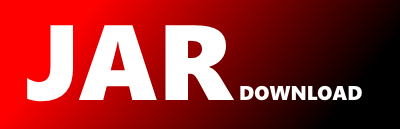
com.pulumi.gcp.cloudfunctions.kotlin.inputs.FunctionSecretVolumeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudfunctions.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudfunctions.inputs.FunctionSecretVolumeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property mountPath The path within the container to mount the secret volume. For example, setting the mount_path as "/etc/secrets" would mount the secret value files under the "/etc/secrets" directory. This directory will also be completely shadowed and unavailable to mount any other secrets. Recommended mount paths: "/etc/secrets" Restricted mount paths: "/cloudsql", "/dev/log", "/pod", "/proc", "/var/log".
* @property projectId Project identifier (due to a known limitation, only project number is supported by this field) of the project that contains the secret. If not set, it will be populated with the function's project, assuming that the secret exists in the same project as of the function.
* @property secret ID of the secret in secret manager (not the full resource name).
* @property versions List of secret versions to mount for this secret. If empty, the "latest" version of the secret will be made available in a file named after the secret under the mount point. Structure is documented below.
*/
public data class FunctionSecretVolumeArgs(
public val mountPath: Output,
public val projectId: Output? = null,
public val secret: Output,
public val versions: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudfunctions.inputs.FunctionSecretVolumeArgs =
com.pulumi.gcp.cloudfunctions.inputs.FunctionSecretVolumeArgs.builder()
.mountPath(mountPath.applyValue({ args0 -> args0 }))
.projectId(projectId?.applyValue({ args0 -> args0 }))
.secret(secret.applyValue({ args0 -> args0 }))
.versions(
versions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [FunctionSecretVolumeArgs].
*/
@PulumiTagMarker
public class FunctionSecretVolumeArgsBuilder internal constructor() {
private var mountPath: Output? = null
private var projectId: Output? = null
private var secret: Output? = null
private var versions: Output>? = null
/**
* @param value The path within the container to mount the secret volume. For example, setting the mount_path as "/etc/secrets" would mount the secret value files under the "/etc/secrets" directory. This directory will also be completely shadowed and unavailable to mount any other secrets. Recommended mount paths: "/etc/secrets" Restricted mount paths: "/cloudsql", "/dev/log", "/pod", "/proc", "/var/log".
*/
@JvmName("ksywtksyvxtfwcng")
public suspend fun mountPath(`value`: Output) {
this.mountPath = value
}
/**
* @param value Project identifier (due to a known limitation, only project number is supported by this field) of the project that contains the secret. If not set, it will be populated with the function's project, assuming that the secret exists in the same project as of the function.
*/
@JvmName("fvkgpkhtlgwytkcg")
public suspend fun projectId(`value`: Output) {
this.projectId = value
}
/**
* @param value ID of the secret in secret manager (not the full resource name).
*/
@JvmName("qoroqimvlmsriqeg")
public suspend fun secret(`value`: Output) {
this.secret = value
}
/**
* @param value List of secret versions to mount for this secret. If empty, the "latest" version of the secret will be made available in a file named after the secret under the mount point. Structure is documented below.
*/
@JvmName("afvfemtichvfglau")
public suspend fun versions(`value`: Output>) {
this.versions = value
}
@JvmName("ecneuktfvwkyhbdm")
public suspend fun versions(vararg values: Output) {
this.versions = Output.all(values.asList())
}
/**
* @param values List of secret versions to mount for this secret. If empty, the "latest" version of the secret will be made available in a file named after the secret under the mount point. Structure is documented below.
*/
@JvmName("vrhagvxphbrxojba")
public suspend fun versions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy