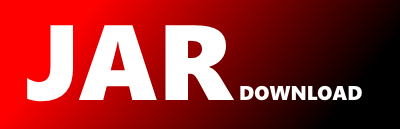
com.pulumi.gcp.billing.kotlin.inputs.BudgetAmountArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.billing.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.billing.inputs.BudgetAmountArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property lastPeriodAmount Configures a budget amount that is automatically set to 100% of
* last period's spend.
* Boolean. Set value to true to use. Do not set to false, instead
* use the `specified_amount` block.
* @property specifiedAmount A specified amount to use as the budget. currencyCode is
* optional. If specified, it must match the currency of the
* billing account. The currencyCode is provided on output.
* Structure is documented below.
*/
public data class BudgetAmountArgs(
public val lastPeriodAmount: Output? = null,
public val specifiedAmount: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.billing.inputs.BudgetAmountArgs =
com.pulumi.gcp.billing.inputs.BudgetAmountArgs.builder()
.lastPeriodAmount(lastPeriodAmount?.applyValue({ args0 -> args0 }))
.specifiedAmount(
specifiedAmount?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BudgetAmountArgs].
*/
@PulumiTagMarker
public class BudgetAmountArgsBuilder internal constructor() {
private var lastPeriodAmount: Output? = null
private var specifiedAmount: Output? = null
/**
* @param value Configures a budget amount that is automatically set to 100% of
* last period's spend.
* Boolean. Set value to true to use. Do not set to false, instead
* use the `specified_amount` block.
*/
@JvmName("fbptufgleljgihci")
public suspend fun lastPeriodAmount(`value`: Output) {
this.lastPeriodAmount = value
}
/**
* @param value A specified amount to use as the budget. currencyCode is
* optional. If specified, it must match the currency of the
* billing account. The currencyCode is provided on output.
* Structure is documented below.
*/
@JvmName("tkielnuvvgcxeuep")
public suspend fun specifiedAmount(`value`: Output) {
this.specifiedAmount = value
}
/**
* @param value Configures a budget amount that is automatically set to 100% of
* last period's spend.
* Boolean. Set value to true to use. Do not set to false, instead
* use the `specified_amount` block.
*/
@JvmName("tsmtbtvotqgjynnx")
public suspend fun lastPeriodAmount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastPeriodAmount = mapped
}
/**
* @param value A specified amount to use as the budget. currencyCode is
* optional. If specified, it must match the currency of the
* billing account. The currencyCode is provided on output.
* Structure is documented below.
*/
@JvmName("sifahyilxodgmxvl")
public suspend fun specifiedAmount(`value`: BudgetAmountSpecifiedAmountArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.specifiedAmount = mapped
}
/**
* @param argument A specified amount to use as the budget. currencyCode is
* optional. If specified, it must match the currency of the
* billing account. The currencyCode is provided on output.
* Structure is documented below.
*/
@JvmName("rspuiyfmdrtjppty")
public suspend fun specifiedAmount(argument: suspend BudgetAmountSpecifiedAmountArgsBuilder.() -> Unit) {
val toBeMapped = BudgetAmountSpecifiedAmountArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.specifiedAmount = mapped
}
internal fun build(): BudgetAmountArgs = BudgetAmountArgs(
lastPeriodAmount = lastPeriodAmount,
specifiedAmount = specifiedAmount,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy