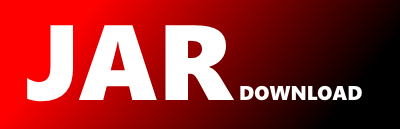
com.pulumi.gcp.cloudfunctionsv2.kotlin.inputs.FunctionEventTriggerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudfunctionsv2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudfunctionsv2.inputs.FunctionEventTriggerArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property eventFilters Criteria used to filter events.
* Structure is documented below.
* @property eventType Required. The type of event to observe.
* @property pubsubTopic The name of a Pub/Sub topic in the same project that will be used
* as the transport topic for the event delivery.
* @property retryPolicy Describes the retry policy in case of function's execution failure.
* Retried execution is charged as any other execution.
* Possible values are: `RETRY_POLICY_UNSPECIFIED`, `RETRY_POLICY_DO_NOT_RETRY`, `RETRY_POLICY_RETRY`.
* @property serviceAccountEmail Optional. The email of the trigger's service account. The service account
* must have permission to invoke Cloud Run services. If empty, defaults to the
* Compute Engine default service account: {project_number}[email protected].
* @property trigger (Output)
* Output only. The resource name of the Eventarc trigger.
* @property triggerRegion The region that the trigger will be in. The trigger will only receive
* events originating in this region. It can be the same
* region as the function, a different region or multi-region, or the global
* region. If not provided, defaults to the same region as the function.
*/
public data class FunctionEventTriggerArgs(
public val eventFilters: Output>? = null,
public val eventType: Output? = null,
public val pubsubTopic: Output? = null,
public val retryPolicy: Output? = null,
public val serviceAccountEmail: Output? = null,
public val trigger: Output? = null,
public val triggerRegion: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudfunctionsv2.inputs.FunctionEventTriggerArgs =
com.pulumi.gcp.cloudfunctionsv2.inputs.FunctionEventTriggerArgs.builder()
.eventFilters(
eventFilters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.eventType(eventType?.applyValue({ args0 -> args0 }))
.pubsubTopic(pubsubTopic?.applyValue({ args0 -> args0 }))
.retryPolicy(retryPolicy?.applyValue({ args0 -> args0 }))
.serviceAccountEmail(serviceAccountEmail?.applyValue({ args0 -> args0 }))
.trigger(trigger?.applyValue({ args0 -> args0 }))
.triggerRegion(triggerRegion?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FunctionEventTriggerArgs].
*/
@PulumiTagMarker
public class FunctionEventTriggerArgsBuilder internal constructor() {
private var eventFilters: Output>? = null
private var eventType: Output? = null
private var pubsubTopic: Output? = null
private var retryPolicy: Output? = null
private var serviceAccountEmail: Output? = null
private var trigger: Output? = null
private var triggerRegion: Output? = null
/**
* @param value Criteria used to filter events.
* Structure is documented below.
*/
@JvmName("eiedvnolrtdblltd")
public suspend fun eventFilters(`value`: Output>) {
this.eventFilters = value
}
@JvmName("mnaathehyucuyvlu")
public suspend fun eventFilters(vararg values: Output) {
this.eventFilters = Output.all(values.asList())
}
/**
* @param values Criteria used to filter events.
* Structure is documented below.
*/
@JvmName("rbtauotqyrqmjvid")
public suspend fun eventFilters(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy