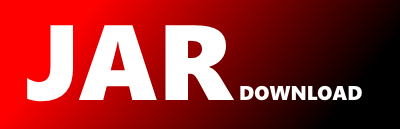
com.pulumi.gcp.certificateauthority.kotlin.inputs.CertificateConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.certificateauthority.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.certificateauthority.inputs.CertificateConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property publicKey A PublicKey describes a public key.
* Structure is documented below.
* The `x509_config` block supports:
* @property subjectConfig Specifies some of the values in a certificate that are related to the subject.
* Structure is documented below.
* @property subjectKeyId When specified this provides a custom SKI to be used in the certificate. This should only be used to maintain a SKI of an existing CA originally created outside CA service, which was not generated using method (1) described in RFC 5280 section 4.2.1.2..
* Structure is documented below.
* @property x509Config Describes how some of the technical X.509 fields in a certificate should be populated.
* Structure is documented below.
*/
public data class CertificateConfigArgs(
public val publicKey: Output,
public val subjectConfig: Output,
public val subjectKeyId: Output? = null,
public val x509Config: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.certificateauthority.inputs.CertificateConfigArgs =
com.pulumi.gcp.certificateauthority.inputs.CertificateConfigArgs.builder()
.publicKey(publicKey.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.subjectConfig(subjectConfig.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.subjectKeyId(subjectKeyId?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.x509Config(x509Config.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CertificateConfigArgs].
*/
@PulumiTagMarker
public class CertificateConfigArgsBuilder internal constructor() {
private var publicKey: Output? = null
private var subjectConfig: Output? = null
private var subjectKeyId: Output? = null
private var x509Config: Output? = null
/**
* @param value A PublicKey describes a public key.
* Structure is documented below.
* The `x509_config` block supports:
*/
@JvmName("qdwunwrbqtuoyapg")
public suspend fun publicKey(`value`: Output) {
this.publicKey = value
}
/**
* @param value Specifies some of the values in a certificate that are related to the subject.
* Structure is documented below.
*/
@JvmName("kfmauoqunpmxelle")
public suspend fun subjectConfig(`value`: Output) {
this.subjectConfig = value
}
/**
* @param value When specified this provides a custom SKI to be used in the certificate. This should only be used to maintain a SKI of an existing CA originally created outside CA service, which was not generated using method (1) described in RFC 5280 section 4.2.1.2..
* Structure is documented below.
*/
@JvmName("sgocxwjepwcfqwau")
public suspend fun subjectKeyId(`value`: Output) {
this.subjectKeyId = value
}
/**
* @param value Describes how some of the technical X.509 fields in a certificate should be populated.
* Structure is documented below.
*/
@JvmName("sxpmtwbqhrlxnrfd")
public suspend fun x509Config(`value`: Output) {
this.x509Config = value
}
/**
* @param value A PublicKey describes a public key.
* Structure is documented below.
* The `x509_config` block supports:
*/
@JvmName("gsaetnajsktqoegb")
public suspend fun publicKey(`value`: CertificateConfigPublicKeyArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.publicKey = mapped
}
/**
* @param argument A PublicKey describes a public key.
* Structure is documented below.
* The `x509_config` block supports:
*/
@JvmName("xwblqrdjlrckgamb")
public suspend fun publicKey(argument: suspend CertificateConfigPublicKeyArgsBuilder.() -> Unit) {
val toBeMapped = CertificateConfigPublicKeyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.publicKey = mapped
}
/**
* @param value Specifies some of the values in a certificate that are related to the subject.
* Structure is documented below.
*/
@JvmName("vjtsryvuawdqtswf")
public suspend fun subjectConfig(`value`: CertificateConfigSubjectConfigArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.subjectConfig = mapped
}
/**
* @param argument Specifies some of the values in a certificate that are related to the subject.
* Structure is documented below.
*/
@JvmName("ogtkgcmlrdldcxbu")
public suspend fun subjectConfig(argument: suspend CertificateConfigSubjectConfigArgsBuilder.() -> Unit) {
val toBeMapped = CertificateConfigSubjectConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.subjectConfig = mapped
}
/**
* @param value When specified this provides a custom SKI to be used in the certificate. This should only be used to maintain a SKI of an existing CA originally created outside CA service, which was not generated using method (1) described in RFC 5280 section 4.2.1.2..
* Structure is documented below.
*/
@JvmName("fmwlwqvlgfjjhxds")
public suspend fun subjectKeyId(`value`: CertificateConfigSubjectKeyIdArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subjectKeyId = mapped
}
/**
* @param argument When specified this provides a custom SKI to be used in the certificate. This should only be used to maintain a SKI of an existing CA originally created outside CA service, which was not generated using method (1) described in RFC 5280 section 4.2.1.2..
* Structure is documented below.
*/
@JvmName("nxeaaavvmacjplts")
public suspend fun subjectKeyId(argument: suspend CertificateConfigSubjectKeyIdArgsBuilder.() -> Unit) {
val toBeMapped = CertificateConfigSubjectKeyIdArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.subjectKeyId = mapped
}
/**
* @param value Describes how some of the technical X.509 fields in a certificate should be populated.
* Structure is documented below.
*/
@JvmName("iohdfsrjaeksjgbm")
public suspend fun x509Config(`value`: CertificateConfigX509ConfigArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.x509Config = mapped
}
/**
* @param argument Describes how some of the technical X.509 fields in a certificate should be populated.
* Structure is documented below.
*/
@JvmName("uakoywioeaeewfls")
public suspend fun x509Config(argument: suspend CertificateConfigX509ConfigArgsBuilder.() -> Unit) {
val toBeMapped = CertificateConfigX509ConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.x509Config = mapped
}
internal fun build(): CertificateConfigArgs = CertificateConfigArgs(
publicKey = publicKey ?: throw PulumiNullFieldException("publicKey"),
subjectConfig = subjectConfig ?: throw PulumiNullFieldException("subjectConfig"),
subjectKeyId = subjectKeyId,
x509Config = x509Config ?: throw PulumiNullFieldException("x509Config"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy