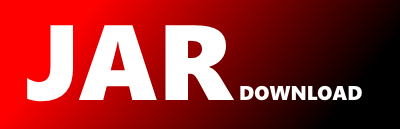
com.pulumi.gcp.binaryauthorization.kotlin.inputs.PolicyDefaultAdmissionRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.binaryauthorization.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.binaryauthorization.inputs.PolicyDefaultAdmissionRuleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property enforcementMode The action when a pod creation is denied by the admission rule.
* Possible values are: `ENFORCED_BLOCK_AND_AUDIT_LOG`, `DRYRUN_AUDIT_LOG_ONLY`.
* - - -
* @property evaluationMode How this admission rule will be evaluated.
* Possible values are: `ALWAYS_ALLOW`, `REQUIRE_ATTESTATION`, `ALWAYS_DENY`.
* @property requireAttestationsBies The resource names of the attestors that must attest to a
* container image. If the attestor is in a different project from the
* policy, it should be specified in the format `projects/*/attestors/*`.
* Each attestor must exist before a policy can reference it. To add an
* attestor to a policy the principal issuing the policy change
* request must be able to read the attestor resource.
* Note: this field must be non-empty when the evaluation_mode field
* specifies REQUIRE_ATTESTATION, otherwise it must be empty.
* */*/
*/
public data class PolicyDefaultAdmissionRuleArgs(
public val enforcementMode: Output,
public val evaluationMode: Output,
public val requireAttestationsBies: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.binaryauthorization.inputs.PolicyDefaultAdmissionRuleArgs =
com.pulumi.gcp.binaryauthorization.inputs.PolicyDefaultAdmissionRuleArgs.builder()
.enforcementMode(enforcementMode.applyValue({ args0 -> args0 }))
.evaluationMode(evaluationMode.applyValue({ args0 -> args0 }))
.requireAttestationsBies(
requireAttestationsBies?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [PolicyDefaultAdmissionRuleArgs].
*/
@PulumiTagMarker
public class PolicyDefaultAdmissionRuleArgsBuilder internal constructor() {
private var enforcementMode: Output? = null
private var evaluationMode: Output? = null
private var requireAttestationsBies: Output>? = null
/**
* @param value The action when a pod creation is denied by the admission rule.
* Possible values are: `ENFORCED_BLOCK_AND_AUDIT_LOG`, `DRYRUN_AUDIT_LOG_ONLY`.
* - - -
*/
@JvmName("npfjkrofwaclewqw")
public suspend fun enforcementMode(`value`: Output) {
this.enforcementMode = value
}
/**
* @param value How this admission rule will be evaluated.
* Possible values are: `ALWAYS_ALLOW`, `REQUIRE_ATTESTATION`, `ALWAYS_DENY`.
*/
@JvmName("ejkemsttellcgnsi")
public suspend fun evaluationMode(`value`: Output) {
this.evaluationMode = value
}
/**
* @param value The resource names of the attestors that must attest to a
* container image. If the attestor is in a different project from the
* policy, it should be specified in the format `projects/*/attestors/*`.
* Each attestor must exist before a policy can reference it. To add an
* attestor to a policy the principal issuing the policy change
* request must be able to read the attestor resource.
* Note: this field must be non-empty when the evaluation_mode field
* specifies REQUIRE_ATTESTATION, otherwise it must be empty.
* */*/
*/
@JvmName("bkgjbeyxralmxhho")
public suspend fun requireAttestationsBies(`value`: Output>) {
this.requireAttestationsBies = value
}
@JvmName("jamaljojnjovjqrm")
public suspend fun requireAttestationsBies(vararg values: Output) {
this.requireAttestationsBies = Output.all(values.asList())
}
/**
* @param values The resource names of the attestors that must attest to a
* container image. If the attestor is in a different project from the
* policy, it should be specified in the format `projects/*/attestors/*`.
* Each attestor must exist before a policy can reference it. To add an
* attestor to a policy the principal issuing the policy change
* request must be able to read the attestor resource.
* Note: this field must be non-empty when the evaluation_mode field
* specifies REQUIRE_ATTESTATION, otherwise it must be empty.
* */*/
*/
@JvmName("nrjxcvsghjocukip")
public suspend fun requireAttestationsBies(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy