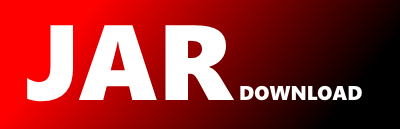
com.pulumi.gcp.certificateauthority.kotlin.inputs.CaPoolPublishingOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.certificateauthority.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.certificateauthority.inputs.CaPoolPublishingOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property encodingFormat Specifies the encoding format of each CertificateAuthority's CA
* certificate and CRLs. If this is omitted, CA certificates and CRLs
* will be published in PEM.
* Possible values are: `PEM`, `DER`.
* @property publishCaCert When true, publishes each CertificateAuthority's CA certificate and includes its URL in the "Authority Information Access"
* X.509 extension in all issued Certificates. If this is false, the CA certificate will not be published and the corresponding
* X.509 extension will not be written in issued certificates.
* @property publishCrl When true, publishes each CertificateAuthority's CRL and includes its URL in the "CRL Distribution Points" X.509 extension
* in all issued Certificates. If this is false, CRLs will not be published and the corresponding X.509 extension will not
* be written in issued certificates. CRLs will expire 7 days from their creation. However, we will rebuild daily. CRLs are
* also rebuilt shortly after a certificate is revoked.
*/
public data class CaPoolPublishingOptionsArgs(
public val encodingFormat: Output? = null,
public val publishCaCert: Output,
public val publishCrl: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.certificateauthority.inputs.CaPoolPublishingOptionsArgs =
com.pulumi.gcp.certificateauthority.inputs.CaPoolPublishingOptionsArgs.builder()
.encodingFormat(encodingFormat?.applyValue({ args0 -> args0 }))
.publishCaCert(publishCaCert.applyValue({ args0 -> args0 }))
.publishCrl(publishCrl.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CaPoolPublishingOptionsArgs].
*/
@PulumiTagMarker
public class CaPoolPublishingOptionsArgsBuilder internal constructor() {
private var encodingFormat: Output? = null
private var publishCaCert: Output? = null
private var publishCrl: Output? = null
/**
* @param value Specifies the encoding format of each CertificateAuthority's CA
* certificate and CRLs. If this is omitted, CA certificates and CRLs
* will be published in PEM.
* Possible values are: `PEM`, `DER`.
*/
@JvmName("vpkncuqnbeeaxehd")
public suspend fun encodingFormat(`value`: Output) {
this.encodingFormat = value
}
/**
* @param value When true, publishes each CertificateAuthority's CA certificate and includes its URL in the "Authority Information Access"
* X.509 extension in all issued Certificates. If this is false, the CA certificate will not be published and the corresponding
* X.509 extension will not be written in issued certificates.
*/
@JvmName("ldtvsufacrupusdl")
public suspend fun publishCaCert(`value`: Output) {
this.publishCaCert = value
}
/**
* @param value When true, publishes each CertificateAuthority's CRL and includes its URL in the "CRL Distribution Points" X.509 extension
* in all issued Certificates. If this is false, CRLs will not be published and the corresponding X.509 extension will not
* be written in issued certificates. CRLs will expire 7 days from their creation. However, we will rebuild daily. CRLs are
* also rebuilt shortly after a certificate is revoked.
*/
@JvmName("gaxbaoqrrmdbfewv")
public suspend fun publishCrl(`value`: Output) {
this.publishCrl = value
}
/**
* @param value Specifies the encoding format of each CertificateAuthority's CA
* certificate and CRLs. If this is omitted, CA certificates and CRLs
* will be published in PEM.
* Possible values are: `PEM`, `DER`.
*/
@JvmName("yijevqjayggidgoi")
public suspend fun encodingFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encodingFormat = mapped
}
/**
* @param value When true, publishes each CertificateAuthority's CA certificate and includes its URL in the "Authority Information Access"
* X.509 extension in all issued Certificates. If this is false, the CA certificate will not be published and the corresponding
* X.509 extension will not be written in issued certificates.
*/
@JvmName("tgapylgknpltbcpc")
public suspend fun publishCaCert(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.publishCaCert = mapped
}
/**
* @param value When true, publishes each CertificateAuthority's CRL and includes its URL in the "CRL Distribution Points" X.509 extension
* in all issued Certificates. If this is false, CRLs will not be published and the corresponding X.509 extension will not
* be written in issued certificates. CRLs will expire 7 days from their creation. However, we will rebuild daily. CRLs are
* also rebuilt shortly after a certificate is revoked.
*/
@JvmName("adfvnjsnfhjfvfch")
public suspend fun publishCrl(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.publishCrl = mapped
}
internal fun build(): CaPoolPublishingOptionsArgs = CaPoolPublishingOptionsArgs(
encodingFormat = encodingFormat,
publishCaCert = publishCaCert ?: throw PulumiNullFieldException("publishCaCert"),
publishCrl = publishCrl ?: throw PulumiNullFieldException("publishCrl"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy