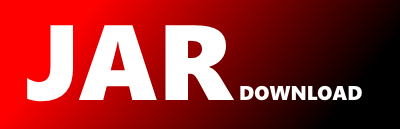
com.pulumi.gcp.bigquery.kotlin.inputs.TableExternalDataConfigurationHivePartitioningOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationHivePartitioningOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property mode When set, what mode of hive partitioning to use when
* reading data. The following modes are supported.
* * AUTO: automatically infer partition key name(s) and type(s).
* * STRINGS: automatically infer partition key name(s). All types are
* Not all storage formats support hive partitioning. Requesting hive
* partitioning on an unsupported format will lead to an error.
* Currently supported formats are: JSON, CSV, ORC, Avro and Parquet.
* * CUSTOM: when set to `CUSTOM`, you must encode the partition key schema within the `source_uri_prefix` by setting `source_uri_prefix` to `gs://bucket/path_to_table/{key1:TYPE1}/{key2:TYPE2}/{key3:TYPE3}`.
* @property requirePartitionFilter If set to true, queries over this table
* require a partition filter that can be used for partition elimination to be
* specified.
* @property sourceUriPrefix When hive partition detection is requested,
* a common for all source uris must be required. The prefix must end immediately
* before the partition key encoding begins. For example, consider files following
* this data layout. `gs://bucket/path_to_table/dt=2019-06-01/country=USA/id=7/file.avro`
* `gs://bucket/path_to_table/dt=2019-05-31/country=CA/id=3/file.avro` When hive
* partitioning is requested with either AUTO or STRINGS detection, the common prefix
* can be either of `gs://bucket/path_to_table` or `gs://bucket/path_to_table/`.
* Note that when `mode` is set to `CUSTOM`, you must encode the partition key schema within the `source_uri_prefix` by setting `source_uri_prefix` to `gs://bucket/path_to_table/{key1:TYPE1}/{key2:TYPE2}/{key3:TYPE3}`.
*/
public data class TableExternalDataConfigurationHivePartitioningOptionsArgs(
public val mode: Output? = null,
public val requirePartitionFilter: Output? = null,
public val sourceUriPrefix: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationHivePartitioningOptionsArgs =
com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationHivePartitioningOptionsArgs.builder()
.mode(mode?.applyValue({ args0 -> args0 }))
.requirePartitionFilter(requirePartitionFilter?.applyValue({ args0 -> args0 }))
.sourceUriPrefix(sourceUriPrefix?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TableExternalDataConfigurationHivePartitioningOptionsArgs].
*/
@PulumiTagMarker
public class TableExternalDataConfigurationHivePartitioningOptionsArgsBuilder internal constructor() {
private var mode: Output? = null
private var requirePartitionFilter: Output? = null
private var sourceUriPrefix: Output? = null
/**
* @param value When set, what mode of hive partitioning to use when
* reading data. The following modes are supported.
* * AUTO: automatically infer partition key name(s) and type(s).
* * STRINGS: automatically infer partition key name(s). All types are
* Not all storage formats support hive partitioning. Requesting hive
* partitioning on an unsupported format will lead to an error.
* Currently supported formats are: JSON, CSV, ORC, Avro and Parquet.
* * CUSTOM: when set to `CUSTOM`, you must encode the partition key schema within the `source_uri_prefix` by setting `source_uri_prefix` to `gs://bucket/path_to_table/{key1:TYPE1}/{key2:TYPE2}/{key3:TYPE3}`.
*/
@JvmName("yrgkhhewuhgjtvwk")
public suspend fun mode(`value`: Output) {
this.mode = value
}
/**
* @param value If set to true, queries over this table
* require a partition filter that can be used for partition elimination to be
* specified.
*/
@JvmName("bcyubxcbhxkudnek")
public suspend fun requirePartitionFilter(`value`: Output) {
this.requirePartitionFilter = value
}
/**
* @param value When hive partition detection is requested,
* a common for all source uris must be required. The prefix must end immediately
* before the partition key encoding begins. For example, consider files following
* this data layout. `gs://bucket/path_to_table/dt=2019-06-01/country=USA/id=7/file.avro`
* `gs://bucket/path_to_table/dt=2019-05-31/country=CA/id=3/file.avro` When hive
* partitioning is requested with either AUTO or STRINGS detection, the common prefix
* can be either of `gs://bucket/path_to_table` or `gs://bucket/path_to_table/`.
* Note that when `mode` is set to `CUSTOM`, you must encode the partition key schema within the `source_uri_prefix` by setting `source_uri_prefix` to `gs://bucket/path_to_table/{key1:TYPE1}/{key2:TYPE2}/{key3:TYPE3}`.
*/
@JvmName("tchrtitfixxutcgw")
public suspend fun sourceUriPrefix(`value`: Output) {
this.sourceUriPrefix = value
}
/**
* @param value When set, what mode of hive partitioning to use when
* reading data. The following modes are supported.
* * AUTO: automatically infer partition key name(s) and type(s).
* * STRINGS: automatically infer partition key name(s). All types are
* Not all storage formats support hive partitioning. Requesting hive
* partitioning on an unsupported format will lead to an error.
* Currently supported formats are: JSON, CSV, ORC, Avro and Parquet.
* * CUSTOM: when set to `CUSTOM`, you must encode the partition key schema within the `source_uri_prefix` by setting `source_uri_prefix` to `gs://bucket/path_to_table/{key1:TYPE1}/{key2:TYPE2}/{key3:TYPE3}`.
*/
@JvmName("iifiltwuvjxmgcin")
public suspend fun mode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mode = mapped
}
/**
* @param value If set to true, queries over this table
* require a partition filter that can be used for partition elimination to be
* specified.
*/
@JvmName("vpuoilfiyeinuoai")
public suspend fun requirePartitionFilter(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requirePartitionFilter = mapped
}
/**
* @param value When hive partition detection is requested,
* a common for all source uris must be required. The prefix must end immediately
* before the partition key encoding begins. For example, consider files following
* this data layout. `gs://bucket/path_to_table/dt=2019-06-01/country=USA/id=7/file.avro`
* `gs://bucket/path_to_table/dt=2019-05-31/country=CA/id=3/file.avro` When hive
* partitioning is requested with either AUTO or STRINGS detection, the common prefix
* can be either of `gs://bucket/path_to_table` or `gs://bucket/path_to_table/`.
* Note that when `mode` is set to `CUSTOM`, you must encode the partition key schema within the `source_uri_prefix` by setting `source_uri_prefix` to `gs://bucket/path_to_table/{key1:TYPE1}/{key2:TYPE2}/{key3:TYPE3}`.
*/
@JvmName("jsjmndnrqorsigfd")
public suspend fun sourceUriPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceUriPrefix = mapped
}
internal fun build(): TableExternalDataConfigurationHivePartitioningOptionsArgs =
TableExternalDataConfigurationHivePartitioningOptionsArgs(
mode = mode,
requirePartitionFilter = requirePartitionFilter,
sourceUriPrefix = sourceUriPrefix,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy