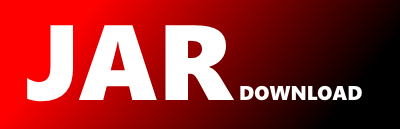
com.pulumi.gcp.certificateauthority.kotlin.inputs.CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.certificateauthority.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.certificateauthority.inputs.CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property certSign The key may be used to sign certificates.
* @property contentCommitment The key may be used for cryptographic commitments. Note that this may also be referred to as "non-repudiation".
* @property crlSign The key may be used sign certificate revocation lists.
* @property dataEncipherment The key may be used to encipher data.
* @property decipherOnly The key may be used to decipher only.
* @property digitalSignature The key may be used for digital signatures.
* @property encipherOnly The key may be used to encipher only.
* @property keyAgreement The key may be used in a key agreement protocol.
* @property keyEncipherment The key may be used to encipher other keys.
*/
public data class CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs(
public val certSign: Output? = null,
public val contentCommitment: Output? = null,
public val crlSign: Output? = null,
public val dataEncipherment: Output? = null,
public val decipherOnly: Output? = null,
public val digitalSignature: Output? = null,
public val encipherOnly: Output? = null,
public val keyAgreement: Output? = null,
public val keyEncipherment: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.certificateauthority.inputs.CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs =
com.pulumi.gcp.certificateauthority.inputs.CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs.builder()
.certSign(certSign?.applyValue({ args0 -> args0 }))
.contentCommitment(contentCommitment?.applyValue({ args0 -> args0 }))
.crlSign(crlSign?.applyValue({ args0 -> args0 }))
.dataEncipherment(dataEncipherment?.applyValue({ args0 -> args0 }))
.decipherOnly(decipherOnly?.applyValue({ args0 -> args0 }))
.digitalSignature(digitalSignature?.applyValue({ args0 -> args0 }))
.encipherOnly(encipherOnly?.applyValue({ args0 -> args0 }))
.keyAgreement(keyAgreement?.applyValue({ args0 -> args0 }))
.keyEncipherment(keyEncipherment?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs].
*/
@PulumiTagMarker
public class CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgsBuilder internal constructor() {
private var certSign: Output? = null
private var contentCommitment: Output? = null
private var crlSign: Output? = null
private var dataEncipherment: Output? = null
private var decipherOnly: Output? = null
private var digitalSignature: Output? = null
private var encipherOnly: Output? = null
private var keyAgreement: Output? = null
private var keyEncipherment: Output? = null
/**
* @param value The key may be used to sign certificates.
*/
@JvmName("hhdytxlitcriwjus")
public suspend fun certSign(`value`: Output) {
this.certSign = value
}
/**
* @param value The key may be used for cryptographic commitments. Note that this may also be referred to as "non-repudiation".
*/
@JvmName("njeibqprnlpboykr")
public suspend fun contentCommitment(`value`: Output) {
this.contentCommitment = value
}
/**
* @param value The key may be used sign certificate revocation lists.
*/
@JvmName("kkknvudpbftmkdrg")
public suspend fun crlSign(`value`: Output) {
this.crlSign = value
}
/**
* @param value The key may be used to encipher data.
*/
@JvmName("dynplickmjiephog")
public suspend fun dataEncipherment(`value`: Output) {
this.dataEncipherment = value
}
/**
* @param value The key may be used to decipher only.
*/
@JvmName("cwsavxgmnfiiystl")
public suspend fun decipherOnly(`value`: Output) {
this.decipherOnly = value
}
/**
* @param value The key may be used for digital signatures.
*/
@JvmName("jlpcrwmddltkqlaq")
public suspend fun digitalSignature(`value`: Output) {
this.digitalSignature = value
}
/**
* @param value The key may be used to encipher only.
*/
@JvmName("tuwqnnnpkeedyvnx")
public suspend fun encipherOnly(`value`: Output) {
this.encipherOnly = value
}
/**
* @param value The key may be used in a key agreement protocol.
*/
@JvmName("hrcmwlmpfnvqsrjj")
public suspend fun keyAgreement(`value`: Output) {
this.keyAgreement = value
}
/**
* @param value The key may be used to encipher other keys.
*/
@JvmName("vdbslxghhbfaaqnw")
public suspend fun keyEncipherment(`value`: Output) {
this.keyEncipherment = value
}
/**
* @param value The key may be used to sign certificates.
*/
@JvmName("govidbumsopilynw")
public suspend fun certSign(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certSign = mapped
}
/**
* @param value The key may be used for cryptographic commitments. Note that this may also be referred to as "non-repudiation".
*/
@JvmName("mbtynmemqfsrdsfc")
public suspend fun contentCommitment(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentCommitment = mapped
}
/**
* @param value The key may be used sign certificate revocation lists.
*/
@JvmName("lngmlhokwuconrfo")
public suspend fun crlSign(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crlSign = mapped
}
/**
* @param value The key may be used to encipher data.
*/
@JvmName("iqeivewtfkhwwdyh")
public suspend fun dataEncipherment(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataEncipherment = mapped
}
/**
* @param value The key may be used to decipher only.
*/
@JvmName("bnvhcttsftnqvjub")
public suspend fun decipherOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.decipherOnly = mapped
}
/**
* @param value The key may be used for digital signatures.
*/
@JvmName("ksvtcuvmquyievrc")
public suspend fun digitalSignature(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.digitalSignature = mapped
}
/**
* @param value The key may be used to encipher only.
*/
@JvmName("vcyfbjwvgobwnwqm")
public suspend fun encipherOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encipherOnly = mapped
}
/**
* @param value The key may be used in a key agreement protocol.
*/
@JvmName("klwwkfplbiepowti")
public suspend fun keyAgreement(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyAgreement = mapped
}
/**
* @param value The key may be used to encipher other keys.
*/
@JvmName("mwpshosvvfsquujq")
public suspend fun keyEncipherment(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyEncipherment = mapped
}
internal fun build(): CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs =
CertificateConfigX509ConfigKeyUsageBaseKeyUsageArgs(
certSign = certSign,
contentCommitment = contentCommitment,
crlSign = crlSign,
dataEncipherment = dataEncipherment,
decipherOnly = decipherOnly,
digitalSignature = digitalSignature,
encipherOnly = encipherOnly,
keyAgreement = keyAgreement,
keyEncipherment = keyEncipherment,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy