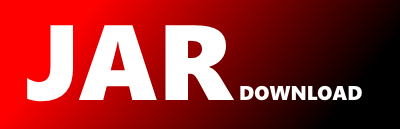
com.pulumi.gcp.certificateauthority.kotlin.inputs.CaPoolIssuancePolicyIdentityConstraintsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.certificateauthority.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.certificateauthority.inputs.CaPoolIssuancePolicyIdentityConstraintsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allowSubjectAltNamesPassthrough If this is set, the SubjectAltNames extension may be copied from a certificate request into the signed certificate.
* Otherwise, the requested SubjectAltNames will be discarded.
* @property allowSubjectPassthrough If this is set, the Subject field may be copied from a certificate request into the signed certificate.
* Otherwise, the requested Subject will be discarded.
* @property celExpression A CEL expression that may be used to validate the resolved X.509 Subject and/or Subject Alternative Name before a
* certificate is signed. To see the full allowed syntax and some examples,
* see https://cloud.google.com/certificate-authority-service/docs/cel-guide
* Structure is documented below.
*/
public data class CaPoolIssuancePolicyIdentityConstraintsArgs(
public val allowSubjectAltNamesPassthrough: Output,
public val allowSubjectPassthrough: Output,
public val celExpression: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.certificateauthority.inputs.CaPoolIssuancePolicyIdentityConstraintsArgs =
com.pulumi.gcp.certificateauthority.inputs.CaPoolIssuancePolicyIdentityConstraintsArgs.builder()
.allowSubjectAltNamesPassthrough(allowSubjectAltNamesPassthrough.applyValue({ args0 -> args0 }))
.allowSubjectPassthrough(allowSubjectPassthrough.applyValue({ args0 -> args0 }))
.celExpression(celExpression?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CaPoolIssuancePolicyIdentityConstraintsArgs].
*/
@PulumiTagMarker
public class CaPoolIssuancePolicyIdentityConstraintsArgsBuilder internal constructor() {
private var allowSubjectAltNamesPassthrough: Output? = null
private var allowSubjectPassthrough: Output? = null
private var celExpression: Output? =
null
/**
* @param value If this is set, the SubjectAltNames extension may be copied from a certificate request into the signed certificate.
* Otherwise, the requested SubjectAltNames will be discarded.
*/
@JvmName("btbqejsyhwgwbfro")
public suspend fun allowSubjectAltNamesPassthrough(`value`: Output) {
this.allowSubjectAltNamesPassthrough = value
}
/**
* @param value If this is set, the Subject field may be copied from a certificate request into the signed certificate.
* Otherwise, the requested Subject will be discarded.
*/
@JvmName("myowvpjgpyknhkgu")
public suspend fun allowSubjectPassthrough(`value`: Output) {
this.allowSubjectPassthrough = value
}
/**
* @param value A CEL expression that may be used to validate the resolved X.509 Subject and/or Subject Alternative Name before a
* certificate is signed. To see the full allowed syntax and some examples,
* see https://cloud.google.com/certificate-authority-service/docs/cel-guide
* Structure is documented below.
*/
@JvmName("cqsekfqwepxodasf")
public suspend fun celExpression(`value`: Output) {
this.celExpression = value
}
/**
* @param value If this is set, the SubjectAltNames extension may be copied from a certificate request into the signed certificate.
* Otherwise, the requested SubjectAltNames will be discarded.
*/
@JvmName("frinexbsrxlsntpr")
public suspend fun allowSubjectAltNamesPassthrough(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.allowSubjectAltNamesPassthrough = mapped
}
/**
* @param value If this is set, the Subject field may be copied from a certificate request into the signed certificate.
* Otherwise, the requested Subject will be discarded.
*/
@JvmName("xexibtaosgmemphp")
public suspend fun allowSubjectPassthrough(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.allowSubjectPassthrough = mapped
}
/**
* @param value A CEL expression that may be used to validate the resolved X.509 Subject and/or Subject Alternative Name before a
* certificate is signed. To see the full allowed syntax and some examples,
* see https://cloud.google.com/certificate-authority-service/docs/cel-guide
* Structure is documented below.
*/
@JvmName("ahgbsjmyuakiamxu")
public suspend fun celExpression(`value`: CaPoolIssuancePolicyIdentityConstraintsCelExpressionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.celExpression = mapped
}
/**
* @param argument A CEL expression that may be used to validate the resolved X.509 Subject and/or Subject Alternative Name before a
* certificate is signed. To see the full allowed syntax and some examples,
* see https://cloud.google.com/certificate-authority-service/docs/cel-guide
* Structure is documented below.
*/
@JvmName("bdqvxvawkathuyod")
public suspend fun celExpression(argument: suspend CaPoolIssuancePolicyIdentityConstraintsCelExpressionArgsBuilder.() -> Unit) {
val toBeMapped = CaPoolIssuancePolicyIdentityConstraintsCelExpressionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.celExpression = mapped
}
internal fun build(): CaPoolIssuancePolicyIdentityConstraintsArgs =
CaPoolIssuancePolicyIdentityConstraintsArgs(
allowSubjectAltNamesPassthrough = allowSubjectAltNamesPassthrough ?: throw
PulumiNullFieldException("allowSubjectAltNamesPassthrough"),
allowSubjectPassthrough = allowSubjectPassthrough ?: throw
PulumiNullFieldException("allowSubjectPassthrough"),
celExpression = celExpression,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy