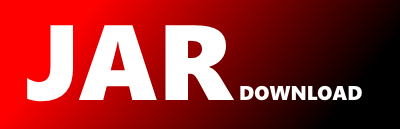
com.pulumi.gcp.cloudfunctionsv2.kotlin.inputs.FunctionBuildConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudfunctionsv2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudfunctionsv2.inputs.FunctionBuildConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property build (Output)
* The Cloud Build name of the latest successful
* deployment of the function.
* @property dockerRepository User managed repository created in Artifact Registry optionally with a customer managed encryption key.
* @property entryPoint The name of the function (as defined in source code) that will be executed.
* Defaults to the resource name suffix, if not specified. For backward
* compatibility, if function with given name is not found, then the system
* will try to use function named "function". For Node.js this is name of a
* function exported by the module specified in source_location.
* @property environmentVariables User-provided build-time environment variables for the function.
* @property runtime The runtime in which to run the function. Required when deploying a new
* function, optional when updating an existing function.
* @property serviceAccount The fully-qualified name of the service account to be used for building the container.
* @property source The location of the function source code.
* Structure is documented below.
* @property workerPool Name of the Cloud Build Custom Worker Pool that should be used to build the function.
*/
public data class FunctionBuildConfigArgs(
public val build: Output? = null,
public val dockerRepository: Output? = null,
public val entryPoint: Output? = null,
public val environmentVariables: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy