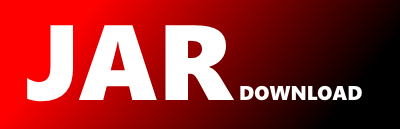
com.pulumi.gcp.cloudfunctionsv2.kotlin.outputs.FunctionServiceConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudfunctionsv2.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property allTrafficOnLatestRevision Whether 100% of traffic is routed to the latest revision. Defaults to true.
* @property availableCpu The number of CPUs used in a single container instance. Default value is calculated from available memory.
* @property availableMemory The amount of memory available for a function.
* Defaults to 256M. Supported units are k, M, G, Mi, Gi. If no unit is
* supplied the value is interpreted as bytes.
* @property environmentVariables Environment variables that shall be available during function execution.
* @property gcfUri (Output)
* URIs of the Service deployed
* @property ingressSettings Available ingress settings. Defaults to "ALLOW_ALL" if unspecified.
* Default value is `ALLOW_ALL`.
* Possible values are: `ALLOW_ALL`, `ALLOW_INTERNAL_ONLY`, `ALLOW_INTERNAL_AND_GCLB`.
* @property maxInstanceCount The limit on the maximum number of function instances that may coexist at a
* given time.
* @property maxInstanceRequestConcurrency Sets the maximum number of concurrent requests that each instance can receive. Defaults to 1.
* @property minInstanceCount The limit on the minimum number of function instances that may coexist at a
* given time.
* @property secretEnvironmentVariables Secret environment variables configuration.
* Structure is documented below.
* @property secretVolumes Secret volumes configuration.
* Structure is documented below.
* @property service Name of the service associated with a Function.
* @property serviceAccountEmail The email of the service account for this function.
* @property timeoutSeconds The function execution timeout. Execution is considered failed and
* can be terminated if the function is not completed at the end of the
* timeout period. Defaults to 60 seconds.
* @property uri (Output)
* URI of the Service deployed.
* @property vpcConnector The Serverless VPC Access connector that this cloud function can connect to.
* @property vpcConnectorEgressSettings Available egress settings.
* Possible values are: `VPC_CONNECTOR_EGRESS_SETTINGS_UNSPECIFIED`, `PRIVATE_RANGES_ONLY`, `ALL_TRAFFIC`.
*/
public data class FunctionServiceConfig(
public val allTrafficOnLatestRevision: Boolean? = null,
public val availableCpu: String? = null,
public val availableMemory: String? = null,
public val environmentVariables: Map? = null,
public val gcfUri: String? = null,
public val ingressSettings: String? = null,
public val maxInstanceCount: Int? = null,
public val maxInstanceRequestConcurrency: Int? = null,
public val minInstanceCount: Int? = null,
public val secretEnvironmentVariables: List? =
null,
public val secretVolumes: List? = null,
public val service: String? = null,
public val serviceAccountEmail: String? = null,
public val timeoutSeconds: Int? = null,
public val uri: String? = null,
public val vpcConnector: String? = null,
public val vpcConnectorEgressSettings: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudfunctionsv2.outputs.FunctionServiceConfig): FunctionServiceConfig = FunctionServiceConfig(
allTrafficOnLatestRevision = javaType.allTrafficOnLatestRevision().map({ args0 ->
args0
}).orElse(null),
availableCpu = javaType.availableCpu().map({ args0 -> args0 }).orElse(null),
availableMemory = javaType.availableMemory().map({ args0 -> args0 }).orElse(null),
environmentVariables = javaType.environmentVariables().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
gcfUri = javaType.gcfUri().map({ args0 -> args0 }).orElse(null),
ingressSettings = javaType.ingressSettings().map({ args0 -> args0 }).orElse(null),
maxInstanceCount = javaType.maxInstanceCount().map({ args0 -> args0 }).orElse(null),
maxInstanceRequestConcurrency = javaType.maxInstanceRequestConcurrency().map({ args0 ->
args0
}).orElse(null),
minInstanceCount = javaType.minInstanceCount().map({ args0 -> args0 }).orElse(null),
secretEnvironmentVariables = javaType.secretEnvironmentVariables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudfunctionsv2.kotlin.outputs.FunctionServiceConfigSecretEnvironmentVariable.Companion.toKotlin(args0)
})
}),
secretVolumes = javaType.secretVolumes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudfunctionsv2.kotlin.outputs.FunctionServiceConfigSecretVolume.Companion.toKotlin(args0)
})
}),
service = javaType.service().map({ args0 -> args0 }).orElse(null),
serviceAccountEmail = javaType.serviceAccountEmail().map({ args0 -> args0 }).orElse(null),
timeoutSeconds = javaType.timeoutSeconds().map({ args0 -> args0 }).orElse(null),
uri = javaType.uri().map({ args0 -> args0 }).orElse(null),
vpcConnector = javaType.vpcConnector().map({ args0 -> args0 }).orElse(null),
vpcConnectorEgressSettings = javaType.vpcConnectorEgressSettings().map({ args0 ->
args0
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy