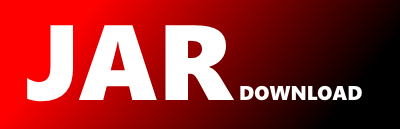
com.pulumi.gcp.bigtable.kotlin.AuthorizedViewArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigtable.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigtable.AuthorizedViewArgs.builder
import com.pulumi.gcp.bigtable.kotlin.inputs.AuthorizedViewSubsetViewArgs
import com.pulumi.gcp.bigtable.kotlin.inputs.AuthorizedViewSubsetViewArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ## Import
* Bigtable Authorized Views can be imported using any of these accepted formats:
* * `projects/{{project}}/instances/{{instance_name}}/tables/{{table_name}}/authorizedViews/{{name}}`
* * `{{project}}/{{instance_name}}/{{table_name}}/{{name}}`
* * `{{instance_name}}/{{table_name}}/{{name}}`
* When using the `pulumi import` command, Bigtable Authorized Views can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:bigtable/authorizedView:AuthorizedView default projects/{{project}}/instances/{{instance_name}}/tables/{{table_name}}/authorizedViews/{{name}}
* ```
* ```sh
* $ pulumi import gcp:bigtable/authorizedView:AuthorizedView default {{project}}/{{instance_name}}/{{table_name}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:bigtable/authorizedView:AuthorizedView default {{instance_name}}/{{table_name}}/{{name}}
* ```
* @property deletionProtection
* @property instanceName The name of the Bigtable instance in which the authorized view belongs.
* @property name The name of the authorized view. Must be 1-50 characters and must only contain hyphens, underscores, periods, letters and numbers.
* @property project The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
* @property subsetView An AuthorizedView permitting access to an explicit subset of a Table. Structure is documented below.
* -----
* @property tableName The name of the Bigtable table in which the authorized view belongs.
*/
public data class AuthorizedViewArgs(
public val deletionProtection: Output? = null,
public val instanceName: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val subsetView: Output? = null,
public val tableName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigtable.AuthorizedViewArgs =
com.pulumi.gcp.bigtable.AuthorizedViewArgs.builder()
.deletionProtection(deletionProtection?.applyValue({ args0 -> args0 }))
.instanceName(instanceName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.subsetView(subsetView?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tableName(tableName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AuthorizedViewArgs].
*/
@PulumiTagMarker
public class AuthorizedViewArgsBuilder internal constructor() {
private var deletionProtection: Output? = null
private var instanceName: Output? = null
private var name: Output? = null
private var project: Output? = null
private var subsetView: Output? = null
private var tableName: Output? = null
/**
* @param value
*/
@JvmName("llwiayjxmgcrjsjp")
public suspend fun deletionProtection(`value`: Output) {
this.deletionProtection = value
}
/**
* @param value The name of the Bigtable instance in which the authorized view belongs.
*/
@JvmName("uuvsifcvprmkfxpv")
public suspend fun instanceName(`value`: Output) {
this.instanceName = value
}
/**
* @param value The name of the authorized view. Must be 1-50 characters and must only contain hyphens, underscores, periods, letters and numbers.
*/
@JvmName("rtkjcfsjiftqlrje")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
*/
@JvmName("fwiohlphijlncvwf")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value An AuthorizedView permitting access to an explicit subset of a Table. Structure is documented below.
* -----
*/
@JvmName("cmxultahlpxjdamn")
public suspend fun subsetView(`value`: Output) {
this.subsetView = value
}
/**
* @param value The name of the Bigtable table in which the authorized view belongs.
*/
@JvmName("nglctlipvjrqnjuh")
public suspend fun tableName(`value`: Output) {
this.tableName = value
}
/**
* @param value
*/
@JvmName("vrrsclmjriixcegf")
public suspend fun deletionProtection(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deletionProtection = mapped
}
/**
* @param value The name of the Bigtable instance in which the authorized view belongs.
*/
@JvmName("taffytcmgnvkleyu")
public suspend fun instanceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceName = mapped
}
/**
* @param value The name of the authorized view. Must be 1-50 characters and must only contain hyphens, underscores, periods, letters and numbers.
*/
@JvmName("dhqxrhpwuclvfkur")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
*/
@JvmName("pnarkuudsiffkdwm")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value An AuthorizedView permitting access to an explicit subset of a Table. Structure is documented below.
* -----
*/
@JvmName("bpugctlueaplhclx")
public suspend fun subsetView(`value`: AuthorizedViewSubsetViewArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subsetView = mapped
}
/**
* @param argument An AuthorizedView permitting access to an explicit subset of a Table. Structure is documented below.
* -----
*/
@JvmName("oytiwgkjvmwraddq")
public suspend fun subsetView(argument: suspend AuthorizedViewSubsetViewArgsBuilder.() -> Unit) {
val toBeMapped = AuthorizedViewSubsetViewArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.subsetView = mapped
}
/**
* @param value The name of the Bigtable table in which the authorized view belongs.
*/
@JvmName("pvhqdyrmoevncvqi")
public suspend fun tableName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tableName = mapped
}
internal fun build(): AuthorizedViewArgs = AuthorizedViewArgs(
deletionProtection = deletionProtection,
instanceName = instanceName,
name = name,
project = project,
subsetView = subsetView,
tableName = tableName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy