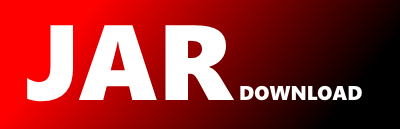
com.pulumi.gcp.bigquery.kotlin.inputs.TableMaterializedViewArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.TableMaterializedViewArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property allowNonIncrementalDefinition Allow non incremental materialized view definition.
* The default value is false.
* @property enableRefresh Specifies whether to use BigQuery's automatic refresh for this materialized view when the base table is updated.
* The default value is true.
* @property query A query whose result is persisted.
* @property refreshIntervalMs The maximum frequency at which this materialized view will be refreshed.
* The default value is 1800000
*/
public data class TableMaterializedViewArgs(
public val allowNonIncrementalDefinition: Output? = null,
public val enableRefresh: Output? = null,
public val query: Output,
public val refreshIntervalMs: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.TableMaterializedViewArgs =
com.pulumi.gcp.bigquery.inputs.TableMaterializedViewArgs.builder()
.allowNonIncrementalDefinition(allowNonIncrementalDefinition?.applyValue({ args0 -> args0 }))
.enableRefresh(enableRefresh?.applyValue({ args0 -> args0 }))
.query(query.applyValue({ args0 -> args0 }))
.refreshIntervalMs(refreshIntervalMs?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TableMaterializedViewArgs].
*/
@PulumiTagMarker
public class TableMaterializedViewArgsBuilder internal constructor() {
private var allowNonIncrementalDefinition: Output? = null
private var enableRefresh: Output? = null
private var query: Output? = null
private var refreshIntervalMs: Output? = null
/**
* @param value Allow non incremental materialized view definition.
* The default value is false.
*/
@JvmName("ovjgpenwahfemdtw")
public suspend fun allowNonIncrementalDefinition(`value`: Output) {
this.allowNonIncrementalDefinition = value
}
/**
* @param value Specifies whether to use BigQuery's automatic refresh for this materialized view when the base table is updated.
* The default value is true.
*/
@JvmName("hqcshnyyhujwttir")
public suspend fun enableRefresh(`value`: Output) {
this.enableRefresh = value
}
/**
* @param value A query whose result is persisted.
*/
@JvmName("pltkmjkuiowmpbfu")
public suspend fun query(`value`: Output) {
this.query = value
}
/**
* @param value The maximum frequency at which this materialized view will be refreshed.
* The default value is 1800000
*/
@JvmName("sbwvtekgfwpfvyob")
public suspend fun refreshIntervalMs(`value`: Output) {
this.refreshIntervalMs = value
}
/**
* @param value Allow non incremental materialized view definition.
* The default value is false.
*/
@JvmName("ilravcljuwyenrmx")
public suspend fun allowNonIncrementalDefinition(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowNonIncrementalDefinition = mapped
}
/**
* @param value Specifies whether to use BigQuery's automatic refresh for this materialized view when the base table is updated.
* The default value is true.
*/
@JvmName("ulavjnqspilgitxp")
public suspend fun enableRefresh(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableRefresh = mapped
}
/**
* @param value A query whose result is persisted.
*/
@JvmName("nqtaudvppgoornvo")
public suspend fun query(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.query = mapped
}
/**
* @param value The maximum frequency at which this materialized view will be refreshed.
* The default value is 1800000
*/
@JvmName("qhvuunoiyrkncwyt")
public suspend fun refreshIntervalMs(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.refreshIntervalMs = mapped
}
internal fun build(): TableMaterializedViewArgs = TableMaterializedViewArgs(
allowNonIncrementalDefinition = allowNonIncrementalDefinition,
enableRefresh = enableRefresh,
query = query ?: throw PulumiNullFieldException("query"),
refreshIntervalMs = refreshIntervalMs,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy