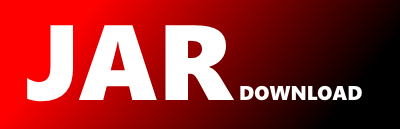
com.pulumi.gcp.billing.kotlin.SubAccount.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.billing.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [SubAccount].
*/
@PulumiTagMarker
public class SubAccountResourceBuilder internal constructor() {
public var name: String? = null
public var args: SubAccountArgs = SubAccountArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SubAccountArgsBuilder.() -> Unit) {
val builder = SubAccountArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SubAccount {
val builtJavaResource = com.pulumi.gcp.billing.SubAccount(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SubAccount(builtJavaResource)
}
}
/**
* Allows creation and management of a Google Cloud Billing Subaccount.
* !> **WARNING:** Deleting this resource will not delete or close the billing subaccount.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const subaccount = new gcp.billing.SubAccount("subaccount", {
* displayName: "My Billing Account",
* masterBillingAccount: "012345-567890-ABCDEF",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* subaccount = gcp.billing.SubAccount("subaccount",
* display_name="My Billing Account",
* master_billing_account="012345-567890-ABCDEF")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var subaccount = new Gcp.Billing.SubAccount("subaccount", new()
* {
* DisplayName = "My Billing Account",
* MasterBillingAccount = "012345-567890-ABCDEF",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/billing"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := billing.NewSubAccount(ctx, "subaccount", &billing.SubAccountArgs{
* DisplayName: pulumi.String("My Billing Account"),
* MasterBillingAccount: pulumi.String("012345-567890-ABCDEF"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.billing.SubAccount;
* import com.pulumi.gcp.billing.SubAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var subaccount = new SubAccount("subaccount", SubAccountArgs.builder()
* .displayName("My Billing Account")
* .masterBillingAccount("012345-567890-ABCDEF")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* subaccount:
* type: gcp:billing:SubAccount
* properties:
* displayName: My Billing Account
* masterBillingAccount: 012345-567890-ABCDEF
* ```
*
* ## Import
* Billing Subaccounts can be imported using any of these accepted formats:
* * `billingAccounts/{billing_account_id}`
* When using the `pulumi import` command, Billing Subaccounts can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:billing/subAccount:SubAccount default billingAccounts/{billing_account_id}
* ```
*/
public class SubAccount internal constructor(
override val javaResource: com.pulumi.gcp.billing.SubAccount,
) : KotlinCustomResource(javaResource, SubAccountMapper) {
/**
* The billing account id.
*/
public val billingAccountId: Output
get() = javaResource.billingAccountId().applyValue({ args0 -> args0 })
/**
* If set to "RENAME_ON_DESTROY" the billing account display_name
* will be changed to "Destroyed" along with a timestamp. If set to "" this will not occur.
* Default is "".
*/
public val deletionPolicy: Output?
get() = javaResource.deletionPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The display name of the billing account.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* The name of the master billing account that the subaccount
* will be created under in the form `{billing_account_id}` or `billingAccounts/{billing_account_id}`.
*/
public val masterBillingAccount: Output
get() = javaResource.masterBillingAccount().applyValue({ args0 -> args0 })
/**
* The resource name of the billing account in the form `billingAccounts/{billing_account_id}`.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* `true` if the billing account is open, `false` if the billing account is closed.
*/
public val `open`: Output
get() = javaResource.`open`().applyValue({ args0 -> args0 })
}
public object SubAccountMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.billing.SubAccount::class == javaResource::class
override fun map(javaResource: Resource): SubAccount = SubAccount(
javaResource as
com.pulumi.gcp.billing.SubAccount,
)
}
/**
* @see [SubAccount].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [SubAccount].
*/
public suspend fun subAccount(name: String, block: suspend SubAccountResourceBuilder.() -> Unit): SubAccount {
val builder = SubAccountResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [SubAccount].
* @param name The _unique_ name of the resulting resource.
*/
public fun subAccount(name: String): SubAccount {
val builder = SubAccountResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy