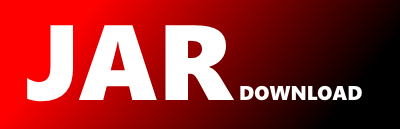
com.pulumi.gcp.cloudfunctions.kotlin.Function.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudfunctions.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionEventTrigger
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionSecretEnvironmentVariable
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionSecretVolume
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionSourceRepository
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionEventTrigger.Companion.toKotlin as functionEventTriggerToKotlin
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionSecretEnvironmentVariable.Companion.toKotlin as functionSecretEnvironmentVariableToKotlin
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionSecretVolume.Companion.toKotlin as functionSecretVolumeToKotlin
import com.pulumi.gcp.cloudfunctions.kotlin.outputs.FunctionSourceRepository.Companion.toKotlin as functionSourceRepositoryToKotlin
/**
* Builder for [Function].
*/
@PulumiTagMarker
public class FunctionResourceBuilder internal constructor() {
public var name: String? = null
public var args: FunctionArgs = FunctionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FunctionArgsBuilder.() -> Unit) {
val builder = FunctionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Function {
val builtJavaResource = com.pulumi.gcp.cloudfunctions.Function(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Function(builtJavaResource)
}
}
/**
* Creates a new Cloud Function. For more information see:
* * [API documentation](https://cloud.google.com/functions/docs/reference/rest/v1/projects.locations.functions)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/functions/docs)
* > **Warning:** As of November 1, 2019, newly created Functions are
* private-by-default and will require [appropriate IAM permissions](https://cloud.google.com/functions/docs/reference/iam/roles)
* to be invoked. See below examples for how to set up the appropriate permissions,
* or view the [Cloud Functions IAM resources](https://www.terraform.io/docs/providers/google/r/cloudfunctions_cloud_function_iam.html)
* for Cloud Functions.
* ## Example Usage
* ### Public Function
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "test-bucket",
* location: "US",
* });
* const archive = new gcp.storage.BucketObject("archive", {
* name: "index.zip",
* bucket: bucket.name,
* source: new pulumi.asset.FileAsset("./path/to/zip/file/which/contains/code"),
* });
* const _function = new gcp.cloudfunctions.Function("function", {
* name: "function-test",
* description: "My function",
* runtime: "nodejs16",
* availableMemoryMb: 128,
* sourceArchiveBucket: bucket.name,
* sourceArchiveObject: archive.name,
* triggerHttp: true,
* entryPoint: "helloGET",
* });
* // IAM entry for all users to invoke the function
* const invoker = new gcp.cloudfunctions.FunctionIamMember("invoker", {
* project: _function.project,
* region: _function.region,
* cloudFunction: _function.name,
* role: "roles/cloudfunctions.invoker",
* member: "allUsers",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* bucket = gcp.storage.Bucket("bucket",
* name="test-bucket",
* location="US")
* archive = gcp.storage.BucketObject("archive",
* name="index.zip",
* bucket=bucket.name,
* source=pulumi.FileAsset("./path/to/zip/file/which/contains/code"))
* function = gcp.cloudfunctions.Function("function",
* name="function-test",
* description="My function",
* runtime="nodejs16",
* available_memory_mb=128,
* source_archive_bucket=bucket.name,
* source_archive_object=archive.name,
* trigger_http=True,
* entry_point="helloGET")
* # IAM entry for all users to invoke the function
* invoker = gcp.cloudfunctions.FunctionIamMember("invoker",
* project=function.project,
* region=function.region,
* cloud_function=function.name,
* role="roles/cloudfunctions.invoker",
* member="allUsers")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "test-bucket",
* Location = "US",
* });
* var archive = new Gcp.Storage.BucketObject("archive", new()
* {
* Name = "index.zip",
* Bucket = bucket.Name,
* Source = new FileAsset("./path/to/zip/file/which/contains/code"),
* });
* var function = new Gcp.CloudFunctions.Function("function", new()
* {
* Name = "function-test",
* Description = "My function",
* Runtime = "nodejs16",
* AvailableMemoryMb = 128,
* SourceArchiveBucket = bucket.Name,
* SourceArchiveObject = archive.Name,
* TriggerHttp = true,
* EntryPoint = "helloGET",
* });
* // IAM entry for all users to invoke the function
* var invoker = new Gcp.CloudFunctions.FunctionIamMember("invoker", new()
* {
* Project = function.Project,
* Region = function.Region,
* CloudFunction = function.Name,
* Role = "roles/cloudfunctions.invoker",
* Member = "allUsers",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudfunctions"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("test-bucket"),
* Location: pulumi.String("US"),
* })
* if err != nil {
* return err
* }
* archive, err := storage.NewBucketObject(ctx, "archive", &storage.BucketObjectArgs{
* Name: pulumi.String("index.zip"),
* Bucket: bucket.Name,
* Source: pulumi.NewFileAsset("./path/to/zip/file/which/contains/code"),
* })
* if err != nil {
* return err
* }
* function, err := cloudfunctions.NewFunction(ctx, "function", &cloudfunctions.FunctionArgs{
* Name: pulumi.String("function-test"),
* Description: pulumi.String("My function"),
* Runtime: pulumi.String("nodejs16"),
* AvailableMemoryMb: pulumi.Int(128),
* SourceArchiveBucket: bucket.Name,
* SourceArchiveObject: archive.Name,
* TriggerHttp: pulumi.Bool(true),
* EntryPoint: pulumi.String("helloGET"),
* })
* if err != nil {
* return err
* }
* // IAM entry for all users to invoke the function
* _, err = cloudfunctions.NewFunctionIamMember(ctx, "invoker", &cloudfunctions.FunctionIamMemberArgs{
* Project: function.Project,
* Region: function.Region,
* CloudFunction: function.Name,
* Role: pulumi.String("roles/cloudfunctions.invoker"),
* Member: pulumi.String("allUsers"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.storage.BucketObject;
* import com.pulumi.gcp.storage.BucketObjectArgs;
* import com.pulumi.gcp.cloudfunctions.Function;
* import com.pulumi.gcp.cloudfunctions.FunctionArgs;
* import com.pulumi.gcp.cloudfunctions.FunctionIamMember;
* import com.pulumi.gcp.cloudfunctions.FunctionIamMemberArgs;
* import com.pulumi.asset.FileAsset;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("test-bucket")
* .location("US")
* .build());
* var archive = new BucketObject("archive", BucketObjectArgs.builder()
* .name("index.zip")
* .bucket(bucket.name())
* .source(new FileAsset("./path/to/zip/file/which/contains/code"))
* .build());
* var function = new Function("function", FunctionArgs.builder()
* .name("function-test")
* .description("My function")
* .runtime("nodejs16")
* .availableMemoryMb(128)
* .sourceArchiveBucket(bucket.name())
* .sourceArchiveObject(archive.name())
* .triggerHttp(true)
* .entryPoint("helloGET")
* .build());
* // IAM entry for all users to invoke the function
* var invoker = new FunctionIamMember("invoker", FunctionIamMemberArgs.builder()
* .project(function.project())
* .region(function.region())
* .cloudFunction(function.name())
* .role("roles/cloudfunctions.invoker")
* .member("allUsers")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: test-bucket
* location: US
* archive:
* type: gcp:storage:BucketObject
* properties:
* name: index.zip
* bucket: ${bucket.name}
* source:
* fn::FileAsset: ./path/to/zip/file/which/contains/code
* function:
* type: gcp:cloudfunctions:Function
* properties:
* name: function-test
* description: My function
* runtime: nodejs16
* availableMemoryMb: 128
* sourceArchiveBucket: ${bucket.name}
* sourceArchiveObject: ${archive.name}
* triggerHttp: true
* entryPoint: helloGET
* # IAM entry for all users to invoke the function
* invoker:
* type: gcp:cloudfunctions:FunctionIamMember
* properties:
* project: ${function.project}
* region: ${function.region}
* cloudFunction: ${function.name}
* role: roles/cloudfunctions.invoker
* member: allUsers
* ```
*
* ### Single User
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "test-bucket",
* location: "US",
* });
* const archive = new gcp.storage.BucketObject("archive", {
* name: "index.zip",
* bucket: bucket.name,
* source: new pulumi.asset.FileAsset("./path/to/zip/file/which/contains/code"),
* });
* const _function = new gcp.cloudfunctions.Function("function", {
* name: "function-test",
* description: "My function",
* runtime: "nodejs16",
* availableMemoryMb: 128,
* sourceArchiveBucket: bucket.name,
* sourceArchiveObject: archive.name,
* triggerHttp: true,
* httpsTriggerSecurityLevel: "SECURE_ALWAYS",
* timeout: 60,
* entryPoint: "helloGET",
* labels: {
* "my-label": "my-label-value",
* },
* environmentVariables: {
* MY_ENV_VAR: "my-env-var-value",
* },
* });
* // IAM entry for a single user to invoke the function
* const invoker = new gcp.cloudfunctions.FunctionIamMember("invoker", {
* project: _function.project,
* region: _function.region,
* cloudFunction: _function.name,
* role: "roles/cloudfunctions.invoker",
* member: "user:[email protected]",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* bucket = gcp.storage.Bucket("bucket",
* name="test-bucket",
* location="US")
* archive = gcp.storage.BucketObject("archive",
* name="index.zip",
* bucket=bucket.name,
* source=pulumi.FileAsset("./path/to/zip/file/which/contains/code"))
* function = gcp.cloudfunctions.Function("function",
* name="function-test",
* description="My function",
* runtime="nodejs16",
* available_memory_mb=128,
* source_archive_bucket=bucket.name,
* source_archive_object=archive.name,
* trigger_http=True,
* https_trigger_security_level="SECURE_ALWAYS",
* timeout=60,
* entry_point="helloGET",
* labels={
* "my-label": "my-label-value",
* },
* environment_variables={
* "MY_ENV_VAR": "my-env-var-value",
* })
* # IAM entry for a single user to invoke the function
* invoker = gcp.cloudfunctions.FunctionIamMember("invoker",
* project=function.project,
* region=function.region,
* cloud_function=function.name,
* role="roles/cloudfunctions.invoker",
* member="user:[email protected]")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "test-bucket",
* Location = "US",
* });
* var archive = new Gcp.Storage.BucketObject("archive", new()
* {
* Name = "index.zip",
* Bucket = bucket.Name,
* Source = new FileAsset("./path/to/zip/file/which/contains/code"),
* });
* var function = new Gcp.CloudFunctions.Function("function", new()
* {
* Name = "function-test",
* Description = "My function",
* Runtime = "nodejs16",
* AvailableMemoryMb = 128,
* SourceArchiveBucket = bucket.Name,
* SourceArchiveObject = archive.Name,
* TriggerHttp = true,
* HttpsTriggerSecurityLevel = "SECURE_ALWAYS",
* Timeout = 60,
* EntryPoint = "helloGET",
* Labels =
* {
* { "my-label", "my-label-value" },
* },
* EnvironmentVariables =
* {
* { "MY_ENV_VAR", "my-env-var-value" },
* },
* });
* // IAM entry for a single user to invoke the function
* var invoker = new Gcp.CloudFunctions.FunctionIamMember("invoker", new()
* {
* Project = function.Project,
* Region = function.Region,
* CloudFunction = function.Name,
* Role = "roles/cloudfunctions.invoker",
* Member = "user:[email protected]",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudfunctions"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("test-bucket"),
* Location: pulumi.String("US"),
* })
* if err != nil {
* return err
* }
* archive, err := storage.NewBucketObject(ctx, "archive", &storage.BucketObjectArgs{
* Name: pulumi.String("index.zip"),
* Bucket: bucket.Name,
* Source: pulumi.NewFileAsset("./path/to/zip/file/which/contains/code"),
* })
* if err != nil {
* return err
* }
* function, err := cloudfunctions.NewFunction(ctx, "function", &cloudfunctions.FunctionArgs{
* Name: pulumi.String("function-test"),
* Description: pulumi.String("My function"),
* Runtime: pulumi.String("nodejs16"),
* AvailableMemoryMb: pulumi.Int(128),
* SourceArchiveBucket: bucket.Name,
* SourceArchiveObject: archive.Name,
* TriggerHttp: pulumi.Bool(true),
* HttpsTriggerSecurityLevel: pulumi.String("SECURE_ALWAYS"),
* Timeout: pulumi.Int(60),
* EntryPoint: pulumi.String("helloGET"),
* Labels: pulumi.Map{
* "my-label": pulumi.Any("my-label-value"),
* },
* EnvironmentVariables: pulumi.Map{
* "MY_ENV_VAR": pulumi.Any("my-env-var-value"),
* },
* })
* if err != nil {
* return err
* }
* // IAM entry for a single user to invoke the function
* _, err = cloudfunctions.NewFunctionIamMember(ctx, "invoker", &cloudfunctions.FunctionIamMemberArgs{
* Project: function.Project,
* Region: function.Region,
* CloudFunction: function.Name,
* Role: pulumi.String("roles/cloudfunctions.invoker"),
* Member: pulumi.String("user:[email protected]"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.storage.BucketObject;
* import com.pulumi.gcp.storage.BucketObjectArgs;
* import com.pulumi.gcp.cloudfunctions.Function;
* import com.pulumi.gcp.cloudfunctions.FunctionArgs;
* import com.pulumi.gcp.cloudfunctions.FunctionIamMember;
* import com.pulumi.gcp.cloudfunctions.FunctionIamMemberArgs;
* import com.pulumi.asset.FileAsset;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("test-bucket")
* .location("US")
* .build());
* var archive = new BucketObject("archive", BucketObjectArgs.builder()
* .name("index.zip")
* .bucket(bucket.name())
* .source(new FileAsset("./path/to/zip/file/which/contains/code"))
* .build());
* var function = new Function("function", FunctionArgs.builder()
* .name("function-test")
* .description("My function")
* .runtime("nodejs16")
* .availableMemoryMb(128)
* .sourceArchiveBucket(bucket.name())
* .sourceArchiveObject(archive.name())
* .triggerHttp(true)
* .httpsTriggerSecurityLevel("SECURE_ALWAYS")
* .timeout(60)
* .entryPoint("helloGET")
* .labels(Map.of("my-label", "my-label-value"))
* .environmentVariables(Map.of("MY_ENV_VAR", "my-env-var-value"))
* .build());
* // IAM entry for a single user to invoke the function
* var invoker = new FunctionIamMember("invoker", FunctionIamMemberArgs.builder()
* .project(function.project())
* .region(function.region())
* .cloudFunction(function.name())
* .role("roles/cloudfunctions.invoker")
* .member("user:[email protected]")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: test-bucket
* location: US
* archive:
* type: gcp:storage:BucketObject
* properties:
* name: index.zip
* bucket: ${bucket.name}
* source:
* fn::FileAsset: ./path/to/zip/file/which/contains/code
* function:
* type: gcp:cloudfunctions:Function
* properties:
* name: function-test
* description: My function
* runtime: nodejs16
* availableMemoryMb: 128
* sourceArchiveBucket: ${bucket.name}
* sourceArchiveObject: ${archive.name}
* triggerHttp: true
* httpsTriggerSecurityLevel: SECURE_ALWAYS
* timeout: 60
* entryPoint: helloGET
* labels:
* my-label: my-label-value
* environmentVariables:
* MY_ENV_VAR: my-env-var-value
* # IAM entry for a single user to invoke the function
* invoker:
* type: gcp:cloudfunctions:FunctionIamMember
* properties:
* project: ${function.project}
* region: ${function.region}
* cloudFunction: ${function.name}
* role: roles/cloudfunctions.invoker
* member: user:[email protected]
* ```
*
* ## Import
* Functions can be imported using the `name` or `{{project}}/{{region}}/name`, e.g.
* * `{{project}}/{{region}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Functions can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:cloudfunctions/function:Function default {{project}}/{{region}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:cloudfunctions/function:Function default {{name}}
* ```
*/
public class Function internal constructor(
override val javaResource: com.pulumi.gcp.cloudfunctions.Function,
) : KotlinCustomResource(javaResource, FunctionMapper) {
/**
* Memory (in MB), available to the function. Default value is `256`. Possible values include `128`, `256`, `512`, `1024`, etc.
*/
public val availableMemoryMb: Output?
get() = javaResource.availableMemoryMb().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A set of key/value environment variable pairs available during build time.
*/
public val buildEnvironmentVariables: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy