATE and the destination table is a partition of a table,
* specified by partition decorators. For normal tables, WRITE_TRUNCATE will always overwrite the schema.
* One or more of the following values are specified:
* ALLOW_FIELD_ADDITION: allow adding a nullable field to the schema.
* ALLOW_FIELD_RELAXATION: allow relaxing a required field in the original schema to nullable.
*/
@JvmName("rtbbdfojivibkbsq")
public suspend fun schemaUpdateOptions(`value`: Output>) {
this.schemaUpdateOptions = value
}
@JvmName("wfkxsbbujrwgfjkq")
public suspend fun schemaUpdateOptions(vararg values: Output) {
this.schemaUpdateOptions = Output.all(values.asList())
}
/**
* @param values Allows the schema of the destination table to be updated as a side effect of the query job.
* Schema update options are supported in two cases: when writeDisposition is WRITE_APPEND;
* when writeDisposition is WRITE_TRUNCATE and the destination table is a partition of a table,
* specified by partition decorators. For normal tables, WRITE_TRUNCATE will always overwrite the schema.
* One or more of the following values are specified:
* ALLOW_FIELD_ADDITION: allow adding a nullable field to the schema.
* ALLOW_FIELD_RELAXATION: allow relaxing a required field in the original schema to nullable.
*/
@JvmName("ompieshwvsovafju")
public suspend fun schemaUpdateOptions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy