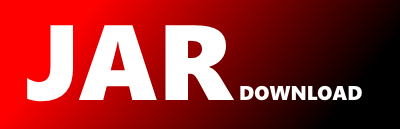
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property autodetect Let BigQuery try to autodetect the schema
* and format of the table.
* @property avroOptions Additional options if `source_format` is set to
* "AVRO". Structure is documented below.
* @property compression The compression type of the data source.
* Valid values are "NONE" or "GZIP".
* @property connectionId The connection specifying the credentials to be used to read
* external storage, such as Azure Blob, Cloud Storage, or S3. The `connection_id` can have
* the form `{{project}}.{{location}}.{{connection_id}}`
* or `projects/{{project}}/locations/{{location}}/connections/{{connection_id}}`.
* ~>**NOTE:** If you set `external_data_configuration.connection_id`, the
* table schema must be specified using the top-level `schema` field
* documented above.
* @property csvOptions Additional properties to set if
* `source_format` is set to "CSV". Structure is documented below.
* @property fileSetSpecType Specifies how source URIs are interpreted for constructing the file set to load.
* By default source URIs are expanded against the underlying storage.
* Other options include specifying manifest files. Only applicable to object storage systems. Docs
* @property googleSheetsOptions Additional options if
* `source_format` is set to "GOOGLE_SHEETS". Structure is
* documented below.
* @property hivePartitioningOptions When set, configures hive partitioning
* support. Not all storage formats support hive partitioning -- requesting hive
* partitioning on an unsupported format will lead to an error, as will providing
* an invalid specification. Structure is documented below.
* @property ignoreUnknownValues Indicates if BigQuery should
* allow extra values that are not represented in the table schema.
* If true, the extra values are ignored. If false, records with
* extra columns are treated as bad records, and if there are too
* many bad records, an invalid error is returned in the job result.
* The default value is false.
* @property jsonExtension Used to indicate that a JSON variant, rather than normal JSON, is being used as the sourceFormat. This should only be used in combination with the `JSON` source format. Valid values are: `GEOJSON`.
* @property jsonOptions Additional properties to set if
* `source_format` is set to "JSON". Structure is documented below.
* @property maxBadRecords The maximum number of bad records that
* BigQuery can ignore when reading data.
* @property metadataCacheMode Metadata Cache Mode for the table. Set this to enable caching of metadata from external data source. Valid values are `AUTOMATIC` and `MANUAL`.
* @property objectMetadata Object Metadata is used to create Object Tables. Object Tables contain a listing of objects (with their metadata) found at the sourceUris. If `object_metadata` is set, `source_format` should be omitted.
* @property parquetOptions Additional properties to set if
* `source_format` is set to "PARQUET". Structure is documented below.
* @property referenceFileSchemaUri When creating an external table, the user can provide a reference file with the table schema. This is enabled for the following formats: AVRO, PARQUET, ORC.
* @property schema A JSON schema for the external table. Schema is required
* for CSV and JSON formats if autodetect is not on. Schema is disallowed
* for Google Cloud Bigtable, Cloud Datastore backups, Avro, Iceberg, ORC and Parquet formats.
* ~>**NOTE:** Because this field expects a JSON string, any changes to the
* string will create a diff, even if the JSON itself hasn't changed.
* Furthermore drift for this field cannot not be detected because BigQuery
* only uses this schema to compute the effective schema for the table, therefore
* any changes on the configured value will force the table to be recreated.
* This schema is effectively only applied when creating a table from an external
* datasource, after creation the computed schema will be stored in
* `google_bigquery_table.schema`
* ~>**NOTE:** If you set `external_data_configuration.connection_id`, the
* table schema must be specified using the top-level `schema` field
* documented above.
* @property sourceFormat The data format. Please see sourceFormat under
* [ExternalDataConfiguration](https://cloud.google.com/bigquery/docs/reference/rest/v2/tables#externaldataconfiguration)
* in Bigquery's public API documentation for supported formats. To use "GOOGLE_SHEETS"
* the `scopes` must include "https://www.googleapis.com/auth/drive.readonly".
* @property sourceUris A list of the fully-qualified URIs that point to
* your data in Google Cloud.
*/
public data class TableExternalDataConfiguration(
public val autodetect: Boolean,
public val avroOptions: TableExternalDataConfigurationAvroOptions? = null,
public val compression: String? = null,
public val connectionId: String? = null,
public val csvOptions: TableExternalDataConfigurationCsvOptions? = null,
public val fileSetSpecType: String? = null,
public val googleSheetsOptions: TableExternalDataConfigurationGoogleSheetsOptions? = null,
public val hivePartitioningOptions: TableExternalDataConfigurationHivePartitioningOptions? = null,
public val ignoreUnknownValues: Boolean? = null,
public val jsonExtension: String? = null,
public val jsonOptions: TableExternalDataConfigurationJsonOptions? = null,
public val maxBadRecords: Int? = null,
public val metadataCacheMode: String? = null,
public val objectMetadata: String? = null,
public val parquetOptions: TableExternalDataConfigurationParquetOptions? = null,
public val referenceFileSchemaUri: String? = null,
public val schema: String? = null,
public val sourceFormat: String? = null,
public val sourceUris: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.bigquery.outputs.TableExternalDataConfiguration): TableExternalDataConfiguration = TableExternalDataConfiguration(
autodetect = javaType.autodetect(),
avroOptions = javaType.avroOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfigurationAvroOptions.Companion.toKotlin(args0)
})
}).orElse(null),
compression = javaType.compression().map({ args0 -> args0 }).orElse(null),
connectionId = javaType.connectionId().map({ args0 -> args0 }).orElse(null),
csvOptions = javaType.csvOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfigurationCsvOptions.Companion.toKotlin(args0)
})
}).orElse(null),
fileSetSpecType = javaType.fileSetSpecType().map({ args0 -> args0 }).orElse(null),
googleSheetsOptions = javaType.googleSheetsOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfigurationGoogleSheetsOptions.Companion.toKotlin(args0)
})
}).orElse(null),
hivePartitioningOptions = javaType.hivePartitioningOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfigurationHivePartitioningOptions.Companion.toKotlin(args0)
})
}).orElse(null),
ignoreUnknownValues = javaType.ignoreUnknownValues().map({ args0 -> args0 }).orElse(null),
jsonExtension = javaType.jsonExtension().map({ args0 -> args0 }).orElse(null),
jsonOptions = javaType.jsonOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfigurationJsonOptions.Companion.toKotlin(args0)
})
}).orElse(null),
maxBadRecords = javaType.maxBadRecords().map({ args0 -> args0 }).orElse(null),
metadataCacheMode = javaType.metadataCacheMode().map({ args0 -> args0 }).orElse(null),
objectMetadata = javaType.objectMetadata().map({ args0 -> args0 }).orElse(null),
parquetOptions = javaType.parquetOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.bigquery.kotlin.outputs.TableExternalDataConfigurationParquetOptions.Companion.toKotlin(args0)
})
}).orElse(null),
referenceFileSchemaUri = javaType.referenceFileSchemaUri().map({ args0 -> args0 }).orElse(null),
schema = javaType.schema().map({ args0 -> args0 }).orElse(null),
sourceFormat = javaType.sourceFormat().map({ args0 -> args0 }).orElse(null),
sourceUris = javaType.sourceUris().map({ args0 -> args0 }),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy