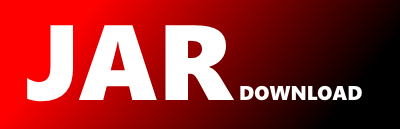
com.pulumi.gcp.bigquery.kotlin.inputs.TableExternalDataConfigurationCsvOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationCsvOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property allowJaggedRows Indicates if BigQuery should accept rows
* that are missing trailing optional columns.
* @property allowQuotedNewlines Indicates if BigQuery should allow
* quoted data sections that contain newline characters in a CSV file.
* The default value is false.
* @property encoding The character encoding of the data. The supported
* values are UTF-8 or ISO-8859-1.
* @property fieldDelimiter The separator for fields in a CSV file.
* @property quote The value that is used to quote data sections in a
* CSV file. If your data does not contain quoted sections, set the
* property value to an empty string. If your data contains quoted newline
* characters, you must also set the `allow_quoted_newlines` property to true.
* The API-side default is `"`, specified in the provider escaped as `\"`. Due to
* limitations with default values, this value is required to be
* explicitly set.
* @property skipLeadingRows The number of rows at the top of a CSV
* file that BigQuery will skip when reading the data.
*/
public data class TableExternalDataConfigurationCsvOptionsArgs(
public val allowJaggedRows: Output? = null,
public val allowQuotedNewlines: Output? = null,
public val encoding: Output? = null,
public val fieldDelimiter: Output? = null,
public val quote: Output,
public val skipLeadingRows: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationCsvOptionsArgs = com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationCsvOptionsArgs.builder()
.allowJaggedRows(allowJaggedRows?.applyValue({ args0 -> args0 }))
.allowQuotedNewlines(allowQuotedNewlines?.applyValue({ args0 -> args0 }))
.encoding(encoding?.applyValue({ args0 -> args0 }))
.fieldDelimiter(fieldDelimiter?.applyValue({ args0 -> args0 }))
.quote(quote.applyValue({ args0 -> args0 }))
.skipLeadingRows(skipLeadingRows?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TableExternalDataConfigurationCsvOptionsArgs].
*/
@PulumiTagMarker
public class TableExternalDataConfigurationCsvOptionsArgsBuilder internal constructor() {
private var allowJaggedRows: Output? = null
private var allowQuotedNewlines: Output? = null
private var encoding: Output? = null
private var fieldDelimiter: Output? = null
private var quote: Output? = null
private var skipLeadingRows: Output? = null
/**
* @param value Indicates if BigQuery should accept rows
* that are missing trailing optional columns.
*/
@JvmName("ochmysiosojismfy")
public suspend fun allowJaggedRows(`value`: Output) {
this.allowJaggedRows = value
}
/**
* @param value Indicates if BigQuery should allow
* quoted data sections that contain newline characters in a CSV file.
* The default value is false.
*/
@JvmName("nmpfxrpqcbjigfey")
public suspend fun allowQuotedNewlines(`value`: Output) {
this.allowQuotedNewlines = value
}
/**
* @param value The character encoding of the data. The supported
* values are UTF-8 or ISO-8859-1.
*/
@JvmName("spdmsvnjoepujldw")
public suspend fun encoding(`value`: Output) {
this.encoding = value
}
/**
* @param value The separator for fields in a CSV file.
*/
@JvmName("wunkpweyhnlsrwim")
public suspend fun fieldDelimiter(`value`: Output) {
this.fieldDelimiter = value
}
/**
* @param value The value that is used to quote data sections in a
* CSV file. If your data does not contain quoted sections, set the
* property value to an empty string. If your data contains quoted newline
* characters, you must also set the `allow_quoted_newlines` property to true.
* The API-side default is `"`, specified in the provider escaped as `\"`. Due to
* limitations with default values, this value is required to be
* explicitly set.
*/
@JvmName("fmdleampotvbmmgt")
public suspend fun quote(`value`: Output) {
this.quote = value
}
/**
* @param value The number of rows at the top of a CSV
* file that BigQuery will skip when reading the data.
*/
@JvmName("fwhtsmsbmjopunas")
public suspend fun skipLeadingRows(`value`: Output) {
this.skipLeadingRows = value
}
/**
* @param value Indicates if BigQuery should accept rows
* that are missing trailing optional columns.
*/
@JvmName("gvjfskcfsuhnndtc")
public suspend fun allowJaggedRows(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowJaggedRows = mapped
}
/**
* @param value Indicates if BigQuery should allow
* quoted data sections that contain newline characters in a CSV file.
* The default value is false.
*/
@JvmName("hxodtggfctqtsoej")
public suspend fun allowQuotedNewlines(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowQuotedNewlines = mapped
}
/**
* @param value The character encoding of the data. The supported
* values are UTF-8 or ISO-8859-1.
*/
@JvmName("kjavrgclcvfqrvhw")
public suspend fun encoding(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encoding = mapped
}
/**
* @param value The separator for fields in a CSV file.
*/
@JvmName("wpxhubalrhusjhew")
public suspend fun fieldDelimiter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldDelimiter = mapped
}
/**
* @param value The value that is used to quote data sections in a
* CSV file. If your data does not contain quoted sections, set the
* property value to an empty string. If your data contains quoted newline
* characters, you must also set the `allow_quoted_newlines` property to true.
* The API-side default is `"`, specified in the provider escaped as `\"`. Due to
* limitations with default values, this value is required to be
* explicitly set.
*/
@JvmName("wvhiifvrogubteow")
public suspend fun quote(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.quote = mapped
}
/**
* @param value The number of rows at the top of a CSV
* file that BigQuery will skip when reading the data.
*/
@JvmName("ytvgoetunnsrjbtw")
public suspend fun skipLeadingRows(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skipLeadingRows = mapped
}
internal fun build(): TableExternalDataConfigurationCsvOptionsArgs =
TableExternalDataConfigurationCsvOptionsArgs(
allowJaggedRows = allowJaggedRows,
allowQuotedNewlines = allowQuotedNewlines,
encoding = encoding,
fieldDelimiter = fieldDelimiter,
quote = quote ?: throw PulumiNullFieldException("quote"),
skipLeadingRows = skipLeadingRows,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy