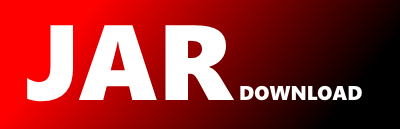
com.pulumi.gcp.cloudbuild.kotlin.inputs.TriggerGithubArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudbuild.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudbuild.inputs.TriggerGithubArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enterpriseConfigResourceName The resource name of the github enterprise config that should be applied to this installation.
* For example: "projects/{$projectId}/locations/{$locationId}/githubEnterpriseConfigs/{$configId}"
* @property name Name of the repository. For example: The name for
* https://github.com/googlecloudplatform/cloud-builders is "cloud-builders".
* @property owner Owner of the repository. For example: The owner for
* https://github.com/googlecloudplatform/cloud-builders is "googlecloudplatform".
* @property pullRequest filter to match changes in pull requests. Specify only one of `pull_request` or `push`.
* Structure is documented below.
* @property push filter to match changes in refs, like branches or tags. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
public data class TriggerGithubArgs(
public val enterpriseConfigResourceName: Output? = null,
public val name: Output? = null,
public val owner: Output? = null,
public val pullRequest: Output? = null,
public val push: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudbuild.inputs.TriggerGithubArgs =
com.pulumi.gcp.cloudbuild.inputs.TriggerGithubArgs.builder()
.enterpriseConfigResourceName(enterpriseConfigResourceName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.owner(owner?.applyValue({ args0 -> args0 }))
.pullRequest(pullRequest?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.push(push?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TriggerGithubArgs].
*/
@PulumiTagMarker
public class TriggerGithubArgsBuilder internal constructor() {
private var enterpriseConfigResourceName: Output? = null
private var name: Output? = null
private var owner: Output? = null
private var pullRequest: Output? = null
private var push: Output? = null
/**
* @param value The resource name of the github enterprise config that should be applied to this installation.
* For example: "projects/{$projectId}/locations/{$locationId}/githubEnterpriseConfigs/{$configId}"
*/
@JvmName("rdqniyjfpegaoicx")
public suspend fun enterpriseConfigResourceName(`value`: Output) {
this.enterpriseConfigResourceName = value
}
/**
* @param value Name of the repository. For example: The name for
* https://github.com/googlecloudplatform/cloud-builders is "cloud-builders".
*/
@JvmName("wprlojavufhcmgvt")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Owner of the repository. For example: The owner for
* https://github.com/googlecloudplatform/cloud-builders is "googlecloudplatform".
*/
@JvmName("dtpbxpeopqewboeu")
public suspend fun owner(`value`: Output) {
this.owner = value
}
/**
* @param value filter to match changes in pull requests. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
@JvmName("lmhnmamjiuvnqchg")
public suspend fun pullRequest(`value`: Output) {
this.pullRequest = value
}
/**
* @param value filter to match changes in refs, like branches or tags. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
@JvmName("uggwixpuhkevyyup")
public suspend fun push(`value`: Output) {
this.push = value
}
/**
* @param value The resource name of the github enterprise config that should be applied to this installation.
* For example: "projects/{$projectId}/locations/{$locationId}/githubEnterpriseConfigs/{$configId}"
*/
@JvmName("gqbrrfyuigedimfd")
public suspend fun enterpriseConfigResourceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enterpriseConfigResourceName = mapped
}
/**
* @param value Name of the repository. For example: The name for
* https://github.com/googlecloudplatform/cloud-builders is "cloud-builders".
*/
@JvmName("qhtqalhvxlkxedgp")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Owner of the repository. For example: The owner for
* https://github.com/googlecloudplatform/cloud-builders is "googlecloudplatform".
*/
@JvmName("tkluwseyhfxiogex")
public suspend fun owner(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.owner = mapped
}
/**
* @param value filter to match changes in pull requests. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
@JvmName("kaodvgqilmvkooov")
public suspend fun pullRequest(`value`: TriggerGithubPullRequestArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pullRequest = mapped
}
/**
* @param argument filter to match changes in pull requests. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
@JvmName("rclqjfptanyiptma")
public suspend fun pullRequest(argument: suspend TriggerGithubPullRequestArgsBuilder.() -> Unit) {
val toBeMapped = TriggerGithubPullRequestArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.pullRequest = mapped
}
/**
* @param value filter to match changes in refs, like branches or tags. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
@JvmName("xhkfvcwbtgswhugo")
public suspend fun push(`value`: TriggerGithubPushArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.push = mapped
}
/**
* @param argument filter to match changes in refs, like branches or tags. Specify only one of `pull_request` or `push`.
* Structure is documented below.
*/
@JvmName("slplsfjsqliriytf")
public suspend fun push(argument: suspend TriggerGithubPushArgsBuilder.() -> Unit) {
val toBeMapped = TriggerGithubPushArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.push = mapped
}
internal fun build(): TriggerGithubArgs = TriggerGithubArgs(
enterpriseConfigResourceName = enterpriseConfigResourceName,
name = name,
owner = owner,
pullRequest = pullRequest,
push = push,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy