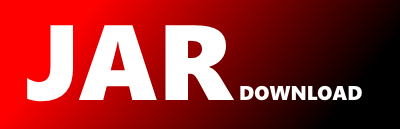
com.pulumi.gcp.certificateauthority.kotlin.inputs.CertificateConfigSubjectConfigSubjectArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.certificateauthority.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.certificateauthority.inputs.CertificateConfigSubjectConfigSubjectArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property commonName The common name of the distinguished name.
* @property countryCode The country code of the subject.
* @property locality The locality or city of the subject.
* @property organization The organization of the subject.
* @property organizationalUnit The organizational unit of the subject.
* @property postalCode The postal code of the subject.
* @property province The province, territory, or regional state of the subject.
* @property streetAddress The street address of the subject.
*/
public data class CertificateConfigSubjectConfigSubjectArgs(
public val commonName: Output,
public val countryCode: Output? = null,
public val locality: Output? = null,
public val organization: Output,
public val organizationalUnit: Output? = null,
public val postalCode: Output? = null,
public val province: Output? = null,
public val streetAddress: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.certificateauthority.inputs.CertificateConfigSubjectConfigSubjectArgs =
com.pulumi.gcp.certificateauthority.inputs.CertificateConfigSubjectConfigSubjectArgs.builder()
.commonName(commonName.applyValue({ args0 -> args0 }))
.countryCode(countryCode?.applyValue({ args0 -> args0 }))
.locality(locality?.applyValue({ args0 -> args0 }))
.organization(organization.applyValue({ args0 -> args0 }))
.organizationalUnit(organizationalUnit?.applyValue({ args0 -> args0 }))
.postalCode(postalCode?.applyValue({ args0 -> args0 }))
.province(province?.applyValue({ args0 -> args0 }))
.streetAddress(streetAddress?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CertificateConfigSubjectConfigSubjectArgs].
*/
@PulumiTagMarker
public class CertificateConfigSubjectConfigSubjectArgsBuilder internal constructor() {
private var commonName: Output? = null
private var countryCode: Output? = null
private var locality: Output? = null
private var organization: Output? = null
private var organizationalUnit: Output? = null
private var postalCode: Output? = null
private var province: Output? = null
private var streetAddress: Output? = null
/**
* @param value The common name of the distinguished name.
*/
@JvmName("lwgaaajipxmbrmcv")
public suspend fun commonName(`value`: Output) {
this.commonName = value
}
/**
* @param value The country code of the subject.
*/
@JvmName("njejxeskptpdrolk")
public suspend fun countryCode(`value`: Output) {
this.countryCode = value
}
/**
* @param value The locality or city of the subject.
*/
@JvmName("uquoehbwvxotssfh")
public suspend fun locality(`value`: Output) {
this.locality = value
}
/**
* @param value The organization of the subject.
*/
@JvmName("ftcbdajxevfnftet")
public suspend fun organization(`value`: Output) {
this.organization = value
}
/**
* @param value The organizational unit of the subject.
*/
@JvmName("wcihxanyptjngmbh")
public suspend fun organizationalUnit(`value`: Output) {
this.organizationalUnit = value
}
/**
* @param value The postal code of the subject.
*/
@JvmName("xjeipehiuupxette")
public suspend fun postalCode(`value`: Output) {
this.postalCode = value
}
/**
* @param value The province, territory, or regional state of the subject.
*/
@JvmName("cuxynihrxgtvhexv")
public suspend fun province(`value`: Output) {
this.province = value
}
/**
* @param value The street address of the subject.
*/
@JvmName("qflnvuathsihhpot")
public suspend fun streetAddress(`value`: Output) {
this.streetAddress = value
}
/**
* @param value The common name of the distinguished name.
*/
@JvmName("ufgjgsfqtrjstpgm")
public suspend fun commonName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.commonName = mapped
}
/**
* @param value The country code of the subject.
*/
@JvmName("dxlhprvkgxiumqkm")
public suspend fun countryCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.countryCode = mapped
}
/**
* @param value The locality or city of the subject.
*/
@JvmName("jdihtmjevvyrhtwt")
public suspend fun locality(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.locality = mapped
}
/**
* @param value The organization of the subject.
*/
@JvmName("oagctymmpqwbmumf")
public suspend fun organization(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.organization = mapped
}
/**
* @param value The organizational unit of the subject.
*/
@JvmName("oemytvsfexlrupqv")
public suspend fun organizationalUnit(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.organizationalUnit = mapped
}
/**
* @param value The postal code of the subject.
*/
@JvmName("wvufgrbbspucllrh")
public suspend fun postalCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postalCode = mapped
}
/**
* @param value The province, territory, or regional state of the subject.
*/
@JvmName("ooiiqympamdpmmyn")
public suspend fun province(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.province = mapped
}
/**
* @param value The street address of the subject.
*/
@JvmName("yustigbsqfgnnljc")
public suspend fun streetAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.streetAddress = mapped
}
internal fun build(): CertificateConfigSubjectConfigSubjectArgs =
CertificateConfigSubjectConfigSubjectArgs(
commonName = commonName ?: throw PulumiNullFieldException("commonName"),
countryCode = countryCode,
locality = locality,
organization = organization ?: throw PulumiNullFieldException("organization"),
organizationalUnit = organizationalUnit,
postalCode = postalCode,
province = province,
streetAddress = streetAddress,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy