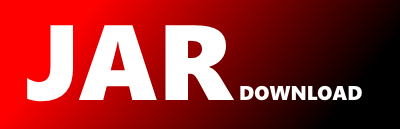
com.pulumi.gcp.certificateauthority.kotlin.inputs.CaPoolIssuancePolicyBaselineValuesCaOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.certificateauthority.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.certificateauthority.inputs.CaPoolIssuancePolicyBaselineValuesCaOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property isCa When true, the "CA" in Basic Constraints extension will be set to true.
* @property maxIssuerPathLength Refers to the "path length constraint" in Basic Constraints extension. For a CA certificate, this value describes the depth of
* subordinate CA certificates that are allowed. If this value is less than 0, the request will fail.
* @property nonCa When true, the "CA" in Basic Constraints extension will be set to false.
* If both `is_ca` and `non_ca` are unset, the extension will be omitted from the CA certificate.
* @property zeroMaxIssuerPathLength When true, the "path length constraint" in Basic Constraints extension will be set to 0.
* if both `max_issuer_path_length` and `zero_max_issuer_path_length` are unset,
* the max path length will be omitted from the CA certificate.
*/
public data class CaPoolIssuancePolicyBaselineValuesCaOptionsArgs(
public val isCa: Output? = null,
public val maxIssuerPathLength: Output? = null,
public val nonCa: Output? = null,
public val zeroMaxIssuerPathLength: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.certificateauthority.inputs.CaPoolIssuancePolicyBaselineValuesCaOptionsArgs =
com.pulumi.gcp.certificateauthority.inputs.CaPoolIssuancePolicyBaselineValuesCaOptionsArgs.builder()
.isCa(isCa?.applyValue({ args0 -> args0 }))
.maxIssuerPathLength(maxIssuerPathLength?.applyValue({ args0 -> args0 }))
.nonCa(nonCa?.applyValue({ args0 -> args0 }))
.zeroMaxIssuerPathLength(zeroMaxIssuerPathLength?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CaPoolIssuancePolicyBaselineValuesCaOptionsArgs].
*/
@PulumiTagMarker
public class CaPoolIssuancePolicyBaselineValuesCaOptionsArgsBuilder internal constructor() {
private var isCa: Output? = null
private var maxIssuerPathLength: Output? = null
private var nonCa: Output? = null
private var zeroMaxIssuerPathLength: Output? = null
/**
* @param value When true, the "CA" in Basic Constraints extension will be set to true.
*/
@JvmName("mumaudhllmukprxs")
public suspend fun isCa(`value`: Output) {
this.isCa = value
}
/**
* @param value Refers to the "path length constraint" in Basic Constraints extension. For a CA certificate, this value describes the depth of
* subordinate CA certificates that are allowed. If this value is less than 0, the request will fail.
*/
@JvmName("baglddedtjsjrdvs")
public suspend fun maxIssuerPathLength(`value`: Output) {
this.maxIssuerPathLength = value
}
/**
* @param value When true, the "CA" in Basic Constraints extension will be set to false.
* If both `is_ca` and `non_ca` are unset, the extension will be omitted from the CA certificate.
*/
@JvmName("ncasowvnnjlwhghe")
public suspend fun nonCa(`value`: Output) {
this.nonCa = value
}
/**
* @param value When true, the "path length constraint" in Basic Constraints extension will be set to 0.
* if both `max_issuer_path_length` and `zero_max_issuer_path_length` are unset,
* the max path length will be omitted from the CA certificate.
*/
@JvmName("macviiijlrirxuyk")
public suspend fun zeroMaxIssuerPathLength(`value`: Output) {
this.zeroMaxIssuerPathLength = value
}
/**
* @param value When true, the "CA" in Basic Constraints extension will be set to true.
*/
@JvmName("usgttvicwwawgvts")
public suspend fun isCa(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isCa = mapped
}
/**
* @param value Refers to the "path length constraint" in Basic Constraints extension. For a CA certificate, this value describes the depth of
* subordinate CA certificates that are allowed. If this value is less than 0, the request will fail.
*/
@JvmName("ttwulcfxkpglaboi")
public suspend fun maxIssuerPathLength(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxIssuerPathLength = mapped
}
/**
* @param value When true, the "CA" in Basic Constraints extension will be set to false.
* If both `is_ca` and `non_ca` are unset, the extension will be omitted from the CA certificate.
*/
@JvmName("tlydqqxcerwwgwny")
public suspend fun nonCa(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nonCa = mapped
}
/**
* @param value When true, the "path length constraint" in Basic Constraints extension will be set to 0.
* if both `max_issuer_path_length` and `zero_max_issuer_path_length` are unset,
* the max path length will be omitted from the CA certificate.
*/
@JvmName("xmywxmyajwofjimy")
public suspend fun zeroMaxIssuerPathLength(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zeroMaxIssuerPathLength = mapped
}
internal fun build(): CaPoolIssuancePolicyBaselineValuesCaOptionsArgs =
CaPoolIssuancePolicyBaselineValuesCaOptionsArgs(
isCa = isCa,
maxIssuerPathLength = maxIssuerPathLength,
nonCa = nonCa,
zeroMaxIssuerPathLength = zeroMaxIssuerPathLength,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy