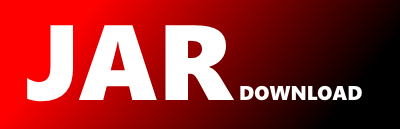
com.pulumi.gcp.cloudasset.kotlin.CloudassetFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudasset.kotlin
import com.pulumi.gcp.cloudasset.CloudassetFunctions.getResourcesSearchAllPlain
import com.pulumi.gcp.cloudasset.CloudassetFunctions.getSearchAllResourcesPlain
import com.pulumi.gcp.cloudasset.kotlin.inputs.GetResourcesSearchAllPlainArgs
import com.pulumi.gcp.cloudasset.kotlin.inputs.GetResourcesSearchAllPlainArgsBuilder
import com.pulumi.gcp.cloudasset.kotlin.inputs.GetSearchAllResourcesPlainArgs
import com.pulumi.gcp.cloudasset.kotlin.inputs.GetSearchAllResourcesPlainArgsBuilder
import com.pulumi.gcp.cloudasset.kotlin.outputs.GetResourcesSearchAllInvokeResult
import com.pulumi.gcp.cloudasset.kotlin.outputs.GetSearchAllResourcesInvokeResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.cloudasset.kotlin.outputs.GetResourcesSearchAllInvokeResult.Companion.toKotlin as getResourcesSearchAllInvokeResultToKotlin
import com.pulumi.gcp.cloudasset.kotlin.outputs.GetSearchAllResourcesInvokeResult.Companion.toKotlin as getSearchAllResourcesInvokeResultToKotlin
public object CloudassetFunctions {
/**
* ## Example Usage
* ### Searching For All Projects In An Org
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const projects = gcp.cloudasset.getResourcesSearchAll({
* scope: "organizations/0123456789",
* assetTypes: ["cloudresourcemanager.googleapis.com/Project"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* projects = gcp.cloudasset.get_resources_search_all(scope="organizations/0123456789",
* asset_types=["cloudresourcemanager.googleapis.com/Project"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var projects = Gcp.CloudAsset.GetResourcesSearchAll.Invoke(new()
* {
* Scope = "organizations/0123456789",
* AssetTypes = new[]
* {
* "cloudresourcemanager.googleapis.com/Project",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudasset"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudasset.LookupResourcesSearchAll(ctx, &cloudasset.LookupResourcesSearchAllArgs{
* Scope: "organizations/0123456789",
* AssetTypes: []string{
* "cloudresourcemanager.googleapis.com/Project",
* },
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudasset.CloudassetFunctions;
* import com.pulumi.gcp.cloudasset.inputs.GetResourcesSearchAllArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var projects = CloudassetFunctions.getResourcesSearchAll(GetResourcesSearchAllArgs.builder()
* .scope("organizations/0123456789")
* .assetTypes("cloudresourcemanager.googleapis.com/Project")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* projects:
* fn::invoke:
* Function: gcp:cloudasset:getResourcesSearchAll
* Arguments:
* scope: organizations/0123456789
* assetTypes:
* - cloudresourcemanager.googleapis.com/Project
* ```
*
* ### Searching For All Projects With CloudBuild API Enabled
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const cloudBuildProjects = gcp.cloudasset.getResourcesSearchAll({
* scope: "organizations/0123456789",
* assetTypes: ["serviceusage.googleapis.com/Service"],
* query: "displayName:cloudbuild.googleapis.com AND state:ENABLED",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* cloud_build_projects = gcp.cloudasset.get_resources_search_all(scope="organizations/0123456789",
* asset_types=["serviceusage.googleapis.com/Service"],
* query="displayName:cloudbuild.googleapis.com AND state:ENABLED")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var cloudBuildProjects = Gcp.CloudAsset.GetResourcesSearchAll.Invoke(new()
* {
* Scope = "organizations/0123456789",
* AssetTypes = new[]
* {
* "serviceusage.googleapis.com/Service",
* },
* Query = "displayName:cloudbuild.googleapis.com AND state:ENABLED",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudasset"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudasset.LookupResourcesSearchAll(ctx, &cloudasset.LookupResourcesSearchAllArgs{
* Scope: "organizations/0123456789",
* AssetTypes: []string{
* "serviceusage.googleapis.com/Service",
* },
* Query: pulumi.StringRef("displayName:cloudbuild.googleapis.com AND state:ENABLED"),
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudasset.CloudassetFunctions;
* import com.pulumi.gcp.cloudasset.inputs.GetResourcesSearchAllArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var cloudBuildProjects = CloudassetFunctions.getResourcesSearchAll(GetResourcesSearchAllArgs.builder()
* .scope("organizations/0123456789")
* .assetTypes("serviceusage.googleapis.com/Service")
* .query("displayName:cloudbuild.googleapis.com AND state:ENABLED")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* cloudBuildProjects:
* fn::invoke:
* Function: gcp:cloudasset:getResourcesSearchAll
* Arguments:
* scope: organizations/0123456789
* assetTypes:
* - serviceusage.googleapis.com/Service
* query: displayName:cloudbuild.googleapis.com AND state:ENABLED
* ```
*
* ### Searching For All Service Accounts In A Project
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const projectServiceAccounts = gcp.cloudasset.getResourcesSearchAll({
* scope: "projects/my-project-id",
* assetTypes: ["iam.googleapis.com/ServiceAccount"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project_service_accounts = gcp.cloudasset.get_resources_search_all(scope="projects/my-project-id",
* asset_types=["iam.googleapis.com/ServiceAccount"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var projectServiceAccounts = Gcp.CloudAsset.GetResourcesSearchAll.Invoke(new()
* {
* Scope = "projects/my-project-id",
* AssetTypes = new[]
* {
* "iam.googleapis.com/ServiceAccount",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudasset"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudasset.LookupResourcesSearchAll(ctx, &cloudasset.LookupResourcesSearchAllArgs{
* Scope: "projects/my-project-id",
* AssetTypes: []string{
* "iam.googleapis.com/ServiceAccount",
* },
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudasset.CloudassetFunctions;
* import com.pulumi.gcp.cloudasset.inputs.GetResourcesSearchAllArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var projectServiceAccounts = CloudassetFunctions.getResourcesSearchAll(GetResourcesSearchAllArgs.builder()
* .scope("projects/my-project-id")
* .assetTypes("iam.googleapis.com/ServiceAccount")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* projectServiceAccounts:
* fn::invoke:
* Function: gcp:cloudasset:getResourcesSearchAll
* Arguments:
* scope: projects/my-project-id
* assetTypes:
* - iam.googleapis.com/ServiceAccount
* ```
*
* @param argument A collection of arguments for invoking getResourcesSearchAll.
* @return A collection of values returned by getResourcesSearchAll.
*/
public suspend fun getResourcesSearchAll(argument: GetResourcesSearchAllPlainArgs): GetResourcesSearchAllInvokeResult =
getResourcesSearchAllInvokeResultToKotlin(getResourcesSearchAllPlain(argument.toJava()).await())
/**
* @see [getResourcesSearchAll].
* @param assetTypes A list of asset types that this request searches for. If empty, it will search all the [supported asset types](https://cloud.google.com/asset-inventory/docs/supported-asset-types).
* @param query The query statement. See [how to construct a query](https://cloud.google.com/asset-inventory/docs/searching-resources#how_to_construct_a_query) for more information. If not specified or empty, it will search all the resources within the specified `scope` and `asset_types`.
* @param scope A scope can be a project, a folder, or an organization. The allowed value must be: organization number (such as "organizations/123"), folder number (such as "folders/1234"), project number (such as "projects/12345") or project id (such as "projects/abc")
* @return A collection of values returned by getResourcesSearchAll.
*/
public suspend fun getResourcesSearchAll(
assetTypes: List? = null,
query: String? = null,
scope: String,
): GetResourcesSearchAllInvokeResult {
val argument = GetResourcesSearchAllPlainArgs(
assetTypes = assetTypes,
query = query,
scope = scope,
)
return getResourcesSearchAllInvokeResultToKotlin(getResourcesSearchAllPlain(argument.toJava()).await())
}
/**
* @see [getResourcesSearchAll].
* @param argument Builder for [com.pulumi.gcp.cloudasset.kotlin.inputs.GetResourcesSearchAllPlainArgs].
* @return A collection of values returned by getResourcesSearchAll.
*/
public suspend fun getResourcesSearchAll(argument: suspend GetResourcesSearchAllPlainArgsBuilder.() -> Unit): GetResourcesSearchAllInvokeResult {
val builder = GetResourcesSearchAllPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getResourcesSearchAllInvokeResultToKotlin(getResourcesSearchAllPlain(builtArgument.toJava()).await())
}
/**
* Searches all Google Cloud resources within the specified scope, such as a project, folder, or organization. See the
* [REST API](https://cloud.google.com/asset-inventory/docs/reference/rest/v1/TopLevel/searchAllResources)
* for more details.
* ## Example Usage
* ### Searching For All Projects In An Org
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const projects = gcp.cloudasset.getSearchAllResources({
* scope: "organizations/0123456789",
* assetTypes: ["cloudresourcemanager.googleapis.com/Project"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* projects = gcp.cloudasset.get_search_all_resources(scope="organizations/0123456789",
* asset_types=["cloudresourcemanager.googleapis.com/Project"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var projects = Gcp.CloudAsset.GetSearchAllResources.Invoke(new()
* {
* Scope = "organizations/0123456789",
* AssetTypes = new[]
* {
* "cloudresourcemanager.googleapis.com/Project",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudasset"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudasset.LookupSearchAllResources(ctx, &cloudasset.LookupSearchAllResourcesArgs{
* Scope: "organizations/0123456789",
* AssetTypes: []string{
* "cloudresourcemanager.googleapis.com/Project",
* },
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudasset.CloudassetFunctions;
* import com.pulumi.gcp.cloudasset.inputs.GetSearchAllResourcesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var projects = CloudassetFunctions.getSearchAllResources(GetSearchAllResourcesArgs.builder()
* .scope("organizations/0123456789")
* .assetTypes("cloudresourcemanager.googleapis.com/Project")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* projects:
* fn::invoke:
* Function: gcp:cloudasset:getSearchAllResources
* Arguments:
* scope: organizations/0123456789
* assetTypes:
* - cloudresourcemanager.googleapis.com/Project
* ```
*
* ### Searching For All Projects With CloudBuild API Enabled
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const cloudBuildProjects = gcp.cloudasset.getSearchAllResources({
* scope: "organizations/0123456789",
* assetTypes: ["serviceusage.googleapis.com/Service"],
* query: "displayName:cloudbuild.googleapis.com AND state:ENABLED",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* cloud_build_projects = gcp.cloudasset.get_search_all_resources(scope="organizations/0123456789",
* asset_types=["serviceusage.googleapis.com/Service"],
* query="displayName:cloudbuild.googleapis.com AND state:ENABLED")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var cloudBuildProjects = Gcp.CloudAsset.GetSearchAllResources.Invoke(new()
* {
* Scope = "organizations/0123456789",
* AssetTypes = new[]
* {
* "serviceusage.googleapis.com/Service",
* },
* Query = "displayName:cloudbuild.googleapis.com AND state:ENABLED",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudasset"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudasset.LookupSearchAllResources(ctx, &cloudasset.LookupSearchAllResourcesArgs{
* Scope: "organizations/0123456789",
* AssetTypes: []string{
* "serviceusage.googleapis.com/Service",
* },
* Query: pulumi.StringRef("displayName:cloudbuild.googleapis.com AND state:ENABLED"),
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudasset.CloudassetFunctions;
* import com.pulumi.gcp.cloudasset.inputs.GetSearchAllResourcesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var cloudBuildProjects = CloudassetFunctions.getSearchAllResources(GetSearchAllResourcesArgs.builder()
* .scope("organizations/0123456789")
* .assetTypes("serviceusage.googleapis.com/Service")
* .query("displayName:cloudbuild.googleapis.com AND state:ENABLED")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* cloudBuildProjects:
* fn::invoke:
* Function: gcp:cloudasset:getSearchAllResources
* Arguments:
* scope: organizations/0123456789
* assetTypes:
* - serviceusage.googleapis.com/Service
* query: displayName:cloudbuild.googleapis.com AND state:ENABLED
* ```
*
* ### Searching For All Service Accounts In A Project
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const projectServiceAccounts = gcp.cloudasset.getSearchAllResources({
* scope: "projects/my-project-id",
* assetTypes: ["iam.googleapis.com/ServiceAccount"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project_service_accounts = gcp.cloudasset.get_search_all_resources(scope="projects/my-project-id",
* asset_types=["iam.googleapis.com/ServiceAccount"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var projectServiceAccounts = Gcp.CloudAsset.GetSearchAllResources.Invoke(new()
* {
* Scope = "projects/my-project-id",
* AssetTypes = new[]
* {
* "iam.googleapis.com/ServiceAccount",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudasset"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudasset.LookupSearchAllResources(ctx, &cloudasset.LookupSearchAllResourcesArgs{
* Scope: "projects/my-project-id",
* AssetTypes: []string{
* "iam.googleapis.com/ServiceAccount",
* },
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudasset.CloudassetFunctions;
* import com.pulumi.gcp.cloudasset.inputs.GetSearchAllResourcesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var projectServiceAccounts = CloudassetFunctions.getSearchAllResources(GetSearchAllResourcesArgs.builder()
* .scope("projects/my-project-id")
* .assetTypes("iam.googleapis.com/ServiceAccount")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* projectServiceAccounts:
* fn::invoke:
* Function: gcp:cloudasset:getSearchAllResources
* Arguments:
* scope: projects/my-project-id
* assetTypes:
* - iam.googleapis.com/ServiceAccount
* ```
*
* @param argument A collection of arguments for invoking getSearchAllResources.
* @return A collection of values returned by getSearchAllResources.
*/
public suspend fun getSearchAllResources(argument: GetSearchAllResourcesPlainArgs): GetSearchAllResourcesInvokeResult =
getSearchAllResourcesInvokeResultToKotlin(getSearchAllResourcesPlain(argument.toJava()).await())
/**
* @see [getSearchAllResources].
* @param assetTypes A list of asset types that this request searches for. If empty, it will search all the [supported asset types](https://cloud.google.com/asset-inventory/docs/supported-asset-types).
* @param query The query statement. See [how to construct a query](https://cloud.google.com/asset-inventory/docs/searching-resources#how_to_construct_a_query) for more information. If not specified or empty, it will search all the resources within the specified `scope` and `asset_types`.
* @param scope A scope can be a project, a folder, or an organization. The search is limited to the resources within the scope. The allowed value must be: organization number (such as "organizations/123"), folder number (such as "folders/1234"), project number (such as "projects/12345") or project id (such as "projects/abc")
* @return A collection of values returned by getSearchAllResources.
*/
public suspend fun getSearchAllResources(
assetTypes: List? = null,
query: String? = null,
scope: String,
): GetSearchAllResourcesInvokeResult {
val argument = GetSearchAllResourcesPlainArgs(
assetTypes = assetTypes,
query = query,
scope = scope,
)
return getSearchAllResourcesInvokeResultToKotlin(getSearchAllResourcesPlain(argument.toJava()).await())
}
/**
* @see [getSearchAllResources].
* @param argument Builder for [com.pulumi.gcp.cloudasset.kotlin.inputs.GetSearchAllResourcesPlainArgs].
* @return A collection of values returned by getSearchAllResources.
*/
public suspend fun getSearchAllResources(argument: suspend GetSearchAllResourcesPlainArgsBuilder.() -> Unit): GetSearchAllResourcesInvokeResult {
val builder = GetSearchAllResourcesPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSearchAllResourcesInvokeResultToKotlin(getSearchAllResourcesPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy