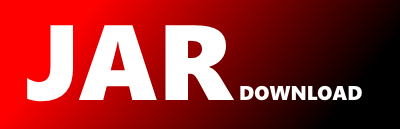
com.pulumi.gcp.cloudidentity.kotlin.CloudidentityFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudidentity.kotlin
import com.pulumi.gcp.cloudidentity.CloudidentityFunctions.getGroupLookupPlain
import com.pulumi.gcp.cloudidentity.CloudidentityFunctions.getGroupMembershipsPlain
import com.pulumi.gcp.cloudidentity.CloudidentityFunctions.getGroupsPlain
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupLookupGroupKey
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupLookupPlainArgs
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupLookupPlainArgsBuilder
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupMembershipsPlainArgs
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupMembershipsPlainArgsBuilder
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupsPlainArgs
import com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupsPlainArgsBuilder
import com.pulumi.gcp.cloudidentity.kotlin.outputs.GetGroupLookupResult
import com.pulumi.gcp.cloudidentity.kotlin.outputs.GetGroupMembershipsResult
import com.pulumi.gcp.cloudidentity.kotlin.outputs.GetGroupsResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.gcp.cloudidentity.kotlin.outputs.GetGroupLookupResult.Companion.toKotlin as getGroupLookupResultToKotlin
import com.pulumi.gcp.cloudidentity.kotlin.outputs.GetGroupMembershipsResult.Companion.toKotlin as getGroupMembershipsResultToKotlin
import com.pulumi.gcp.cloudidentity.kotlin.outputs.GetGroupsResult.Companion.toKotlin as getGroupsResultToKotlin
public object CloudidentityFunctions {
/**
* Use this data source to look up the resource name of a Cloud Identity Group by its [EntityKey](https://cloud.google.com/identity/docs/reference/rest/v1/EntityKey), i.e. the group's email.
* https://cloud.google.com/identity/docs/concepts/overview#groups
* ## Example Usage
* ```tf
* data "google_cloud_identity_group_lookup" "group" {
* group_key {
* id = "[email protected]"
* }
* }
* ```
* @param argument A collection of arguments for invoking getGroupLookup.
* @return A collection of values returned by getGroupLookup.
*/
public suspend fun getGroupLookup(argument: GetGroupLookupPlainArgs): GetGroupLookupResult =
getGroupLookupResultToKotlin(getGroupLookupPlain(argument.toJava()).await())
/**
* @see [getGroupLookup].
* @param groupKey The EntityKey of the Group to lookup. A unique identifier for an entity in the Cloud Identity Groups API.
* An entity can represent either a group with an optional namespace or a user without a namespace.
* The combination of id and namespace must be unique; however, the same id can be used with different namespaces. Structure is documented below.
* @return A collection of values returned by getGroupLookup.
*/
public suspend fun getGroupLookup(groupKey: GetGroupLookupGroupKey): GetGroupLookupResult {
val argument = GetGroupLookupPlainArgs(
groupKey = groupKey,
)
return getGroupLookupResultToKotlin(getGroupLookupPlain(argument.toJava()).await())
}
/**
* @see [getGroupLookup].
* @param argument Builder for [com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupLookupPlainArgs].
* @return A collection of values returned by getGroupLookup.
*/
public suspend fun getGroupLookup(argument: suspend GetGroupLookupPlainArgsBuilder.() -> Unit): GetGroupLookupResult {
val builder = GetGroupLookupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGroupLookupResultToKotlin(getGroupLookupPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to get list of the Cloud Identity Group Memberships within a given Group.
* https://cloud.google.com/identity/docs/concepts/overview#memberships
* ## Example Usage
* ```tf
* data "google_cloud_identity_group_memberships" "members" {
* group = "groups/123eab45c6defghi"
* }
* ```
* @param argument A collection of arguments for invoking getGroupMemberships.
* @return A collection of values returned by getGroupMemberships.
*/
public suspend fun getGroupMemberships(argument: GetGroupMembershipsPlainArgs): GetGroupMembershipsResult =
getGroupMembershipsResultToKotlin(getGroupMembershipsPlain(argument.toJava()).await())
/**
* @see [getGroupMemberships].
* @param group The parent Group resource under which to lookup the Membership names. Must be of the form groups/{group_id}.
* @return A collection of values returned by getGroupMemberships.
*/
public suspend fun getGroupMemberships(group: String): GetGroupMembershipsResult {
val argument = GetGroupMembershipsPlainArgs(
group = group,
)
return getGroupMembershipsResultToKotlin(getGroupMembershipsPlain(argument.toJava()).await())
}
/**
* @see [getGroupMemberships].
* @param argument Builder for [com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupMembershipsPlainArgs].
* @return A collection of values returned by getGroupMemberships.
*/
public suspend fun getGroupMemberships(argument: suspend GetGroupMembershipsPlainArgsBuilder.() -> Unit): GetGroupMembershipsResult {
val builder = GetGroupMembershipsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGroupMembershipsResultToKotlin(getGroupMembershipsPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to get list of the Cloud Identity Groups under a customer or namespace.
* https://cloud.google.com/identity/docs/concepts/overview#groups
* ## Example Usage
* ```tf
* data "google_cloud_identity_groups" "groups" {
* parent = "customers/A01b123xz"
* }
* ```
* @param argument A collection of arguments for invoking getGroups.
* @return A collection of values returned by getGroups.
*/
public suspend fun getGroups(argument: GetGroupsPlainArgs): GetGroupsResult =
getGroupsResultToKotlin(getGroupsPlain(argument.toJava()).await())
/**
* @see [getGroups].
* @param parent The parent resource under which to list all Groups. Must be of the form identitysources/{identity_source_id} for external- identity-mapped groups or customers/{customer_id} for Google Groups.
* @return A collection of values returned by getGroups.
*/
public suspend fun getGroups(parent: String): GetGroupsResult {
val argument = GetGroupsPlainArgs(
parent = parent,
)
return getGroupsResultToKotlin(getGroupsPlain(argument.toJava()).await())
}
/**
* @see [getGroups].
* @param argument Builder for [com.pulumi.gcp.cloudidentity.kotlin.inputs.GetGroupsPlainArgs].
* @return A collection of values returned by getGroups.
*/
public suspend fun getGroups(argument: suspend GetGroupsPlainArgsBuilder.() -> Unit): GetGroupsResult {
val builder = GetGroupsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGroupsResultToKotlin(getGroupsPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy