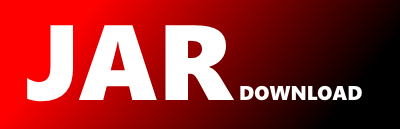
com.pulumi.gcp.clouddomains.kotlin.inputs.RegistrationContactSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.clouddomains.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.clouddomains.inputs.RegistrationContactSettingsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property adminContact Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
* @property privacy Required. Privacy setting for the contacts associated with the Registration.
* Values are PUBLIC_CONTACT_DATA, PRIVATE_CONTACT_DATA, and REDACTED_CONTACT_DATA
* @property registrantContact Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
* @property technicalContact Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
public data class RegistrationContactSettingsArgs(
public val adminContact: Output,
public val privacy: Output,
public val registrantContact: Output,
public val technicalContact: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.clouddomains.inputs.RegistrationContactSettingsArgs =
com.pulumi.gcp.clouddomains.inputs.RegistrationContactSettingsArgs.builder()
.adminContact(adminContact.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.privacy(privacy.applyValue({ args0 -> args0 }))
.registrantContact(registrantContact.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.technicalContact(
technicalContact.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [RegistrationContactSettingsArgs].
*/
@PulumiTagMarker
public class RegistrationContactSettingsArgsBuilder internal constructor() {
private var adminContact: Output? = null
private var privacy: Output? = null
private var registrantContact: Output? = null
private var technicalContact: Output? = null
/**
* @param value Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("cjnsyjtogeiuatrv")
public suspend fun adminContact(`value`: Output) {
this.adminContact = value
}
/**
* @param value Required. Privacy setting for the contacts associated with the Registration.
* Values are PUBLIC_CONTACT_DATA, PRIVATE_CONTACT_DATA, and REDACTED_CONTACT_DATA
*/
@JvmName("gehxjbxbmsbjcubb")
public suspend fun privacy(`value`: Output) {
this.privacy = value
}
/**
* @param value Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("efaokyrnmiudnaay")
public suspend fun registrantContact(`value`: Output) {
this.registrantContact = value
}
/**
* @param value Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("ghelndnyqlvvlduo")
public suspend fun technicalContact(`value`: Output) {
this.technicalContact = value
}
/**
* @param value Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("kqvegdmltscavnwq")
public suspend fun adminContact(`value`: RegistrationContactSettingsAdminContactArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.adminContact = mapped
}
/**
* @param argument Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("fwbsmlveamsnhqhy")
public suspend fun adminContact(argument: suspend RegistrationContactSettingsAdminContactArgsBuilder.() -> Unit) {
val toBeMapped = RegistrationContactSettingsAdminContactArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.adminContact = mapped
}
/**
* @param value Required. Privacy setting for the contacts associated with the Registration.
* Values are PUBLIC_CONTACT_DATA, PRIVATE_CONTACT_DATA, and REDACTED_CONTACT_DATA
*/
@JvmName("ckeqxkupcvqlbahi")
public suspend fun privacy(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privacy = mapped
}
/**
* @param value Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("pqcmsjgedncrliba")
public suspend fun registrantContact(`value`: RegistrationContactSettingsRegistrantContactArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.registrantContact = mapped
}
/**
* @param argument Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("mjwqjspnjamglxbj")
public suspend fun registrantContact(argument: suspend RegistrationContactSettingsRegistrantContactArgsBuilder.() -> Unit) {
val toBeMapped = RegistrationContactSettingsRegistrantContactArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.registrantContact = mapped
}
/**
* @param value Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("kkedjteaetxwyoap")
public suspend fun technicalContact(`value`: RegistrationContactSettingsTechnicalContactArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.technicalContact = mapped
}
/**
* @param argument Caution: Anyone with access to this email address, phone number, and/or postal address can take control of the domain.
* Warning: For new Registrations, the registrant receives an email confirmation that they must complete within 15 days to
* avoid domain suspension.
* Structure is documented below.
*/
@JvmName("esljixnbwvgvwjkj")
public suspend fun technicalContact(argument: suspend RegistrationContactSettingsTechnicalContactArgsBuilder.() -> Unit) {
val toBeMapped = RegistrationContactSettingsTechnicalContactArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.technicalContact = mapped
}
internal fun build(): RegistrationContactSettingsArgs = RegistrationContactSettingsArgs(
adminContact = adminContact ?: throw PulumiNullFieldException("adminContact"),
privacy = privacy ?: throw PulumiNullFieldException("privacy"),
registrantContact = registrantContact ?: throw PulumiNullFieldException("registrantContact"),
technicalContact = technicalContact ?: throw PulumiNullFieldException("technicalContact"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy