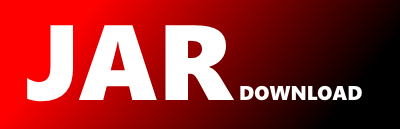
com.pulumi.gcp.cloudfunctions.kotlin.outputs.GetFunctionResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudfunctions.kotlin.outputs
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getFunction.
* @property availableMemoryMb Available memory (in MB) to the function.
* @property buildEnvironmentVariables
* @property buildWorkerPool
* @property description Description of the function.
* @property dockerRegistry
* @property dockerRepository
* @property effectiveLabels
* @property entryPoint Name of a JavaScript function that will be executed when the Google Cloud Function is triggered.
* @property environmentVariables
* @property eventTriggers A source that fires events in response to a condition in another service. Structure is documented below.
* @property httpsTriggerSecurityLevel
* @property httpsTriggerUrl If function is triggered by HTTP, trigger URL is set here.
* @property id The provider-assigned unique ID for this managed resource.
* @property ingressSettings Controls what traffic can reach the function.
* @property kmsKeyName
* @property labels All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
* @property maxInstances The limit on the maximum number of function instances that may coexist at a given time. If unset or set to `0`, the API default will be used.
* @property minInstances
* @property name The name of the Cloud Function.
* @property project
* @property pulumiLabels
* @property region
* @property runtime The runtime in which the function is running.
* @property secretEnvironmentVariables
* @property secretVolumes
* @property serviceAccountEmail The service account email to be assumed by the cloud function.
* @property sourceArchiveBucket The GCS bucket containing the zip archive which contains the function.
* @property sourceArchiveObject The source archive object (file) in archive bucket.
* @property sourceRepositories The URL of the Cloud Source Repository that the function is deployed from. Structure is documented below.
* @property status
* @property timeout Function execution timeout (in seconds).
* @property triggerHttp If function is triggered by HTTP, this boolean is set.
* @property versionId
* @property vpcConnector The VPC Network Connector that this cloud function can connect to.
* @property vpcConnectorEgressSettings The egress settings for the connector, controlling what traffic is diverted through it.
*/
public data class GetFunctionResult(
public val availableMemoryMb: Int,
public val buildEnvironmentVariables: Map,
public val buildWorkerPool: String,
public val description: String,
public val dockerRegistry: String,
public val dockerRepository: String,
public val effectiveLabels: Map,
public val entryPoint: String,
public val environmentVariables: Map,
public val eventTriggers: List,
public val httpsTriggerSecurityLevel: String,
public val httpsTriggerUrl: String,
public val id: String,
public val ingressSettings: String,
public val kmsKeyName: String,
public val labels: Map,
public val maxInstances: Int,
public val minInstances: Int,
public val name: String,
public val project: String? = null,
public val pulumiLabels: Map,
public val region: String? = null,
public val runtime: String,
public val secretEnvironmentVariables: List,
public val secretVolumes: List,
public val serviceAccountEmail: String,
public val sourceArchiveBucket: String,
public val sourceArchiveObject: String,
public val sourceRepositories: List,
public val status: String,
public val timeout: Int,
public val triggerHttp: Boolean,
public val versionId: String,
public val vpcConnector: String,
public val vpcConnectorEgressSettings: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudfunctions.outputs.GetFunctionResult): GetFunctionResult = GetFunctionResult(
availableMemoryMb = javaType.availableMemoryMb(),
buildEnvironmentVariables = javaType.buildEnvironmentVariables().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
buildWorkerPool = javaType.buildWorkerPool(),
description = javaType.description(),
dockerRegistry = javaType.dockerRegistry(),
dockerRepository = javaType.dockerRepository(),
effectiveLabels = javaType.effectiveLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
entryPoint = javaType.entryPoint(),
environmentVariables = javaType.environmentVariables().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
eventTriggers = javaType.eventTriggers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudfunctions.kotlin.outputs.GetFunctionEventTrigger.Companion.toKotlin(args0)
})
}),
httpsTriggerSecurityLevel = javaType.httpsTriggerSecurityLevel(),
httpsTriggerUrl = javaType.httpsTriggerUrl(),
id = javaType.id(),
ingressSettings = javaType.ingressSettings(),
kmsKeyName = javaType.kmsKeyName(),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
maxInstances = javaType.maxInstances(),
minInstances = javaType.minInstances(),
name = javaType.name(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
pulumiLabels = javaType.pulumiLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
region = javaType.region().map({ args0 -> args0 }).orElse(null),
runtime = javaType.runtime(),
secretEnvironmentVariables = javaType.secretEnvironmentVariables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudfunctions.kotlin.outputs.GetFunctionSecretEnvironmentVariable.Companion.toKotlin(args0)
})
}),
secretVolumes = javaType.secretVolumes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudfunctions.kotlin.outputs.GetFunctionSecretVolume.Companion.toKotlin(args0)
})
}),
serviceAccountEmail = javaType.serviceAccountEmail(),
sourceArchiveBucket = javaType.sourceArchiveBucket(),
sourceArchiveObject = javaType.sourceArchiveObject(),
sourceRepositories = javaType.sourceRepositories().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudfunctions.kotlin.outputs.GetFunctionSourceRepository.Companion.toKotlin(args0)
})
}),
status = javaType.status(),
timeout = javaType.timeout(),
triggerHttp = javaType.triggerHttp(),
versionId = javaType.versionId(),
vpcConnector = javaType.vpcConnector(),
vpcConnectorEgressSettings = javaType.vpcConnectorEgressSettings(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy