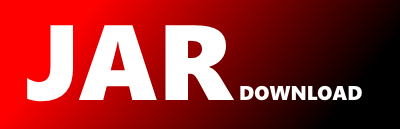
com.pulumi.gcp.bigquery.kotlin.inputs.TableExternalDataConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property autodetect Let BigQuery try to autodetect the schema
* and format of the table.
* @property avroOptions Additional options if `source_format` is set to
* "AVRO". Structure is documented below.
* @property compression The compression type of the data source.
* Valid values are "NONE" or "GZIP".
* @property connectionId The connection specifying the credentials to be used to read
* external storage, such as Azure Blob, Cloud Storage, or S3. The `connection_id` can have
* the form `{{project}}.{{location}}.{{connection_id}}`
* or `projects/{{project}}/locations/{{location}}/connections/{{connection_id}}`.
* ~>**NOTE:** If you set `external_data_configuration.connection_id`, the
* table schema must be specified using the top-level `schema` field
* documented above.
* @property csvOptions Additional properties to set if
* `source_format` is set to "CSV". Structure is documented below.
* @property fileSetSpecType Specifies how source URIs are interpreted for constructing the file set to load.
* By default source URIs are expanded against the underlying storage.
* Other options include specifying manifest files. Only applicable to object storage systems. Docs
* @property googleSheetsOptions Additional options if
* `source_format` is set to "GOOGLE_SHEETS". Structure is
* documented below.
* @property hivePartitioningOptions When set, configures hive partitioning
* support. Not all storage formats support hive partitioning -- requesting hive
* partitioning on an unsupported format will lead to an error, as will providing
* an invalid specification. Structure is documented below.
* @property ignoreUnknownValues Indicates if BigQuery should
* allow extra values that are not represented in the table schema.
* If true, the extra values are ignored. If false, records with
* extra columns are treated as bad records, and if there are too
* many bad records, an invalid error is returned in the job result.
* The default value is false.
* @property jsonExtension Used to indicate that a JSON variant, rather than normal JSON, is being used as the sourceFormat. This should only be used in combination with the `JSON` source format. Valid values are: `GEOJSON`.
* @property jsonOptions Additional properties to set if
* `source_format` is set to "JSON". Structure is documented below.
* @property maxBadRecords The maximum number of bad records that
* BigQuery can ignore when reading data.
* @property metadataCacheMode Metadata Cache Mode for the table. Set this to enable caching of metadata from external data source. Valid values are `AUTOMATIC` and `MANUAL`.
* @property objectMetadata Object Metadata is used to create Object Tables. Object Tables contain a listing of objects (with their metadata) found at the sourceUris. If `object_metadata` is set, `source_format` should be omitted.
* @property parquetOptions Additional properties to set if
* `source_format` is set to "PARQUET". Structure is documented below.
* @property referenceFileSchemaUri When creating an external table, the user can provide a reference file with the table schema. This is enabled for the following formats: AVRO, PARQUET, ORC.
* @property schema A JSON schema for the external table. Schema is required
* for CSV and JSON formats if autodetect is not on. Schema is disallowed
* for Google Cloud Bigtable, Cloud Datastore backups, Avro, Iceberg, ORC and Parquet formats.
* ~>**NOTE:** Because this field expects a JSON string, any changes to the
* string will create a diff, even if the JSON itself hasn't changed.
* Furthermore drift for this field cannot not be detected because BigQuery
* only uses this schema to compute the effective schema for the table, therefore
* any changes on the configured value will force the table to be recreated.
* This schema is effectively only applied when creating a table from an external
* datasource, after creation the computed schema will be stored in
* `google_bigquery_table.schema`
* ~>**NOTE:** If you set `external_data_configuration.connection_id`, the
* table schema must be specified using the top-level `schema` field
* documented above.
* @property sourceFormat The data format. Please see sourceFormat under
* [ExternalDataConfiguration](https://cloud.google.com/bigquery/docs/reference/rest/v2/tables#externaldataconfiguration)
* in Bigquery's public API documentation for supported formats. To use "GOOGLE_SHEETS"
* the `scopes` must include "https://www.googleapis.com/auth/drive.readonly".
* @property sourceUris A list of the fully-qualified URIs that point to
* your data in Google Cloud.
*/
public data class TableExternalDataConfigurationArgs(
public val autodetect: Output,
public val avroOptions: Output? = null,
public val compression: Output? = null,
public val connectionId: Output? = null,
public val csvOptions: Output? = null,
public val fileSetSpecType: Output? = null,
public val googleSheetsOptions: Output? =
null,
public val hivePartitioningOptions: Output? = null,
public val ignoreUnknownValues: Output? = null,
public val jsonExtension: Output? = null,
public val jsonOptions: Output? = null,
public val maxBadRecords: Output? = null,
public val metadataCacheMode: Output? = null,
public val objectMetadata: Output? = null,
public val parquetOptions: Output? = null,
public val referenceFileSchemaUri: Output? = null,
public val schema: Output? = null,
public val sourceFormat: Output? = null,
public val sourceUris: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationArgs =
com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationArgs.builder()
.autodetect(autodetect.applyValue({ args0 -> args0 }))
.avroOptions(avroOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.compression(compression?.applyValue({ args0 -> args0 }))
.connectionId(connectionId?.applyValue({ args0 -> args0 }))
.csvOptions(csvOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fileSetSpecType(fileSetSpecType?.applyValue({ args0 -> args0 }))
.googleSheetsOptions(
googleSheetsOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.hivePartitioningOptions(
hivePartitioningOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.ignoreUnknownValues(ignoreUnknownValues?.applyValue({ args0 -> args0 }))
.jsonExtension(jsonExtension?.applyValue({ args0 -> args0 }))
.jsonOptions(jsonOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maxBadRecords(maxBadRecords?.applyValue({ args0 -> args0 }))
.metadataCacheMode(metadataCacheMode?.applyValue({ args0 -> args0 }))
.objectMetadata(objectMetadata?.applyValue({ args0 -> args0 }))
.parquetOptions(parquetOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.referenceFileSchemaUri(referenceFileSchemaUri?.applyValue({ args0 -> args0 }))
.schema(schema?.applyValue({ args0 -> args0 }))
.sourceFormat(sourceFormat?.applyValue({ args0 -> args0 }))
.sourceUris(sourceUris.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [TableExternalDataConfigurationArgs].
*/
@PulumiTagMarker
public class TableExternalDataConfigurationArgsBuilder internal constructor() {
private var autodetect: Output? = null
private var avroOptions: Output? = null
private var compression: Output? = null
private var connectionId: Output? = null
private var csvOptions: Output? = null
private var fileSetSpecType: Output? = null
private var googleSheetsOptions: Output? =
null
private var hivePartitioningOptions:
Output? = null
private var ignoreUnknownValues: Output? = null
private var jsonExtension: Output? = null
private var jsonOptions: Output? = null
private var maxBadRecords: Output? = null
private var metadataCacheMode: Output? = null
private var objectMetadata: Output? = null
private var parquetOptions: Output? = null
private var referenceFileSchemaUri: Output? = null
private var schema: Output? = null
private var sourceFormat: Output? = null
private var sourceUris: Output>? = null
/**
* @param value Let BigQuery try to autodetect the schema
* and format of the table.
*/
@JvmName("tqjcexdhyklobfpv")
public suspend fun autodetect(`value`: Output) {
this.autodetect = value
}
/**
* @param value Additional options if `source_format` is set to
* "AVRO". Structure is documented below.
*/
@JvmName("evertddxjbnbyvni")
public suspend fun avroOptions(`value`: Output) {
this.avroOptions = value
}
/**
* @param value The compression type of the data source.
* Valid values are "NONE" or "GZIP".
*/
@JvmName("fhwkfpurupgcjjwp")
public suspend fun compression(`value`: Output) {
this.compression = value
}
/**
* @param value The connection specifying the credentials to be used to read
* external storage, such as Azure Blob, Cloud Storage, or S3. The `connection_id` can have
* the form `{{project}}.{{location}}.{{connection_id}}`
* or `projects/{{project}}/locations/{{location}}/connections/{{connection_id}}`.
* ~>**NOTE:** If you set `external_data_configuration.connection_id`, the
* table schema must be specified using the top-level `schema` field
* documented above.
*/
@JvmName("vnhatgjpiuwjitrs")
public suspend fun connectionId(`value`: Output) {
this.connectionId = value
}
/**
* @param value Additional properties to set if
* `source_format` is set to "CSV". Structure is documented below.
*/
@JvmName("ilfcvhtdvbbmgouj")
public suspend fun csvOptions(`value`: Output) {
this.csvOptions = value
}
/**
* @param value Specifies how source URIs are interpreted for constructing the file set to load.
* By default source URIs are expanded against the underlying storage.
* Other options include specifying manifest files. Only applicable to object storage systems. Docs
*/
@JvmName("wtymhexeexnitfkv")
public suspend fun fileSetSpecType(`value`: Output) {
this.fileSetSpecType = value
}
/**
* @param value Additional options if
* `source_format` is set to "GOOGLE_SHEETS". Structure is
* documented below.
*/
@JvmName("ajpmjthjemdwyfqa")
public suspend fun googleSheetsOptions(`value`: Output) {
this.googleSheetsOptions = value
}
/**
* @param value When set, configures hive partitioning
* support. Not all storage formats support hive partitioning -- requesting hive
* partitioning on an unsupported format will lead to an error, as will providing
* an invalid specification. Structure is documented below.
*/
@JvmName("qpkjanpuumdvunny")
public suspend fun hivePartitioningOptions(`value`: Output) {
this.hivePartitioningOptions = value
}
/**
* @param value Indicates if BigQuery should
* allow extra values that are not represented in the table schema.
* If true, the extra values are ignored. If false, records with
* extra columns are treated as bad records, and if there are too
* many bad records, an invalid error is returned in the job result.
* The default value is false.
*/
@JvmName("orjndwviwarottsw")
public suspend fun ignoreUnknownValues(`value`: Output) {
this.ignoreUnknownValues = value
}
/**
* @param value Used to indicate that a JSON variant, rather than normal JSON, is being used as the sourceFormat. This should only be used in combination with the `JSON` source format. Valid values are: `GEOJSON`.
*/
@JvmName("uepnlghcjocrijcf")
public suspend fun jsonExtension(`value`: Output) {
this.jsonExtension = value
}
/**
* @param value Additional properties to set if
* `source_format` is set to "JSON". Structure is documented below.
*/
@JvmName("kvjsdmcwnudvdktf")
public suspend fun jsonOptions(`value`: Output) {
this.jsonOptions = value
}
/**
* @param value The maximum number of bad records that
* BigQuery can ignore when reading data.
*/
@JvmName("ibfuceovkcfjpxyi")
public suspend fun maxBadRecords(`value`: Output) {
this.maxBadRecords = value
}
/**
* @param value Metadata Cache Mode for the table. Set this to enable caching of metadata from external data source. Valid values are `AUTOMATIC` and `MANUAL`.
*/
@JvmName("tabljuulcslqqash")
public suspend fun metadataCacheMode(`value`: Output) {
this.metadataCacheMode = value
}
/**
* @param value Object Metadata is used to create Object Tables. Object Tables contain a listing of objects (with their metadata) found at the sourceUris. If `object_metadata` is set, `source_format` should be omitted.
*/
@JvmName("tyvaahkliodikmss")
public suspend fun objectMetadata(`value`: Output) {
this.objectMetadata = value
}
/**
* @param value Additional properties to set if
* `source_format` is set to "PARQUET". Structure is documented below.
*/
@JvmName("umdhrqsfoygvqqvb")
public suspend fun parquetOptions(`value`: Output) {
this.parquetOptions = value
}
/**
* @param value When creating an external table, the user can provide a reference file with the table schema. This is enabled for the following formats: AVRO, PARQUET, ORC.
*/
@JvmName("eilerxvnxwcdgtle")
public suspend fun referenceFileSchemaUri(`value`: Output) {
this.referenceFileSchemaUri = value
}
/**
* @param value A JSON schema for the external table. Schema is required
* for CSV and JSON formats if autodetect is not on. Schema is disallowed
* for Google Cloud Bigtable, Cloud Datastore backups, Avro, Iceberg, ORC and Parquet formats.
* ~>**NOTE:** Because this field expects a JSON string, any changes to the
* string will create a diff, even if the JSON itself hasn't changed.
* Furthermore drift for this field cannot not be detected because BigQuery
* only uses this schema to compute the effective schema for the table, therefore
* any changes on the configured value will force the table to be recreated.
* This schema is effectively only applied when creating a table from an external
* datasource, after creation the computed schema will be stored in
* `google_bigquery_table.schema`
* ~>**NOTE:** If you set `external_data_configuration.connection_id`, the
* table schema must be specified using the top-level `schema` field
* documented above.
*/
@JvmName("ngfhkvjbktifmjye")
public suspend fun schema(`value`: Output) {
this.schema = value
}
/**
* @param value The data format. Please see sourceFormat under
* [ExternalDataConfiguration](https://cloud.google.com/bigquery/docs/reference/rest/v2/tables#externaldataconfiguration)
* in Bigquery's public API documentation for supported formats. To use "GOOGLE_SHEETS"
* the `scopes` must include "https://www.googleapis.com/auth/drive.readonly".
*/
@JvmName("wdnjqipqoeynvcil")
public suspend fun sourceFormat(`value`: Output) {
this.sourceFormat = value
}
/**
* @param value A list of the fully-qualified URIs that point to
* your data in Google Cloud.
*/
@JvmName("mqfipoijgghhxhmt")
public suspend fun sourceUris(`value`: Output>) {
this.sourceUris = value
}
@JvmName("tcjitghybglwfkfc")
public suspend fun sourceUris(vararg values: Output) {
this.sourceUris = Output.all(values.asList())
}
/**
* @param values A list of the fully-qualified URIs that point to
* your data in Google Cloud.
*/
@JvmName("cenncxejheulwwdp")
public suspend fun sourceUris(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy