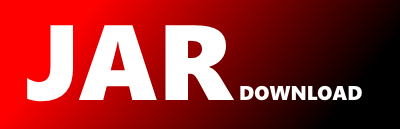
com.pulumi.gcp.clouddomains.kotlin.inputs.RegistrationManagementSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.clouddomains.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.clouddomains.inputs.RegistrationManagementSettingsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property preferredRenewalMethod The desired renewal method for this Registration. The actual renewalMethod is automatically updated to reflect this choice.
* If unset or equal to RENEWAL_METHOD_UNSPECIFIED, the actual renewalMethod is treated as if it were set to AUTOMATIC_RENEWAL.
* You cannot use RENEWAL_DISABLED during resource creation, and you can update the renewal status only when the Registration
* resource has state ACTIVE or SUSPENDED.
* When preferredRenewalMethod is set to AUTOMATIC_RENEWAL, the actual renewalMethod can be set to RENEWAL_DISABLED in case of
* problems with the billing account or reported domain abuse. In such cases, check the issues field on the Registration. After
* the problem is resolved, the renewalMethod is automatically updated to preferredRenewalMethod in a few hours.
* @property renewalMethod (Output)
* Output only. The actual renewal method for this Registration. When preferredRenewalMethod is set to AUTOMATIC_RENEWAL,
* the actual renewalMethod can be equal to RENEWAL_DISABLED—for example, when there are problems with the billing account
* or reported domain abuse. In such cases, check the issues field on the Registration. After the problem is resolved, the
* renewalMethod is automatically updated to preferredRenewalMethod in a few hours.
* @property transferLockState Controls whether the domain can be transferred to another registrar. Values are UNLOCKED or LOCKED.
*/
public data class RegistrationManagementSettingsArgs(
public val preferredRenewalMethod: Output? = null,
public val renewalMethod: Output? = null,
public val transferLockState: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.clouddomains.inputs.RegistrationManagementSettingsArgs =
com.pulumi.gcp.clouddomains.inputs.RegistrationManagementSettingsArgs.builder()
.preferredRenewalMethod(preferredRenewalMethod?.applyValue({ args0 -> args0 }))
.renewalMethod(renewalMethod?.applyValue({ args0 -> args0 }))
.transferLockState(transferLockState?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegistrationManagementSettingsArgs].
*/
@PulumiTagMarker
public class RegistrationManagementSettingsArgsBuilder internal constructor() {
private var preferredRenewalMethod: Output? = null
private var renewalMethod: Output? = null
private var transferLockState: Output? = null
/**
* @param value The desired renewal method for this Registration. The actual renewalMethod is automatically updated to reflect this choice.
* If unset or equal to RENEWAL_METHOD_UNSPECIFIED, the actual renewalMethod is treated as if it were set to AUTOMATIC_RENEWAL.
* You cannot use RENEWAL_DISABLED during resource creation, and you can update the renewal status only when the Registration
* resource has state ACTIVE or SUSPENDED.
* When preferredRenewalMethod is set to AUTOMATIC_RENEWAL, the actual renewalMethod can be set to RENEWAL_DISABLED in case of
* problems with the billing account or reported domain abuse. In such cases, check the issues field on the Registration. After
* the problem is resolved, the renewalMethod is automatically updated to preferredRenewalMethod in a few hours.
*/
@JvmName("dxixjlgmycjmwwns")
public suspend fun preferredRenewalMethod(`value`: Output) {
this.preferredRenewalMethod = value
}
/**
* @param value (Output)
* Output only. The actual renewal method for this Registration. When preferredRenewalMethod is set to AUTOMATIC_RENEWAL,
* the actual renewalMethod can be equal to RENEWAL_DISABLED—for example, when there are problems with the billing account
* or reported domain abuse. In such cases, check the issues field on the Registration. After the problem is resolved, the
* renewalMethod is automatically updated to preferredRenewalMethod in a few hours.
*/
@JvmName("uwvotjtawaswjhxu")
public suspend fun renewalMethod(`value`: Output) {
this.renewalMethod = value
}
/**
* @param value Controls whether the domain can be transferred to another registrar. Values are UNLOCKED or LOCKED.
*/
@JvmName("lswttjlgousaocuq")
public suspend fun transferLockState(`value`: Output) {
this.transferLockState = value
}
/**
* @param value The desired renewal method for this Registration. The actual renewalMethod is automatically updated to reflect this choice.
* If unset or equal to RENEWAL_METHOD_UNSPECIFIED, the actual renewalMethod is treated as if it were set to AUTOMATIC_RENEWAL.
* You cannot use RENEWAL_DISABLED during resource creation, and you can update the renewal status only when the Registration
* resource has state ACTIVE or SUSPENDED.
* When preferredRenewalMethod is set to AUTOMATIC_RENEWAL, the actual renewalMethod can be set to RENEWAL_DISABLED in case of
* problems with the billing account or reported domain abuse. In such cases, check the issues field on the Registration. After
* the problem is resolved, the renewalMethod is automatically updated to preferredRenewalMethod in a few hours.
*/
@JvmName("pgxaitqinykofgpt")
public suspend fun preferredRenewalMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preferredRenewalMethod = mapped
}
/**
* @param value (Output)
* Output only. The actual renewal method for this Registration. When preferredRenewalMethod is set to AUTOMATIC_RENEWAL,
* the actual renewalMethod can be equal to RENEWAL_DISABLED—for example, when there are problems with the billing account
* or reported domain abuse. In such cases, check the issues field on the Registration. After the problem is resolved, the
* renewalMethod is automatically updated to preferredRenewalMethod in a few hours.
*/
@JvmName("mdwllsfxrgtfbnfu")
public suspend fun renewalMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.renewalMethod = mapped
}
/**
* @param value Controls whether the domain can be transferred to another registrar. Values are UNLOCKED or LOCKED.
*/
@JvmName("rucwfbqylapiajii")
public suspend fun transferLockState(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transferLockState = mapped
}
internal fun build(): RegistrationManagementSettingsArgs = RegistrationManagementSettingsArgs(
preferredRenewalMethod = preferredRenewalMethod,
renewalMethod = renewalMethod,
transferLockState = transferLockState,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy